How Can You Effectively Implement Waiting in Python?
In the fast-paced world of programming, efficiency is key, but sometimes, a little patience goes a long way. Whether you’re building a web scraper, automating tasks, or developing a game, there are moments when your code needs to pause and wait. This might be to allow a resource to load, to synchronize threads, or to introduce a delay for user experience. In Python, mastering the art of waiting can enhance your code’s functionality and responsiveness, ensuring it runs smoothly without unnecessary hiccups.
Understanding how to effectively implement wait times in Python can significantly improve your programming toolkit. From simple pauses that allow for smoother execution to more complex synchronization techniques that manage concurrent tasks, Python offers a variety of methods to control the flow of your code. By leveraging built-in libraries and functions, you can create more robust applications that handle timing and delays gracefully.
As we delve deeper into the various techniques for waiting in Python, you’ll discover the nuances of time management within your code. We’ll explore different scenarios where waiting might be necessary, the tools available for implementing these pauses, and best practices to ensure your code remains efficient and user-friendly. Whether you’re a novice looking to understand the basics or an experienced developer seeking to refine your skills, the insights to come will empower you to wield the power of
Using time.sleep()
The simplest method to implement a waiting period in Python is through the `time.sleep()` function. This function pauses the execution of the current thread for a specified number of seconds. The argument can be a floating-point number to indicate a fractional second.
Example usage:
python
import time
print(“Wait for 2 seconds…”)
time.sleep(2)
print(“Done waiting!”)
Key points about `time.sleep()`:
- It accepts a single argument: the number of seconds to sleep.
- It blocks the current thread, meaning no other tasks can be executed in that thread during the sleep period.
Using threading.Event() for more control
For scenarios requiring more granular control over execution, the `threading` module provides the `Event` class. This allows threads to wait until an event is set.
Example:
python
import threading
event = threading.Event()
def wait_for_event():
print(“Waiting for the event to be set…”)
event.wait() # Wait until the event is set
print(“Event is set, continuing execution.”)
thread = threading.Thread(target=wait_for_event)
thread.start()
# Simulate some processing
time.sleep(3)
event.set() # Set the event to allow the thread to continue
Using asyncio for asynchronous waiting
In asynchronous programming, the `asyncio` library provides an elegant way to wait without blocking the entire program. The `await asyncio.sleep()` function is non-blocking and allows other tasks to run while waiting.
Example:
python
import asyncio
async def main():
print(“Waiting asynchronously for 2 seconds…”)
await asyncio.sleep(2)
print(“Done waiting!”)
asyncio.run(main())
Wait with a timeout
Sometimes, it is useful to implement a wait with a timeout, especially when dealing with events or conditions that may not occur. The `threading.Event.wait()` method can accept a timeout argument.
Example:
python
event = threading.Event()
def wait_with_timeout():
print(“Waiting for the event with a timeout of 5 seconds…”)
result = event.wait(timeout=5)
if not result:
print(“Timeout occurred, continuing execution.”)
else:
print(“Event was set before timeout.”)
thread = threading.Thread(target=wait_with_timeout)
thread.start()
Comparative Summary of Waiting Methods
The following table summarizes the different methods for implementing wait in Python, highlighting their characteristics and use cases:
Method | Blocking | Asynchronous | Use Case |
---|---|---|---|
time.sleep() | Yes | No | Simple delays |
threading.Event | Yes (until event is set) | No | Thread synchronization |
asyncio.sleep() | No | Yes | Non-blocking asynchronous tasks |
threading.Event with timeout | Yes (with timeout) | No | Waiting with timeout option |
By understanding these various methods, developers can choose the most appropriate technique for introducing delays in their Python applications based on their specific requirements.
Using the time.sleep() Function
The most straightforward way to introduce a delay in your Python code is by using the `time.sleep()` function from the built-in `time` module. This function suspends execution for a specified number of seconds.
Example usage:
python
import time
print(“Waiting for 5 seconds…”)
time.sleep(5)
print(“Done waiting!”)
This approach is ideal for simple delays, such as pacing loops or waiting for a specific condition.
Implementing Delays with Asyncio
For asynchronous programming, `asyncio` provides a method to wait without blocking the entire program. The `asyncio.sleep()` function allows you to create non-blocking waits.
Example usage:
python
import asyncio
async def main():
print(“Waiting for 3 seconds…”)
await asyncio.sleep(3)
print(“Done waiting!”)
asyncio.run(main())
This method is particularly useful in applications that require concurrent operations, such as web servers or applications with I/O-bound tasks.
Using Threading with Event Objects
If you are working with multi-threading, you can use an `Event` object from the `threading` module to implement a wait mechanism.
Example usage:
python
import threading
event = threading.Event()
def wait_and_set():
print(“Waiting for 4 seconds…”)
time.sleep(4)
event.set()
print(“Event set!”)
thread = threading.Thread(target=wait_and_set)
thread.start()
event.wait()
print(“Event was triggered, continuing execution.”)
This method is beneficial when you need to synchronize threads and wait for a specific condition or signal.
Using the timeit Module for Timing Code Execution
If the goal is to measure execution time rather than introduce a wait, the `timeit` module can be used. This module provides a simple way to time small bits of Python code.
Example usage:
python
import timeit
execution_time = timeit.timeit(“time.sleep(2)”, setup=”import time”, number=1)
print(f”Execution time: {execution_time} seconds”)
Using `timeit` allows you to benchmark code snippets without manually introducing delays.
Combining Wait with Condition Variables
Condition variables provide a way for threads to wait until they are notified. This is particularly useful for managing complex inter-thread communication.
Example usage:
python
from threading import Condition
import time
condition = Condition()
def waiting_thread():
with condition:
print(“Waiting for the condition to be notified…”)
condition.wait()
print(“Condition has been notified!”)
def notifying_thread():
time.sleep(5)
with condition:
print(“Notifying the waiting thread…”)
condition.notify()
thread1 = threading.Thread(target=waiting_thread)
thread2 = threading.Thread(target=notifying_thread)
thread1.start()
thread2.start()
This approach ensures that the waiting thread only continues execution when a specific condition is met, enhancing synchronization in multithreaded applications.
Expert Insights on Implementing Waits in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, implementing waits can be crucial for synchronizing tasks, especially in asynchronous programming. Utilizing the `asyncio.sleep()` function allows developers to pause execution without blocking the entire thread, which is essential for maintaining responsiveness in applications.”
Mark Thompson (Lead Developer, Python Development Group). “For scenarios involving web scraping or API calls, the `time.sleep()` function is often employed to avoid overwhelming servers with requests. This simple approach can help manage rate limits effectively, ensuring compliance with usage policies while maintaining the flow of data retrieval.”
Linda Garcia (Automation Specialist, Quality Assurance Solutions). “In automated testing frameworks, implementing waits is vital to ensure that elements are fully loaded before interactions occur. Using explicit waits with libraries like Selenium allows for more precise control over timing, which enhances the reliability of test scripts and reduces flakiness.”
Frequently Asked Questions (FAQs)
How can I pause execution in Python?
You can pause execution in Python using the `time.sleep(seconds)` function from the `time` module, where `seconds` is the number of seconds to wait.
What is the purpose of the `time.sleep()` function?
The `time.sleep()` function is used to delay the execution of the program for a specified amount of time, which can be useful for controlling the timing of events in your code.
Can I wait for a fraction of a second in Python?
Yes, you can specify a fractional value in `time.sleep()`, such as `time.sleep(0.5)` to pause for half a second.
Are there alternatives to `time.sleep()` for waiting in Python?
Yes, alternatives include using `threading.Event().wait(timeout)` for more complex scenarios or `asyncio.sleep()` in asynchronous programming to yield control without blocking.
How do I implement a countdown timer in Python?
You can implement a countdown timer using a loop that decrements a variable and calls `time.sleep(1)` to wait one second between decrements, displaying the countdown each time.
Is it possible to wait for user input in Python?
Yes, you can use the `input()` function to wait for user input, which halts program execution until the user provides input and presses Enter.
In Python, waiting or introducing delays in code execution can be achieved through various methods, each suited for different scenarios. The most common approach is using the `time.sleep()` function from the built-in `time` module, which pauses the execution of the program for a specified number of seconds. This method is straightforward and effective for simple delays, such as in scripts that require waiting between actions.
For more complex applications, particularly those involving asynchronous programming, the `asyncio` library provides the `await asyncio.sleep()` function. This method allows for non-blocking waits, enabling other tasks to run concurrently while waiting, which is essential in applications that require high responsiveness, such as web servers or GUI applications.
Additionally, in scenarios where waiting is contingent on certain conditions, techniques such as polling with loops or using threading events can be employed. These methods allow for more control over the waiting process, ensuring that the program can react to changes in state or user input while still managing delays effectively.
In summary, Python offers multiple ways to implement waiting in code, ranging from simple time delays to more sophisticated asynchronous handling. Understanding the context and requirements of your application will guide you in selecting the most appropriate waiting mechanism, ultimately enhancing the
Author Profile
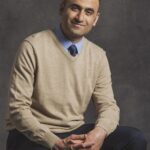
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?