How Can You Easily Uncomment Code in Python?
In the world of programming, comments serve as essential tools for communication, allowing developers to annotate their code, explain complex logic, and provide context for future reference. However, there are times when you may find yourself needing to revert a section of code from a commented state back to an active one. This process, known as uncommenting, is a fundamental skill every Python programmer should master. Whether you’re debugging, collaborating with others, or simply refining your code, understanding how to uncomment effectively can enhance your workflow and improve code readability.
Uncommenting in Python is a straightforward yet vital task that can significantly impact your coding experience. Unlike some programming languages that may have complex comment syntax, Python keeps it simple, allowing developers to easily toggle between commented and uncommented code. This flexibility not only aids in testing and troubleshooting but also helps maintain clarity in collaborative projects where multiple developers may be working on the same codebase.
As you delve deeper into the nuances of uncommenting in Python, you’ll discover various techniques and best practices that can streamline your coding process. From single-line comments to multi-line blocks, mastering the art of uncommenting will empower you to manipulate your code with confidence and precision. Get ready to unlock the full potential of your Python scripts by learning how to manage comments effectively
Uncommenting in Python
Uncommenting in Python is a straightforward process, essential for modifying code during development. In Python, comments are lines of text that the interpreter ignores, which can help document code or disable certain functionalities temporarily. To uncomment a line, you essentially remove the comment marker.
In Python, comments are initiated with the “ symbol. When a line begins with this symbol, the interpreter treats it as a comment. To uncomment, simply delete the “ at the start of the line.
Steps to Uncomment Code
- Locate the Commented Line: Identify the line of code you wish to uncomment.
- Delete the Comment Symbol: Remove the “ symbol from the beginning of that line.
- Review for Syntax Errors: After uncommenting, ensure the line is syntactically correct and fits well within the surrounding code.
Example of Commenting and Uncommenting
Consider the following code snippet where one line is commented out:
“`python
print(“This line is commented out.”)
print(“This line will execute.”)
“`
To uncomment the first line, you would modify it as follows:
“`python
print(“This line is commented out.”)
print(“This line will execute.”)
“`
Tools and Shortcuts
Most integrated development environments (IDEs) and text editors offer shortcuts to uncomment multiple lines of code simultaneously:
- Visual Studio Code: Select the lines and press `Ctrl` + `/` (Windows) or `Cmd` + `/` (Mac).
- PyCharm: Highlight the lines and use `Ctrl` + `/` (Windows/Linux) or `Cmd` + `/` (Mac).
- Sublime Text: Highlight the commented lines and use `Ctrl` + `/` (Windows/Linux) or `Cmd` + `/` (Mac).
Common Practices
When uncommenting code, it’s important to consider the following best practices:
- Review Context: Ensure that uncommented code aligns with the current logic of your program.
- Maintain Readability: Keep your code clean and avoid uncommenting large blocks of code that may confuse other developers.
- Use Version Control: If you’re unsure about uncommenting a significant piece of code, consider using version control systems to track changes.
Action | Keyboard Shortcut | IDE/Text Editor |
---|---|---|
Uncomment Line | Ctrl + / | Visual Studio Code |
Uncomment Line | Cmd + / | Visual Studio Code (Mac) |
Uncomment Line | Ctrl + / | PyCharm |
Uncomment Line | Cmd + / | PyCharm (Mac) |
Uncomment Line | Ctrl + / | Sublime Text |
Uncomment Line | Cmd + / | Sublime Text (Mac) |
By mastering the uncommenting process, you can efficiently manage your code and enhance its functionality, ensuring a smoother development workflow.
Understanding Comments in Python
In Python, comments are designated using the hash symbol (“). Any text following this symbol on the same line is considered a comment and is not executed as part of the program. This is useful for adding notes or explanations within the code.
How to Uncomment Lines in Python
To uncomment a line or a block of code in Python, you simply need to remove the hash symbol from the beginning of the line or lines you wish to execute. Below are the methods for uncommenting code in different scenarios.
Uncommenting Single Lines
When you have a single line of code that is commented out, you can uncomment it by:
- Locating the line with the “ symbol.
- Deleting the “ at the beginning of that line.
Example:
“`python
print(“This line is commented out.”)
print(“This line is active.”)
“`
After Uncommenting:
“`python
print(“This line is commented out.”)
print(“This line is active.”)
“`
Uncommenting Multiple Lines
For multiple lines of code that are commented out, you can uncomment them by removing the “ from each line individually. Alternatively, many Integrated Development Environments (IDEs) or text editors provide shortcuts to uncomment multiple lines simultaneously.
Example:
“`python
print(“First line”)
print(“Second line”)
print(“Third line”)
“`
Uncommenting Manually:
“`python
print(“First line”)
print(“Second line”)
print(“Third line”)
“`
Using IDE Features:
- In Visual Studio Code: Select the lines and use the shortcut `Ctrl` + `K` followed by `Ctrl` + `U`.
- In PyCharm: Select the lines and use `Ctrl` + `/` to toggle comments.
Comments vs. Docstrings
While comments are brief annotations, docstrings are a type of comment used to explain the purpose of a function or module. Docstrings are enclosed in triple quotes (`”’` or `”””`) and can span multiple lines.
Example of a Docstring:
“`python
def example_function():
“””This function does nothing.”””
pass
“`
Uncommenting Docstrings:
To uncomment a docstring, simply remove the triple quotes surrounding it. However, if a function is commented out entirely (using “), follow the same process as with regular comments.
Best Practices for Using Comments
- Clarity: Ensure comments clarify the code rather than repeat it.
- Consistency: Use a consistent commenting style throughout your codebase.
- Relevance: Regularly update comments to reflect changes in the code.
- Avoid Over-commenting: Strive to write self-explanatory code, minimizing the need for comments.
By following these guidelines, you will maintain clean and understandable code while effectively using comments and docstrings.
Expert Insights on Uncommenting in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Uncommenting in Python is a straightforward process, typically involving the removal of the hash symbol () at the beginning of a line. This action restores the line to an executable state, allowing it to be processed by the Python interpreter.”
Michael Chen (Lead Python Instructor, Code Academy). “For beginners, understanding how to uncomment code is crucial. In many code editors, you can also use keyboard shortcuts, such as Ctrl + /, to toggle comments on and off, streamlining the coding process significantly.”
Sarah Thompson (Technical Writer, Python Programming Journal). “It’s important to remember that uncommenting should be done thoughtfully. Ensure that the uncommented code is relevant and necessary for the program’s functionality to avoid introducing errors.”
Frequently Asked Questions (FAQs)
How do I uncomment a single line in Python?
To uncomment a single line in Python, simply remove the “ symbol at the beginning of the line. This will enable the line of code to be executed.
Can I uncomment multiple lines at once in Python?
Yes, you can uncomment multiple lines by selecting the lines and removing the “ symbols simultaneously, depending on your code editor or IDE. Some editors offer shortcuts for this action.
What is the keyboard shortcut to uncomment code in popular IDEs?
In many IDEs, such as PyCharm or Visual Studio Code, you can uncomment selected lines by using `Ctrl + /` on Windows or `Cmd + /` on macOS. This toggles the comment status of the selected lines.
Are there any tools that can help with uncommenting in Python?
Yes, many code editors and IDEs provide built-in features for commenting and uncommenting code, as well as plugins that enhance this functionality. Check the documentation of your specific tool for details.
Is there a difference between commenting and uncommenting in Python?
Yes, commenting involves adding “ to the beginning of a line to prevent it from executing, while uncommenting removes the “, allowing the line to be executed as part of the code.
What happens if I uncomment a line that contains a syntax error?
If you uncomment a line that contains a syntax error, Python will raise an error when you attempt to run the code. It is essential to ensure that the uncommented line is syntactically correct before execution.
In Python, uncommenting code is a straightforward process that involves removing the comment syntax from lines of code. Comments in Python are typically initiated using the hash symbol (), which tells the interpreter to ignore the text that follows on that line. To uncomment a line, one simply needs to delete the hash symbol at the beginning of the line. This action transforms the commented line back into executable code, allowing it to be processed by the Python interpreter.
Additionally, when dealing with multiple lines of code, it is possible to uncomment them in bulk. Many code editors and integrated development environments (IDEs) offer features that allow users to select multiple lines and toggle comments on or off. This can significantly enhance productivity, especially in larger scripts where numerous lines may need to be uncommented simultaneously.
It is also important to recognize the role of comments in programming. While uncommenting is essential for executing previously disabled code, comments serve as a valuable tool for documentation and code readability. They help developers understand the purpose and functionality of specific code segments, making it easier to maintain and collaborate on projects. Therefore, while the act of uncommenting is simple, it is part of a broader practice of writing clear and maintainable code.
Author Profile
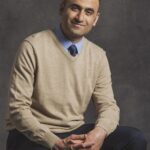
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?