How Can You Effectively Use Modulus in Python?
In the world of programming, understanding how to manipulate numbers is a fundamental skill that can unlock a myriad of possibilities. Among the various operations available, the modulus operator stands out as a powerful tool for performing division with a twist. Whether you’re a seasoned coder or a curious beginner, mastering the modulus operation in Python can enhance your ability to solve problems efficiently and creatively. This article will guide you through the ins and outs of using the modulus operator, showcasing its practical applications and offering insights into its behavior in different scenarios.
The modulus operator, represented by the percentage sign (%) in Python, is used to find the remainder of a division operation. This seemingly simple function can yield significant results, particularly in tasks involving even and odd number determination, cyclic patterns, and more. As you delve deeper, you’ll discover how this operator can be seamlessly integrated into your code, enabling you to tackle various mathematical challenges with ease.
Moreover, the versatility of the modulus operator extends beyond basic arithmetic. It plays a crucial role in algorithms, data structures, and even game development. By understanding how to effectively utilize the modulus operator in Python, you can elevate your programming skills and broaden your problem-solving toolkit. Get ready to explore the fascinating world of modulus operations and unlock new dimensions in your coding journey!
Using the Modulus Operator
The modulus operator in Python is represented by the percent sign `%`. It is used to determine the remainder of a division operation between two numbers. This operator is particularly useful in various programming scenarios, such as checking for even or odd numbers, cycling through a list, or performing arithmetic operations on large numbers without losing precision.
To use the modulus operator, the syntax is straightforward:
“`python
result = a % b
“`
Here, `a` is the dividend, and `b` is the divisor. The result will be the remainder of the division of `a` by `b`.
Examples of Modulus in Python
To illustrate the use of the modulus operator, consider the following examples:
“`python
Example 1: Basic modulus operation
result1 = 10 % 3 result1 will be 1
Example 2: Even or odd check
number = 15
if number % 2 == 0:
print(f”{number} is even.”)
else:
print(f”{number} is odd.”) This will print: 15 is odd.
Example 3: Cycling through a list
my_list = [‘a’, ‘b’, ‘c’, ‘d’]
index = 5
print(my_list[index % len(my_list)]) This will print: ‘b’
“`
In the examples above, the first demonstrates a simple modulus operation that yields a remainder. The second example shows how to determine if a number is even or odd using the modulus operator. The third example illustrates how to cycle through a list by using the modulus operator to wrap around when the index exceeds the list length.
Common Use Cases for Modulus
The modulus operator has several practical applications in programming, including:
- Determining even or odd numbers: If a number modulo 2 results in 0, it is even; otherwise, it is odd.
- Implementing cyclic behavior: Useful in scenarios like round-robin scheduling, where tasks need to be assigned in a circular manner.
- Limiting a value within a range: It can help keep values within specified bounds, such as keeping an index within the length of an array.
Modulus with Negative Numbers
Python handles the modulus operation with negative numbers differently than some other programming languages. The result of the modulus operation will always have the same sign as the divisor. Here’s a table that illustrates this behavior:
Dividend (a) | Divisor (b) | Result (a % b) |
---|---|---|
10 | 3 | 1 |
10 | -3 | -2 |
-10 | 3 | 1 |
-10 | -3 | -1 |
This behavior is crucial for developers to understand, as it can affect the logic of programs where negative numbers are involved.
Understanding the Modulus Operator
In Python, the modulus operator is represented by the percentage sign `%`. It is used to find the remainder of the division between two numbers. The syntax is straightforward:
“`python
remainder = a % b
“`
Here, `a` is the dividend and `b` is the divisor. The result, `remainder`, will be the leftover after dividing `a` by `b`.
Basic Usage of Modulus
The modulus operator can be applied to both integers and floats. Here are some examples to illustrate its use:
“`python
Integer modulus
result1 = 10 % 3 result1 will be 1
Float modulus
result2 = 10.5 % 3.2 result2 will be 1.9
“`
When using integers, the output is the integer remainder. When applied to floats, it returns the float remainder.
Common Applications of Modulus
The modulus operator is particularly useful in several scenarios:
- Determining Even or Odd Numbers:
- An even number can be identified if `number % 2 == 0`.
- An odd number can be identified if `number % 2 != 0`.
- Cycling Through a Range:
- Useful in scenarios where you need to loop through a set of values, such as an index in a circular list.
- Constraints in Algorithms:
- Frequently used in algorithms that require constraints based on periodicity, such as in hashing functions.
Examples of Modulus in Python
Here are practical examples to demonstrate the versatility of the modulus operator:
“`python
Check if a number is even or odd
number = 42
if number % 2 == 0:
print(f”{number} is even.”)
else:
print(f”{number} is odd.”)
Cycling through indices
elements = [‘a’, ‘b’, ‘c’, ‘d’]
for i in range(10):
print(elements[i % len(elements)]) This will cycle through the elements
“`
Modulus with Negative Numbers
When dealing with negative numbers, the behavior of the modulus operator can be different from other programming languages. In Python, the modulus result will always have the same sign as the divisor:
“`python
print(-10 % 3) Output will be 2
print(10 % -3) Output will be -2
print(-10 % -3) Output will be -1
“`
This property is essential to consider in calculations involving negative values.
Using Modulus in Conditional Statements
The modulus operator is often combined with conditional statements to execute certain code based on specific conditions:
“`python
for num in range(1, 21):
if num % 5 == 0:
print(f”{num} is divisible by 5.”)
“`
This snippet checks numbers from 1 to 20 and prints those that are divisible by 5.
Advanced Modulus Applications
Modulus can also be utilized in more complex mathematical operations and algorithms, such as:
Operation | Example |
---|---|
Finding periodicity | `n % period == 0` |
Hashing algorithms | `hash_value = key % table_size` |
Random number generation | `random_number = random.randint(0, max_value – 1) % some_divisor` |
Utilizing the modulus operator effectively can significantly enhance the functionality and performance of your Python programs, especially in algorithm design and data manipulation.
Mastering the Modulus Operator in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The modulus operator in Python, represented by the percent sign (%), is essential for determining the remainder of a division operation. This operator is particularly useful in scenarios such as checking for even or odd numbers, where you can use `number % 2` to ascertain the parity of a number.
Michael Thompson (Python Programming Instructor, Code Academy). Understanding how to effectively use the modulus operator can significantly enhance your programming skills. It allows for the implementation of algorithms that require periodic checks or conditions, such as looping through a list and performing actions on every nth element, which can be elegantly achieved using `if index % n == 0`.
Lisa Nguyen (Data Scientist, Analytics Solutions). In data analysis, the modulus operator is invaluable for grouping data. For instance, when working with time series data, you can use the modulus operator to categorize timestamps into specific intervals, making it easier to analyze trends or patterns over time by using expressions like `timestamp % interval`.
Frequently Asked Questions (FAQs)
What is the modulus operator in Python?
The modulus operator in Python is represented by the percentage symbol `%`, and it returns the remainder of a division operation between two numbers.
How do you use the modulus operator in a Python expression?
You can use the modulus operator by placing it between two numbers, such as `result = a % b`, where `a` is divided by `b`, and `result` stores the remainder.
Can you use the modulus operator with negative numbers in Python?
Yes, the modulus operator can be used with negative numbers. The result will always have the same sign as the divisor, which can lead to results that differ from other programming languages.
What is the output of `10 % 3` in Python?
The output of `10 % 3` is `1`, as 10 divided by 3 equals 3 with a remainder of 1.
How can the modulus operator be useful in programming?
The modulus operator is useful for determining whether a number is even or odd, implementing cyclic behavior, and handling tasks like pagination in applications.
Are there any common mistakes when using the modulus operator in Python?
Common mistakes include misunderstanding the sign of the result with negative numbers and assuming the operator behaves the same way across different programming languages.
The modulus operator in Python, represented by the percentage sign (%), is a powerful tool used to obtain the remainder of a division operation. This operator can be applied to integers and floating-point numbers, making it versatile for various programming tasks. When using the modulus operator, the syntax is straightforward: `a % b`, where `a` is the dividend and `b` is the divisor. The result will be the remainder after dividing `a` by `b`. This functionality is particularly useful in scenarios such as determining even or odd numbers, handling cyclic operations, and implementing algorithms that require periodicity.
One of the key takeaways when using the modulus operator is its application in control structures. For example, it can be effectively utilized in loops to execute certain actions at regular intervals. Additionally, the modulus operator is instrumental in tasks involving number theory, such as finding factors or checking divisibility. Understanding the behavior of the modulus operator with negative numbers is also crucial, as it can yield results that may differ from other programming languages. In Python, the result of `a % b` always has the same sign as the divisor `b`, which is an important aspect to keep in mind.
mastering the use of the modulus operator
Author Profile
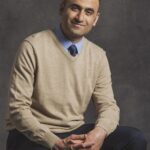
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?