How Can You Use Python to Build Your Own Website?
Creating a website can seem like a daunting task, especially for those who are new to programming. However, with Python, one of the most versatile and powerful programming languages available today, building a website becomes an accessible and enjoyable endeavor. Whether you’re looking to develop a personal blog, an online portfolio, or a complex web application, Python offers a range of frameworks and tools that streamline the process, making it easier than ever to bring your ideas to life.
In this article, we will explore the various ways you can leverage Python to create a website, from simple static pages to dynamic, interactive web applications. We’ll delve into popular frameworks such as Flask and Django, which provide robust solutions for web development, allowing you to focus on building features rather than getting bogged down by the intricacies of web technologies. Additionally, we’ll touch on essential concepts like routing, templates, and database integration, which are crucial for developing a fully functional site.
By the end of this guide, you’ll have a solid understanding of how to use Python to make a website, empowering you to embark on your own web development journey. Whether you’re a complete beginner or someone looking to expand your skill set, this article will equip you with the knowledge and confidence to turn your web ideas into reality. So, let’s dive
Choosing a Web Framework
Selecting the right web framework is crucial for developing a website in Python. The framework you choose can significantly influence the development speed, scalability, and maintenance of your web application. Popular frameworks include:
- Flask: A lightweight and flexible micro-framework ideal for small applications or for those who prefer to build their components from scratch.
- Django: A high-level framework that follows the “batteries-included” philosophy, providing many built-in features such as an ORM, authentication, and an admin panel.
- FastAPI: Designed for building APIs with modern Python features, it leverages asynchronous programming and is known for its high performance.
Each framework has its strengths and use cases, and your choice should align with your project’s requirements.
Setting Up Your Development Environment
To begin building a website using Python, you need to set up your development environment. Follow these steps:
- Install Python: Ensure you have the latest version of Python installed on your machine. You can download it from the official Python website.
- Set Up a Virtual Environment: Using a virtual environment helps manage dependencies for different projects. You can create one using:
“`bash
python -m venv myenv
“`
Activate it with:
- On Windows: `myenv\Scripts\activate`
- On macOS/Linux: `source myenv/bin/activate`
- Install the Framework: Depending on your choice of framework, install it via pip. For example:
“`bash
pip install Flask
“`
or
“`bash
pip install Django
“`
Building Your First Web Application
Once your environment is set up, you can start building your web application. Below is an example of how to create a simple web app using Flask.
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
To run this application, save it as `app.py` and execute it with:
“`bash
python app.py
“`
Your web application will be accessible at `http://127.0.0.1:5000`.
Understanding Routing and Views
Routing is a core concept in web development that maps URLs to functions in your application. Each route corresponds to a specific view (a function that handles a request).
Route | Function | Description |
---|---|---|
`/` | `home()` | Displays the homepage |
`/about` | `about()` | Displays information about the site |
`/contact` | `contact()` | Displays a contact form |
You can define additional routes in your Flask application as follows:
“`python
@app.route(‘/about’)
def about():
return “About Us”
@app.route(‘/contact’)
def contact():
return “Contact Us”
“`
This structure allows you to expand your application easily by adding more routes and corresponding views.
Templates and Static Files
To create dynamic content, you can use templates. Flask utilizes Jinja2 templating engine, which allows you to create HTML files with placeholders for dynamic data.
- Create a `templates` folder in your project directory.
- Add an HTML file, e.g., `index.html`:
“`html
{{ title }}
Welcome to my website!
“`
- Render this template in your route:
“`python
from flask import render_template
@app.route(‘/’)
def home():
return render_template(‘index.html’, title=’Home Page’)
“`
Static files, such as CSS and JavaScript, can be placed in a `static` folder, allowing you to manage your website’s front-end resources effectively.
Database Integration
For applications that require data persistence, integrating a database is essential. Django comes with its own ORM, while Flask can be paired with SQLAlchemy or other ORMs.
Basic steps for database integration:
- Choose a database (e.g., SQLite, PostgreSQL).
- Install the necessary libraries, like `Flask-SQLAlchemy` for Flask.
- Define your database models.
- Perform CRUD operations through your application logic.
By following these guidelines, you can effectively utilize Python to create a comprehensive web application tailored to your specific needs.
Choosing a Framework
Selecting an appropriate framework is crucial when using Python to develop a website. Several popular frameworks cater to different needs and complexity levels:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with built-in features like an ORM, authentication, and an admin panel.
- Flask: A lightweight WSGI web application framework designed for simplicity and flexibility. Flask is ideal for smaller applications or microservices.
- FastAPI: A modern, fast (high-performance) framework for building APIs with Python 3.6+ based on standard Python type hints. It’s especially good for asynchronous programming.
- Pyramid: A flexible framework that can scale from simple to complex applications. It allows developers to choose their components.
Framework | Use Case | Learning Curve | Built-in Features |
---|---|---|---|
Django | Full-featured web apps | Moderate | ORM, Admin, Auth |
Flask | Microservices, small apps | Easy | Minimalistic |
FastAPI | APIs, async apps | Moderate | Async support, type hints |
Pyramid | Flexible, scalable apps | Moderate | Customizable |
Setting Up the Environment
To start developing with Python, you need to set up a suitable environment:
- Install Python: Download and install Python from the [official website](https://www.python.org/downloads/).
- Create a Virtual Environment:
- Use `venv` to create isolated environments:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
“`
- Install Required Packages:
- Use `pip` to install your chosen framework:
“`bash
pip install django or flask, fastapi, pyramid
“`
Building the Application
Once the environment is set up, you can start building your application:
- For Django:
- Create a new project:
“`bash
django-admin startproject myproject
cd myproject
python manage.py runserver
“`
- Build your models, views, and templates.
- For Flask:
- Create a simple application:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- For FastAPI:
- Build a simple API:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
async def read_root():
return {“Hello”: “World”}
“`
Deploying the Website
After development, deployment is necessary to make your website accessible:
- Choose a Hosting Service:
- Options include Heroku, AWS, DigitalOcean, and PythonAnywhere.
- Prepare Your Application:
- For Django, ensure settings are production-ready, collect static files, and set up a WSGI server like Gunicorn.
- For Flask or FastAPI, prepare a WSGI server configuration.
- Deployment Steps:
- Push your code to the chosen platform (e.g., GitHub for Heroku).
- Set environment variables for production settings.
- Start your application using the hosting service’s instructions.
Adding a Database
Integrating a database allows you to store and manage data effectively:
- Choose a Database:
- Common options include PostgreSQL, MySQL, and SQLite.
- For Django:
- Modify the `settings.py` file to configure the database connection.
- For Flask:
- Use SQLAlchemy or Flask-SQLAlchemy to manage database interactions.
- For FastAPI:
- Integrate with databases using SQLAlchemy or async libraries like databases.
Framework | Database Support | ORM Support |
---|---|---|
Django | PostgreSQL, MySQL, SQLite | Django ORM |
Flask | Various SQL databases | SQLAlchemy |
FastAPI | Various SQL databases | SQLAlchemy, Tortoise ORM |
Pyramid | Various SQL databases | SQLAlchemy |
Testing Your Application
Testing ensures your application functions correctly:
- Use built-in testing frameworks:
- Django has a comprehensive testing framework.
- Flask supports unit testing with the `unittest` module.
- FastAPI provides testing features through `TestClient`.
- Key testing components:
- Unit tests for individual functions.
- Integration tests for the application as a whole.
- Use mocking libraries to simulate database or API interactions.
Expert Insights on Using Python to Build a Website
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Using Python to create a website can be highly efficient, especially with frameworks like Django and Flask. These frameworks provide robust tools for rapid development, allowing developers to focus on functionality rather than repetitive coding tasks.”
Michael Thompson (Web Development Instructor, Code Academy). “For beginners, I recommend starting with Flask due to its simplicity and flexibility. It allows you to learn the basics of web development without overwhelming you with too many features right away.”
Sarah Lee (Full-Stack Developer, Digital Solutions Group). “Integrating Python with front-end technologies like HTML, CSS, and JavaScript is crucial. Understanding how to connect your Python backend with a responsive front end will significantly enhance the user experience of your website.”
Frequently Asked Questions (FAQs)
How can I start using Python to make a website?
To start using Python for web development, you need to choose a web framework such as Flask or Django. Install the framework, set up a virtual environment, and create a basic project structure to begin building your website.
What are the best Python frameworks for web development?
The best Python frameworks for web development include Django, Flask, FastAPI, and Pyramid. Django is suitable for large applications, while Flask is ideal for smaller projects due to its simplicity and flexibility.
Do I need to know HTML and CSS to use Python for web development?
Yes, a basic understanding of HTML and CSS is essential for web development with Python. These technologies are used for structuring and styling web pages, while Python handles the backend logic.
Can I use Python for both frontend and backend development?
Python is primarily used for backend development. However, you can integrate it with frontend technologies like JavaScript, HTML, and CSS to create a full-stack web application. Tools like Brython allow some Python code to run in the browser.
How do I deploy a Python web application?
To deploy a Python web application, choose a hosting service that supports Python, such as Heroku, AWS, or DigitalOcean. Prepare your application for production, configure the server, and upload your code to make it accessible online.
What databases can I use with Python web applications?
You can use various databases with Python web applications, including SQLite, PostgreSQL, MySQL, and MongoDB. The choice of database often depends on the specific needs of your application and the framework you are using.
In summary, using Python to create a website involves several key steps and considerations. Python offers a variety of frameworks and libraries, such as Flask and Django, which simplify the web development process. These frameworks provide essential tools for routing, templating, and managing databases, enabling developers to focus on building functionality rather than dealing with low-level details.
Additionally, understanding the fundamentals of HTML, CSS, and JavaScript is crucial for creating a well-rounded web application. While Python handles the backend logic, these front-end technologies are necessary for designing the user interface and ensuring a seamless user experience. Integrating Python with these technologies allows for dynamic content generation and interactive features.
Moreover, deploying the website requires knowledge of web servers and cloud services. Platforms like Heroku, AWS, and DigitalOcean facilitate the hosting of Python applications, making them accessible to users worldwide. It is also essential to consider security practices and performance optimization to ensure the website runs efficiently and safely.
Ultimately, leveraging Python for web development not only enhances productivity but also provides a robust environment for building scalable applications. With the right tools and knowledge, developers can create sophisticated websites that meet diverse user needs and business objectives.
Author Profile
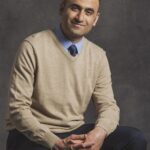
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?