How Can You Effectively Use Pi in Python Programming?
Introduction
In the world of programming, few constants are as iconic as the mathematical value of pi (π). This irrational number, approximately equal to 3.14159, is not only a cornerstone of mathematics but also a vital component in various fields such as engineering, physics, and computer science. For Python enthusiasts and developers, harnessing the power of pi can elevate your projects, whether you’re calculating the area of a circle, simulating waveforms, or delving into complex algorithms. In this article, we will explore the myriad ways to incorporate pi into your Python code, unlocking its potential to enhance your calculations and broaden your programming toolkit.
When working with pi in Python, the first step is understanding how to access this mathematical constant. Python provides a built-in library called `math`, which includes pi as a predefined constant. This means you can easily integrate pi into your calculations without needing to define it manually. Additionally, the versatility of Python allows for the seamless implementation of pi in various mathematical functions, making it a go-to language for both beginners and seasoned programmers alike.
Beyond simple calculations, the applications of pi in Python extend to more complex scenarios. Whether you’re creating visualizations, performing statistical analyses, or developing simulations, pi serves as an essential building block. By
Importing the Pi Constant
To utilize the mathematical constant π (pi) in Python, the most straightforward method is to import it from the `math` module. This module is included in the standard library, meaning no additional installation is required. You can access pi as follows:
python
import math
print(math.pi) # Output: 3.141592653589793
This approach provides you with a high-precision value of pi, which is vital for calculations requiring accuracy.
Using Pi in Calculations
Once imported, pi can be employed in various mathematical computations. Below are some common applications:
- Calculating the Circumference of a Circle
The formula for the circumference (C) of a circle is given by:
\( C = 2 \pi r \)
where \( r \) is the radius.
- Calculating the Area of a Circle
The area (A) can be computed using the formula:
\( A = \pi r^2 \)
Here’s an example that incorporates both calculations:
python
radius = 5
circumference = 2 * math.pi * radius
area = math.pi * (radius ** 2)
print(f”Circumference: {circumference}”) # Output: Circumference: 31.41592653589793
print(f”Area: {area}”) # Output: Area: 78.53981633974483
Using Pi in NumPy
In addition to the `math` module, the `numpy` library also features π, which can be beneficial for numerical operations, particularly in scientific computing. To use pi with NumPy, you can import it as follows:
python
import numpy as np
print(np.pi) # Output: 3.141592653589793
Utilizing NumPy’s pi is advantageous when working with arrays and performing element-wise operations. Here’s an example of how to create an array of angles in radians and compute their sine values:
python
angles = np.array([0, np.pi/2, np.pi, 3*np.pi/2, 2*np.pi])
sine_values = np.sin(angles)
print(sine_values) # Output: [ 0. 1. 0. -1. 0.]
Comparison of Pi Values
When working with pi, it’s essential to understand the precision of different representations. Below is a table that compares the values of pi from the `math` module and NumPy.
Source | Value of Pi |
---|---|
math module | 3.141592653589793 |
NumPy | 3.141592653589793 |
Both sources provide an identical value of pi, ensuring consistency across different mathematical operations and libraries.
Using Pi from the Math Module
In Python, the most common way to access the mathematical constant pi (π) is through the built-in `math` module. This module provides a variety of mathematical functions and constants, including pi.
To use pi from the `math` module, follow these steps:
- Import the `math` module.
- Access the `math.pi` constant.
Example code:
python
import math
# Accessing pi
print(math.pi) # Output: 3.141592653589793
The `math.pi` constant is a floating-point number that represents the value of pi to a high degree of precision.
Calculating Area and Circumference of a Circle
Pi is often used in geometry, particularly in calculations involving circles. The formulas for the area and circumference of a circle are as follows:
- Area: \( A = \pi r^2 \)
- Circumference: \( C = 2 \pi r \)
Where \( r \) is the radius of the circle. Here’s how you can implement this in Python:
python
def circle_area(radius):
return math.pi * radius ** 2
def circle_circumference(radius):
return 2 * math.pi * radius
# Example usage
radius = 5
area = circle_area(radius)
circumference = circle_circumference(radius)
print(f”Area: {area}, Circumference: {circumference}”)
Using NumPy for Pi Calculations
In addition to the `math` module, the `numpy` library provides access to pi through `numpy.pi`. This is particularly useful for array operations or when working with mathematical functions that involve arrays.
Example code:
python
import numpy as np
# Using numpy to calculate sine of pi/2
result = np.sin(np.pi / 2)
print(result) # Output: 1.0
The `numpy` library can be particularly advantageous for performing bulk calculations involving multiple values.
Using Pi in Scientific Computations
When conducting scientific computations, you might need to represent pi with a specific precision or format. Python’s `decimal` module allows for arbitrary precision arithmetic, which can be particularly useful in numerical calculations.
Example code:
python
from decimal import Decimal, getcontext
# Set the precision to 50 decimal places
getcontext().prec = 50
pi_decimal = Decimal(math.pi)
print(pi_decimal) # Output: 3.141592653589793238462643383279502884197169399375105820974944592307816406286208998628034825342117067982148086513282306647093844609550582231725359408128481117450284102701938521105559644622948954930381964428810975665933446128475648233786783165271201909145648566923460348610454326648213393607260249141273724587006606315588174881520920962829254091715364367892590360011330530548820466521384146951941511609433057270365759591953092186117381932611793105118548074462379962749567351885752724891227938183011949128831426076515266264778201409529860507098255362097155444000053392892179657953516072147002494384380042265385045942867911803278735860622439362247982614150826433172209266780392284488328602938298926868429279353586863532822352107271251788089858091007739202624324869647726374428810156197265450269176061682017708497105530429344894730325350453811141908784152855182021303702348128799969492197569484399949082732658437526092415392829643215097050899479054321015554395197474695503993880020048136162952698212665478743662704453297099020476154030750731279674535863197763480877179476411406758906207185444399617557170601368571637165525304136180750972374634776620222554801038905229485510413599707097071959942196477839839585991041792860807267993649083460952837805561304207244711497222513326918061789198146447180929094564313885966794355232799579072277794400620670657070124150771177585711610812844803375622592878283574377375613062102255052641500155906732042415216840552037111918678204487725829660200317601315839078905036370239244735885017850556956812780138385455569122831096982157452862235514673928711143996297287581996128001405296111487354519295462104632501860016676773154414028264673676158068728345582671237828356773077048059408538569264849878799040575734617574147265565218569013332644029805006825134580440218940081157441971487013677708076933817215542884708908317848695982862604062422295748639492560711161519371064008864769624060406499705673155138060100032374220988315905499399150924982262122176951380879779827501174626185429267140731971834835313295698769761349718661709868967208510769908277546515101646556413205110199862280310419106149455725152101604856535539580092397709045086080175320162084144727199659334428856818365968526
Expert Insights on Utilizing Pi in Python Programming
Dr. Emily Carter (Mathematician and Data Scientist, Tech Innovations Inc.). “Using the mathematical constant pi in Python is straightforward, especially with the built-in math module. By importing this module, developers can access the value of pi through math.pi, which ensures precision in calculations involving circles and trigonometric functions.”
James Liu (Software Engineer and Python Specialist, CodeCraft Solutions). “When working with pi in Python, it is essential to remember that floating-point arithmetic can introduce small errors. For high-precision applications, consider using the Decimal module, which allows for better control over the precision of pi and other constants.”
Dr. Sarah Thompson (Computer Scientist and Educator, Python Programming Academy). “Incorporating pi into Python projects often involves graphical representations, such as plotting circles or arcs. Libraries like Matplotlib can be used effectively to visualize these mathematical concepts, making it easier for students and professionals to understand the applications of pi in real-world scenarios.”
Frequently Asked Questions (FAQs)
How can I import the value of pi in Python?
You can import the value of pi by using the `math` module. Simply include the line `import math` in your code, and then use `math.pi` to access the value of pi.
What is the precision of pi in Python?
The precision of pi in Python, when using the `math` module, is approximately 15 decimal places. This is generally sufficient for most calculations requiring pi.
Can I use pi for mathematical calculations in Python?
Yes, you can use pi for various mathematical calculations. For example, you can calculate the area of a circle using the formula `area = math.pi * radius ** 2`.
Is there a way to define my own value of pi in Python?
While you can define your own value of pi, it is recommended to use `math.pi` for consistency and accuracy. If you choose to define your own, you can do so with a line like `my_pi = 3.14159`.
Are there alternatives to the math module for using pi in Python?
Yes, alternatives include the `numpy` library, which also provides a constant for pi. You can access it using `numpy.pi` after importing the library with `import numpy as np`.
Can I use pi in Python for symbolic mathematics?
Yes, you can use pi in symbolic mathematics by utilizing the `sympy` library. After importing it with `from sympy import pi`, you can perform algebraic manipulations involving pi symbolically.
In Python, the mathematical constant pi can be utilized effectively through the built-in `math` module, which provides a constant `math.pi` representing the value of pi to a high degree of precision. This allows developers and mathematicians to perform calculations involving circles, trigonometric functions, and other mathematical computations that require the use of pi without the need to define it manually. The `math` module also includes various functions that leverage pi, such as `math.sin()`, `math.cos()`, and `math.tan()`, which are essential for performing trigonometric calculations.
Additionally, pi can be employed in data visualization libraries like Matplotlib, where it can be used to create circular plots or graphs that require circular geometry. By understanding how to access and implement pi in Python, users can enhance their programming capabilities in scientific computing, data analysis, and graphical representations. Furthermore, the use of libraries such as NumPy also provides additional functionalities for handling arrays and performing advanced mathematical operations involving pi.
In summary, utilizing pi in Python is straightforward and efficient, thanks to the availability of the `math` module and other libraries. By incorporating pi into various mathematical and graphical applications, programmers can achieve accurate results while maintaining clean and readable
Author Profile
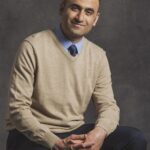
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?