How Can You Effectively Suppress Warnings in Python?
In the world of programming, warnings can often feel like an unwelcome distraction, cluttering your console and drawing your attention away from the tasks that truly matter. As Python developers, we frequently encounter warnings that arise from deprecated features, potential issues in our code, or even third-party libraries that may not align perfectly with our current environment. While these warnings serve an important purpose—alerting us to potential pitfalls—they can sometimes overwhelm us, especially when we’re focused on developing new features or debugging existing ones. So, how do we strike a balance between being informed and maintaining a clean workspace?
In this article, we’ll explore effective strategies for suppressing warnings in Python, allowing you to refine your coding experience without losing sight of critical information. We’ll discuss the various contexts in which warnings may arise, the implications of ignoring them, and the tools Python offers to manage these notifications. By understanding how to control the flow of warnings, you can create a more streamlined development process, enabling you to concentrate on writing clean, efficient code while still being aware of any significant issues that may need your attention.
Whether you’re a seasoned developer or a newcomer to Python, mastering the art of warning suppression can enhance your productivity and improve your coding environment. Join us as we delve
Using the warnings Module
The built-in `warnings` module in Python provides a straightforward way to manage warning messages. This module allows you to filter out warnings based on specific criteria, suppressing them as needed. To suppress warnings, you can use the `filterwarnings` function. Here’s how to do it:
“`python
import warnings
Suppress all warnings
warnings.filterwarnings(“ignore”)
“`
This command will suppress all warnings that would normally be displayed. You can also be more selective in your suppression by specifying the category of warnings you want to ignore. For instance, to ignore only `DeprecationWarning`, you can do the following:
“`python
warnings.filterwarnings(“ignore”, category=DeprecationWarning)
“`
Context Managers for Temporary Suppression
If you want to suppress warnings for a specific block of code rather than globally, you can utilize a context manager. This approach is useful when you only want to ignore warnings in certain areas of your code.
Here’s an example using the `warnings` module:
“`python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
Code that might generate warnings
“`
Within the `with` block, all warnings will be ignored, but outside of it, warnings will be displayed as usual.
Suppressing Warnings in Third-Party Libraries
Sometimes, third-party libraries may generate warnings that are not relevant to your application. You can suppress these warnings selectively by utilizing the `filterwarnings` function with regular expressions. Here is an example:
“`python
warnings.filterwarnings(“ignore”, message=”.*unreachable code detected.*”)
“`
This will ignore any warnings that match the specified message pattern.
Table of Warning Categories
Warning Category | Description |
---|---|
DeprecationWarning | Indicates that a feature is deprecated and may be removed in future releases. |
SyntaxWarning | Alerts about suspicious syntax that may cause issues. |
RuntimeWarning | Warns about dubious runtime behavior, often related to numerical issues. |
FutureWarning | Indicates that a feature may change in the future and could affect your code. |
These warning categories can be targeted for suppression using the methods outlined above.
Using Logging to Manage Warnings
In some cases, it may be preferable to redirect warnings to a logging system instead of suppressing them completely. This can be done by configuring the `warnings` module to work with the `logging` module. Here’s how:
“`python
import warnings
import logging
logging.basicConfig(level=logging.INFO)
warnings.simplefilter(“always”) Show warnings
logging.captureWarnings(True)
Code that generates warnings
“`
By capturing warnings, they will be logged as messages instead of being displayed, allowing you to keep track of them while preventing them from cluttering your output.
By employing these methods, you can effectively manage and suppress warnings in your Python applications, ensuring cleaner and more manageable output during execution.
Using the `warnings` Module
Python provides a built-in module named `warnings` that allows you to control how warnings are issued and displayed. To suppress warnings, you can use the `filterwarnings` function from this module.
- To suppress all warnings:
“`python
import warnings
warnings.filterwarnings(“ignore”)
“`
- To suppress specific warnings, you can provide the category:
“`python
warnings.filterwarnings(“ignore”, category=DeprecationWarning)
“`
You can also reset the warning filters with:
“`python
warnings.resetwarnings()
“`
Suppressing Warnings in Context
Sometimes, you may want to suppress warnings only for a specific block of code. This can be done using the `warnings` context manager:
“`python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
Code that generates warnings goes here
“`
This method ensures that warnings are only suppressed within the context, allowing them to be visible outside of it.
Using the `PYTHONWARNINGS` Environment Variable
You can also control warning suppression through the `PYTHONWARNINGS` environment variable before running your script. This can be particularly useful in production environments.
To suppress all warnings:
“`bash
export PYTHONWARNINGS=”ignore”
“`
To suppress specific warnings:
“`bash
export PYTHONWARNINGS=”ignore::DeprecationWarning”
“`
This approach offers a way to manage warnings without modifying the code.
Suppressing Warnings in Third-Party Libraries
When using third-party libraries that generate warnings, you might want to suppress these warnings without altering the library code. You can still use the `warnings` module effectively:
“`python
import warnings
import some_third_party_lib
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
some_third_party_lib.some_function() Function that raises warnings
“`
This encapsulates the library function call while suppressing any warnings emitted during its execution.
Considerations for Suppressing Warnings
While suppressing warnings can make your output cleaner, it is essential to consider the implications:
- Loss of Important Information: Warnings often indicate potential issues in the code that may need attention.
- Debugging Difficulty: Suppressing warnings may hide critical messages that could aid in debugging.
Hence, it is advisable to use warning suppression judiciously and only in scenarios where it is necessary for clarity or functionality.
Example of Suppressing Warnings
Here is a practical example of suppressing warnings in a Python script:
“`python
import warnings
def deprecated_function():
warnings.warn(“This function is deprecated”, DeprecationWarning)
Suppressing warnings for deprecated_function
with warnings.catch_warnings():
warnings.simplefilter(“ignore”, DeprecationWarning)
deprecated_function() No warning will be printed
Outside the context, the warning will still be shown
deprecated_function() Warning will be printed
“`
This example illustrates how warnings can be managed effectively without altering their inherent functionality.
Expert Insights on Suppressing Warnings in Python
Dr. Emily Carter (Senior Software Engineer, CodeSafe Solutions). “Suppressing warnings in Python can be crucial for maintaining clean output, especially in production environments. Utilizing the `warnings` module, developers can filter out specific warnings or ignore them entirely, allowing for a more streamlined user experience.”
James Liu (Python Developer Advocate, Tech Innovations Inc.). “It is essential to approach warning suppression judiciously. While the `warnings.filterwarnings()` function is effective, developers should ensure they are not inadvertently hiding important alerts that could indicate underlying issues within the code.”
Sarah Thompson (Data Scientist, Analytics Hub). “In data science projects, where libraries often generate warnings, it can be beneficial to suppress these messages during exploratory analysis. However, I recommend documenting any suppression to maintain transparency in the code and avoid potential pitfalls during deployment.”
Frequently Asked Questions (FAQs)
How can I suppress warnings in Python?
You can suppress warnings in Python by using the `warnings` module. Specifically, you can use `warnings.filterwarnings(‘ignore’)` to ignore all warnings.
Is there a way to suppress specific types of warnings?
Yes, you can suppress specific warnings by using `warnings.filterwarnings(‘ignore’, category=WarningType)`, where `WarningType` is the specific warning class you want to suppress.
Can I suppress warnings only for a specific block of code?
Yes, you can suppress warnings for a specific block of code by using the `warnings` context manager. Wrap your code block with `with warnings.catch_warnings():` and set the filter within that context.
What is the difference between `warnings.filterwarnings()` and `warnings.simplefilter()`?
`warnings.filterwarnings()` allows for more granular control over which warnings to suppress, while `warnings.simplefilter()` applies a blanket rule to all warnings of a specified type.
Are there any risks associated with suppressing warnings?
Suppressing warnings can lead to missing important notifications about potential issues in your code, which may result in bugs or unintended behavior. It is advisable to use suppression judiciously.
How can I re-enable warnings after suppressing them?
To re-enable warnings, you can call `warnings.filterwarnings(‘default’)` or reset the filter to its original state using `warnings.resetwarnings()`.
Suppressing warnings in Python can be essential for maintaining clean output, especially when dealing with libraries that generate numerous warnings that may not be relevant to the task at hand. Python provides the `warnings` module, which allows developers to control the visibility of warnings through various functions. The most common approach is using `warnings.filterwarnings()` to specify which warnings to ignore, or using `warnings.simplefilter()` to set a global filter for all warnings.
Additionally, developers can suppress warnings within specific code blocks using the `warnings.catch_warnings()` context manager. This method is particularly useful when you want to ignore warnings for a particular section of code without affecting the entire program. By employing these techniques, developers can ensure that their applications run smoothly without unnecessary interruptions from warnings.
It is important to note that while suppressing warnings can improve the user experience, it should be done judiciously. Ignoring warnings may lead to overlooking potential issues in the code that could affect functionality or performance. Therefore, it is advisable to review the warnings being suppressed and address the underlying causes whenever possible.
Author Profile
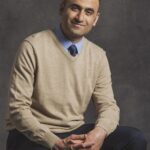
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?