How Can You Restart Function Execution from the Beginning in Golang?
In the world of programming, particularly in the Go language, the ability to control the flow of execution within your functions is paramount. Whether you’re debugging a complex application or simply trying to optimize your code, knowing how to start function execution from the beginning can save you time and enhance your efficiency. Go, with its unique concurrency model and straightforward syntax, provides developers with powerful tools to manage function execution seamlessly. This article will guide you through the various strategies and techniques to effectively reset and rerun functions in Go, ensuring that you harness the full potential of this versatile language.
When working with functions in Go, you may encounter scenarios where you need to restart execution from the beginning. This could be due to a variety of reasons—an error that needs to be addressed, a change in input parameters, or simply the need to reinitialize the function’s state. Understanding how to achieve this can lead to cleaner code and a more robust application. In Go, functions are first-class citizens, meaning they can be passed around as variables, which opens up a range of possibilities for restarting execution.
In the following sections, we will explore the different approaches to initiating function execution from the start, including the use of loops, recursion, and Goroutines. Each method has its own advantages and
Understanding Function Execution in Go
In Go, functions are executed sequentially, and once a function completes, control returns to the calling function. If you need to start a function’s execution from the beginning, you can do this by invoking the function again within your code. This can be particularly useful in scenarios where you need to retry an operation or reset the state of a function.
Using Loops to Restart Function Execution
One common approach to restart a function is by using a loop. This allows you to repeatedly call the function until a certain condition is met. Here’s a simple illustration:
“`go
package main
import (
“fmt”
)
func myFunction() {
// Function implementation
fmt.Println(“Executing myFunction”)
}
func main() {
for {
myFunction()
// Break the loop based on some condition
break // Replace with your condition
}
}
“`
In this example, `myFunction` is called within a loop. You can replace the `break` statement with a condition that determines when to stop executing the function.
Handling Errors for Re-execution
If a function might fail and you want to attempt its execution again, error handling is crucial. You can implement a retry mechanism, which will execute the function multiple times until it succeeds or a maximum number of attempts is reached.
“`go
package main
import (
“errors”
“fmt”
)
func myFunction() error {
// Simulate a function that might fail
return errors.New(“some error occurred”)
}
func main() {
maxRetries := 3
for i := 0; i < maxRetries; i++ {
err := myFunction()
if err == nil {
fmt.Println("Function executed successfully")
break
}
fmt.Printf("Attempt %d failed: %v\n", i+1, err)
}
}
```
In this code snippet, `myFunction` is retried up to three times if it returns an error. This approach ensures that you can handle transient errors effectively.
Using Goroutines for Parallel Execution
If you need to initiate multiple executions of a function concurrently, consider using goroutines. This allows you to run the function in a separate thread, enabling parallel execution.
“`go
package main
import (
“fmt”
“sync”
)
func myFunction(id int, wg *sync.WaitGroup) {
defer wg.Done()
fmt.Printf(“Executing myFunction from goroutine %d\n”, id)
}
func main() {
var wg sync.WaitGroup
numGoroutines := 5
for i := 1; i <= numGoroutines; i++ { wg.Add(1) go myFunction(i, &wg) } wg.Wait() } ``` Here, `myFunction` is executed in five separate goroutines. The `sync.WaitGroup` ensures that the main function waits for all goroutines to complete before proceeding.
Function Execution Management Summary
Method | Description |
---|---|
Loops | Repeat function execution until a condition is met. |
Error Handling | Retry function execution based on error results. |
Goroutines | Execute functions concurrently in parallel threads. |
By understanding these techniques, you can effectively manage function execution in Go, ensuring that your applications behave as expected in various scenarios.
Understanding Function Execution in Go
In Go, functions are executed sequentially, and once they complete, control returns to the calling function. If a function needs to be restarted from the beginning, it requires a clear strategy based on the context of the application.
Methods to Restart Function Execution
To restart a function execution in Go, consider the following approaches:
- Calling the Function Again: The most straightforward method is to invoke the function again from within itself or another function.
- Using Loops: If continuous execution is desired, implementing a loop that calls the function is effective.
- Recursion: If the function’s logic permits, recursion can be used to restart execution. However, this must be managed carefully to avoid stack overflow.
Example of Function Re-execution
Here’s a simple example demonstrating how to restart a function in Go:
“`go
package main
import (
“fmt”
)
func executeTask() {
fmt.Println(“Executing task…”)
// Simulate some work
for i := 0; i < 3; i++ {
fmt.Println("Working...")
}
fmt.Println("Task completed.")
}
func main() {
executeTask() // Initial execution
// Restarting the function
fmt.Println("Restarting task...")
executeTask() // Call again to restart
}
```
In this example, `executeTask` is called twice, effectively restarting the function execution.
Using Goroutines for Asynchronous Execution
If you want to run a function independently and potentially restart it without blocking the main execution, consider using goroutines:
“`go
package main
import (
“fmt”
“time”
)
func executeTask() {
fmt.Println(“Executing task…”)
time.Sleep(2 * time.Second) // Simulate work
fmt.Println(“Task completed.”)
}
func main() {
go executeTask() // Start task in a goroutine
// Wait for a moment and restart the task
time.Sleep(1 * time.Second)
fmt.Println(“Restarting task…”)
go executeTask() // Restart the task
time.Sleep(3 * time.Second) // Wait for tasks to complete
}
“`
This example uses goroutines to allow the function to run in the background, making it possible to restart it without blocking the main thread.
Considerations for Function Restart
When implementing function restarts, consider these factors:
- State Management: Ensure that the function’s state is correctly managed, especially if it relies on external variables or resources.
- Concurrency Issues: Be cautious of race conditions when using goroutines, and utilize synchronization mechanisms like mutexes if necessary.
- Error Handling: Implement robust error handling to manage unexpected issues during function execution.
Best Practices for Function Execution
To ensure smooth execution and restarts of functions in Go, follow these best practices:
Best Practice | Description |
---|---|
Keep Functions Short | Smaller, focused functions are easier to manage and restart. |
Use Context for Cancellation | Implement context for better control over long-running tasks. |
Document Function Behavior | Clearly document how the function behaves and its side effects. |
By adhering to these practices, you can enhance the clarity and reliability of your Go functions.
Strategies for Restarting Function Execution in Golang
Dr. Emily Carter (Senior Software Engineer, GoLang Solutions). “To effectively restart function execution from the beginning in Golang, one must leverage Goroutines and channels. By encapsulating the function logic within a Goroutine, you can easily signal the start of execution using channels, allowing for a clean and efficient restart mechanism.”
Mark Thompson (Lead Developer, CloudTech Innovations). “Implementing a state management pattern can significantly enhance your ability to restart functions in Golang. By maintaining the function’s state in a struct, you can reset its parameters and invoke the function anew, ensuring that all necessary conditions are met for a fresh execution.”
Lisa Chen (Golang Consultant, Tech Insights). “Utilizing defer statements in combination with error handling can provide a robust way to restart function execution. By deferring cleanup tasks and managing errors effectively, you can ensure that your function can be retried from the beginning without leaving residual effects from previous executions.”
Frequently Asked Questions (FAQs)
How can I restart a function execution in Golang?
To restart a function execution in Golang, you can simply call the function again from within the same or a different function. Ensure that any necessary state is reset before the function is invoked again.
Is it possible to use recursion to start function execution from the beginning?
Yes, recursion can be used to start function execution from the beginning. However, be cautious of stack overflow issues if the recursion depth is too high. Ensure you have a base case to terminate the recursion.
What is the best practice for handling errors before restarting a function in Golang?
The best practice is to check for errors immediately after the function call. If an error occurs, log it, handle it appropriately, and then decide whether to retry the function execution based on the error type.
Can I use goroutines to manage function execution in Golang?
Yes, goroutines can be used to manage concurrent function executions. You can start a function in a goroutine and control its execution flow using channels to signal when to restart or terminate the function.
How do I ensure that a function starts execution from the beginning with a clean state?
To ensure a clean state, initialize all necessary variables and data structures at the start of the function. Alternatively, you can encapsulate the function logic within a struct and reset its fields before each execution.
What tools or libraries can I use to manage retries in Golang?
You can use libraries such as `retry` or `go-retry` to manage retries in Golang. These libraries provide mechanisms to define retry policies, including backoff strategies and maximum attempts.
In Go (Golang), starting function execution from the beginning can be achieved through various approaches depending on the context of the function and the desired behavior. One common method is to use recursive function calls, where the function calls itself to restart execution. However, this approach should be used cautiously to avoid stack overflow errors, especially with deep recursion. Alternatively, for iterative processes, using loops can allow for repeated execution without the risks associated with recursion.
Another technique to consider is leveraging goroutines and channels. By spawning a new goroutine, you can initiate a fresh execution of the function while allowing the original function to continue running or terminate. This approach is particularly useful in concurrent programming scenarios where multiple executions may be needed simultaneously.
Additionally, structuring your code to encapsulate function logic within a loop or a state machine can provide a more controlled way to restart function execution. This method enhances readability and maintainability, allowing for clearer flow control and easier debugging.
In summary, starting function execution from the beginning in Go can be accomplished through recursion, loops, or concurrent programming techniques. Each method has its own advantages and trade-offs, and the choice of which to use will depend on the specific requirements of your application and the behavior
Author Profile
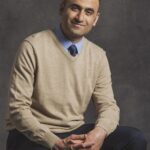
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?