How Can You Effectively Use APIs with Python?
In today’s digital landscape, APIs (Application Programming Interfaces) have become the backbone of modern software development, enabling seamless communication between different applications and services. Whether you’re building a web application, automating tasks, or integrating third-party services, knowing how to use APIs effectively with Python can open up a world of possibilities. With its simplicity and versatility, Python has emerged as a favorite among developers for interacting with APIs, making it an essential skill for anyone looking to harness the power of data and services available online.
Understanding how to use APIs with Python involves grasping the fundamental concepts of HTTP requests, responses, and the various data formats commonly used, such as JSON and XML. Python’s rich ecosystem of libraries, such as `requests`, simplifies the process of making API calls, allowing developers to focus on building functionality rather than getting bogged down in complex syntax. Whether you’re fetching data from a public API, sending data to a server, or even creating your own API, Python provides the tools and frameworks necessary to streamline these interactions.
As we delve deeper into this topic, you’ll discover practical techniques for authenticating with APIs, handling errors, and parsing responses to extract meaningful information. With a solid understanding of these principles, you’ll be well-equipped to leverage the vast array of APIs available, transforming your
Understanding API Requests
When working with APIs in Python, it is essential to comprehend the structure and types of requests you can make. The most common types of HTTP requests are GET, POST, PUT, DELETE, and PATCH. Each serves a specific purpose:
- GET: Retrieve data from a server.
- POST: Send data to a server, often resulting in the creation of a new resource.
- PUT: Update an existing resource with new data.
- DELETE: Remove a resource from the server.
- PATCH: Apply partial modifications to a resource.
These request types form the foundation for interacting with RESTful APIs, allowing you to perform CRUD (Create, Read, Update, Delete) operations.
Using the Requests Library
The Requests library is a popular choice for making HTTP requests in Python due to its simplicity and ease of use. To get started, you first need to install the library if you haven’t already:
bash
pip install requests
After installation, you can make requests using the following basic structure:
python
import requests
response = requests.get(‘https://api.example.com/data’)
print(response.json())
The `response` object contains various attributes that provide information about the request, such as:
- `status_code`: The HTTP status code returned by the server.
- `headers`: The headers returned in the response.
- `json()`: A method to convert the response to JSON format.
Making Different Types of Requests
Here are examples of how to make different types of requests using the Requests library:
python
# GET request
response = requests.get(‘https://api.example.com/data’)
# POST request
data = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=data)
# PUT request
update_data = {‘key’: ‘new_value’}
response = requests.put(‘https://api.example.com/data/1’, json=update_data)
# DELETE request
response = requests.delete(‘https://api.example.com/data/1’)
Each of these requests can include headers and parameters as needed.
Handling Responses
Once you receive a response from an API, you need to handle it appropriately. Here’s how to check the status code and parse the JSON response:
python
if response.status_code == 200:
data = response.json()
# Process the data
else:
print(f”Error: {response.status_code}”)
To better visualize how response data can be structured, consider the following example:
Key | Type | Description |
---|---|---|
id | Integer | Unique identifier for the resource |
name | String | Name of the resource |
created_at | String (ISO 8601) | Timestamp when the resource was created |
This table illustrates a common structure for JSON responses, giving insight into the types of data you can expect when interacting with an API.
Authentication and Headers
Many APIs require authentication to ensure secure access. Common methods include API keys, OAuth tokens, and Basic Auth. Here’s how to include headers for authentication:
python
headers = {
‘Authorization’: ‘Bearer YOUR_API_TOKEN’,
‘Content-Type’: ‘application/json’
}
response = requests.get(‘https://api.example.com/protected-data’, headers=headers)
Ensure that you check the API documentation for the required authentication method and the specific headers needed for your requests.
Understanding APIs
APIs, or Application Programming Interfaces, serve as intermediaries that allow different software applications to communicate with each other. They define the methods and data formats that applications can use to request and exchange information. When working with APIs in Python, understanding the following components is essential:
- Endpoints: URLs through which an API can be accessed.
- Methods: Standard HTTP methods such as GET, POST, PUT, DELETE that specify the action to be performed.
- Headers: Metadata sent with requests, often containing authentication tokens or content types.
- Payload: Data sent with requests, particularly relevant for POST and PUT methods.
Setting Up Your Environment
Before you begin using an API with Python, ensure you have the necessary tools installed. The `requests` library is a popular choice for making HTTP requests. To install it, run the following command:
bash
pip install requests
Making API Requests
To interact with an API, you can use various methods provided by the `requests` library. Here’s a brief overview of how to make basic requests:
- GET Request: Used to retrieve data from an API.
python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json()
- POST Request: Used to send data to an API.
python
import requests
payload = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=payload)
data = response.json()
- Handling Responses: Check the response status and handle data appropriately.
python
if response.status_code == 200:
print(“Success:”, data)
else:
print(“Error:”, response.status_code)
Authentication Methods
Many APIs require authentication to secure access. Common methods include:
Authentication Type | Description | Example |
---|---|---|
API Key | A unique key provided by the API | `headers = {‘Authorization’: ‘Bearer YOUR_API_KEY’}` |
OAuth | Token-based authentication | Use a library such as `requests-oauthlib` |
Basic Auth | Username and password | `response = requests.get(url, auth=(‘user’, ‘pass’))` |
Parsing API Responses
API responses are often in JSON format. Use the `json()` method to convert the response into a Python dictionary:
python
data = response.json()
When parsing, you can access specific elements using standard dictionary methods:
python
value = data[‘key’]
For more complex data structures, leverage loops and conditions to extract the necessary information:
python
for item in data[‘items’]:
print(item[‘name’])
Error Handling
When working with APIs, it’s crucial to implement error handling to manage unexpected responses or connection issues. Use try-except blocks to catch exceptions:
python
try:
response = requests.get(‘https://api.example.com/data’)
response.raise_for_status() # Raises an error for bad responses
except requests.exceptions.HTTPError as err:
print(f”HTTP error occurred: {err}”)
except Exception as e:
print(f”An error occurred: {e}”)
Best Practices
Adhering to best practices when using APIs can enhance your application’s robustness:
- Rate Limiting: Be aware of the API’s rate limits and implement throttling as needed.
- Timeouts: Set timeouts for requests to avoid hanging connections.
- Logging: Maintain logs of requests and responses for debugging purposes.
- Documentation: Familiarize yourself with the API’s documentation for endpoints, parameters, and response structures.
Expert Insights on Using APIs with Python
Dr. Emily Carter (Lead Software Engineer, Tech Innovations Inc.). “To effectively use APIs with Python, one must first understand the fundamentals of HTTP requests. Utilizing libraries such as `requests` simplifies the process of sending GET and POST requests, which are essential for interacting with most APIs.”
Michael Chen (Senior Data Scientist, Data Solutions Group). “Incorporating APIs into Python projects can significantly enhance data retrieval capabilities. I recommend using JSON for data interchange, as it is lightweight and easily parsed in Python using the built-in `json` module.”
Sarah Thompson (API Integration Specialist, CloudTech Services). “When working with APIs in Python, error handling is crucial. Implementing try-except blocks can help manage exceptions that arise from failed API calls, ensuring that the application remains robust and user-friendly.”
Frequently Asked Questions (FAQs)
What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. It defines the methods and data formats that applications can use for requests and responses.
How do I install the necessary libraries to use an API with Python?
To use an API with Python, you typically need the `requests` library. You can install it using pip with the command: `pip install requests`.
How do I make a GET request to an API using Python?
You can make a GET request using the `requests` library as follows:
python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json()
This retrieves data from the specified API endpoint.
How do I handle API authentication in Python?
API authentication can be handled by including an API key or token in the request headers. For example:
python
headers = {‘Authorization’: ‘Bearer your_api_token’}
response = requests.get(‘https://api.example.com/data’, headers=headers)
What should I do if I receive an error response from the API?
If you receive an error response, check the status code and the error message returned by the API. Common status codes include 400 for bad requests and 401 for unauthorized access. Review the API documentation for troubleshooting steps.
How can I parse JSON data returned from an API in Python?
You can parse JSON data using the `json()` method of the response object. For example:
python
data = response.json()
This converts the JSON response into a Python dictionary, allowing you to access the data easily.
In summary, utilizing APIs with Python is a fundamental skill for developers looking to integrate external services and data into their applications. The process typically involves making HTTP requests to the API endpoint, handling the response, and parsing the returned data. Python’s libraries, such as `requests`, simplify this process, allowing for efficient interaction with APIs. Understanding the structure of the API, including authentication methods and data formats, is crucial for successful implementation.
Moreover, error handling and response validation are essential components of working with APIs. Developers should anticipate potential issues, such as network errors or invalid responses, and implement appropriate error handling mechanisms to ensure robustness. Additionally, utilizing tools like Postman for testing API requests can enhance the development process by allowing developers to experiment with API calls before integrating them into their Python code.
Key takeaways include the importance of thoroughly reading API documentation to understand the available endpoints, required parameters, and response formats. Furthermore, leveraging Python’s capabilities for data manipulation, such as using libraries like `json` for parsing JSON responses, can significantly enhance the efficiency of API interactions. Overall, mastering API usage in Python opens up a wide range of possibilities for building dynamic applications that can leverage external data and services.
Author Profile
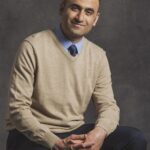
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?