How Can You Effectively Stop an Infinite Loop in Python?
Infinite loops can be a programmer’s worst nightmare, often leading to unresponsive applications and frustrating debugging sessions. Whether you’re a seasoned developer or just starting your journey in Python, encountering an infinite loop can disrupt your workflow and hinder your progress. Understanding how to stop an infinite loop is not only crucial for maintaining the efficiency of your code but also for honing your skills as a programmer. In this article, we will explore the causes of infinite loops in Python, effective strategies for halting them, and best practices to prevent them from occurring in the first place.
Infinite loops typically arise from logical errors in your code, where the termination condition is never met. This can happen due to various reasons, such as incorrect loop conditions, misplaced break statements, or even user input that fails to trigger the exit condition. Recognizing these pitfalls is essential for any developer, as it allows you to troubleshoot and resolve issues quickly, ensuring your programs run smoothly.
While stopping an infinite loop may seem daunting, there are several techniques at your disposal. From using keyboard interrupts to employing debugging tools, you can regain control of your program and prevent potential data loss. Moreover, understanding how to identify and fix the root cause of these loops will empower you to write cleaner, more efficient code in the future.
Identifying Infinite Loops
Infinite loops in Python occur when the termination condition is never met, causing the program to run endlessly. To effectively stop an infinite loop, it is crucial first to identify its presence within your code. Common signs include:
- High CPU usage
- Unresponsive program behavior
- Excessive output in the console
To diagnose an infinite loop, you can use print statements or logging to determine if the loop is executing as intended. For example, if you have a loop that processes items and the output is static, it may indicate that the loop condition is not changing.
Using Keyboard Interrupts
If you find yourself running an infinite loop during program execution, one of the quickest methods to stop it is by using a keyboard interrupt. This can be done by:
- Pressing `Ctrl + C` in the terminal or command prompt
- Closing the program window if it’s running in an IDE
This sends a signal to the Python interpreter to stop the execution of the current script.
Debugging Techniques
Utilizing debugging tools can help you identify the root cause of an infinite loop. Here are some techniques:
- Step-through Debugging: Use an IDE with debugging capabilities to step through your code line by line.
- Conditional Breakpoints: Set breakpoints that only trigger when certain conditions are met, helping you pinpoint where the loop fails to exit.
- Stack Traces: If an error occurs, examining the stack trace can provide insights into where the loop is getting stuck.
Common Causes of Infinite Loops
Understanding what leads to infinite loops can help prevent them in the future. Some common causes include:
- Incorrect Loop Conditions: Using an improper comparison operator or logic that never evaluates to .
- Improper Updates to Loop Variables: Failing to modify loop variables within the loop body.
- External Dependencies: Relying on input from users or external systems that may not change as expected.
Cause | Description | Example |
---|---|---|
Incorrect Loop Conditions | Conditions that are always true | while True: pass |
Improper Updates | Not updating the loop variable | i = 0; while i < 10: pass |
External Dependencies | Waiting for user input that never comes | while user_input != “exit”: pass |
Preventing Infinite Loops
To avoid the occurrence of infinite loops, consider implementing the following strategies:
- Set a Maximum Iteration Count: Limit the number of iterations within your loop to prevent it from running indefinitely.
- Use Timeout Mechanisms: Introduce a timeout that breaks the loop if it runs for too long.
- Review Logic Thoroughly: Before executing loops, ensure that the logic is sound and that all conditions will eventually be met.
By incorporating these practices, you can enhance the reliability of your code and mitigate the risks associated with infinite loops.
Understanding Infinite Loops in Python
An infinite loop occurs when a loop continues to execute indefinitely due to a condition that never evaluates to . In Python, this can happen with constructs such as `while` loops, `for` loops, or recursive function calls.
Common causes include:
- Incorrect loop condition: The termination condition is never met.
- Mismanagement of loop variables: Variables that control the loop’s execution are not updated correctly.
- External factors: Waiting for user input or external data that never arrives.
Identifying an Infinite Loop
To diagnose an infinite loop in your Python code, consider the following strategies:
- Print Debugging: Insert print statements to track the flow of execution and variable values.
“`python
while True:
print(“Looping…”)
“`
- Use a Debugger: Utilize debugging tools (like `pdb`) to step through the code and inspect the loop conditions in real-time.
- Logging: Implement logging to capture more detailed information about the program’s execution.
Stopping an Infinite Loop in a Running Program
If you find yourself in an infinite loop while executing a script, you can halt its execution using:
- Keyboard Interrupt: Press `Ctrl + C` in the terminal or command prompt to stop the running program.
- Task Manager: On Windows, use Task Manager (Ctrl + Shift + Esc) to end the Python process. On macOS, use Activity Monitor to do the same.
Preventing Infinite Loops in Code
To avoid infinite loops in the first place, consider implementing best practices:
- Set Loop Limits: Include a maximum number of iterations as a safeguard.
“`python
max_iterations = 1000
count = 0
while count < max_iterations:
Your loop code
count += 1
```
- Validate Conditions: Ensure that the conditions used for loop termination are logically sound and will eventually be met.
- Use Break Statements: Implement `break` statements to exit loops when certain criteria are met.
“`python
while True:
user_input = input(“Type ‘exit’ to quit: “)
if user_input == ‘exit’:
break
“`
Common Patterns Leading to Infinite Loops
Understanding common coding patterns that can lead to infinite loops can help in avoiding them:
Pattern | Description |
---|---|
`while True` without exit | Using a perpetual loop without a clear exit condition. |
`for` loop with incorrect range | Providing an incorrect range that does not allow the loop to terminate. |
Recursive functions | Failing to reach a base case in recursive calls can lead to infinite recursion. |
Using Try-Except for Handling Unexpected Infinite Loops
In cases where an infinite loop might be caused by unpredictable factors, wrapping the loop in a `try-except` block can help manage exceptions gracefully.
“`python
try:
while True:
Code that may cause an infinite loop
except KeyboardInterrupt:
print(“Loop terminated by user.”)
“`
This approach allows for a graceful exit and can help in maintaining the stability of the program.
Expert Strategies for Halting Infinite Loops in Python
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “To effectively stop an infinite loop in Python, one must first identify the loop’s termination condition. Implementing a timeout mechanism or using a keyboard interrupt (Ctrl+C) can provide immediate relief during development. Additionally, employing debugging tools like pdb can help trace the loop’s execution.”
Michael Chen (Lead Python Developer, Tech Innovations Group). “In my experience, the best approach to prevent infinite loops is to incorporate robust error handling and logging. By utilizing try-except blocks, you can catch unexpected behavior and exit the loop gracefully, ensuring that your application remains stable.”
Sarah Thompson (Computer Science Educator, Online Learning Platform). “Teaching students to recognize the signs of an infinite loop is crucial. I recommend using print statements or logging to monitor loop iterations. This practice not only aids in debugging but also reinforces the importance of defining clear exit conditions in their code.”
Frequently Asked Questions (FAQs)
What is an infinite loop in Python?
An infinite loop in Python occurs when a loop continues to execute indefinitely because its terminating condition is never met. This can lead to unresponsive programs or excessive resource consumption.
How can I identify an infinite loop in my code?
You can identify an infinite loop by observing the program’s behavior. If the program runs for an unusually long time without producing output or completing, it may be stuck in an infinite loop. Additionally, using debugging tools or inserting print statements can help track the loop’s execution.
What are common causes of infinite loops in Python?
Common causes include incorrect loop conditions, failure to update loop variables, or using a condition that always evaluates to true. Logical errors in the code can also contribute to unintended infinite loops.
How can I stop an infinite loop while running a Python program?
You can stop an infinite loop by interrupting the program’s execution. In most environments, pressing `Ctrl + C` in the terminal will raise a KeyboardInterrupt, terminating the loop and the program.
What programming practices can help prevent infinite loops?
To prevent infinite loops, ensure that loop conditions are correctly defined and that loop variables are updated appropriately within the loop. Implementing a maximum iteration count or using break statements can also help manage loop execution.
Is there a way to debug an infinite loop in Python?
Yes, you can use debugging tools such as Python’s built-in debugger (`pdb`) to step through your code line by line. Additionally, utilizing logging or print statements to monitor variable values can provide insights into the loop’s behavior and help identify the cause of the infinite loop.
Stopping an infinite loop in Python is crucial for maintaining the efficiency and functionality of your programs. Infinite loops occur when the terminating condition of a loop is never met, causing the program to run indefinitely. Understanding the causes of infinite loops, such as incorrect loop conditions or improper updates to loop variables, is essential for prevention and resolution. Utilizing debugging techniques, such as print statements or breakpoints, can help identify the source of the loop and facilitate its termination.
There are several methods to stop an infinite loop while a program is running. The most straightforward approach is to use keyboard interrupts, such as pressing Ctrl+C in the command line or terminal. This sends a signal to the Python interpreter to terminate the program. Additionally, implementing proper exit conditions within the loop, using the ‘break’ statement, or setting a maximum iteration limit can prevent infinite loops from occurring in the first place.
In summary, recognizing the signs of an infinite loop and employing effective debugging strategies are vital skills for any Python programmer. By understanding how to interrupt a running loop and implementing safeguards within your code, you can enhance the robustness of your applications and avoid the pitfalls associated with infinite loops. Continuous learning and practice in managing loops will ultimately contribute to more efficient and reliable programming outcomes
Author Profile
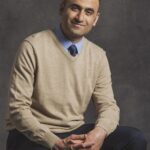
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?