How Can You Write a Program in Python? A Step-by-Step Guide
In today’s digital age, programming has become an essential skill, and Python stands out as one of the most accessible and versatile languages for beginners and seasoned developers alike. Whether you’re looking to automate mundane tasks, analyze data, or develop web applications, learning how to write a program in Python can open up a world of possibilities. With its clean syntax and extensive libraries, Python enables you to transform your ideas into functional code with ease. In this article, we will guide you through the fundamental concepts and practical steps to embark on your Python programming journey.
To write a program in Python, one must first grasp the foundational elements of the language, including variables, data types, and control structures. Understanding these core concepts lays the groundwork for developing more complex applications. Python’s readability and simplicity make it an ideal choice for newcomers, allowing you to focus on problem-solving rather than getting bogged down by intricate syntax.
Once you have a firm grasp of the basics, the next step is to explore the various tools and environments available for coding in Python. From integrated development environments (IDEs) to online coding platforms, there are numerous options to suit your preferences. As you progress, you’ll discover the importance of writing clean, efficient code and the value of debugging and testing your programs
Understanding Python Syntax
Python syntax is designed to be clear and readable, which is one of the reasons it is favored by beginners and experienced programmers alike. Unlike many programming languages, Python uses indentation to define the structure and flow of the code rather than braces or keywords. This enforces a clean and visually appealing layout that enhances code readability.
Key elements of Python syntax include:
- Variables: Created by simply assigning a value to a name, e.g., `x = 10`.
- Data Types: Python supports various data types such as integers, floats, strings, and lists.
- Comments: Use the “ symbol to add comments for better code documentation.
Basic Structure of a Python Program
A typical Python program consists of the following components:
- Import Statements: Used to include external modules or libraries.
- Function Definitions: Defined using the `def` keyword, which allows you to encapsulate code for reuse.
- Main Execution Block: The entry point of the program, often protected by the `if __name__ == “__main__”:` conditional.
Here is a simple example of a basic Python program structure:
“`python
Importing necessary libraries
import math
Function definition
def calculate_square_root(number):
return math.sqrt(number)
Main execution block
if __name__ == “__main__”:
result = calculate_square_root(16)
print(“The square root is:”, result)
“`
Common Python Data Structures
Python provides several built-in data structures that can be utilized to manage collections of data. The most common include:
- Lists: Ordered and mutable collections.
- Tuples: Ordered and immutable collections.
- Dictionaries: Key-value pairs for quick lookups.
- Sets: Unordered collections of unique elements.
Here’s a table summarizing these data structures:
Data Structure | Mutability | Use Case |
---|---|---|
List | Mutable | Ordered collections, allow duplicates |
Tuple | Immutable | Fixed collections, used for data integrity |
Dictionary | Mutable | Key-value pairs for fast access |
Set | Mutable | Unique collections, used for membership testing |
Control Flow in Python
Control flow statements allow you to dictate the order in which code executes based on certain conditions. The primary control flow structures in Python include:
– **Conditional Statements**: Use `if`, `elif`, and `else` to execute code based on conditions.
– **Loops**: Utilize `for` and `while` loops to repeat actions.
Example of a conditional statement:
“`python
age = 18
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
Example of a loop:
“`python
for i in range(5):
print(“Iteration:”, i)
“`
Writing Functions in Python
Functions in Python are defined using the `def` keyword and are essential for creating reusable blocks of code. A function can take parameters, perform operations, and return results. Here’s an example:
“`python
def multiply(a, b):
return a * b
result = multiply(4, 5)
print(“The product is:”, result)
“`
Functions enhance modularity and make testing easier, as each function can be evaluated independently.
Understanding the Basics of Python Programming
To effectively write a program in Python, it is crucial to grasp the foundational concepts of the language. Python is known for its simplicity and readability, making it an ideal choice for beginners. Here are some fundamental elements:
- Variables: Store data values.
- Data Types: Common types include integers, floats, strings, and booleans.
- Operators: Used for performing operations on variables and values (e.g., arithmetic, comparison, logical).
- Control Structures: Includes conditionals (if, else) and loops (for, while).
Setting Up Your Environment
Before writing Python code, set up your development environment. You can choose between using an Integrated Development Environment (IDE) or a simple text editor.
Popular IDEs and Text Editors:
IDE/Text Editor | Features |
---|---|
PyCharm | Advanced features for professional development |
Visual Studio Code | Lightweight, customizable, with extensive extensions |
Jupyter Notebook | Ideal for data science and interactive coding |
Sublime Text | Fast and powerful with a clean interface |
Install Python from the official website, ensuring that you have the correct version for your operating system.
Writing Your First Python Program
The classic first program in Python is the “Hello, World!” example. Open your chosen IDE or text editor and create a new file named `hello.py`. Write the following code:
“`python
print(“Hello, World!”)
“`
To execute the program, use the command line or terminal:
“`bash
python hello.py
“`
This will output:
“`
Hello, World!
“`
Basic Syntax and Structure
Understanding Python’s syntax is essential for writing effective programs. Key components include:
- Indentation: Python uses indentation to define code blocks. Consistent spacing is crucial.
- Comments: Use “ for single-line comments and triple quotes for multi-line comments.
Example:
“`python
This is a single-line comment
“””
This is a multi-line comment
“””
“`
Utilizing Functions
Functions in Python allow you to encapsulate code for reuse. Define a function using the `def` keyword.
Example:
“`python
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
“`
This function will output:
“`
Hello, Alice!
“`
Working with Data Structures
Python offers several built-in data structures that facilitate data organization. Key data structures include:
- Lists: Ordered, mutable collections.
- Tuples: Ordered, immutable collections.
- Dictionaries: Key-value pairs, unordered collections.
- Sets: Unordered collections of unique elements.
Example of a dictionary:
“`python
student = {
“name”: “John”,
“age”: 21,
“courses”: [“Math”, “Science”]
}
“`
Error Handling
Implement error handling using `try` and `except` blocks to manage exceptions gracefully.
Example:
“`python
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero!”)
“`
This code will output:
“`
Cannot divide by zero!
“`
Conclusion on Python Programming Principles
Familiarizing yourself with these fundamental principles and practices will significantly enhance your ability to write effective Python programs. As you progress, explore advanced topics such as object-oriented programming, libraries, and frameworks to deepen your understanding and proficiency in Python.
Expert Insights on Writing Programs in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When writing a program in Python, it is crucial to start with a clear understanding of the problem you are trying to solve. Break down the requirements into manageable parts and utilize Python’s extensive libraries to streamline your development process.”
Michael Thompson (Python Developer Advocate, CodeCraft Academy). “Emphasizing code readability is essential when writing in Python. Use meaningful variable names and adhere to PEP 8 guidelines to ensure that your code is not only functional but also maintainable by others in the future.”
Sarah Nguyen (Lead Data Scientist, Data Insights Corp.). “To effectively write a program in Python, leverage interactive environments like Jupyter Notebooks for prototyping. This approach allows for immediate feedback and iterative development, which can significantly enhance your coding efficiency.”
Frequently Asked Questions (FAQs)
What are the basic steps to write a program in Python?
To write a program in Python, start by installing Python on your machine. Next, choose a code editor or an integrated development environment (IDE) such as PyCharm or Visual Studio Code. Write your code in the editor, save the file with a `.py` extension, and run it using the command line or the IDE’s built-in features.
What is the syntax for printing output in Python?
In Python, you can print output using the `print()` function. For example, `print(“Hello, World!”)` will display the text “Hello, World!” in the console.
How do I handle user input in a Python program?
To handle user input, use the `input()` function. For instance, `name = input(“Enter your name: “)` prompts the user for their name and stores it in the variable `name`.
What are variables and data types in Python?
Variables in Python are used to store data values. Python supports several data types, including integers, floats, strings, and lists. You can declare a variable by assigning a value, such as `age = 30` for an integer.
How do I create functions in Python?
To create a function in Python, use the `def` keyword followed by the function name and parentheses. For example:
“`python
def greet(name):
print(“Hello, ” + name)
“`
This defines a function named `greet` that takes one parameter and prints a greeting.
What are libraries and how do I use them in Python?
Libraries in Python are collections of pre-written code that provide additional functionality. To use a library, you must first import it using the `import` statement, such as `import math` for mathematical functions. You can then call functions from the library, like `math.sqrt(16)` to calculate the square root of 16.
Writing a program in Python involves several fundamental steps that are essential for both beginners and experienced developers. First, it is crucial to have a clear understanding of the problem you intend to solve. This includes defining the requirements and outlining the desired functionality of the program. Once the problem is well-defined, you can begin planning your program structure, which typically involves breaking down the solution into smaller, manageable components or functions.
Next, setting up your development environment is vital. This includes installing Python and any necessary libraries or frameworks that will aid in your programming tasks. Utilizing an Integrated Development Environment (IDE) or a code editor can significantly enhance your coding experience by providing features like syntax highlighting, debugging tools, and code completion. After the environment is ready, you can start writing the code, following best practices such as using meaningful variable names, adhering to PEP 8 style guidelines, and including comments for clarity.
Testing and debugging your program is another critical aspect of the development process. It is important to run your code frequently to identify and fix any errors or bugs. Implementing unit tests can help ensure that individual components of your program work as intended. Finally, once the program is complete, consider documenting your code and sharing it with others, whether
Author Profile
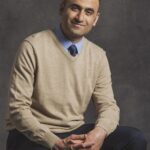
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?