How Can You Easily Subtract Numbers in Python?
### Introduction
In the world of programming, mastering basic operations is essential for building a solid foundation. Among these operations, subtraction stands out as one of the simplest yet most crucial mathematical functions. Whether you’re a beginner taking your first steps into Python or an experienced coder looking to brush up on your skills, understanding how to subtract in Python can enhance your coding efficiency and problem-solving capabilities. This article will guide you through the nuances of subtraction in Python, empowering you to perform calculations with ease and precision.
Subtraction in Python is straightforward, thanks to the language’s intuitive syntax and robust mathematical capabilities. At its core, Python treats subtraction as an operation between two numerical values, allowing you to manipulate integers, floats, and even complex numbers with a few simple commands. The beauty of Python lies in its versatility; you can easily integrate subtraction into larger algorithms, making it a fundamental skill for any aspiring programmer.
As we delve deeper into the topic, we’ll explore various methods of performing subtraction, from basic arithmetic to more advanced applications. You’ll learn how to handle different data types, manage errors, and even implement subtraction in real-world scenarios. By the end of this article, you’ll not only know how to subtract in Python but also appreciate the broader implications of mathematical operations in programming. So, let’s
Basic Subtraction Syntax
In Python, subtraction is performed using the minus sign (`-`). This operator can be used with integers, floats, and even complex numbers. Here’s a simple example of how subtraction is implemented:
python
result = 10 – 5
print(result) # Output: 5
This straightforward syntax allows for easy implementation of subtraction in various contexts, from basic arithmetic to more complex calculations.
Subtracting Variables
You can also subtract values stored in variables. This is particularly useful when dealing with dynamic data. Here’s an example:
python
a = 15
b = 6
result = a – b
print(result) # Output: 9
In this case, the values of `a` and `b` are subtracted, demonstrating how variables can be utilized in arithmetic operations.
Subtracting from Lists
When working with lists, subtraction can be handled in various ways, depending on the intended outcome. One common approach is using list comprehensions to subtract corresponding elements from two lists of equal length:
python
list1 = [10, 20, 30]
list2 = [1, 2, 3]
result = [a – b for a, b in zip(list1, list2)]
print(result) # Output: [9, 18, 27]
Here, the `zip` function pairs the elements of the two lists, allowing for element-wise subtraction.
Subtraction with NumPy
For more advanced numerical operations, the NumPy library is invaluable. It allows for efficient subtraction of arrays. Here’s how you can use NumPy for subtraction:
python
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([1, 2, 3])
result = array1 – array2
print(result) # Output: [ 9 18 27]
Using NumPy not only simplifies the syntax but also enhances performance, especially with large datasets.
Subtraction with Error Handling
When performing subtraction, especially with user inputs or external data, it’s vital to incorporate error handling. This can be done using try-except blocks to manage potential exceptions:
python
try:
a = float(input(“Enter first number: “))
b = float(input(“Enter second number: “))
result = a – b
print(“Result:”, result)
except ValueError:
print(“Please enter valid numbers.”)
This code ensures that if the user inputs an invalid value, a friendly error message is displayed rather than crashing the program.
Subtraction Table
The following table illustrates the results of subtracting various numbers:
Minuend | Subtrahend | Result |
---|---|---|
10 | 5 | 5 |
20 | 15 | 5 |
30 | 10 | 20 |
50.5 | 20.2 | 30.3 |
This table serves as a quick reference for understanding the subtraction operation across different values.
Basic Subtraction in Python
In Python, subtraction can be performed using the minus operator (`-`). This operator can be utilized with integers, floats, and even complex numbers.
Example:
python
# Subtracting two integers
result = 10 – 5
print(result) # Output: 5
# Subtracting two floats
result = 10.5 – 2.5
print(result) # Output: 8.0
Subtracting Multiple Numbers
When subtracting multiple numbers, you can chain the operations together. Python evaluates the expression from left to right.
Example:
python
result = 20 – 5 – 3
print(result) # Output: 12
Alternatively, you can use the built-in `reduce` function from the `functools` module for more complex scenarios.
Example:
python
from functools import reduce
numbers = [20, 5, 3]
result = reduce(lambda x, y: x – y, numbers)
print(result) # Output: 12
Subtraction with Variables
Subtraction can also be performed using variables. This is useful for calculations where the values may change dynamically.
Example:
python
a = 15
b = 7
result = a – b
print(result) # Output: 8
Handling Negative Results
Python naturally handles negative results when the minuend (the number from which another is subtracted) is smaller than the subtrahend (the number being subtracted).
Example:
python
result = 5 – 10
print(result) # Output: -5
Using Subtraction in Functions
Subtraction can be incorporated into functions to enhance code modularity and reusability.
Example:
python
def subtract(x, y):
return x – y
result = subtract(10, 4)
print(result) # Output: 6
Subtraction with Lists
When working with lists, subtraction can be performed element-wise. This can be achieved using list comprehensions or numpy for more advanced operations.
Using List Comprehensions:
python
list1 = [10, 20, 30]
list2 = [1, 2, 3]
result = [x – y for x, y in zip(list1, list2)]
print(result) # Output: [9, 18, 27]
Using Numpy:
python
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([1, 2, 3])
result = array1 – array2
print(result) # Output: [ 9 18 27]
Subtraction with Error Handling
When performing arithmetic operations, it’s important to consider potential errors, such as subtracting incompatible types. Using try-except blocks can help manage these exceptions.
Example:
python
def safe_subtract(x, y):
try:
return x – y
except TypeError:
return “Error: Incompatible types for subtraction.”
result = safe_subtract(10, ‘5’)
print(result) # Output: Error: Incompatible types for subtraction.
Subtraction in Python is straightforward and can be performed in various ways, accommodating both simple arithmetic and more complex scenarios involving lists and functions. Proper error handling ensures that operations are robust against invalid inputs.
Expert Insights on Subtracting in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Subtracting numbers in Python is straightforward, as it utilizes the minus operator (-). This operator can be applied to integers and floats, making it versatile for various applications in programming.”
Michael Chen (Python Programming Instructor, Code Academy). “When teaching subtraction in Python, I emphasize the importance of understanding data types. Ensuring that the values being subtracted are compatible types—like both being integers or floats—can prevent runtime errors.”
Sarah Thompson (Data Scientist, Analytics Solutions). “In data analysis, subtracting values from datasets can be performed using libraries like NumPy. This allows for efficient computation on large arrays, which is crucial for handling big data.”
Frequently Asked Questions (FAQs)
How do I perform subtraction in Python?
You can perform subtraction in Python using the minus operator (`-`). For example, `result = a – b` subtracts `b` from `a`.
Can I subtract multiple numbers in one line?
Yes, you can subtract multiple numbers in one line by chaining the subtraction operator. For example, `result = a – b – c` subtracts both `b` and `c` from `a`.
What happens if I subtract a larger number from a smaller number?
If you subtract a larger number from a smaller number, Python will return a negative result. For example, `result = 5 – 10` will yield `-5`.
Is it possible to subtract floating-point numbers in Python?
Yes, Python supports subtraction of floating-point numbers. For example, `result = 5.5 – 2.3` will yield `3.2`.
Can I use variables for subtraction in Python?
Absolutely. You can use variables to store numbers and perform subtraction. For instance, `x = 10` and `y = 4` allows you to compute `result = x – y`.
What is the output type of a subtraction operation in Python?
The output type of a subtraction operation in Python depends on the types of the operands. If both operands are integers, the result is an integer. If either operand is a float, the result is a float.
In Python, subtraction is a straightforward operation that can be performed using the minus sign (-) operator. This operator works with various data types, including integers, floats, and even complex numbers. Python’s dynamic typing allows for seamless subtraction between compatible types, making it a versatile choice for mathematical operations. Additionally, Python supports the use of built-in functions and libraries that can enhance mathematical capabilities, such as NumPy for array operations.
When performing subtraction, it is essential to consider the data types involved, as subtracting incompatible types will result in a TypeError. For instance, subtracting a string from an integer is not permissible. Moreover, Python’s handling of negative numbers and zero is intuitive, allowing users to easily manipulate values in mathematical expressions. Understanding operator precedence is also crucial, as it affects the order of operations in complex calculations.
In summary, subtracting in Python is a fundamental operation that is easy to implement and understand. The use of the minus operator, combined with Python’s dynamic typing and support for various data types, makes it a powerful tool for developers and data analysts alike. By leveraging Python’s capabilities, users can efficiently perform subtraction and integrate it into larger mathematical computations.
Author Profile
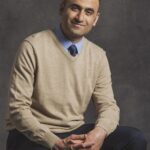
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?