How Can You Easily Swap Variables in Python?
In the world of programming, the ability to manipulate and manage data efficiently is crucial. One fundamental operation that every programmer encounters is the swapping of variables. Whether you’re a novice coder just stepping into the realm of Python or a seasoned developer looking to refine your skills, understanding how to swap variables seamlessly can enhance your coding prowess and streamline your workflow. This seemingly simple task is not only a building block for more complex algorithms but also a testament to Python’s elegant syntax and powerful features.
Swapping variables in Python can be approached in various ways, each with its own advantages and nuances. The traditional method, often seen in other programming languages, involves using a temporary variable to hold one value while the other is assigned. However, Python offers a more intuitive and concise way to achieve this, leveraging its unique tuple unpacking feature. This not only simplifies the code but also makes it more readable and expressive, aligning with Python’s philosophy of simplicity and clarity.
As we delve deeper into the mechanics of variable swapping, we will explore different techniques, compare their efficiency, and highlight scenarios where each method shines. Whether you’re looking to optimize your code or simply understand the underlying principles, mastering the art of variable swapping will undoubtedly bolster your confidence as a Python programmer. Join us as we unravel the intricacies of
Swapping Variables Using Tuple Unpacking
One of the most elegant and Pythonic ways to swap variables is through tuple unpacking. This approach utilizes Python’s ability to handle tuples and multiple assignments in a single line of code. The syntax is straightforward and intuitive, making it a popular choice among Python developers.
To swap two variables `a` and `b`, you can simply use the following syntax:
“`python
a, b = b, a
“`
This line effectively creates a tuple with the values of `b` and `a`, and then unpacks it back into `a` and `b`, thus achieving the swap in a clean and efficient manner.
Swapping Variables Using a Temporary Variable
Another conventional method for swapping two variables is by using a temporary variable. This approach is more explicit and may be familiar to those coming from other programming languages.
Here’s how you can do it:
“`python
temp = a
a = b
b = temp
“`
In this method, a temporary variable `temp` is created to hold the value of `a`. The value of `b` is then assigned to `a`, and finally, the value stored in `temp` is assigned back to `b`. Although this method is clear, it is less concise than tuple unpacking.
Swapping Variables Using Arithmetic Operations
It is also possible to swap variables using arithmetic operations. This method does not require a temporary variable but has some limitations, particularly with data types and potential overflow issues in other programming languages.
You can swap two variables `a` and `b` like this:
“`python
a = a + b
b = a – b
a = a – b
“`
This method works by manipulating the values of `a` and `b` through addition and subtraction. However, it is not commonly used in Python due to readability and the potential for unexpected behavior with non-numeric types.
Comparison of Swapping Methods
The following table summarizes the different methods for swapping variables in Python, highlighting their syntax and key characteristics.
Method | Syntax | Key Characteristics |
---|---|---|
Tuple Unpacking | a, b = b, a |
Concise, Pythonic, and preferred method |
Temporary Variable | temp = a; a = b; b = temp |
Explicit and clear, but more verbose |
Arithmetic Operations | a = a + b; b = a - b; a = a - b |
Less readable, potential for overflow, not recommended |
Each method has its merits, but tuple unpacking is generally favored in Python for its simplicity and readability. Understanding these methods allows developers to choose the most appropriate approach based on context and coding standards.
Swapping Variables in Python
Swapping variables in Python can be achieved through several straightforward methods. Below are the most common approaches used by developers.
Tuple Packing and Unpacking
Python allows for elegant variable swapping using tuple packing and unpacking. This method is both concise and efficient.
“`python
a = 5
b = 10
a, b = b, a
“`
In this example:
- The values of `a` and `b` are packed into a tuple `(10, 5)`.
- Python then unpacks this tuple back into the variables, effectively swapping their values.
Using a Temporary Variable
Another traditional method for swapping variables involves the use of a temporary variable. This approach is universally applicable in many programming languages.
“`python
a = 5
b = 10
temp = a
a = b
b = temp
“`
Here, the steps are:
- A temporary variable `temp` is used to hold the value of `a`.
- The value of `b` is assigned to `a`.
- Finally, the value stored in `temp` is assigned to `b`.
Using Arithmetic Operations
You can also swap variables using arithmetic operations, although this method is less common due to potential risks such as overflow in some languages.
“`python
a = 5
b = 10
a = a + b a now becomes 15
b = a – b b becomes 5 (original value of a)
a = a – b a becomes 10 (original value of b)
“`
This technique works as follows:
- The sum of `a` and `b` is stored in `a`.
- The original value of `a` is then retrieved by subtracting `b` from the sum.
- Finally, the original value of `b` is obtained by subtracting the new value of `b` from the sum.
Using Bitwise XOR
A more unconventional method involves using the XOR bitwise operator. This method is less readable and not recommended for general use but demonstrates an alternative approach.
“`python
a = 5
b = 10
a = a ^ b Step 1
b = a ^ b Step 2
a = a ^ b Step 3
“`
The breakdown of this process is:
- The first step combines `a` and `b` using XOR, storing the result in `a`.
- The second step retrieves the original value of `a` into `b`.
- The third step retrieves the original value of `b` into `a`.
Comparison of Methods
Method | Readability | Efficiency | Risk of Overflow |
---|---|---|---|
Tuple Packing and Unpacking | High | O(1) | No |
Temporary Variable | Medium | O(1) | No |
Arithmetic Operations | Low | O(1) | Yes |
Bitwise XOR | Low | O(1) | No |
In summary, the most Pythonic way to swap variables is by using tuple packing and unpacking, as it is both readable and efficient. Other methods may be used depending on specific requirements or constraints within the codebase.
Expert Insights on Variable Swapping in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Swapping variables in Python is elegantly simple due to the language’s support for tuple unpacking. This feature allows developers to exchange values without the need for a temporary variable, enhancing code readability and efficiency.”
James Liu (Python Developer Advocate, CodeCraft Solutions). “The idiomatic way to swap variables in Python involves a single line of code: `a, b = b, a`. This not only simplifies the process but also reduces the likelihood of errors commonly associated with more verbose swapping methods.”
Dr. Sarah Thompson (Computer Science Professor, University of Technology). “Understanding how to swap variables is fundamental for new programmers. Python’s approach to variable assignment and swapping illustrates the language’s design philosophy of simplicity and clarity, making it an excellent choice for teaching programming concepts.”
Frequently Asked Questions (FAQs)
How do I swap two variables in Python?
You can swap two variables in Python using a single line of code: `a, b = b, a`. This utilizes Python’s tuple unpacking feature to exchange the values.
Is there a built-in function for swapping variables in Python?
Python does not have a specific built-in function for swapping variables. However, the tuple unpacking method is the most efficient and idiomatic way to achieve this.
Can I swap more than two variables at once in Python?
Yes, you can swap multiple variables simultaneously. For example, `a, b, c = c, a, b` will rotate the values among three variables.
What happens if I try to swap variables of different data types?
Swapping variables of different data types in Python is permissible and will not cause any errors. The variables will simply hold the new values regardless of their original types.
Are there any performance considerations when swapping variables in Python?
The tuple unpacking method for swapping variables is efficient and performs well in Python. Performance differences are negligible for typical use cases, but for very large datasets, consider the context of your operations.
Can I swap variables in a function and reflect the changes outside the function?
In Python, swapping variables inside a function will not affect the original variables outside the function unless you return the swapped values or use mutable types like lists or dictionaries to reflect changes.
Swapping variables in Python is a straightforward process that can be accomplished using multiple methods. The most efficient and Pythonic way to swap two variables is by utilizing tuple unpacking. This technique allows for a clean and concise syntax, enabling developers to exchange the values of two variables in a single line of code. For example, one can simply write `a, b = b, a`, which effectively swaps the values of `a` and `b` without the need for a temporary variable.
Another method to swap variables involves using a temporary variable. This traditional approach requires a third variable to hold one of the values during the exchange. While this method is clear and understandable, it is less elegant than tuple unpacking. For instance, the code would look like this: `temp = a; a = b; b = temp`. Although effective, this method is not as concise as the tuple unpacking method.
while there are various ways to swap variables in Python, tuple unpacking stands out as the most efficient and readable option. It not only simplifies the code but also aligns with Python’s design philosophy of readability and simplicity. Developers are encouraged to adopt this method for its clarity and effectiveness in variable manipulation.
Author Profile
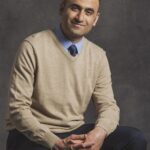
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?