How Can You Effectively Stop a Node.js Server?
How to Stop a Node.js Server: A Comprehensive Guide
In the world of web development, Node.js has emerged as a powerhouse for building scalable and efficient applications. However, as with any technology, there comes a time when you need to halt your Node.js server, whether for maintenance, updates, or simply to free up system resources. Knowing how to stop your server correctly is crucial not only for maintaining the health of your application but also for ensuring a seamless experience for your users. In this article, we will explore the various methods to gracefully shut down a Node.js server, equipping you with the knowledge to manage your server effectively.
Stopping a Node.js server may seem straightforward, but it involves understanding the nuances of how your application operates. Depending on how your server is set up, you might have different options at your disposal. From command-line commands to programmatic approaches, each method has its own implications and best practices. Additionally, knowing how to handle ongoing requests and clean up resources is vital to prevent data loss and ensure a smooth transition.
As we dive deeper into the topic, we will cover the most common scenarios for stopping a Node.js server, including manual termination, using process managers, and implementing shutdown hooks. Whether you’re a seasoned developer or just starting your journey
Using the Command Line
To stop a Node.js server from the command line, you can utilize the process ID (PID) of the running Node.js application. Here are the steps to follow:
- First, identify the running Node.js process. You can do this by executing the following command in your terminal:
“`bash
ps aux | grep node
“`
- This will display a list of Node.js processes. Look for the line that corresponds to your application, and note the PID, which is usually the second column in the output.
- Once you have the PID, you can terminate the process using the kill command:
“`bash
kill
“`
Replace `
- If the process does not terminate gracefully, you can forcefully kill it by using:
“`bash
kill -9
“`
Using npm Scripts
If your Node.js server was started using an npm script, you can stop it by simply terminating the script. Here’s how you can manage it:
- If you started your server with:
“`bash
npm start
“`
- You can stop it by pressing `Ctrl + C` in the terminal where it’s running. This command sends an interrupt signal to the process, which typically results in a graceful shutdown.
Using Process Managers
Many developers use process managers like PM2 to manage Node.js applications. If you are using PM2, stopping your server is straightforward:
- First, list all running processes managed by PM2 with:
“`bash
pm2 list
“`
- Identify your application from the list and note its name or ID.
- Stop the application using:
“`bash
pm2 stop
“`
- If you want to stop all running applications managed by PM2, you can use:
“`bash
pm2 stop all
“`
Graceful Shutdown
For a more controlled shutdown, especially in production environments, implementing a graceful shutdown is essential. This ensures that all ongoing requests are completed before the server closes. To achieve this, you can listen for termination signals in your Node.js application:
“`javascript
process.on(‘SIGINT’, () => {
console.log(‘Received SIGINT. Shutting down gracefully.’);
// Close server and cleanup tasks
server.close(() => {
console.log(‘Server closed’);
process.exit(0);
});
});
“`
This code listens for the `SIGINT` signal (triggered by `Ctrl + C`) and allows the server to finish processing ongoing requests before shutting down.
Method | Command | Notes |
---|---|---|
Command Line | kill <PID> | Use `ps aux | grep node` to find PID |
npm Script | Ctrl + C | Works for scripts started with npm |
PM2 | pm2 stop <app-name> | Use PM2 to manage processes |
Stopping a Node.js Server Gracefully
When managing a Node.js server, it is crucial to stop it gracefully to ensure that all ongoing processes are completed and resources are released properly. Here are several methods to achieve this:
– **Using Ctrl + C in the Terminal**:
- This is the most straightforward method. If you are running your Node.js application in a terminal, simply press `Ctrl + C`. This sends a SIGINT signal to the process, allowing Node.js to perform any cleanup operations.
– **Programmatically Stopping the Server**:
- You can also stop the server programmatically by using the `server.close()` method. This method stops the server from accepting new connections and keeps existing connections open until they are handled.
“`javascript
const http = require(‘http’);
const server = http.createServer((req, res) => {
res.writeHead(200);
res.end(‘Hello World’);
});
server.listen(3000, () => {
console.log(‘Server running at http://localhost:3000/’);
});
// Function to stop the server
function stopServer() {
server.close(() => {
console.log(‘Server stopped gracefully’);
});
}
“`
Killing the Node.js Process
In cases where the server does not respond to the graceful shutdown or if you need to forcefully terminate it, you can kill the process directly. This is typically done using process management tools or the command line.
- Using the Command Line:
- First, identify the process ID (PID) of the running Node.js server using a command such as `ps` or `pgrep`.
“`bash
Find PID of Node.js processes
ps aux | grep node
“`
- Once you have the PID, you can terminate it using the `kill` command.
“`bash
kill
“`
- If the process does not terminate, you can use the `-9` option to forcefully kill it.
“`bash
kill -9
“`
- Using PM2:
- If you are using PM2, a popular process manager for Node.js applications, stopping your server is simplified with built-in commands.
“`bash
pm2 stop
“`
- To stop all applications managed by PM2, you can use:
“`bash
pm2 stop all
“`
Monitoring Server Shutdown
To ensure that your Node.js server is shutting down correctly, consider implementing logging during the shutdown process. This can help you identify any issues that may occur when attempting to stop the server.
– **Example of Logging Shutdown**:
“`javascript
process.on(‘SIGINT’, () => {
console.log(‘Received SIGINT. Gracefully shutting down the server…’);
stopServer();
});
“`
This code snippet listens for the SIGINT signal and invokes the `stopServer` function, allowing for graceful shutdown while logging the action.
Employing these strategies will provide you with various options to stop your Node.js server effectively, whether gracefully or forcefully, depending on your specific needs.
Expert Strategies for Stopping Node.js Servers
Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively stop a Node.js server, you can use the terminal command `Ctrl + C` while the server is running in the foreground. This sends an interrupt signal that gracefully shuts down the server. For production environments, consider implementing a shutdown handler in your code to manage ongoing requests before termination.”
James Liu (DevOps Specialist, Cloud Solutions Group). “In a production setting, it is essential to stop your Node.js server without causing disruptions. Utilize process managers like PM2, which allows you to stop the server with commands like `pm2 stop
`. This ensures that your application can be restarted easily and maintains the state of your processes.”
Sarah Thompson (Lead Backend Developer, CodeCraft Labs). “For developers working with Docker, stopping a Node.js server can be done using `docker stop
`. This command stops the container running your Node.js application, ensuring that all resources are released appropriately. Always ensure that your application handles the shutdown process to avoid data loss.”
Frequently Asked Questions (FAQs)
How can I stop a Node.js server that is running in the terminal?
To stop a Node.js server running in the terminal, you can simply press `Ctrl + C`. This command sends a termination signal to the process, effectively shutting it down.
Is there a way to programmatically stop a Node.js server?
Yes, you can programmatically stop a Node.js server by calling the `close` method on the server instance. For example: `server.close()` will stop accepting new connections and close existing ones.
What command can I use to stop a Node.js server running in the background?
If the Node.js server is running in the background, you can find its process ID (PID) using the `ps` command, then use `kill
Can I stop a Node.js server using a specific port number?
You cannot directly stop a server by specifying a port number. However, you can find the PID of the process using the port with commands like `lsof -i :
What should I do if my Node.js server does not stop using Ctrl + C?
If the server does not stop with `Ctrl + C`, it may be unresponsive due to an ongoing operation. In such cases, you can forcefully terminate it using `kill -9
Is there a way to handle server shutdown gracefully in Node.js?
Yes, you can handle server shutdown gracefully by listening for termination signals (like `SIGINT` or `SIGTERM`). In your server code, you can implement a cleanup routine before calling `server.close()`.
stopping a Node.js server can be accomplished through various methods, depending on how the server was initiated and the environment in which it is running. Commonly, developers use commands in the terminal to terminate the process, such as using Ctrl+C for interactive sessions or employing process management tools like PM2 for more robust server management. Understanding the context in which your Node.js application operates is crucial for effectively stopping the server without causing disruptions.
Additionally, for applications running in production, it is essential to implement graceful shutdown procedures. This involves handling ongoing requests and closing connections properly before shutting down the server. Utilizing signals such as SIGINT or SIGTERM can facilitate this process, allowing the server to complete its current operations and release resources appropriately.
Moreover, leveraging tools and libraries designed for process management can enhance the control you have over your Node.js applications. These tools not only simplify the stopping process but also provide features for monitoring and restarting servers automatically, which can be invaluable for maintaining uptime and reliability.
In summary, effectively stopping a Node.js server requires an understanding of the operational context and the appropriate methods to ensure a clean and efficient shutdown. By adopting best practices and utilizing available tools, developers can manage their Node.js applications
Author Profile
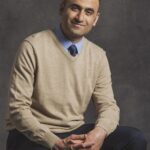
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?