How Can You Effectively Store Data in Python?
In the digital age, data is the lifeblood of innovation and decision-making. Whether you’re a budding programmer, a seasoned data scientist, or simply someone looking to manage information more effectively, understanding how to store data in Python is an essential skill. Python, with its versatility and rich ecosystem of libraries, offers a multitude of ways to handle data, making it a go-to language for developers across various domains. From simple lists to complex databases, the methods of data storage in Python are as diverse as the applications they support.
At its core, storing data in Python involves selecting the right structure or medium to keep your information organized, accessible, and efficient. Python provides built-in data types like lists, tuples, and dictionaries, which are perfect for smaller datasets or temporary storage needs. However, as projects scale, you may find yourself needing more robust solutions, such as files, databases, or even cloud storage. Each method comes with its own set of advantages and challenges, making it crucial to understand the context in which you’ll be working.
As you delve deeper into the world of data storage in Python, you’ll discover various techniques tailored to different scenarios. Whether you’re looking to persist data between program runs, share information across systems, or analyze large datasets, Python equips you with the tools
Using Variables
In Python, the simplest way to store data is through variables. Variables act as containers for data values, allowing you to easily reference and manipulate them throughout your code. You can assign values to variables using the assignment operator (`=`). For instance:
“`python
name = “Alice”
age = 30
height = 5.5
“`
Here, `name` stores a string, `age` stores an integer, and `height` holds a floating-point number. Variables can be reassigned and their types changed dynamically, which is a flexible feature of Python.
Lists
Lists are one of the most versatile data structures in Python, allowing you to store ordered collections of items. A list can hold elements of different types, including other lists. You define a list using square brackets `[]`, with elements separated by commas:
“`python
fruits = [“apple”, “banana”, “cherry”]
numbers = [1, 2, 3, 4, 5]
mixed = [1, “apple”, 3.14, True]
“`
You can access list items by their index, which starts from 0:
“`python
print(fruits[0]) Output: apple
“`
You can also modify lists using methods such as `append()`, `remove()`, and `sort()`.
Dictionaries
Dictionaries provide a way to store data in key-value pairs, making it easy to retrieve values by their corresponding keys. They are defined using curly braces `{}` with a colon separating keys and values:
“`python
person = {
“name”: “Alice”,
“age”: 30,
“height”: 5.5
}
“`
To access a value, use its key:
“`python
print(person[“name”]) Output: Alice
“`
Dictionaries are particularly useful for representing structured data and support operations like adding, updating, and deleting key-value pairs.
Sets
Sets are unordered collections of unique elements. They are defined using curly braces or the `set()` function, and are particularly useful for membership testing and eliminating duplicates from a list:
“`python
unique_numbers = {1, 2, 3, 4, 4, 5} Only unique elements will be stored
“`
You can perform set operations like union, intersection, and difference:
“`python
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1.union(set2)) Output: {1, 2, 3, 4, 5}
“`
Files
Storing data in files allows for persistent storage beyond the lifetime of a program’s execution. Python provides built-in functions for file operations. To write data to a file, you can use the following approach:
“`python
with open(‘data.txt’, ‘w’) as file:
file.write(“Hello, World!”)
“`
To read data from a file:
“`python
with open(‘data.txt’, ‘r’) as file:
content = file.read()
print(content) Output: Hello, World!
“`
Data Serialization
For more complex data structures or when data needs to be stored in a format that can be easily transported or used by other applications, serialization is essential. Python’s `pickle` module allows for object serialization. Here’s an example:
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
with open(‘data.pkl’, ‘rb’) as file:
loaded_data = pickle.load(file)
print(loaded_data) Output: {‘name’: ‘Alice’, ‘age’: 30}
“`
Comparison of Data Structures
Data Structure | Characteristics | Use Cases |
---|---|---|
List | Ordered, mutable, allows duplicates | Storing collections of items |
Dictionary | Unordered, mutable, key-value pairs | Mapping relationships between data |
Set | Unordered, mutable, unique elements | Membership testing, eliminating duplicates |
File | Persistent storage | Saving data across sessions |
Data Storage Options in Python
Python provides various options for data storage, each suited to different use cases. The following methods are commonly used to store data effectively.
Using Built-in Data Structures
Python’s built-in data structures allow for efficient in-memory data storage. The primary structures include:
- Lists: Ordered collections that can contain elements of different types.
- Tuples: Immutable ordered collections, suitable for fixed data sets.
- Dictionaries: Key-value pairs that allow for fast data retrieval.
- Sets: Unordered collections of unique elements.
Example of using a dictionary:
“`python
data = {
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
“`
File-Based Storage
For persistent storage, Python can read from and write to various file formats:
- Text Files: Simple and human-readable.
- CSV Files: Ideal for tabular data, easily handled with the `csv` module.
- JSON Files: Great for structured data, handled via the `json` module.
- Binary Files: Efficient for complex data types, used with the `pickle` module.
Example of writing to a CSV file:
“`python
import csv
data = [[“Name”, “Age”, “City”], [“Alice”, 30, “New York”], [“Bob”, 25, “Los Angeles”]]
with open(‘data.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
Database Storage
For large datasets or structured data, databases are preferable. Python supports various database systems:
Database Type | Description | Libraries |
---|---|---|
Relational | Structured data with SQL queries | `sqlite3`, `SQLAlchemy` |
NoSQL | Unstructured data, flexible schema | `MongoDB`, `Cassandra` |
In-memory | Fast access for transient data | `Redis`, `Memcached` |
Example of using SQLite:
“`python
import sqlite3
conn = sqlite3.connect(‘example.db’)
c = conn.cursor()
c.execute(”’CREATE TABLE users (name TEXT, age INTEGER)”’)
c.execute(“INSERT INTO users VALUES (‘Alice’, 30)”)
conn.commit()
conn.close()
“`
Data Serialization
Serialization allows for data to be converted into a format suitable for storage or transmission. Common libraries include:
- Pickle: Python’s built-in serialization format.
- JSON: A text-based format ideal for interoperability.
- MessagePack: A binary format that is more efficient than JSON.
Example of using Pickle:
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
“`
Cloud Storage Solutions
For scalable storage needs, cloud services are increasingly popular. Options include:
- Amazon S3: For object storage.
- Google Cloud Storage: For scalable storage solutions.
- Firebase: For real-time database capabilities.
Libraries such as `boto3` for AWS or `google-cloud-storage` for Google Cloud facilitate interaction with these services.
Best Practices for Data Storage
- Choose the right storage based on data size, access speed, and structure.
- Regularly back up important data.
- Use appropriate data formats for your use case to optimize performance.
- Ensure data security through encryption and access controls.
By utilizing the appropriate storage method and adhering to best practices, you can effectively manage data in Python, ensuring both performance and reliability.
Expert Insights on Data Storage in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When storing data in Python, utilizing built-in data structures such as lists, dictionaries, and sets is essential for efficient data manipulation. For larger datasets, consider leveraging libraries like Pandas, which provide powerful data handling capabilities.”
Mark Thompson (Software Engineer, Data Solutions Group). “For persistent data storage, using databases is crucial. Python’s SQLAlchemy library allows for seamless integration with various database systems, enabling developers to manage data effectively while maintaining scalability.”
Linda Zhang (Cloud Architect, Future Tech Labs). “In the era of cloud computing, utilizing cloud storage solutions such as AWS S3 or Google Cloud Storage can greatly enhance data accessibility and security. Python’s Boto3 library simplifies the interaction with these services, making data storage straightforward.”
Frequently Asked Questions (FAQs)
How can I store data in Python using lists?
Lists in Python allow you to store multiple items in a single variable. You can create a list by enclosing your data in square brackets, separating items with commas. For example, `my_list = [1, 2, 3]`.
What are dictionaries in Python and how do I use them for data storage?
Dictionaries in Python are collections of key-value pairs. You can store data by defining keys that map to specific values. For instance, `my_dict = {‘name’: ‘Alice’, ‘age’: 30}` allows you to access data via keys.
Can I store data in files using Python?
Yes, Python provides various methods to store data in files. You can use built-in functions like `open()`, `write()`, and `read()` to create and manipulate text or binary files. For example, `with open(‘data.txt’, ‘w’) as file: file.write(‘Hello, World!’)`.
What libraries can I use for data storage in Python?
Several libraries facilitate data storage in Python, including `pandas` for data manipulation and analysis, `sqlite3` for database management, and `json` for handling JSON data. Each library serves specific use cases.
How do I store data in a database using Python?
You can store data in a database using libraries like `sqlite3` for SQLite databases or `SQLAlchemy` for more complex database interactions. Establish a connection to the database, create tables, and execute SQL commands to insert data.
What is the best way to serialize data in Python?
The best way to serialize data in Python is to use the `pickle` module, which allows you to convert Python objects into a byte stream. This enables you to save complex data structures to files and retrieve them later. For example, `import pickle; pickle.dump(my_data, open(‘data.pkl’, ‘wb’))`.
storing data in Python can be accomplished through various methods, each suited to different use cases and requirements. The primary options include using built-in data structures such as lists, dictionaries, and sets for temporary storage, as well as external storage solutions like files and databases for more permanent data management. By understanding the strengths and limitations of each method, developers can choose the most appropriate approach for their specific needs.
Additionally, Python offers several libraries and frameworks that facilitate data storage and retrieval. For instance, the `pickle` module allows for object serialization, making it easy to save complex data structures to files. On the other hand, libraries like `pandas` provide powerful data manipulation capabilities, enabling efficient handling of large datasets. For persistent storage, relational databases can be accessed using libraries such as `sqlite3` or `SQLAlchemy`, while NoSQL databases can be interfaced with through libraries like `pymongo` for MongoDB.
Ultimately, the choice of data storage method in Python should be guided by factors such as data size, access frequency, and the complexity of the data structure. By leveraging the appropriate tools and techniques, developers can ensure that their data is stored efficiently, securely, and in a manner that supports their
Author Profile
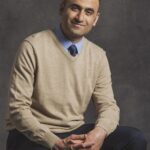
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?