How Can You Effectively Target img src in CSS?
In the world of web design, the visual appeal of a site is often as crucial as its functionality. Images play a pivotal role in capturing attention and conveying messages, but how do you ensure that your images not only look great but also align seamlessly with your overall design? This is where CSS (Cascading Style Sheets) comes into play. By mastering the art of targeting image sources (img src) in CSS, you can elevate your web design to new heights, creating a more polished and professional look. Whether you’re looking to adjust sizes, apply filters, or enhance responsiveness, understanding how to effectively style images is essential for any web developer or designer.
When it comes to styling images, the process may seem straightforward at first glance, but there are nuances that can make a significant difference. CSS offers a variety of selectors and properties that allow you to manipulate images in ways that can enhance user experience and aesthetic appeal. From controlling dimensions and alignment to applying hover effects and transitions, the possibilities are vast. By learning how to target img src in CSS, you can create a cohesive visual narrative that resonates with your audience.
Furthermore, the ability to style images effectively can improve your website’s performance and accessibility. With the right techniques, you can ensure that images load efficiently and
Understanding the img src Attribute
The `img` tag in HTML is used to embed images in web pages. The `src` attribute specifies the path to the image file. To style images using CSS, it is important to recognize that the styling can be applied to the `img` element itself rather than targeting the `src` attribute directly. CSS selectors can be utilized to select the `img` element based on its attributes, classes, or IDs.
Targeting Images with CSS Selectors
To effectively target images using CSS, you can use various selectors. Here are some common methods:
- Element Selector: Targets all `img` elements.
“`css
img {
border: 2px solid black;
}
“`
- Class Selector: Targets images with a specific class.
“`css
.highlighted-image {
border: 2px solid red;
}
“`
- ID Selector: Targets a specific image using its ID.
“`css
main-image {
width: 100%;
height: auto;
}
“`
- Attribute Selector: Targets images based on their `src` attribute.
“`css
img[src=”path/to/image.jpg”] {
opacity: 0.5;
}
“`
Using Attribute Selectors
Attribute selectors provide a powerful way to style elements based on their attributes. When targeting `img` elements, you can specify conditions to match the `src` value:
Selector Type | Example | Description |
---|---|---|
Exact Match | `img[src=”image.jpg”]` | Selects images with an exact `src` value. |
Starts With | `img[src^=”https://”]` | Selects images with `src` values starting with `https://`. |
Ends With | `img[src$=”.png”]` | Selects images with `src` values ending with `.png`. |
Contains | `img[src*=”logo”]` | Selects images with `src` values containing the word “logo”. |
Styling Images Conditionally
In scenarios where you need to apply styles conditionally based on various attributes, you can combine CSS rules with logical operators. For example:
“`css
img[src^=”https://”][alt=”banner”] {
display: block;
margin: auto;
}
“`
This rule targets images that start with `https://` in their `src` attribute and have an `alt` attribute of “banner”. This way, you can ensure that specific styles are applied only to relevant images.
Best Practices for Image Styling
When styling images with CSS, consider the following best practices:
- Use Responsive Units: Use percentages or viewport units to ensure images are responsive.
- Optimize Images: Always optimize images for the web to improve loading times.
- Accessibility: Ensure that all images have appropriate `alt` attributes for screen readers.
- Consistent Naming: Use consistent naming conventions for classes and IDs to maintain clarity in your stylesheets.
By following these guidelines and techniques, you can effectively target and style images in your web projects using CSS.
Targeting Images with CSS
To effectively target images within your HTML using CSS, you can utilize various selectors and properties depending on your specific requirements. Here are the primary methods to achieve this:
Using the `img` Selector
The simplest way to target all images on a page is by using the `img` selector. This method applies styles universally to every image element.
“`css
img {
border: 2px solid black; /* Adds a solid border around all images */
max-width: 100%; /* Ensures images are responsive */
height: auto; /* Maintains aspect ratio */
}
“`
Targeting Specific Images
If you want to apply styles to specific images, you can use classes or IDs. Here’s how to do this:
- Using Classes: This allows you to target multiple images sharing the same class.
“`html
“`
“`css
.thumbnail {
width: 150px; /* Sets a fixed width for thumbnail images */
border-radius: 8px; /* Rounds the corners */
}
“`
- Using IDs: This is useful for styling a unique image.
“`html
“`
“`css
featured-image {
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.5); /* Adds shadow effect */
width: 100%; /* Full width */
}
“`
Targeting Images in Specific Containers
You might need to apply styles to images contained within certain elements. This can be done with descendant selectors.
“`html

“`
“`css
.gallery img {
opacity: 0.8; /* Slightly transparent images */
transition: opacity 0.3s ease; /* Smooth transition for hover effects */
}
.gallery img:hover {
opacity: 1; /* Fully opaque on hover */
}
“`
Responsive Images
To ensure images adapt to varying screen sizes, CSS properties like `max-width` and `height` are critical. Consider the following strategies:
- Fluid Images: Use percentages or relative units for responsive designs.
“`css
img {
max-width: 100%; /* Ensures images do not exceed their container’s width */
height: auto; /* Maintains original aspect ratio */
}
“`
- Media Queries: Adjust image styles for different screen sizes.
“`css
@media (max-width: 600px) {
img {
width: 100%; /* Images take full width on smaller screens */
}
}
“`
Advanced Image Targeting Techniques
For more complex layouts, you may need advanced selectors:
- Attribute Selectors: Target images based on specific attributes.
“`css
img[src$=”.png”] {
border: 1px solid green; /* Styles only PNG images */
}
“`
- Pseudo-classes: Style images based on their state.
“`css
img:hover {
transform: scale(1.05); /* Slightly enlarges image on hover */
}
“`
- Nth-child Selector: Style specific images in a list.
“`css
ul li img:nth-child(2) {
border: 2px dashed red; /* Styles the second image in a list */
}
“`
By employing these various techniques, you can effectively target and style images within your web projects, enhancing both visual appeal and user experience.
Expert Insights on Targeting Image Sources in CSS
Emily Chen (Front-End Developer, CreativeTech Solutions). “To effectively target image sources in CSS, it is crucial to utilize the `background-image` property for elements where images are not directly part of the HTML structure. This allows for greater flexibility in design and responsiveness.”
Michael Thompson (Web Design Consultant, Digital Visionaries). “Using CSS to manipulate the `src` attribute of an `
` tag directly is not possible. Instead, developers should consider using CSS classes or IDs to apply styles to images, ensuring that they maintain a clean separation between content and presentation.”
Sarah Patel (UI/UX Designer, Innovate Design Group). “For advanced targeting of images, CSS selectors can be combined with JavaScript to dynamically change the `src` attribute based on user interaction or other conditions. This approach enhances user experience by providing contextually relevant images.”
Frequently Asked Questions (FAQs)
How can I select an image by its src attribute in CSS?
You cannot directly select an image by its `src` attribute using CSS alone. CSS does not provide a method to target elements based on their attributes. However, you can use JavaScript or jQuery to achieve this.
Is it possible to style an image based on its src attribute?
Yes, you can style an image based on its `src` attribute using JavaScript. By selecting the image element and checking its `src`, you can apply specific styles dynamically.
Can I use attribute selectors in CSS for images?
Yes, you can use attribute selectors in CSS to select elements based on specific attributes. For example, `img[src=”image.jpg”] { /* styles */ }` will apply styles to images with a specific `src`.
What are some common CSS properties I can apply to images?
Common CSS properties for images include `width`, `height`, `border`, `opacity`, and `filter`. These properties can enhance the visual presentation of images.
How to apply different styles to images with different src values?
To apply different styles based on `src` values, use JavaScript to check the `src` and then add a specific class to the image element. You can then define styles for that class in your CSS.
Can I use CSS to hide an image based on its src attribute?
CSS alone cannot hide an image based on its `src` attribute. However, you can achieve this with JavaScript by selecting the image and applying a `display: none;` style based on the `src` value.
targeting the `img` element’s `src` attribute directly through CSS is not possible, as CSS does not have the capability to select or manipulate attributes in the same way that JavaScript does. However, CSS can effectively style `img` elements based on their attributes, classes, or parent elements. By utilizing selectors such as attribute selectors, class selectors, and descendant selectors, developers can achieve the desired visual effects for images within their web pages.
Furthermore, understanding how to apply styles to images can enhance the user experience and contribute to the overall aesthetics of a website. For instance, using classes to group images together allows for consistent styling across multiple elements. Additionally, employing pseudo-classes can help in creating interactive effects, such as hover states, which can engage users more effectively.
Ultimately, while CSS cannot target the `src` attribute directly, it remains a powerful tool for styling images. Developers should consider combining CSS with JavaScript for more dynamic image handling, such as changing the `src` attribute based on user interactions. This approach allows for greater flexibility and control over how images are presented on a website.
Author Profile
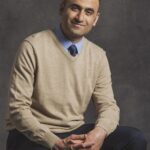
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?