How Can You Effectively Update a Dictionary in Python?
In the dynamic world of programming, dictionaries stand out as one of the most versatile data structures in Python. They allow developers to store and manipulate data in key-value pairs, making it easy to access, update, and manage information efficiently. Whether you’re building a simple application or a complex system, knowing how to update a dictionary is an essential skill that can enhance your coding prowess and streamline your workflows. In this article, we will explore the various methods and best practices for updating dictionaries in Python, empowering you to handle data with confidence and precision.
Updating a dictionary in Python is a straightforward yet powerful operation that can significantly impact how your programs function. From adding new entries to modifying existing ones, the ability to manipulate dictionaries effectively opens up a world of possibilities. You’ll discover that Python provides several intuitive methods to achieve these updates, each suited to different scenarios and use cases. Understanding these techniques not only boosts your coding efficiency but also enhances the readability and maintainability of your code.
As we delve deeper into the topic, we will examine the nuances of dictionary updates, including how to merge dictionaries, remove items, and handle potential pitfalls. Whether you are a beginner looking to grasp the fundamentals or an experienced developer seeking to refine your skills, this comprehensive guide will equip you with the
Updating a Dictionary Using Assignment
To update a dictionary in Python, one of the simplest methods is to use assignment. You can directly assign a new value to an existing key or add a new key-value pair. This method allows for straightforward updates without requiring additional functions or methods.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘a’] = 3 Update the value for key ‘a’
my_dict[‘c’] = 4 Add a new key ‘c’
“`
After these operations, `my_dict` will be `{‘a’: 3, ‘b’: 2, ‘c’: 4}`.
Using the update() Method
The `update()` method is another effective way to update a dictionary. This method accepts another dictionary or an iterable of key-value pairs and merges it with the original dictionary. If a key already exists, its value will be updated; if it does not exist, the key-value pair will be added.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.update({‘b’: 3, ‘c’: 4}) Updates ‘b’ and adds ‘c’
“`
After executing this code, `my_dict` will be `{‘a’: 1, ‘b’: 3, ‘c’: 4}`.
Updating Multiple Keys at Once
You can also update multiple keys using dictionary unpacking, which was introduced in Python 3.5. This technique allows you to create a new dictionary with updated values by merging existing dictionaries.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
new_values = {‘a’: 3, ‘c’: 4}
updated_dict = {my_dict, new_values}
“`
In this case, `updated_dict` will contain `{‘a’: 3, ‘b’: 2, ‘c’: 4}`.
Updating with Conditional Logic
Sometimes, updates may need to be conditional based on certain criteria. You can achieve this by iterating through the dictionary and applying your conditions.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key in my_dict:
if my_dict[key] < 3:
my_dict[key] += 1 Increment values less than 3
```
After running this code, `my_dict` will be `{'a': 2, 'b': 3, 'c': 3}`.
Table of Update Methods
Method | Description | Example |
---|---|---|
Assignment | Directly assigning a new value to a key. | my_dict[‘a’] = 3 |
update() | Merges another dictionary or iterable. | my_dict.update({‘b’: 3}) |
Dictionary Unpacking | Merges multiple dictionaries using unpacking. | updated_dict = {**my_dict, **new_values} |
Conditional Logic | Updates based on specific conditions. | if my_dict[key] < 3: my_dict[key] += 1 |
Updating a Dictionary in Python
Updating a dictionary in Python can be achieved through various methods, each suited to different use cases. Here are the most common techniques:
Using Assignment
You can update the value of an existing key directly by assigning a new value to it. If the key does not exist, this method will create a new key-value pair.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘a’] = 3 Updates the value for key ‘a’
my_dict[‘c’] = 4 Adds a new key ‘c’ with value 4
“`
Using the update() Method
The `update()` method allows you to update multiple key-value pairs at once. You can pass another dictionary or an iterable of key-value pairs.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.update({‘b’: 3, ‘c’: 4}) Updates ‘b’ and adds ‘c’
“`
Method | Description |
---|---|
Assignment | Directly set a key to a new value. |
`update()` | Update multiple keys from another dict. |
Using setdefault() Method
The `setdefault()` method is useful when you want to update a key only if it does not already exist. If the key exists, it will return its value; if not, it will set it to the specified default value.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.setdefault(‘b’, 3) ‘b’ remains 2
my_dict.setdefault(‘c’, 4) ‘c’ is added with value 4
“`
Using Dictionary Comprehension
You can also use dictionary comprehension to create a new dictionary based on an existing one, effectively updating values conditionally.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
updated_dict = {k: (v + 1) if k == ‘a’ else v for k, v in my_dict.items()}
“`
Using the Pipe Operator (Python 3.9 and later)
In Python 3.9 and later, you can use the pipe operator (`|`) to merge dictionaries, which can also be seen as an update.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
new_dict = {‘b’: 3, ‘c’: 4}
merged_dict = my_dict | new_dict Results in {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Handling Non-Existent Keys
When updating dictionaries, it’s important to consider how to handle non-existent keys. The following methods are particularly useful:
- `get()` method: Retrieve a value with a fallback if the key does not exist.
“`python
value = my_dict.get(‘d’, 0) Returns 0 if ‘d’ is not found
“`
- Using `in` keyword: Check for key existence before updating.
“`python
if ‘d’ in my_dict:
my_dict[‘d’] += 1
else:
my_dict[‘d’] = 1
“`
These techniques provide flexibility in managing and updating dictionaries, catering to various programming needs and preferences.
Expert Insights on Updating Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). Updating a dictionary in Python can be achieved through various methods, including the use of the `update()` method, which allows merging another dictionary or adding key-value pairs. This method is efficient and straightforward, making it a preferred choice among developers.
Michael Chen (Data Scientist, Tech Innovations Inc.). One of the most versatile ways to update a dictionary is by using dictionary unpacking with the `**` operator. This method not only allows for updating existing keys but also facilitates the addition of new keys in a clean and readable manner, which is essential for maintaining code clarity.
Sarah Patel (Python Instructor, Code Academy). It is crucial to understand that dictionaries in Python are mutable. This means that you can update them in place without creating a new dictionary. Techniques like direct assignment and the `setdefault()` method can be particularly useful for managing default values while updating.
Frequently Asked Questions (FAQs)
How can I update a value in a Python dictionary?
You can update a value in a dictionary by using the key associated with that value. For example, `my_dict[‘key’] = new_value` will change the value of ‘key’ to `new_value`.
What method can I use to add multiple key-value pairs to a dictionary?
You can use the `update()` method to add multiple key-value pairs. For instance, `my_dict.update({‘key1’: value1, ‘key2’: value2})` will add or update the specified keys in the dictionary.
Is it possible to update a dictionary using another dictionary?
Yes, you can update a dictionary with another dictionary using the `update()` method. For example, `my_dict.update(another_dict)` will merge `another_dict` into `my_dict`, updating existing keys and adding new ones.
How do I remove a key-value pair while updating a dictionary?
To remove a key-value pair while updating, you can use the `del` statement before or after updating. For example, `del my_dict[‘key’]` will remove the specified key from the dictionary.
Can I use dictionary comprehension to update a dictionary?
Yes, dictionary comprehension can be utilized to create a new dictionary based on existing keys and values. For example, `my_dict = {k: v * 2 for k, v in my_dict.items()}` will update all values by multiplying them by 2.
What happens if I update a dictionary with a key that does not exist?
If you update a dictionary with a key that does not exist, Python will add that key-value pair to the dictionary. For example, `my_dict[‘new_key’] = new_value` will create a new entry in `my_dict`.
Updating a dictionary in Python is a straightforward process that can be accomplished using several methods. The most common approaches include using the `update()` method, the assignment operator, and dictionary comprehensions. Each of these methods allows for the addition of new key-value pairs or the modification of existing ones, making it easy to manage and manipulate dictionary data structures effectively.
The `update()` method is particularly useful when you want to merge another dictionary or an iterable of key-value pairs into an existing dictionary. This method modifies the original dictionary in place and can handle both new keys and updates to existing keys seamlessly. Alternatively, using the assignment operator allows for direct updates to specific keys, providing a clear and concise way to change values. Dictionary comprehensions offer a powerful and flexible approach to create new dictionaries based on existing ones, which can also include updates to values as needed.
In summary, Python provides multiple efficient ways to update dictionaries, catering to various programming needs. Understanding these methods enhances your ability to work with dictionaries, which are fundamental data structures in Python. By leveraging these techniques, developers can ensure their code remains clean, efficient, and easy to maintain.
Author Profile
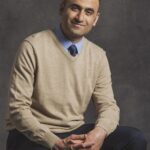
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?