How Can You Subscribe to a URL in Angular?
In the dynamic world of web development, Angular has emerged as a powerful framework that enables developers to create robust and scalable applications. One of the key features that makes Angular so versatile is its ability to handle asynchronous operations seamlessly. Among these operations, subscribing to URLs is a fundamental aspect that allows developers to manage data retrieval and user interactions efficiently. Whether you’re building a simple application or a complex enterprise solution, understanding how to subscribe to URLs in Angular is essential for enhancing user experience and maintaining data integrity.
Subscribing to URLs in Angular involves leveraging the framework’s built-in services and observables to listen for changes or updates in the data stream. This process is crucial for applications that require real-time data updates or need to respond to user inputs dynamically. By mastering this technique, developers can create applications that are not only responsive but also capable of handling multiple data sources without compromising performance.
As you delve deeper into this topic, you’ll discover various methods and best practices for subscribing to URLs, including the use of Angular’s HttpClient and Router modules. Understanding these concepts will empower you to build applications that are both efficient and user-friendly, ensuring that your development skills remain sharp in an ever-evolving technological landscape. Get ready to explore the intricacies of URL subscriptions in Angular and unlock the full potential
Understanding Observables in Angular
In Angular, subscribing to a URL typically involves making HTTP requests using the `HttpClient` module, which returns an observable. Observables are a powerful feature of the RxJS library that Angular uses for handling asynchronous operations. When you subscribe to an observable, you essentially register a callback function that will be executed whenever new data is emitted.
The basic syntax for subscribing to an observable involves the following steps:
- Import `HttpClient` from `@angular/common/http`.
- Inject the `HttpClient` service into your component or service.
- Call a method like `get()`, `post()`, etc., on the `HttpClient` instance to return an observable.
- Use the `subscribe()` method to handle the data or errors returned from the request.
Here is a simple code example illustrating these steps:
“`typescript
import { Component } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
@Component({
selector: ‘app-example’,
templateUrl: ‘./example.component.html’,
})
export class ExampleComponent {
constructor(private http: HttpClient) {}
fetchData() {
this.http.get(‘https://api.example.com/data’)
.subscribe(
data => {
console.log(‘Data received:’, data);
},
error => {
console.error(‘Error occurred:’, error);
}
);
}
}
“`
Subscribing to a URL with Parameters
In some cases, you may need to send parameters along with your request. This can be accomplished by using the `HttpParams` class from `@angular/common/http`. Below is an example that demonstrates how to include URL parameters in your subscription.
“`typescript
import { HttpParams } from ‘@angular/common/http’;
fetchDataWithParams() {
const params = new HttpParams()
.set(‘param1’, ‘value1’)
.set(‘param2’, ‘value2’);
this.http.get(‘https://api.example.com/data’, { params })
.subscribe(
data => {
console.log(‘Data with parameters:’, data);
},
error => {
console.error(‘Error occurred with parameters:’, error);
}
);
}
“`
Handling Multiple Subscriptions
When working with multiple observables, it’s essential to manage subscriptions effectively to avoid memory leaks. You can use operators like `forkJoin`, `combineLatest`, or `merge` to manage multiple requests. Here is a comparison of these operators:
Operator | Description | Use Case |
---|---|---|
forkJoin | Waits for all observables to complete and returns the last emitted value from each. | When you need results from multiple requests simultaneously. |
combineLatest | Emits the latest values from each observable whenever any observable emits. | When you need real-time updates from multiple sources. |
merge | Combines multiple observables into a single observable, emitting values as they come. | When you want to handle multiple streams of data independently. |
Using these operators allows you to handle multiple HTTP requests more elegantly while ensuring that your application remains efficient and responsive.
Understanding Observables in Angular
In Angular, the foundation for subscribing to URLs is built on Observables, which are a part of the RxJS library. Observables represent a stream of data that can be observed over time, allowing for asynchronous programming. When working with HTTP requests, Angular’s `HttpClient` returns Observables that can be subscribed to in order to handle data responses.
Key features of Observables include:
- Laziness: Observables are not executed until they are subscribed to.
- Composability: They can be combined using various operators to create complex data flows.
- Multiple Values: Observables can emit multiple values over time.
Subscribing to an Observable URL
To subscribe to a URL in Angular, follow these steps:
- Import the necessary modules:
Ensure you import `HttpClientModule` in your Angular module and `HttpClient` in the service where you will make the HTTP request.
“`typescript
import { HttpClientModule } from ‘@angular/common/http’;
import { HttpClient } from ‘@angular/common/http’;
“`
- Inject HttpClient into your service:
In your Angular service, inject `HttpClient` via the constructor to enable HTTP requests.
“`typescript
constructor(private http: HttpClient) { }
“`
- Create a method to fetch data:
Define a method that calls an API endpoint and returns an Observable.
“`typescript
getData(url: string): Observable
return this.http.get(url);
}
“`
- **Subscribe to the Observable**:
In your component, call the method from the service and subscribe to it to handle the response.
“`typescript
this.myService.getData(‘https://api.example.com/data’)
.subscribe(response => {
console.log(response);
}, error => {
console.error(‘Error fetching data:’, error);
});
“`
Handling Errors and Unsubscribing
It is crucial to handle errors properly and manage subscriptions to avoid memory leaks. Here are some best practices:
– **Error Handling**:
Use the second argument of `subscribe` to handle errors gracefully.
“`typescript
this.myService.getData(‘https://api.example.com/data’)
.subscribe({
next: response => console.log(response),
error: err => console.error(‘Error:’, err)
});
“`
– **Unsubscribing**:
To prevent memory leaks, you should unsubscribe from Observables when they are no longer needed, especially in components.
“`typescript
private subscription: Subscription;
ngOnInit() {
this.subscription = this.myService.getData(‘https://api.example.com/data’)
.subscribe(response => console.log(response));
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
“`
Using Async Pipe in Templates
Instead of manually subscribing in the component, you can use Angular’s Async Pipe in templates. This approach automatically subscribes and unsubscribes as needed.
- Return the Observable from the service:
No changes are needed in your service method.
- Use Async Pipe in the template:
In your component’s HTML, use the async pipe to bind the Observable directly to the template.
“`html
{{ data | json }}
“`
This method simplifies the code and efficiently manages subscriptions for you.
Expert Insights on Subscribing to URLs in Angular
Dr. Emily Carter (Senior Software Engineer, Angular Development Group). “Subscribing to a URL in Angular typically involves utilizing the HttpClient module. By making an HTTP request to the desired endpoint, developers can leverage observables to handle asynchronous data streams effectively.”
Mark Thompson (Lead Frontend Architect, Tech Innovations Inc.). “When subscribing to a URL in Angular, it is crucial to manage subscriptions properly to avoid memory leaks. Using the async pipe in templates or implementing unsubscribe logic in the component lifecycle can greatly enhance performance.”
Lisa Tran (Angular Consultant, CodeCraft Solutions). “In Angular, subscribing to a URL is not just about fetching data; it is also about error handling and response management. Implementing proper error handling mechanisms within your subscription can significantly improve user experience.”
Frequently Asked Questions (FAQs)
How do I subscribe to a URL in Angular?
To subscribe to a URL in Angular, use the HttpClient module to make an HTTP request. Import the HttpClientModule in your app module, inject HttpClient into your service or component, and use the `get()` method to fetch data from the URL. Then, subscribe to the observable returned by the `get()` method.
What is the purpose of subscribing to an observable in Angular?
Subscribing to an observable in Angular allows you to receive data emitted by the observable. It enables you to handle asynchronous data streams, update your UI with the fetched data, and manage error handling effectively.
Can I unsubscribe from a URL subscription in Angular?
Yes, you can unsubscribe from a URL subscription in Angular to prevent memory leaks. Use the `unsubscribe()` method on the subscription object returned by the `subscribe()` method. It is recommended to unsubscribe in the `ngOnDestroy()` lifecycle hook.
What happens if I don’t unsubscribe from an observable in Angular?
If you don’t unsubscribe from an observable in Angular, it may lead to memory leaks. The observable will continue to emit values even after the component is destroyed, which can consume resources and potentially cause performance issues.
How can I handle errors when subscribing to a URL in Angular?
You can handle errors by providing an error callback function in the `subscribe()` method. Alternatively, use the `catchError` operator from RxJS to manage errors more gracefully within your observable chain.
Is it possible to use async/await with HTTP requests in Angular?
Yes, you can use async/await with HTTP requests in Angular by converting the observable to a promise using the `toPromise()` method. However, it’s recommended to stick with observables for better integration with Angular’s reactive programming model.
Subscribing to a URL in Angular typically involves using the HttpClient module to make HTTP requests. This process allows developers to interact with APIs and retrieve data effectively. By utilizing observables, Angular provides a robust way to handle asynchronous data streams, making it easy to subscribe to the responses from HTTP calls. The HttpClient service simplifies the process of making GET, POST, PUT, and DELETE requests, and it automatically handles JSON data, which is commonly used in web applications.
When subscribing to a URL, it is essential to manage the subscription properly to avoid memory leaks. Developers should unsubscribe from observables when they are no longer needed, particularly in components that may be destroyed or recreated during the application lifecycle. This can be achieved using the `ngOnDestroy` lifecycle hook or by employing the `takeUntil` operator in conjunction with a subject to manage the subscription lifecycle effectively.
In summary, subscribing to a URL in Angular involves leveraging the HttpClient module to perform HTTP requests and manage responses using observables. Proper subscription management is crucial for maintaining application performance and preventing memory leaks. By following best practices in Angular’s reactive programming paradigm, developers can create efficient and responsive applications that seamlessly interact with external data sources.
Author Profile
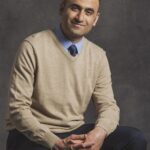
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?