How Can You Stop a Running Python Code Effectively?
In the world of programming, particularly with Python, the ability to control the execution of your code is just as crucial as writing it. Whether you’re debugging a script that seems to run indefinitely, or you’re developing a complex application and need to halt processes for any reason, knowing how to stop Python code effectively can save you time, resources, and frustration. In this article, we will explore various methods to gracefully terminate your Python scripts, ensuring that you can maintain control over your programming environment.
Stopping Python code can be necessary for a variety of reasons, from handling unexpected errors to simply needing to pause execution for testing purposes. Python provides several built-in mechanisms that allow developers to halt their scripts, whether through keyboard interrupts, exception handling, or programmatically using specific commands. Understanding these methods not only enhances your coding skills but also equips you with the tools to manage your workflow more efficiently.
As we delve into the various techniques for stopping Python code, we will cover both interactive and programmatic approaches. You’ll learn how to effectively use keyboard shortcuts, manage exceptions, and leverage control flow statements to ensure that your scripts can be halted safely and effectively. By mastering these techniques, you’ll be better prepared to navigate the complexities of Python programming and maintain a smooth coding experience.
Methods to Stop Python Code Execution
There are several effective methods to stop the execution of Python code, each suited for different scenarios. Understanding these methods can enhance your debugging skills and provide better control over your scripts.
Using Keyboard Interrupt
The simplest way to stop a running Python program is by using a keyboard interrupt. This is particularly useful when running scripts in a terminal or command prompt.
- Windows: Press `Ctrl + C`.
- Linux/Mac: Press `Ctrl + C`.
When you press this combination, Python raises a `KeyboardInterrupt` exception, which you can handle in your code if necessary.
Programmatically Stopping Execution
You can also stop the execution of Python code programmatically using the `sys.exit()` function. This is especially useful in larger applications where you may want to terminate execution based on certain conditions.
Example:
python
import sys
# Some conditions
if some_condition:
sys.exit(“Exiting the program due to condition.”)
This function accepts an optional argument, which can be an integer or a string. If an integer is provided, it is returned as the exit status; if a string is provided, it will be printed to stderr.
Using Exceptions to Stop Code
Another approach involves raising an exception to halt execution. You can define custom exceptions or use built-in ones like `SystemExit`:
python
class CustomExit(Exception):
pass
raise CustomExit(“Stopping execution.”)
This method allows for more control over how the program terminates and can be caught by exception handling if you need to perform cleanup tasks.
Stopping Threads and Processes
When dealing with multi-threading or multi-processing, stopping code execution requires more specific approaches.
For Threads:
Python does not have a direct way to kill threads. Instead, you can use a flag to signal a thread to stop:
python
import threading
stop_thread =
def thread_function():
while not stop_thread:
# Thread work here
pass
thread = threading.Thread(target=thread_function)
thread.start()
# To stop
stop_thread = True
thread.join() # Wait for the thread to finish
For Processes:
When using the `multiprocessing` module, you can terminate a process using the `terminate()` method.
python
from multiprocessing import Process
def process_function():
while True:
pass # Infinite loop
process = Process(target=process_function)
process.start()
# To stop
process.terminate()
process.join() # Clean up
Comparison of Methods
Method | Use Case | Control Level |
---|---|---|
Keyboard Interrupt | Interactive scripts | Low |
sys.exit() | Programmatic termination | Moderate |
Raising Exceptions | Conditional termination | High |
Thread Flag | Stopping threads safely | Moderate |
Process Termination | Stopping processes forcibly | High |
Understanding these methods provides flexibility in managing your Python scripts and applications, enhancing both performance and reliability.
Methods to Stop Python Code Execution
To halt the execution of a Python program, there are several approaches depending on the context and the environment in which the code is running. Below are some common methods.
Using Keyboard Interrupt
In many cases, especially during development in a terminal or command prompt, you can interrupt the execution of a script using the following keyboard shortcut:
- Ctrl + C: This sends a keyboard interrupt signal to the program, effectively stopping it immediately.
This method is particularly useful for scripts that are running indefinitely, such as those using loops or waiting for input.
Using the sys.exit() Function
If you want to programmatically terminate a Python script, you can use the `sys.exit()` function. This method allows you to stop execution at a specific point in your code.
python
import sys
# Some code here
if some_condition:
sys.exit(“Stopping execution due to some condition.”)
The `sys.exit()` function raises a `SystemExit` exception, which can be caught in an outer layer if necessary.
Using os._exit() Function
For a more abrupt termination of the script, you can use `os._exit()`. This function exits the program without performing any cleanup actions.
python
import os
# Some code here
if some_critical_condition:
os._exit(1) # Exits with a status code
This method is generally not recommended unless you’re in a situation where you want to terminate immediately without cleanup, like in multi-threaded applications.
Stopping a Running Script in an IDE
In Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, or Jupyter Notebooks, you can stop the execution of your code using built-in commands:
- PyCharm: Click on the red square (stop button) in the console.
- Visual Studio Code: Use the command palette (Ctrl + Shift + P) and type “Stop Running”.
- Jupyter Notebooks: Click on the “Stop” button in the toolbar.
Handling Long-Running Processes
For scripts that may run for an extended period and need to be stopped gracefully, you can implement signal handling. This is particularly useful for server applications or long-running tasks.
python
import signal
import time
def signal_handler(sig, frame):
print(“Gracefully shutting down…”)
sys.exit(0)
signal.signal(signal.SIGINT, signal_handler)
while True:
print(“Running…”)
time.sleep(1)
This code snippet allows the program to handle keyboard interrupts gracefully, ensuring that any necessary cleanup can occur before the program exits.
Using a Timeout
In scenarios where you want to impose a time limit on execution, using a timeout can be beneficial. This can be achieved through threading or asynchronous programming.
python
import threading
def long_running_task():
while True:
print(“Working…”)
task_thread = threading.Thread(target=long_running_task)
task_thread.start()
task_thread.join(timeout=5) # Wait for 5 seconds
if task_thread.is_alive():
print(“Terminating the task…”)
# You may need to implement additional logic to stop the thread
This example demonstrates how to run a task in a separate thread and impose a timeout for its execution.
Implementing proper methods to stop Python code execution is crucial for effective programming practices. Understanding these techniques will help maintain control over your scripts and improve the overall robustness of your applications.
Expert Insights on Stopping Python Code Execution
Dr. Emily Carter (Software Development Specialist, Tech Innovations Inc.). “To effectively stop Python code execution, developers can utilize keyboard interrupts, such as pressing Ctrl+C in the terminal. This method is particularly useful during long-running processes, allowing for an immediate halt without the need for complex error handling.”
Michael Chen (Lead Python Engineer, CodeCraft Solutions). “Implementing exception handling within your Python scripts can provide a controlled way to stop execution. By using try-except blocks, developers can gracefully handle specific conditions that warrant stopping the code, ensuring that resources are released properly.”
Lisa Patel (Senior Data Scientist, Data Insights Group). “For scripts running in a Jupyter Notebook, the stop button in the interface is an effective tool for halting execution. Additionally, using the `sys.exit()` function within the code can programmatically terminate the script at any point, which is useful for conditional exits.”
Frequently Asked Questions (FAQs)
How can I stop a running Python script in the terminal?
You can stop a running Python script in the terminal by pressing `Ctrl + C`. This sends a keyboard interrupt signal to the process, terminating it.
What command can I use to terminate a Python process in Windows?
In Windows, you can use the `taskkill` command in the Command Prompt. For example, `taskkill /F /IM python.exe` will forcefully terminate all running Python processes.
Is there a way to stop a Python script programmatically?
Yes, you can use the `sys.exit()` function within your script to terminate it at a specific point. This raises a `SystemExit` exception, which stops the program.
How can I stop a Python script running in an IDE like PyCharm?
In PyCharm, you can stop the execution of a running script by clicking the red stop button in the Run tool window, or by using the keyboard shortcut `Ctrl + F2`.
What should I do if my Python script is unresponsive?
If your script is unresponsive, you may need to use the Task Manager on Windows or Activity Monitor on macOS to forcefully end the Python process.
Can I set a timeout to automatically stop a Python script?
Yes, you can use the `signal` module to set a timeout. By defining a signal handler and using `signal.alarm()`, you can raise an exception to stop the script after a specified duration.
stopping Python code can be achieved through various methods, depending on the context in which the code is running. For scripts executed in a terminal or command line, the most straightforward approach is to use keyboard interrupts, such as Ctrl+C, which sends a signal to terminate the running process. Additionally, when working within integrated development environments (IDEs), there are often built-in options to stop the execution of code, providing users with a convenient way to halt processes without needing to resort to command line interventions.
Furthermore, for long-running scripts or applications, implementing proper exception handling and using flags or conditions can allow for graceful termination of processes. This approach not only enhances user experience but also ensures that resources are managed effectively, preventing potential data corruption or memory leaks. Utilizing the `sys.exit()` function can also be beneficial for programmatically stopping code execution at specific points, providing developers with control over their program flow.
In summary, understanding how to effectively stop Python code is crucial for developers to manage their applications efficiently. Whether through keyboard interrupts, IDE features, or programmatic methods, having a clear strategy for terminating processes can significantly improve workflow and debugging efforts. By considering these methods, developers can enhance their coding practices and ensure smoother execution of
Author Profile
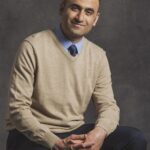
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?