How Can I Write a Python Program? A Step-by-Step Guide for Beginners
How to Write a Python Program: A Beginner’s Guide to Coding Magic
In an age where technology shapes our daily lives, programming has become an essential skill for both personal and professional growth. Among the myriad of programming languages available, Python stands out as one of the most accessible and versatile options for beginners. Whether you aspire to develop web applications, automate mundane tasks, or dive into the world of data science, learning how to write a Python program is your first step towards unlocking a realm of possibilities. This article will guide you through the foundational concepts and practical steps to embark on your coding journey.
Writing a Python program may seem daunting at first, but with a clear understanding of its syntax and structure, you’ll find it to be an intuitive and enjoyable process. Python’s readability and simplicity allow newcomers to grasp programming concepts without getting bogged down by complex jargon. From setting up your development environment to crafting your first lines of code, this guide will provide you with the essential tools and knowledge to start creating your own programs.
As you delve deeper into the world of Python, you’ll discover the power of libraries and frameworks that can enhance your coding experience and expand your capabilities. With a supportive community and a wealth of resources at your fingertips, the journey of learning how to write a
Understanding Python Basics
To effectively write a Python program, it’s essential to grasp the fundamental concepts of the language. Python is known for its readability and simplicity, making it an excellent choice for beginners and experienced programmers alike. Key elements to understand include variables, data types, control structures, functions, and modules.
- Variables: Used to store data values. Python is dynamically typed, meaning you do not need to declare the type of variable.
- Data Types: Common types include integers, floats, strings, and booleans. Understanding how to work with these types is crucial for effective programming.
- Control Structures: These include conditionals (`if`, `elif`, `else`) and loops (`for`, `while`), which allow you to control the flow of your program.
Setting Up Your Environment
Before writing your first Python program, you must set up your development environment. This typically involves installing Python and an Integrated Development Environment (IDE) or a text editor.
- Download Python: Visit the official [Python website](https://www.python.org/downloads/) to download the latest version.
- Choose an IDE/Text Editor: Options include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Sublime Text
The choice of IDE depends on your personal preference and the complexity of the projects you intend to work on.
IDE/Text Editor | Key Features | Best For |
---|---|---|
PyCharm | Code completion, debugging tools, integrated version control | Professional development |
Visual Studio Code | Lightweight, customizable, vast extensions library | General purpose |
Jupyter Notebook | Interactive coding, visualization support | Data science and analysis |
Sublime Text | Fast performance, distraction-free writing | Simple scripting and quick edits |
Writing Your First Python Program
Once your environment is set up, you can proceed to write your first Python program. A traditional first program is to print “Hello, World!” to the console.
- Open your chosen IDE or text editor.
- Create a new file and name it `hello.py`.
- Type the following code:
“`python
print(“Hello, World!”)
“`
- Save the file and run it. In most IDEs, you can run the program directly. In command line, you can execute it by navigating to the file’s directory and typing:
“`bash
python hello.py
“`
This simple program demonstrates the basic syntax and functionality of Python.
Debugging and Testing Your Code
Debugging is an essential part of programming, allowing you to identify and fix errors in your code. Python offers built-in tools and practices for effective debugging:
- Print Statements: Use `print()` to output variable values and track the flow of execution.
- Python Debugger (pdb): A powerful tool that allows you to set breakpoints, step through code, and inspect variables.
- Unit Testing: Use the `unittest` framework to create tests for your code, ensuring it behaves as expected.
By incorporating these debugging techniques, you can enhance your programming skills and produce more reliable code.
Understanding the Basics of Python
Python is a versatile and powerful programming language that is widely used for various applications, from web development to data analysis. Before writing a program, it’s essential to familiarize yourself with the fundamental concepts of Python, including:
- Syntax: The set of rules that defines the combinations of symbols that are considered to be correctly structured programs in Python.
- Variables: Named storage locations that hold data which can be modified during program execution.
- Data Types: Common data types in Python include:
- Integers (`int`)
- Floating-point numbers (`float`)
- Strings (`str`)
- Lists (`list`)
- Dictionaries (`dict`)
Understanding these elements will help in writing effective and efficient Python programs.
Setting Up Your Python Environment
Before you can write a Python program, you need to set up your development environment. Here’s how to do it:
- Install Python: Download the latest version of Python from the official website (python.org) and follow the installation instructions for your operating system.
- Choose an Integrated Development Environment (IDE): Some popular IDEs for Python include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Verify Installation: Open a terminal or command prompt and type:
“`bash
python –version
“`
This command should display the installed version of Python.
Writing Your First Python Program
To write a simple Python program, follow these steps:
- Open your chosen IDE.
- Create a new file with a `.py` extension.
- Write the following code:
“`python
print(“Hello, World!”)
“`
- Save the file.
- Run the program:
- In a terminal, navigate to the directory where your file is located and type:
“`bash
python your_file_name.py
“`
This program outputs “Hello, World!” to the console, serving as a foundational example of how Python code is structured and executed.
Basic Python Constructs
When writing Python programs, it’s crucial to understand several key constructs:
- Control Structures: These include conditional statements and loops.
- If Statement: Allows for decision-making.
“`python
if condition:
code to execute if condition is true
“`
- For Loop: Iterates over a sequence.
“`python
for item in sequence:
code to execute for each item
“`
- Functions: Reusable blocks of code that perform a specific task.
“`python
def function_name(parameters):
code to execute
return result
“`
- Error Handling: Use try-except blocks to manage exceptions.
“`python
try:
code that may cause an exception
except ExceptionType:
code to execute if exception occurs
“`
Organizing Your Code
As your programs grow, organization becomes essential. Here are best practices:
- Modularization: Break your code into functions or classes to enhance readability and maintainability.
- Comments: Use comments to explain complex logic or decisions within your code.
“`python
This is a comment explaining the following line of code
“`
- Consistent Naming Conventions: Use descriptive variable names and follow Python naming conventions (e.g., snake_case for variables and functions).
Testing and Debugging Your Program
Testing and debugging are vital components of the programming process. Consider the following strategies:
- Print Statements: Use print statements to track variable values and program flow.
- Unit Testing: Implement unit tests using the `unittest` module to ensure each part of your program works as expected.
- Debugging Tools: Utilize debugging tools available in your IDE to step through your code and inspect variables in real time.
By mastering these aspects, you will be well on your way to writing effective and efficient Python programs.
Expert Insights on Writing Python Programs
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To write an effective Python program, one must first understand the problem domain thoroughly. This involves breaking down the problem into manageable components and leveraging Python’s extensive libraries to streamline the development process.”
James Liu (Python Developer Advocate, CodeCrafters). “A successful Python program is not just about syntax; it is about writing clean, maintainable code. Adopting best practices such as PEP 8 for style guidelines can significantly enhance the readability and efficiency of your code.”
Sarah Thompson (Lead Data Scientist, Data Insights Group). “When writing a Python program, especially for data analysis, it is crucial to focus on data structures and algorithms that best suit your data. Utilizing libraries like Pandas and NumPy can greatly improve both performance and ease of use.”
Frequently Asked Questions (FAQs)
What are the basic steps to write a Python program?
To write a Python program, start by installing Python on your system. Then, open a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode. Write your code in the editor, save the file with a `.py` extension, and run the program using the command line or the IDE’s run feature.
How do I execute a Python script?
To execute a Python script, open your command line interface, navigate to the directory containing your script, and type `python script_name.py`, replacing `script_name.py` with your actual file name. Press Enter to run the program.
What is the syntax for defining a function in Python?
In Python, a function is defined using the `def` keyword followed by the function name and parentheses. For example:
“`python
def my_function():
function body
“`
You can include parameters within the parentheses if needed.
How can I handle errors in a Python program?
Error handling in Python is accomplished using `try` and `except` blocks. Place the code that may raise an exception within the `try` block, and handle the exception in the `except` block. This prevents the program from crashing and allows for graceful error management.
What libraries are commonly used in Python programming?
Commonly used libraries in Python include NumPy for numerical computations, Pandas for data manipulation and analysis, Matplotlib for data visualization, and requests for making HTTP requests. Each library serves specific purposes and enhances Python’s functionality.
How do I comment my code in Python?
In Python, comments can be added using the “ symbol for single-line comments. For multi-line comments, use triple quotes (`”’` or `”””`). Comments are ignored by the interpreter and are useful for documenting code.
Writing a Python program involves several key steps that ensure the development process is efficient and effective. Initially, it is crucial to define the problem you aim to solve clearly. This foundational step allows you to outline the program’s requirements and functionalities. Following this, setting up a suitable development environment, including installing Python and any necessary libraries or frameworks, is essential for a smooth coding experience.
Once the environment is ready, the next step is to design the program’s structure. This includes determining the appropriate algorithms and data structures to use, as well as planning the overall flow of the program. Writing clean, well-documented code is vital, as it enhances readability and maintainability. Utilizing version control systems, like Git, can also aid in managing changes and collaborating with others effectively.
After coding, thorough testing is imperative to identify and rectify any bugs or issues. This ensures that the program functions as intended and meets the initial requirements. Finally, once the program is complete, consider deploying it and gathering user feedback for future improvements. This iterative process not only refines the current program but also enhances your skills as a programmer.
Author Profile
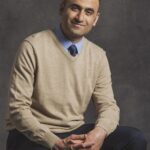
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?