How Can You Effectively Undo a Git Pull?
When working with Git, the version control system that has become the backbone of collaborative software development, the ability to manage changes effectively is crucial. One common scenario that developers face is the need to undo a `git pull`. Whether it’s due to unexpected merges, unwanted changes, or simply a mistake in pulling the latest updates, knowing how to revert a pull can save you from potential headaches and keep your project on track. In this article, we’ll explore the various methods to undo a `git pull`, empowering you to regain control over your codebase with confidence.
Understanding how to undo a `git pull` is essential for maintaining the integrity of your project. When you execute a pull command, Git fetches changes from a remote repository and merges them into your local branch. This process can sometimes introduce conflicts or unwanted changes that you may not be ready to handle. Fortunately, Git provides several tools and commands that allow you to backtrack and restore your previous state, ensuring that you can continue working without the burden of unintended modifications.
As we delve deeper into the topic, we’ll discuss the different scenarios that might require you to undo a pull, the implications of each method, and best practices for managing your Git workflow. Whether you’re a seasoned developer or just starting out, understanding how
Understanding the Impact of a Git Pull
When you execute a `git pull`, you are essentially fetching changes from a remote repository and merging them into your current branch. This operation combines the functionalities of `git fetch` and `git merge`. As a result, if the changes from the remote repository conflict with your local changes, you may end up in a merge conflict situation that requires resolution.
To undo a `git pull`, it is important to first understand the current state of your repository. Here are key considerations:
- Local Changes: If you have uncommitted changes in your working directory, they will be impacted by the pull.
- Merge Conflicts: If the pull resulted in conflicts, you will need to resolve these before proceeding.
- Commit History: It’s essential to know whether a new commit was created as a result of the pull.
Methods to Undo a Git Pull
There are several methods to undo the effects of a `git pull`, depending on the situation:
- Using `git reset`: This method is suitable if you want to revert to the state before the pull.
- Soft Reset: Keeps your changes in the staging area.
“`bash
git reset –soft HEAD~1
“`
- Mixed Reset: Keeps your changes in the working directory but unstaged.
“`bash
git reset HEAD~1
“`
- Hard Reset: Discards all changes, returning to the last commit.
“`bash
git reset –hard HEAD~1
“`
- Using `git reflog`: This command allows you to view your previous HEAD positions.
- Find the reference for the commit before the pull.
- Reset to that commit:
“`bash
git reset –hard
“`
- Using `git revert`: If you want to keep the history intact, you can create a new commit that undoes the changes.
“`bash
git revert HEAD
“`
Choosing the Right Method
The choice of method depends on your specific scenario, as summarized in the following table:
Method | Use Case | Impact on Changes |
---|---|---|
Soft Reset | Undo pull but keep staged changes | Changes remain staged |
Mixed Reset | Undo pull and keep working directory changes | Changes remain in working directory |
Hard Reset | Completely discard changes | All changes lost |
Reflog Reset | Return to a specific previous state | Changes based on selected commit |
Revert | Keep history intact while undoing changes | New commit created |
Each method has its own implications for your project history and working directory, so it is crucial to choose the one that aligns with your needs. Always ensure that you have backups or that you have committed or stashed any important changes before proceeding with any undo operations.
Understanding the Impact of a Git Pull
When executing a `git pull`, you are essentially combining two actions: fetching changes from a remote repository and merging those changes into your current branch. This can lead to potential conflicts or introduce unwanted changes in your working directory. Understanding how to effectively undo a `git pull` is crucial for maintaining the integrity of your codebase.
Identifying the Need to Undo a Git Pull
Before proceeding, it’s important to assess why you need to undo the pull. Common scenarios include:
- Unintended merge conflicts
- of bugs or broken features
- Pulling changes that were not meant for the current branch
Recognizing the reason can help you choose the appropriate method to revert the changes.
Methods to Undo a Git Pull
There are several strategies to undo a `git pull`, depending on the context and your specific needs.
Using `git reset`
If you have not yet committed any changes after the pull, you can use the `git reset` command.
- Soft Reset: Keeps your changes in the working directory.
“`bash
git reset –soft HEAD~1
“`
- Mixed Reset: Resets the index but keeps your working directory intact.
“`bash
git reset HEAD~1
“`
- Hard Reset: Discards all changes, returning to the last commit.
“`bash
git reset –hard HEAD~1
“`
Reset Type | Description | Command |
---|---|---|
Soft | Keeps changes in working directory | `git reset –soft HEAD~1` |
Mixed | Resets index; keeps working changes | `git reset HEAD~1` |
Hard | Discards all changes | `git reset –hard HEAD~1` |
Using `git reflog`
If you have already made commits after the pull, you can use `git reflog` to find the previous state of your branch.
- View the log of all actions:
“`bash
git reflog
“`
- Identify the commit hash before the pull.
- Reset to that commit:
“`bash
git reset –hard
“`
Reverting Commits
If you need to selectively undo changes from the pull without affecting other commits, you can use `git revert`.
- Find the commit hash of the merge:
“`bash
git log
“`
- Revert the specific commit:
“`bash
git revert
“`
This creates a new commit that undoes the changes from the specified commit, preserving the history.
Handling Merge Conflicts
In cases where a `git pull` results in merge conflicts, you must resolve these issues before proceeding. You can either:
- Manually edit the conflicting files.
- Use a merge tool to assist in resolving conflicts.
After resolving conflicts, ensure to commit the changes to finalize the merge.
Best Practices for Future Pulls
To minimize the need to undo a pull in the future, consider the following practices:
- Always fetch changes before merging to review incoming updates.
- Use feature branches to isolate changes until they are ready for integration.
- Regularly commit your changes to avoid losing work during merges.
By implementing these practices, you can enhance your workflow and reduce the frequency of needing to undo `git pull` operations.
Expert Insights on Reversing a Git Pull
Dr. Emily Carter (Senior Software Engineer, CodeMasters Inc.). “To effectively undo a git pull, one can use the command `git reset –hard HEAD~1` to revert to the previous commit. This command discards any changes made during the pull, so it is crucial to ensure that no important work is lost before executing it.”
Michael Chen (DevOps Specialist, Tech Innovations). “If you have already pushed changes after the pull, consider using `git revert` instead. This command creates a new commit that undoes the changes made by the pull, allowing you to maintain a clean history while effectively reversing the unwanted modifications.”
Sarah Patel (Git Training Instructor, LearnGitNow). “It is essential to understand the context of the pull before deciding how to undo it. Using `git reflog` can help you identify the previous state of the repository, enabling you to choose the most appropriate method—whether that’s resetting, reverting, or checking out a specific commit.”
Frequently Asked Questions (FAQs)
How can I undo a git pull if I haven’t made any new commits?
You can undo a git pull by using the command `git reset –hard HEAD@{1}`. This command resets your branch to the state it was in before the last pull, effectively discarding any changes made by the pull.
What if I have made commits after the git pull?
If you have made commits after the git pull, you can use `git reflog` to find the commit hash before the pull and then execute `git reset –hard
Is there a way to undo a git pull without losing changes?
Yes, you can use `git reset –soft HEAD@{1}` to undo the pull while keeping your changes staged. This allows you to review and selectively commit or discard the changes made by the pull.
Can I use git stash before undoing a git pull?
Yes, you can use `git stash` to save your current changes before undoing the pull. After stashing, you can safely perform the undo operation and then apply your stashed changes using `git stash apply`.
What happens to uncommitted changes after a git pull?
Uncommitted changes may lead to merge conflicts during a git pull. If you want to undo the pull and keep your uncommitted changes, it is advisable to stash them first before executing the undo command.
Are there any risks associated with using git reset?
Yes, using `git reset –hard` can lead to permanent loss of uncommitted changes and commits. Always ensure you have backups or have stashed your changes before using this command to avoid unintended data loss.
In summary, undoing a git pull involves several methods depending on the specific scenario and the desired outcome. The most common approach is to use the `git reset` command, which can revert the repository to a previous state before the pull was executed. This method is particularly useful when you want to discard the changes introduced by the pull entirely. Alternatively, if you wish to keep the changes but revert to the previous commit, `git revert` can be employed to create a new commit that undoes the changes from the pull.
Another important aspect to consider is the state of your working directory and whether you have uncommitted changes. If you have local modifications that you want to preserve, using `git stash` before performing a reset or revert can be beneficial. This allows you to temporarily save your changes and reapply them after you have undone the pull.
It is also crucial to understand the implications of each method. Using `git reset` can alter the commit history, which may not be desirable in collaborative environments. In contrast, `git revert` maintains the commit history while effectively canceling out the changes from the pull. Therefore, the choice between these methods should be made with consideration of the project’s workflow and collaboration practices.
Author Profile
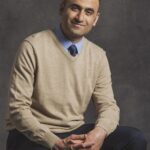
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?