How Can You Effectively Stop Code Execution in Python?
In the world of programming, especially with a versatile language like Python, knowing how to control the flow of your code is essential for effective development. Whether you’re debugging a complex algorithm, managing resource-intensive tasks, or simply experimenting with new ideas, the ability to stop or terminate your code at will can save you time and frustration. Understanding the various methods to halt execution not only enhances your coding efficiency but also empowers you to create more robust applications. In this article, we will explore the different techniques available in Python to gracefully stop your code, ensuring you have the tools needed to handle any situation that arises during your programming journey.
When coding in Python, there are various scenarios where you might need to stop the execution of your script. This could be due to an infinite loop, a condition that no longer holds true, or simply a need to exit a program early. Python provides several built-in mechanisms that allow you to interrupt the flow of your code safely and effectively. Each method has its own use cases and implications, making it crucial to understand when and how to apply them.
In addition to the standard techniques for stopping code execution, Python also offers advanced options that cater to more complex requirements. From handling exceptions to integrating with external libraries, the ways to halt your code can
Using Keyboard Interrupts
One of the most straightforward ways to stop a running Python program is by using a keyboard interrupt. This can typically be done by pressing `Ctrl + C` in the command line or terminal where the Python script is executing. When this combination is pressed, it raises a `KeyboardInterrupt` exception, which can be caught in the code if necessary.
To handle this exception, you can wrap your code in a try-except block:
“`python
try:
while True:
print(“Running… Press Ctrl+C to stop.”)
except KeyboardInterrupt:
print(“Program stopped by user.”)
“`
This allows for graceful termination, providing the opportunity to execute any cleanup code before the program exits.
Using the sys.exit() Function
Another method to stop a Python program is to use the `sys.exit()` function. This function raises a `SystemExit` exception, which can be caught in the same way as `KeyboardInterrupt`. This is particularly useful in scripts where you want to exit based on specific conditions.
First, you need to import the `sys` module:
“`python
import sys
if some_condition:
print(“Exiting the program.”)
sys.exit()
“`
This method provides the ability to specify an exit status, which can be useful for signaling the outcome of the program to the operating system.
Using Exceptions to Control Flow
You can also define your own exceptions to manage the flow of your program. This approach allows for complex and controlled exits based on custom logic. Here’s an example:
“`python
class StopExecution(Exception):
pass
try:
Some code logic
raise StopExecution(“Stopping the execution.”)
except StopExecution as e:
print(e)
“`
This method can be particularly helpful in larger applications where you need to manage various exit points in an organized manner.
Stopping Threads and Processes
When working with threads or processes, simply stopping the main program does not halt background operations. To effectively stop these, you can use flags or built-in methods provided by the threading and multiprocessing modules.
For threading:
“`python
import threading
import time
def worker(stop_event):
while not stop_event.is_set():
print(“Working…”)
time.sleep(1)
stop_event = threading.Event()
thread = threading.Thread(target=worker, args=(stop_event,))
thread.start()
Allow the thread to run for a short duration
time.sleep(5)
stop_event.set() Signal the thread to stop
thread.join() Wait for the thread to finish
print(“Thread has been stopped.”)
“`
For multiprocessing:
“`python
from multiprocessing import Process
import time
def worker():
while True:
print(“Working in process…”)
time.sleep(1)
process = Process(target=worker)
process.start()
Allow the process to run for a short duration
time.sleep(5)
process.terminate() Immediately terminate the process
process.join() Wait for the process to finish
print(“Process has been stopped.”)
“`
Comparison of Stopping Methods
Method | Description | Use Case |
---|---|---|
Keyboard Interrupt | Stops the program using Ctrl+C | Interactive scripts |
sys.exit() | Exits the program with an optional status | Condition-based exits |
Custom Exceptions | Allows for graceful exits based on logic | Complex applications |
Threading Event | Signals threads to stop execution | Multithreaded applications |
Process Termination | Forcefully stops a running process | Multiprocessing applications |
Each method provides unique benefits depending on the context and requirements of your program, ensuring flexibility in managing execution flow.
Using Keyboard Interrupts
One of the simplest ways to stop a running Python program is by using keyboard interrupts. This is particularly useful during development or when a script is running in a terminal.
- Ctrl + C: This key combination sends a SIGINT (interrupt signal) to the program, which typically causes it to stop execution. It is a common method used in command-line environments.
Implementing Timeout with Signals
For more controlled termination of a program, especially when dealing with long-running processes, the `signal` module can be utilized to set a timeout.
“`python
import signal
def handler(signum, frame):
raise Exception(“Execution timed out”)
signal.signal(signal.SIGALRM, handler)
signal.alarm(5) Set a timeout of 5 seconds
try:
Code that may run too long
while True:
pass
except Exception as e:
print(e)
finally:
signal.alarm(0) Disable the alarm
“`
This code will raise an exception if the block runs longer than the specified time, allowing for graceful termination.
Using the sys.exit() Function
In scenarios where you want to exit a program programmatically, the `sys.exit()` function from the `sys` module can be effective.
“`python
import sys
def some_function():
Condition to stop execution
if some_condition:
sys.exit(“Exiting the program”)
some_function()
“`
This function raises a `SystemExit` exception, which can be caught if needed but will terminate the program if not.
Handling Exceptions for Clean Exit
Managing exceptions can also be a way to control program termination. By wrapping your code in a try-except block, you can handle errors gracefully and exit the program cleanly.
“`python
try:
Some code that might raise an exception
risky_operation()
except Exception as e:
print(f”An error occurred: {e}”)
sys.exit(1) Exit with a specific error code
“`
This approach ensures that resources are properly released before terminating.
Using the os._exit() Function
For a more abrupt termination, you can use the `os._exit()` function. This should be used with caution, as it does not clean up the program’s resources.
“`python
import os
def terminate_program():
os._exit(0) Exit immediately with a status code
“`
This function is particularly useful in multi-threaded applications where you want to stop all threads immediately.
Terminating Threads
When dealing with threads, stopping them can be more complex. Python does not provide a direct way to kill threads. Instead, you should design threads to check for a termination flag.
“`python
import threading
import time
stop_flag =
def worker():
while not stop_flag:
Perform work
time.sleep(1)
thread = threading.Thread(target=worker)
thread.start()
To stop the thread
stop_flag = True
thread.join() Wait for the thread to finish
“`
This approach allows the thread to end gracefully rather than being forcefully terminated.
Using Context Managers
To ensure that resources are freed properly, consider using context managers. They allow for clean exit from blocks of code.
“`python
from contextlib import contextmanager
@contextmanager
def controlled_execution():
try:
yield
except Exception as e:
print(f”Error: {e}”)
finally:
print(“Cleaning up resources.”)
with controlled_execution():
Code that might fail
risky_operation()
“`
This pattern ensures that cleanup code runs even if an error occurs.
Expert Strategies for Stopping Code Execution in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively stop code execution in Python, one can utilize the built-in `sys.exit()` function, which raises a `SystemExit` exception. This method is particularly useful for terminating scripts gracefully and ensuring that any necessary cleanup operations are performed.”
James Liu (Python Developer Advocate, CodeMasters). “Another approach to halting execution is using the `KeyboardInterrupt` exception, which can be triggered by pressing `Ctrl+C` during runtime. This is especially handy for long-running scripts, allowing developers to regain control without needing to modify the code.”
Sarah Thompson (Lead Data Scientist, Analytics Hub). “In scenarios where you need to stop code execution based on specific conditions, implementing a conditional statement that raises an exception can be effective. For instance, using `raise Exception(‘Stopping execution’)` allows for controlled termination, providing clarity on why the code was halted.”
Frequently Asked Questions (FAQs)
How can I stop a running Python script in the terminal?
You can stop a running Python script in the terminal by pressing `Ctrl + C`. This sends a keyboard interrupt signal, terminating the script gracefully.
What is the purpose of the `sys.exit()` function in Python?
The `sys.exit()` function is used to exit from Python. It raises a `SystemExit` exception, which can be caught in outer levels of the program, allowing for a clean exit.
Can I use `os._exit()` to stop a Python program?
Yes, `os._exit()` can be used to terminate a program immediately without cleanup. It is generally not recommended unless necessary, as it does not call cleanup handlers.
How do I stop a Python script from an IDE like PyCharm?
In PyCharm, you can stop a running script by clicking the red square (stop button) in the console window. This will terminate the execution of the script.
What happens if I stop a Python script that is writing to a file?
If you stop a Python script while it is writing to a file, the file may become corrupted or incomplete. It is advisable to implement proper error handling and file closing mechanisms.
Is there a way to programmatically stop a Python script?
Yes, you can programmatically stop a Python script using the `raise SystemExit` statement or by calling `sys.exit()`. This allows for controlled termination within the code.
In Python, stopping code execution can be achieved through various methods depending on the context and requirements of the program. The most straightforward way to terminate a running script is by using the built-in `exit()` or `sys.exit()` functions, which raise a `SystemExit` exception. This allows for a clean exit from the program, ensuring that any necessary cleanup can be performed before termination. Additionally, keyboard interrupts, such as pressing Ctrl+C in a terminal, can also halt execution, making it a useful tool during development or debugging sessions.
For more complex scenarios, such as stopping code execution within loops or functions, the `break` statement can be employed to exit from loops prematurely. In cases where specific conditions must be met to stop execution, using exceptions can provide a robust solution. Custom exceptions can be defined and raised to signal when the program should cease operations, allowing for greater control and flexibility in managing program flow.
Ultimately, understanding the various methods to stop code execution in Python is essential for effective programming. It not only enhances the ability to manage program behavior but also contributes to writing cleaner and more maintainable code. By employing these techniques thoughtfully, developers can improve the overall user experience and ensure that their applications respond appropriately to user inputs
Author Profile
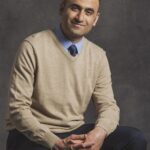
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?