How Can You Write a List in Reverse Order Using Python?
### Introduction
In the world of programming, the ability to manipulate data structures efficiently is a fundamental skill every developer should master. One common task that arises frequently is the need to reverse a list. Whether you’re organizing data for better readability, preparing it for output, or simply experimenting with algorithms, knowing how to write a list in reverse order can save you time and enhance your coding prowess. In Python, a language celebrated for its simplicity and versatility, there are several elegant methods to achieve this, each with its own unique advantages.
Reversing a list in Python is not just about flipping the order of elements; it’s about understanding the various tools and techniques the language offers. From built-in functions to slicing techniques, Python provides multiple ways to accomplish this task, catering to different programming styles and preferences. As we delve deeper into the topic, you’ll discover how these methods can be applied in real-world scenarios, making your code more efficient and readable.
Moreover, grasping the concept of list reversal opens the door to more complex data manipulation tasks. It lays the groundwork for understanding algorithms and data structures, which are crucial for any aspiring programmer. So, whether you’re a novice eager to learn or an experienced coder looking to refine your skills, this exploration of reversing lists in Python will equip you with
Methods to Reverse a List in Python
In Python, there are several effective methods to reverse a list. Each method has its specific use cases and advantages. Below are some of the most common approaches.
Using the reverse() Method
The `reverse()` method is a built-in list method that reverses the elements of a list in place. This means that the original list is modified, and no new list is created.
python
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
Using Slicing
Slicing is a powerful feature in Python that allows you to create a new list that is a reversed version of the original. This method does not modify the original list.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Using the reversed() Function
The `reversed()` function returns an iterator that accesses the given list in the reverse order. To convert this iterator back to a list, you can use the `list()` constructor.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = list(reversed(my_list))
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Using a Loop
You can also reverse a list by iterating through it in reverse order. This method is more verbose but useful for more complex scenarios.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = []
for item in my_list:
reversed_list.insert(0, item)
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Comparison of Methods
The following table summarizes the characteristics of each method for reversing a list in Python:
Method | Modifies Original List | Returns New List | Time Complexity |
---|---|---|---|
reverse() | Yes | No | O(n) |
Slicing | No | Yes | O(n) |
reversed() | No | Yes | O(n) |
Loop | No | Yes | O(n^2) |
Choosing the right method depends on whether you need to maintain the original list, the complexity of the operation, and your specific use case.
Methods to Reverse a List in Python
In Python, there are several methods to reverse a list. Each method has its own use case depending on the requirements of your code. Below are the most common techniques:
Using the `reverse()` Method
The `reverse()` method reverses the elements of a list in place, meaning it modifies the original list.
python
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
Using Slicing
List slicing provides a concise way to create a reversed copy of a list. The syntax `list[::-1]` generates a new list with the elements in reverse order.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Using the `reversed()` Function
The `reversed()` function returns an iterator that accesses the given list in reverse order. To convert it back to a list, you can use the `list()` constructor.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = list(reversed(my_list))
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Using a Loop
A manual approach involves using a loop to append elements from the end of the list to a new list. This method is less efficient but useful for educational purposes.
python
my_list = [1, 2, 3, 4, 5]
reversed_list = []
for i in range(len(my_list) – 1, -1, -1):
reversed_list.append(my_list[i])
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Performance Considerations
When choosing a method to reverse a list, consider the following performance aspects:
Method | In-place Modification | Time Complexity | Space Complexity |
---|---|---|---|
`reverse()` | Yes | O(n) | O(1) |
Slicing | No | O(n) | O(n) |
`reversed()` | No | O(n) | O(n) |
Loop | No | O(n) | O(n) |
Choosing the appropriate method depends on whether you need to modify the original list or create a new one. For large lists, using `reverse()` is generally the most efficient option due to its in-place nature and lower space requirements.
Expert Insights on Writing Lists in Reverse Order in Python
Dr. Emily Carter (Senior Software Engineer, CodeCrafters Inc.). “To write a list in reverse order in Python, utilizing the built-in method `reverse()` is one of the most efficient approaches. This method modifies the list in place, which is particularly useful for memory management in larger datasets.”
Michael Chen (Data Scientist, Analytics Hub). “For those who prefer a more functional programming style, using slicing is an elegant solution. By employing the syntax `list[::-1]`, one can create a reversed copy of the list without altering the original, which is beneficial for maintaining data integrity.”
Sarah Johnson (Python Instructor, Tech Academy). “Another effective method is to use the `reversed()` function, which returns an iterator that can be converted back into a list. This approach is particularly useful when working with large lists where performance is a concern, as it avoids the overhead of creating a new list until necessary.”
Frequently Asked Questions (FAQs)
How can I reverse a list in Python?
You can reverse a list in Python using the `reverse()` method, which modifies the list in place, or by using slicing with `list[::-1]`, which creates a new reversed list.
What is the difference between `reverse()` and `reversed()` in Python?
The `reverse()` method reverses the list in place and returns `None`, while `reversed()` returns an iterator that accesses the given list in the reverse order without modifying the original list.
Can I reverse a list of strings in Python?
Yes, you can reverse a list of strings using the same methods, `reverse()` or slicing, as you would with a list of any other data type.
Is there a way to reverse a list without using built-in functions?
Yes, you can reverse a list manually by using a loop to swap elements from the start and end of the list until you reach the middle.
How do I reverse a list of numbers in Python?
You can reverse a list of numbers in Python using the same techniques, such as the `reverse()` method or slicing, as you would with any list.
What happens if I try to reverse an empty list in Python?
Reversing an empty list using either `reverse()` or slicing will simply return an empty list, as there are no elements to reverse.
Writing a list in reverse order in Python can be accomplished through various methods, each offering unique advantages depending on the specific context and requirements of the task. The most straightforward approach is to utilize the built-in `reverse()` method, which modifies the list in place. Alternatively, the slicing technique allows for a concise way to create a new reversed list without altering the original. Lastly, the `reversed()` function provides an iterator that can be converted back into a list, offering flexibility in how the reversed data is utilized.
When choosing a method to reverse a list, it is essential to consider factors such as the need to preserve the original list, the size of the data, and the clarity of the code. The `reverse()` method is efficient for in-place modifications, while slicing is often favored for its readability and simplicity. The `reversed()` function is particularly useful in scenarios where an iterator is more appropriate, allowing for memory-efficient operations on large datasets.
Python provides multiple effective methods for reversing a list, each suited to different programming needs. Understanding these options enables developers to select the most appropriate technique, enhancing both code performance and maintainability. By leveraging these tools, programmers can efficiently manipulate lists to meet their specific requirements
Author Profile
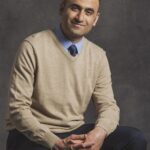
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?