How Can You Effectively Store an Array in JavaScript?
Introduction
In the world of JavaScript, arrays are one of the most fundamental data structures, serving as a powerful tool for organizing and manipulating collections of data. Whether you’re building a simple web application or a complex software solution, understanding how to effectively store and manage arrays can significantly enhance your programming capabilities. As you dive into the intricacies of JavaScript, you’ll discover that mastering arrays not only streamlines your code but also opens up a realm of possibilities for data handling and algorithm implementation.
Storing an array in JavaScript is a straightforward process, but it comes with a variety of techniques and best practices that can optimize your code. From declaring arrays using different syntaxes to leveraging built-in methods for manipulation, the language offers flexibility that caters to both beginners and seasoned developers. As you explore these methods, you’ll learn how to create arrays that are not just functional but also efficient and easy to maintain.
Moreover, understanding the nuances of array storage is crucial in scenarios involving dynamic data, such as user inputs or API responses. By grasping how to store arrays effectively, you can ensure that your applications remain responsive and capable of handling data changes seamlessly. Join us as we delve deeper into the various ways to store arrays in JavaScript, equipping you with the knowledge to elevate your
Declaring an Array
In JavaScript, arrays can be declared using either the array literal syntax or the `Array` constructor. The array literal syntax is often preferred for its simplicity and readability.
- Array Literal Syntax:
javascript
const fruits = [‘apple’, ‘banana’, ‘cherry’];
- Array Constructor:
javascript
const fruits = new Array(‘apple’, ‘banana’, ‘cherry’);
Using the literal syntax is generally more common due to its straightforward nature.
Accessing Array Elements
Elements in an array can be accessed using their index, which starts at 0. This means the first element is accessed with index 0, the second with index 1, and so forth.
javascript
const fruits = [‘apple’, ‘banana’, ‘cherry’];
console.log(fruits[0]); // Output: apple
console.log(fruits[1]); // Output: banana
Storing Different Data Types
JavaScript arrays are flexible and can store elements of different data types, including strings, numbers, objects, and even other arrays. Here’s an example:
javascript
const mixedArray = [42, ‘hello’, { name: ‘John’ }, [1, 2, 3]];
Common Array Methods
JavaScript provides a rich set of methods for manipulating arrays. Some of the most commonly used methods include:
- push(): Adds one or more elements to the end of an array.
- pop(): Removes the last element from an array and returns it.
- shift(): Removes the first element from an array and returns it.
- unshift(): Adds one or more elements to the beginning of an array.
- slice(): Returns a shallow copy of a portion of an array into a new array object.
Here’s a brief table summarizing these methods:
Method | Description |
---|---|
push() | Adds elements to the end |
pop() | Removes the last element |
shift() | Removes the first element |
unshift() | Adds elements to the beginning |
slice() | Creates a shallow copy of a portion |
Iterating Over Arrays
To process each element in an array, you can use various iteration methods. The most common are `for` loops, `forEach()`, and the newer `map()` method.
- Using a for loop:
javascript
const fruits = [‘apple’, ‘banana’, ‘cherry’];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
- **Using forEach()**:
javascript
fruits.forEach(fruit => {
console.log(fruit);
});
– **Using map()**:
javascript
const upperFruits = fruits.map(fruit => fruit.toUpperCase());
console.log(upperFruits); // Output: [‘APPLE’, ‘BANANA’, ‘CHERRY’]
Multidimensional Arrays
JavaScript also supports multidimensional arrays, which are arrays of arrays. This is useful for representing matrices or grids.
javascript
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
You can access elements in a multidimensional array by specifying multiple indices:
javascript
console.log(matrix[1][2]); // Output: 6
Utilizing these techniques allows for efficient data storage and manipulation in JavaScript, making arrays a powerful tool for developers.
Using Arrays in JavaScript
In JavaScript, arrays are versatile objects that can store multiple values in a single variable. They are dynamic in nature, allowing for easy addition, removal, and iteration of elements.
Creating an Array
Arrays can be created in several ways:
- Array Literal Syntax:
javascript
const fruits = [‘Apple’, ‘Banana’, ‘Cherry’];
- Array Constructor:
javascript
const vegetables = new Array(‘Carrot’, ‘Potato’, ‘Tomato’);
- Using Array.of():
javascript
const numbers = Array.of(1, 2, 3, 4, 5);
- Using Array.from():
javascript
const stringArray = Array.from(‘Hello’); // [‘H’, ‘e’, ‘l’, ‘l’, ‘o’]
Accessing Array Elements
Elements in an array can be accessed using their index, which starts from zero. For example:
javascript
const colors = [‘Red’, ‘Green’, ‘Blue’];
console.log(colors[0]); // Output: Red
Modifying Arrays
You can modify arrays by adding or removing elements. Common methods include:
- Adding Elements:
- `push()`: Adds one or more elements to the end of an array.
- `unshift()`: Adds one or more elements to the beginning of an array.
javascript
colors.push(‘Yellow’); // Adds ‘Yellow’ at the end
colors.unshift(‘Pink’); // Adds ‘Pink’ at the beginning
- Removing Elements:
- `pop()`: Removes the last element from an array.
- `shift()`: Removes the first element from an array.
javascript
colors.pop(); // Removes ‘Yellow’
colors.shift(); // Removes ‘Pink’
Iterating Over Arrays
JavaScript offers various methods to iterate through arrays:
- for Loop:
javascript
for (let i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
- **forEach() Method**:
javascript
colors.forEach(color => {
console.log(color);
});
– **map() Method**:
javascript
const upperColors = colors.map(color => color.toUpperCase());
Array Methods
JavaScript provides a rich set of built-in methods for array manipulation. Here are some key methods:
Method | Description |
---|---|
`slice()` | Returns a shallow copy of a portion of an array. |
`splice()` | Changes the contents of an array by removing or replacing existing elements. |
`concat()` | Merges two or more arrays into a new array. |
`join()` | Joins all elements of an array into a string. |
`filter()` | Creates a new array with all elements that pass a test. |
Storing Arrays in Objects
Arrays can also be stored as properties within objects, allowing for structured data management. For example:
javascript
const library = {
books: [‘1984’, ‘Brave New World’, ‘Fahrenheit 451’],
addBook: function(book) {
this.books.push(book);
}
};
library.addBook(‘The Great Gatsby’);
console.log(library.books); // [‘1984’, ‘Brave New World’, ‘Fahrenheit 451’, ‘The Great Gatsby’]
Nested Arrays
JavaScript allows for arrays to contain other arrays, enabling the creation of multi-dimensional arrays. For instance:
javascript
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(matrix[1][2]); // Output: 6
Expert Insights on Storing Arrays in JavaScript
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When storing arrays in JavaScript, it is essential to understand that arrays are dynamic objects. Utilizing methods such as `push()`, `pop()`, and `splice()` allows for efficient manipulation and storage of array elements, adapting to the needs of your application seamlessly.”
Michael Thompson (JavaScript Developer Advocate, CodeCraft). “In JavaScript, arrays can be stored in various ways, including local storage for persistent data. Using `JSON.stringify()` to convert an array into a string format before storage ensures that complex data structures are preserved and can be easily retrieved with `JSON.parse()`.”
Lisa Patel (Full-Stack Developer, Web Solutions Group). “It is crucial to consider the performance implications when storing large arrays in JavaScript. Techniques such as lazy loading and pagination can help manage memory usage effectively while ensuring that the application remains responsive and efficient.”
Frequently Asked Questions (FAQs)
How do I declare an array in JavaScript?
You can declare an array in JavaScript using square brackets `[]`, such as `let myArray = [1, 2, 3];` or by using the `Array` constructor like `let myArray = new Array(1, 2, 3);`.
What are the different ways to store data in an array?
You can store various data types in an array, including numbers, strings, objects, and even other arrays. For example, `let mixedArray = [1, ‘two’, { key: ‘value’ }, [3, 4]];` demonstrates this versatility.
How can I add elements to an existing array?
You can add elements using the `push()` method to append to the end, or `unshift()` to add to the beginning. For example, `myArray.push(4);` adds `4` to the end of the array.
How do I access elements in an array?
Elements in an array can be accessed using their index, which starts at `0`. For example, `let firstElement = myArray[0];` retrieves the first element of the array.
Can I store functions in an array?
Yes, functions can be stored in an array just like any other data type. For instance, `let functionArray = [function() { return ‘Hello’; }, function() { return ‘World’; }];` allows you to store and call functions from the array.
What is the difference between an array and an object in JavaScript?
An array is a special type of object designed for ordered data storage, where elements are accessed via numerical indexes. An object, on the other hand, is a collection of key-value pairs, providing a more flexible structure for storing related data.
Storing an array in JavaScript can be accomplished through various methods, depending on the context and requirements of the application. The most common way is to declare an array using the array literal syntax, which involves enclosing a comma-separated list of values within square brackets. For example, `let myArray = [1, 2, 3, 4];` creates an array containing four elements. Additionally, JavaScript provides the `Array` constructor, allowing for the creation of arrays using the syntax `let myArray = new Array(1, 2, 3, 4);`.
Another important aspect of storing arrays in JavaScript is the ability to manipulate them using various built-in methods. Functions such as `push()`, `pop()`, `shift()`, and `unshift()` enable developers to add or remove elements from arrays dynamically. Furthermore, arrays in JavaScript can also hold mixed data types, including numbers, strings, and even other arrays or objects, providing flexibility in data storage.
For persistent storage, JavaScript offers options such as localStorage and sessionStorage, which allow developers to store arrays as strings. This can be achieved by converting the array to a JSON string using `JSON.stringify()`
Author Profile
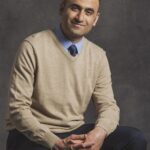
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?