How Can You Style a JavaScript Dropdown Using Styled Components?
### Introduction
In the ever-evolving world of web development, creating visually appealing and interactive user interfaces is paramount. One popular approach to achieving this is through the use of styled-components, a library that allows developers to write CSS directly within their JavaScript code. Among the myriad of UI components, dropdowns are essential for enhancing user experience, providing a clean and efficient way to present options without cluttering the interface. In this article, we will explore how to effectively style dropdown components using styled-components in JavaScript, ensuring that your dropdowns not only function seamlessly but also align perfectly with your design vision.
Dropdowns are more than just functional elements; they are a critical part of user interaction that can significantly impact usability and aesthetics. By leveraging styled-components, developers can create custom dropdowns that are both dynamic and visually cohesive with the rest of the application. This approach allows for greater flexibility in styling, enabling you to tailor each dropdown’s appearance to match your brand’s identity while maintaining a clean and maintainable codebase.
As we delve deeper into the world of styled-components, we will uncover techniques to enhance the dropdown experience, from basic styling to advanced animations and responsive designs. Whether you are a seasoned developer or just starting out, mastering the art of styling dropdowns with styled-components
Creating a Styled Dropdown with Styled Components
To create a dropdown menu using Styled Components in JavaScript, you can follow a structured approach that emphasizes both functionality and styling. Begin by setting up the styled components necessary for the dropdown and then integrate them into your React component.
Setting Up Styled Components
First, ensure you have Styled Components installed in your project. You can install it via npm:
bash
npm install styled-components
Next, create styled components for the dropdown container, the toggle button, and the dropdown items. Here’s an example of how to structure these components:
javascript
import styled from ‘styled-components’;
const DropdownContainer = styled.div`
position: relative;
display: inline-block;
`;
const DropdownButton = styled.button`
background-color: #4caf50;
color: white;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
&:hover {
background-color: #45a049;
}
`;
const DropdownList = styled.ul`
position: absolute;
background-color: white;
border: 1px solid #ccc;
list-style: none;
padding: 0;
margin: 0;
width: 100%;
display: ${props => (props.isOpen ? ‘block’ : ‘none’)};
`;
const DropdownItem = styled.li`
padding: 10px;
&:hover {
background-color: #f1f1f1;
}
`;
Implementing the Dropdown Logic
Now that you have the styled components, you can implement the dropdown logic in your React component. Below is a sample implementation:
javascript
import React, { useState } from ‘react’;
const Dropdown = () => {
const [isOpen, setIsOpen] = useState();
const toggleDropdown = () => {
setIsOpen(!isOpen);
};
return (
);
};
export default Dropdown;
Styling Considerations
When styling dropdowns with Styled Components, consider the following:
- Responsive Design: Ensure the dropdown adapts to different screen sizes. Use media queries within your styled components if necessary.
- Accessibility: Implement keyboard navigation and ARIA attributes for improved accessibility.
- Animations: You can add CSS transitions to enhance the visual experience when toggling the dropdown.
Example of Responsive Styling
You might want to apply responsive styles to your dropdown components. Here’s how you can modify the button to ensure it maintains a good appearance on smaller screens:
javascript
const DropdownButton = styled.button`
background-color: #4caf50;
color: white;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
@media (max-width: 600px) {
font-size: 14px;
padding: 8px;
}
&:hover {
background-color: #45a049;
}
`;
Example Table of Dropdown Options
You may also want to present the dropdown items in a tabular format within your dropdown list. Below is an example of how to include a table:
Option | Description |
---|---|
Option 1 | This is the first option. |
Option 2 | This is the second option. |
Option 3 | This is the third option. |
By following these guidelines, you can create a visually appealing and functional dropdown using Styled Components in your JavaScript project.
Creating a Styled Components Dropdown
Using Styled Components in JavaScript, specifically with React, allows for the creation of dynamic and visually appealing dropdown menus. Here’s a comprehensive approach to implementing a styled dropdown component.
Setting Up the Project
To begin, ensure you have a React project set up. You can use Create React App for a quick setup. Install Styled Components by running:
bash
npm install styled-components
Building the Dropdown Component
Create a new component file named `Dropdown.js`. This component will consist of a button to toggle the dropdown and a list of options.
javascript
import React, { useState } from ‘react’;
import styled from ‘styled-components’;
const DropdownContainer = styled.div`
position: relative;
display: inline-block;
`;
const DropdownButton = styled.button`
background-color: #3498db;
color: white;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
&:hover {
background-color: #2980b9;
}
`;
const DropdownList = styled.ul`
position: absolute;
background-color: white;
border: 1px solid #ccc;
margin: 0;
padding: 0;
list-style: none;
width: 100%;
z-index: 1;
display: ${({ isOpen }) => (isOpen ? ‘block’ : ‘none’)};
`;
const DropdownItem = styled.li`
padding: 10px;
cursor: pointer;
&:hover {
background-color: #f1f1f1;
}
`;
const Dropdown = ({ options }) => {
const [isOpen, setIsOpen] = useState();
const toggleDropdown = () => setIsOpen(!isOpen);
return (
{options.map((option, index) => (
{option}
))}
);
};
export default Dropdown;
Usage of the Dropdown Component
To use the dropdown component, import it into your main application file (e.g., `App.js`) and pass an array of options.
javascript
import React from ‘react’;
import Dropdown from ‘./Dropdown’;
const App = () => {
const options = [‘Option 1’, ‘Option 2’, ‘Option 3’];
return (
Styled Dropdown Example
);
};
export default App;
Styling Considerations
When styling your dropdown, consider the following aspects:
– **Color Scheme**: Choose colors that align with your overall application theme.
– **Accessibility**: Ensure that the dropdown is navigable via keyboard and screen readers.
– **Transitions**: Add CSS transitions to enhance the user experience when opening and closing the dropdown.
Example of adding a transition:
javascript
const DropdownList = styled.ul`
transition: all 0.3s ease;
opacity: ${({ isOpen }) => (isOpen ? ‘1’ : ‘0’)};
visibility: ${({ isOpen }) => (isOpen ? ‘visible’ : ‘hidden’)};
`;
Component Structure
This styled dropdown component is modular and can be easily integrated into any React application. By leveraging Styled Components, you can maintain a clean and scalable codebase while ensuring your dropdown is visually appealing and functional.
Expert Insights on Styling Dropdowns with Styled Components in JavaScript
Emily Carter (Frontend Developer, Tech Innovations Inc.). “When styling dropdowns using Styled Components in JavaScript, it’s crucial to leverage the power of props to create dynamic styles. This allows for a more flexible and reusable component that can adapt to different themes or user interactions.”
Michael Chen (UI/UX Designer, Creative Solutions). “A well-styled dropdown should not only look appealing but also enhance user experience. Utilizing Styled Components enables you to encapsulate styles and maintain a clean component structure, making it easier to manage complex dropdown states.”
Sarah Patel (Software Engineer, Modern Web Development). “Incorporating animations and transitions within Styled Components can significantly improve the visual feedback of dropdowns. By using keyframes and transitions, you can create a more engaging interaction that draws users in.”
Frequently Asked Questions (FAQs)
How do I create a dropdown using styled-components in JavaScript?
To create a dropdown using styled-components, define a styled component for the dropdown container and the options. Use React state to manage the visibility of the dropdown and handle the selection of options.
Can I customize the styles of a dropdown with styled-components?
Yes, styled-components allows you to customize the styles of your dropdown extensively. You can define styles for the dropdown container, options, and any hover effects using template literals and props.
How do I handle dropdown selection in a styled-components dropdown?
To handle dropdown selection, you can create an onClick event for each option. Update the component’s state with the selected value, and conditionally render the dropdown based on that state.
Is it possible to add animations to the dropdown using styled-components?
Absolutely. You can use CSS transitions or animations within styled-components to add effects such as fading in and out or sliding down when the dropdown is opened or closed.
Can I use icons in my styled-components dropdown?
Yes, you can incorporate icons into your styled-components dropdown. Simply include an icon component within the styled option components and style them accordingly.
What are the benefits of using styled-components for dropdowns in JavaScript?
Using styled-components for dropdowns provides scoped styles, dynamic styling based on props, and a cleaner component structure. It enhances maintainability and readability of your code.
In summary, styling a dropdown component using styled-components in JavaScript involves a systematic approach that combines the power of React with the flexibility of CSS-in-JS. By utilizing styled-components, developers can create reusable and encapsulated styles that enhance the maintainability of their code. The process typically includes defining the dropdown’s structure, applying styles to various states such as hover and focus, and ensuring responsiveness across different devices.
Key takeaways from this discussion highlight the importance of leveraging styled-components for better organization and readability of styles. This method allows for dynamic styling based on props, enabling developers to create interactive and visually appealing dropdowns. Additionally, incorporating accessibility features is crucial to ensure that the dropdown is usable for all users, including those who rely on keyboard navigation and screen readers.
Furthermore, testing the dropdown component across various browsers and devices is essential to ensure consistent user experience. By following best practices in styling and component design, developers can create dropdowns that not only function well but also align with the overall aesthetic of the application. Overall, mastering styled-components for dropdowns can significantly enhance the development workflow and user interface quality in JavaScript applications.
Author Profile
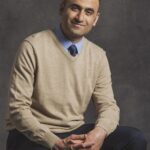
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?