How Can You Effectively Use APIs in Python?
In today’s digital landscape, APIs (Application Programming Interfaces) have become the backbone of modern software development, enabling seamless communication between different applications and services. For Python developers, harnessing the power of APIs opens up a world of possibilities, from integrating third-party services to accessing vast datasets. Whether you’re building a web application, automating tasks, or conducting data analysis, understanding how to effectively use APIs in Python can significantly enhance your projects and streamline your workflow.
Using APIs in Python is not just about sending requests and receiving responses; it’s about leveraging the capabilities of external services to enrich your applications. With libraries like `requests`, Python makes it easy to interact with APIs, allowing developers to focus on building features rather than getting bogged down in the complexities of network communication. The process typically involves understanding the API’s documentation, crafting the right requests, and handling the data returned in a format that your application can utilize.
As you delve deeper into the world of APIs, you’ll discover various authentication methods, data formats like JSON, and best practices for error handling. This knowledge will empower you to create robust applications that can interact with a plethora of services, from social media platforms to cloud storage solutions. Join us as we explore the essential techniques and tools that will elevate your Python programming skills and unlock the
Understanding API Requests
When working with APIs in Python, the primary interaction revolves around making requests and handling responses. An API request typically consists of several components, including the endpoint, headers, and parameters.
- Endpoint: The URL where the API can be accessed.
- Headers: Information sent with the request, often including authentication tokens.
- Parameters: Data sent to the API to specify the request, such as query strings or JSON payloads.
To make these requests, the popular `requests` library is often used due to its simplicity and effectiveness. Here is an example of how to perform a GET request:
python
import requests
response = requests.get(‘https://api.example.com/data’, headers={‘Authorization’: ‘Bearer YOUR_TOKEN’})
data = response.json()
print(data)
In this example, the `requests.get` method retrieves data from the specified endpoint, and the response is parsed as JSON.
Handling API Responses
API responses typically return data in formats such as JSON or XML. It is crucial to check the response status to handle errors appropriately. Common HTTP status codes include:
Status Code | Description |
---|---|
200 | OK |
400 | Bad Request |
401 | Unauthorized |
404 | Not Found |
500 | Internal Server Error |
By examining the status code, you can determine the next steps. For example:
python
if response.status_code == 200:
print(“Success:”, data)
elif response.status_code == 404:
print(“Error: Data not found.”)
else:
print(“Error:”, response.status_code)
This code snippet provides a basic structure for handling various API response scenarios.
Making Different Types of Requests
APIs often require different types of requests based on the operation being performed. The most common are GET, POST, PUT, and DELETE.
- GET: Retrieve data from the server.
- POST: Send new data to the server.
- PUT: Update existing data on the server.
- DELETE: Remove data from the server.
Here’s how to implement a POST request:
python
data = {‘name’: ‘John’, ‘age’: 30}
response = requests.post(‘https://api.example.com/users’, json=data)
In this example, a new user is created by sending JSON data to the server.
Authentication with APIs
Many APIs require authentication to ensure that the requests are made by authorized users. The most common methods of authentication include:
- API Keys: A simple token provided by the API provider.
- OAuth: A more complex authentication method often used for user-specific data.
When using API keys, you typically include them in the request headers:
python
headers = {‘Authorization’: ‘Bearer YOUR_API_KEY’}
response = requests.get(‘https://api.example.com/secure-data’, headers=headers)
For OAuth, the process involves obtaining an access token through a series of redirects and then using that token in subsequent requests.
Rate Limiting and Best Practices
When using APIs, be aware of rate limits that restrict the number of requests you can make in a given time frame. Exceeding these limits can result in temporary bans or throttled access.
- Best Practices:
- Check the API documentation for rate limits.
- Implement exponential backoff in your error handling to manage retries gracefully.
- Cache responses when appropriate to reduce the number of requests.
By adhering to these practices, you can ensure a more efficient and effective interaction with APIs in Python.
Understanding APIs
APIs, or Application Programming Interfaces, serve as intermediaries that allow different software applications to communicate. They enable developers to leverage existing services and functionalities without having to build them from scratch. In Python, utilizing APIs typically involves making HTTP requests to access data or services provided by the API.
Making HTTP Requests
Python provides several libraries to interact with APIs, with the most popular being `requests`. This library simplifies sending HTTP requests and handling responses.
To install the `requests` library, use the following command:
pip install requests
Here’s how to make a basic GET request:
python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json() # Convert response to JSON format
print(data)
Handling API Responses
When you make an API request, you typically receive a response in JSON format. It’s essential to check the status code to ensure the request was successful.
Status codes to be aware of include:
- 200: OK – The request has succeeded.
- 400: Bad Request – The server could not understand the request.
- 401: Unauthorized – Authentication is required.
- 404: Not Found – The requested resource could not be found.
- 500: Internal Server Error – The server encountered an error.
Example of handling the response:
python
if response.status_code == 200:
data = response.json()
else:
print(f”Error: {response.status_code}”)
Making POST Requests
In addition to GET requests, APIs often require POST requests to send data. For example, to create a new resource, you can use the following structure:
python
payload = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
response = requests.post(‘https://api.example.com/resource’, json=payload)
if response.status_code == 201:
print(“Resource created successfully.”)
Working with API Authentication
Many APIs require authentication, which can include API keys, OAuth tokens, or other methods. Below is an example of using an API key in the header:
python
headers = {‘Authorization’: ‘Bearer YOUR_API_KEY’}
response = requests.get(‘https://api.example.com/protected-data’, headers=headers)
Rate Limiting and Error Handling
APIs often have rate limits to prevent abuse. It’s crucial to handle errors gracefully and implement retries with exponential backoff when necessary.
Consider the following error handling approach:
python
import time
def make_request_with_retries(url, headers, retries=3):
for i in range(retries):
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json()
elif response.status_code in [429, 500]: # Rate limit or server error
time.sleep(2 ** i) # Exponential backoff
else:
print(f”Error: {response.status_code}”)
return None
Parsing JSON Data
Once you receive a JSON response, you can parse it to extract the desired information. Python’s built-in `json` library makes this straightforward.
Example of parsing JSON:
python
data = response.json()
for item in data[‘items’]:
print(item[‘name’], item[‘value’])
Best Practices
When working with APIs in Python, consider the following best practices:
- Read the API documentation thoroughly to understand the endpoints, parameters, and expected responses.
- Use environment variables to store sensitive information like API keys.
- Implement logging to track requests and responses for debugging.
- Test your code with various inputs and edge cases to ensure robustness.
Expert Insights on Using APIs in Python
Dr. Emily Chen (Lead Software Engineer, Tech Innovations Inc.). “When utilizing APIs in Python, it is crucial to understand the structure of the API documentation. This will guide you in making effective requests and handling responses properly, ensuring that your application communicates seamlessly with external services.”
Mark Thompson (Senior Data Scientist, Data Insights Group). “Leveraging libraries such as Requests and JSON in Python simplifies the process of interacting with APIs. These tools not only streamline the code but also enhance readability and maintainability, which is essential for long-term projects.”
Sarah Patel (API Integration Specialist, Cloud Solutions Ltd.). “Error handling is a vital aspect of working with APIs in Python. Implementing robust error-checking mechanisms allows developers to gracefully manage unexpected issues, ensuring that applications remain stable and user-friendly.”
Frequently Asked Questions (FAQs)
What are APIs and why are they important in Python?
APIs, or Application Programming Interfaces, allow different software applications to communicate with each other. In Python, APIs are crucial for integrating external services, accessing data, and enhancing functionality without having to build everything from scratch.
How can I make a simple API request in Python?
To make a simple API request in Python, you can use the `requests` library. First, install it using `pip install requests`. Then, use `response = requests.get(‘API_URL’)` to send a GET request, and access the response data with `response.json()` if the response is in JSON format.
What is the difference between GET and POST requests?
GET requests retrieve data from a specified resource, while POST requests send data to a server to create or update a resource. GET requests are idempotent and can be cached, whereas POST requests are not idempotent and typically involve data submission.
How do I handle API authentication in Python?
API authentication can be handled using headers, tokens, or OAuth. For simple token-based authentication, include the token in your request headers like this: `headers = {‘Authorization’: ‘Bearer YOUR_TOKEN’}` and pass it to your request.
What should I do if I encounter errors while using an API?
If you encounter errors, first check the API documentation for error codes and their meanings. Use error handling in your Python code with try-except blocks to manage exceptions and log errors for further analysis.
How can I parse JSON data returned from an API in Python?
To parse JSON data, use the `json()` method from the response object. For example, `data = response.json()` converts the JSON response into a Python dictionary, allowing you to access the data using standard dictionary methods.
Using APIs in Python is a powerful way to enhance the functionality of applications by integrating external services and data sources. The process typically involves sending HTTP requests to an API endpoint, which can be accomplished using libraries such as `requests`. These libraries simplify the process of making requests, handling responses, and managing authentication, allowing developers to focus on the core logic of their applications.
Understanding the structure of API requests and responses is crucial. Most APIs follow RESTful principles, utilizing standard HTTP methods such as GET, POST, PUT, and DELETE. Additionally, APIs often return data in JSON format, which can be easily parsed in Python. Familiarity with these concepts enables developers to effectively interact with various APIs, whether for retrieving data, submitting forms, or integrating third-party services.
Moreover, error handling and rate limiting are essential considerations when working with APIs. Implementing robust error handling ensures that applications can gracefully manage issues such as network failures or unexpected API responses. Additionally, being aware of rate limits imposed by APIs helps prevent service disruptions and ensures compliance with usage policies.
In summary, leveraging APIs in Python empowers developers to build more dynamic and feature-rich applications. By mastering the tools and techniques for making API calls, processing responses, and
Author Profile
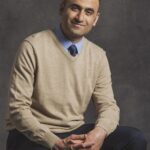
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?