How Can You Style a JavaScript Dropdown for a Seamless User Experience?
### Introduction
In the world of web development, user experience is paramount, and one of the most common yet often overlooked elements is the dropdown menu. A well-styled dropdown can enhance the aesthetic appeal of your website while ensuring that navigation remains intuitive and user-friendly. If you’ve ever found yourself frustrated with the default appearance of JavaScript dropdowns, you’re not alone. Fortunately, with a little creativity and some CSS magic, you can transform these functional elements into visually stunning components that align perfectly with your site’s design.
Styling a JavaScript dropdown involves more than just changing colors and fonts; it’s about creating a seamless integration between functionality and design. From customizing the appearance of the dropdown itself to ensuring that it responds elegantly to user interactions, there are numerous techniques that can elevate your dropdown menus from mundane to extraordinary. Whether you’re looking to achieve a minimalist look or a more vibrant, eye-catching style, the possibilities are endless.
In this article, we will explore the various methods and best practices for styling JavaScript dropdowns. We’ll delve into the importance of usability alongside aesthetics, and how to leverage CSS and JavaScript to create dropdowns that not only look great but also enhance the overall user experience. Get ready to unlock the potential of your dropdown menus and make them a standout feature
Styling the Dropdown with CSS
To create an aesthetically pleasing dropdown menu in JavaScript, leveraging CSS is essential. CSS allows you to customize the appearance of the dropdown, enhancing user experience. Below are some fundamental styling techniques that can be applied:
- Basic Styles: Set the width, height, background color, and border properties to define the dropdown’s overall appearance.
- Hover Effects: Utilize pseudo-classes like `:hover` to add interactive effects when users move their cursor over options.
- Transitions: Implement CSS transitions to create smooth effects when the dropdown opens or closes.
Here is a simple example of CSS to style a dropdown:
css
.dropdown {
position: relative;
display: inline-block;
}
.dropdown-content {
display: none;
position: absolute;
background-color: #f9f9f9;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
}
.dropdown:hover .dropdown-content {
display: block;
}
.dropdown-content a {
color: black;
padding: 12px 16px;
text-decoration: none;
display: block;
}
.dropdown-content a:hover {
background-color: #f1f1f1;
}
JavaScript Functionality
Integrating JavaScript enhances the interactivity of the dropdown. It allows you to control the dropdown’s behavior, such as opening, closing, and handling user selections. Here’s a basic implementation:
javascript
document.addEventListener(‘DOMContentLoaded’, function() {
const dropdown = document.querySelector(‘.dropdown’);
const dropdownContent = dropdown.querySelector(‘.dropdown-content’);
dropdown.addEventListener(‘click’, function() {
dropdownContent.classList.toggle(‘show’);
});
window.onclick = function(event) {
if (!event.target.matches(‘.dropdown’)) {
dropdownContent.classList.remove(‘show’);
}
};
});
Accessibility Considerations
When styling and scripting a dropdown, it’s crucial to ensure accessibility. An accessible dropdown allows all users, including those with disabilities, to navigate your site effectively. Consider the following points:
- Keyboard Navigation: Ensure users can navigate through the dropdown using the keyboard (e.g., using the Tab and Enter keys).
- ARIA Roles: Use appropriate ARIA roles to enhance screen reader accessibility. For example, use `role=”menu”` for the dropdown and `role=”menuitem”` for each option.
Accessibility Feature | Description |
---|---|
Keyboard Support | Enable navigation via keyboard keys. |
ARIA Roles | Include roles to assist screen readers. |
Focus Management | Ensure focus returns to the dropdown after selection. |
Responsive Design
In today’s mobile-first world, making sure your dropdown menus are responsive is vital. Use media queries in your CSS to adjust the layout based on screen size. For instance:
css
@media screen and (max-width: 600px) {
.dropdown-content {
min-width: 100%;
}
}
This media query ensures that on smaller screens, the dropdown expands to fit the screen width, improving usability on mobile devices.
By implementing these styling and functional practices, you can create a visually appealing and user-friendly dropdown menu that enhances the overall experience on your web application.
Styling a JavaScript Dropdown
To effectively style a dropdown menu in JavaScript, the combination of HTML, CSS, and potentially JavaScript for behavior is essential. This section will cover how to create a styled dropdown, customize its appearance, and enhance user experience.
HTML Structure for Dropdown
A simple dropdown can be created using the `
Basic CSS Styles
To enhance the visual appeal of the dropdown, apply CSS styles. Here are some common properties you can modify:
css
#myDropdown {
width: 200px;
padding: 10px;
border: 2px solid #4CAF50;
border-radius: 5px;
background-color: #f9f9f9;
font-size: 16px;
color: #333;
cursor: pointer;
}
#myDropdown option {
padding: 10px;
}
Custom Dropdown with CSS and JavaScript
For a more advanced and customizable dropdown, consider creating a custom dropdown menu using div elements. This approach allows for more detailed styling and interactivity.
CSS for Custom Dropdown
Apply the following CSS to style the custom dropdown:
css
.custom-dropdown {
position: relative;
display: inline-block;
}
.dropbtn {
background-color: #4CAF50;
color: white;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
border-radius: 5px;
}
.dropdown-content {
display: none;
position: absolute;
background-color: #f9f9f9;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
}
.dropdown-content a {
color: black;
padding: 12px 16px;
text-decoration: none;
display: block;
}
.dropdown-content a:hover {
background-color: #ddd;
}
.custom-dropdown:hover .dropdown-content {
display: block;
}
JavaScript for Interaction
To add functionality to the custom dropdown, include JavaScript to handle user interactions, such as opening and closing the dropdown.
javascript
document.querySelector(‘.dropbtn’).addEventListener(‘click’, function() {
document.querySelector(‘.dropdown-content’).classList.toggle(‘show’);
});
window.onclick = function(event) {
if (!event.target.matches(‘.dropbtn’)) {
var dropdowns = document.getElementsByClassName(“dropdown-content”);
for (var i = 0; i < dropdowns.length; i++) {
var openDropdown = dropdowns[i];
if (openDropdown.classList.contains('show')) {
openDropdown.classList.remove('show');
}
}
}
};
Accessibility Considerations
When styling and creating custom dropdowns, ensure they are accessible:
- Use semantic HTML elements where possible.
- Implement keyboard navigation.
- Provide aria attributes for screen readers.
These practices ensure that all users can interact with your dropdown effectively, enhancing overall usability.
Expert Insights on Styling JavaScript Dropdowns
Emily Carter (UI/UX Designer, Creative Tech Solutions). “When styling JavaScript dropdowns, it is essential to prioritize user experience. Utilize CSS transitions to create smooth animations, and ensure that the dropdown is accessible by using ARIA attributes. This approach not only enhances aesthetics but also improves usability for all users.”
James Lin (Front-End Developer, CodeCrafters Inc.). “Incorporating custom styles into JavaScript dropdowns can significantly elevate the visual appeal of a website. I recommend using a combination of Flexbox for layout and CSS variables for theming, which allows for easy adjustments and a cohesive design throughout the application.”
Sarah Thompson (Web Accessibility Specialist, Inclusive Design Group). “Styling JavaScript dropdowns should not compromise accessibility. It is crucial to ensure that all interactive elements are keyboard navigable and that color contrasts meet WCAG standards. This ensures that dropdowns are usable by individuals with disabilities, promoting an inclusive web experience.”
Frequently Asked Questions (FAQs)
How can I change the background color of a JavaScript dropdown?
You can change the background color of a dropdown by using CSS. Apply a custom class to the `
What CSS properties can I use to style the dropdown options?
You can use properties such as `color`, `font-size`, `padding`, and `border` to style the dropdown options. However, keep in mind that the styling of options may be limited by the browser’s default rendering.
Is it possible to add images to dropdown options using JavaScript?
Yes, you can create a custom dropdown using HTML, CSS, and JavaScript to include images. Standard `
How do I make a dropdown responsive using CSS?
To make a dropdown responsive, use relative units like percentages or `vw` for width, and ensure that the dropdown’s parent container is flexible. Media queries can also help adjust styles for different screen sizes.
Can I use JavaScript to dynamically change the dropdown styles?
Yes, you can use JavaScript to dynamically change styles by accessing the dropdown element through the DOM and modifying its `style` properties or adding/removing CSS classes based on user interactions.
What libraries can assist in styling JavaScript dropdowns?
Libraries like jQuery UI, Select2, and Bootstrap provide enhanced dropdown components with customizable styles and functionalities, making it easier to implement advanced features.
Styling a JavaScript dropdown involves a combination of HTML, CSS, and JavaScript to create an aesthetically pleasing and functional user interface component. The process typically begins with the creation of the dropdown structure using HTML, followed by the application of CSS for visual styling. This includes setting properties such as colors, fonts, and spacing, which can significantly enhance the user experience. Additionally, JavaScript is used to manage the dropdown’s behavior, such as opening and closing the menu, handling user interactions, and ensuring accessibility.
One of the key takeaways is the importance of maintaining a balance between functionality and design. A well-styled dropdown should not only look good but also be intuitive and easy to use. Utilizing CSS frameworks or libraries can expedite the styling process and ensure consistency across different browsers and devices. Furthermore, incorporating responsive design principles is crucial, as it allows the dropdown to adapt seamlessly to various screen sizes, enhancing usability on mobile devices.
Another significant insight is the role of accessibility in dropdown styling. It is essential to ensure that dropdowns are navigable via keyboard and screen readers, making them inclusive for all users. Implementing ARIA (Accessible Rich Internet Applications) attributes can improve the accessibility of dropdown menus, ensuring that they are usable
Author Profile
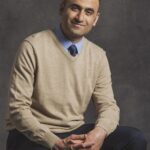
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?