How Can You Write a Python Script from Scratch?
### Introduction
In today’s digital age, the ability to write a Python script is an invaluable skill that opens doors to endless possibilities in programming, data analysis, automation, and more. Python, known for its simplicity and readability, has become one of the most popular programming languages worldwide. Whether you’re a complete novice eager to dip your toes into the world of coding or a seasoned developer looking to expand your toolkit, mastering the art of scripting in Python can empower you to tackle a variety of tasks efficiently. This article will guide you through the essential steps and considerations for writing your very own Python script, setting the stage for your journey into the realm of programming.
Creating a Python script involves more than just typing code; it requires an understanding of the language’s syntax, the logic behind programming concepts, and the specific problem you aim to solve. From defining variables and functions to implementing control flow and handling errors, each element plays a crucial role in crafting a functional script. As you embark on this journey, you’ll discover how to harness Python’s capabilities to automate repetitive tasks, analyze data, or even develop your own applications.
Moreover, the beauty of Python lies in its extensive libraries and frameworks, which can significantly enhance your scripting experience. By leveraging these tools, you can streamline your workflow and focus on
Choosing a Development Environment
Selecting the right development environment is crucial for writing Python scripts efficiently. You can choose between various Integrated Development Environments (IDEs) and text editors, each offering distinct features. Common options include:
- PyCharm: A powerful IDE specifically designed for Python, featuring code analysis, a graphical debugger, and an integrated testing environment.
- Visual Studio Code: A lightweight editor with extensive extensions for Python development, allowing for customization and flexibility.
- Jupyter Notebook: Ideal for data analysis and visualization, enabling you to write and execute Python code in a notebook format.
- Sublime Text: A fast, versatile text editor that supports many programming languages, including Python, with a variety of plugins.
Consider your needs, such as debugging capabilities, project management tools, or support for multiple languages, when choosing your environment.
Writing Your First Script
To create a Python script, follow these steps:
- Open your chosen development environment.
- Create a new file and save it with a `.py` extension (e.g., `my_script.py`).
- Start writing your Python code. Here’s a simple example:
python
print(“Hello, World!”)
This basic script prints a message to the console. To run your script, use the command line or the IDE’s built-in functionality.
Understanding Basic Syntax and Structure
Python’s syntax is designed to be clean and readable, which is one of its primary appeals. Key elements of Python syntax include:
- Comments: Use the `#` symbol to add comments in your code for clarification.
- Variables: Assign values to variables using the equals sign (`=`). For example:
python
name = “Alice”
age = 30
- Data Types: Familiarize yourself with Python’s built-in data types:
- Strings
- Integers
- Floats
- Lists
- Dictionaries
Here’s a brief overview of these data types in table format:
Data Type | Description | Example |
---|---|---|
String | A sequence of characters. | “Hello, World!” |
Integer | A whole number. | 42 |
Float | A number with a decimal point. | 3.14 |
List | An ordered collection of items. | [1, 2, 3] |
Dictionary | A collection of key-value pairs. | {“name”: “Alice”, “age”: 30} |
Understanding these elements allows you to create more complex scripts effectively.
Running Your Script
After writing your script, you need to execute it to see the results. Depending on your environment, you can run your script in several ways:
- Command Line: Navigate to the directory containing your script and type:
python my_script.py
- IDE: Most IDEs offer a “Run” button or menu option that executes the current script.
Make sure you have Python installed and properly configured in your system’s PATH to run scripts from the command line.
Debugging and Error Handling
Debugging is an essential part of the coding process. Python provides various tools for identifying and fixing errors:
- Syntax Errors: These occur when your code violates the language’s rules. Python will provide an error message indicating the type and location of the error.
- Runtime Errors: These occur during execution. Use try-except blocks to handle exceptions gracefully.
Example of error handling:
python
try:
result = 10 / 0
except ZeroDivisionError:
print(“You can’t divide by zero!”)
Utilizing debugging tools in your IDE can help you step through your code and inspect variables at runtime, making it easier to identify issues.
Setting Up Your Environment
To write a Python script, you first need to set up your development environment. This involves selecting the appropriate tools and ensuring Python is installed.
- Install Python: Download the latest version from the [official Python website](https://www.python.org/downloads/). Follow the installation instructions specific to your operating system.
- Choose an IDE or Text Editor: Popular choices include:
- PyCharm: A powerful IDE with robust features for Python development.
- Visual Studio Code: A lightweight editor with extensive plugins and support for Python.
- Sublime Text: Known for its speed and simplicity.
- Set Up Virtual Environments: Use `venv` or `virtualenv` to create isolated environments for your projects, preventing dependency conflicts.
Writing Your First Script
Once your environment is set up, you can start writing your script.
- Create a New File: Use your chosen IDE or text editor to create a new file with a `.py` extension (e.g., `my_script.py`).
- Write Code: Begin with a simple print statement to verify everything is functioning correctly:
python
print(“Hello, World!”)
- Save Your File: Ensure your changes are saved before running the script.
Running Your Script
To execute your Python script, follow these steps:
- Using Command Line:
- Open your terminal or command prompt.
- Navigate to the directory containing your script using the `cd` command.
- Run the script with the command:
bash
python my_script.py
- Using an IDE: Most IDEs have a built-in run feature. Simply click the run button or use the designated keyboard shortcut.
Debugging Your Code
Debugging is an essential aspect of writing scripts. Here are some common techniques:
- Print Statements: Insert print statements to output variable values at different stages of execution.
- Using a Debugger: Most IDEs offer debugging tools that allow you to step through code, inspect variables, and set breakpoints.
- Error Messages: Pay attention to error messages in the console, which can guide you to the source of the problem.
Enhancing Your Script
After successfully running your initial script, consider adding more functionality:
- Functions: Organize your code into reusable functions.
python
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
- Libraries: Import libraries to extend functionality. For instance, use `import math` for mathematical operations or `import requests` for web requests.
Library | Purpose |
---|---|
NumPy | Numerical operations and data handling |
Pandas | Data manipulation and analysis |
Flask | Web development and building web applications |
Best Practices
Adhering to coding standards can enhance the readability and maintainability of your scripts:
- Use Meaningful Variable Names: Choose descriptive names that convey the purpose of the variable.
- Comment Your Code: Add comments to explain complex logic or provide context for future reference.
- Follow PEP 8 Guidelines: Python’s official style guide recommends practices such as consistent indentation and line length limits.
Version Control
Utilizing version control systems like Git is crucial for managing changes and collaborating with others:
- Initialize a Repository: Use `git init` to create a new repository.
- Commit Changes: Stage and commit your changes with:
bash
git add my_script.py
git commit -m “Initial script creation”
- Push to Remote: If using a service like GitHub, link your local repository and push changes with:
bash
git remote add origin
git push -u origin master
Guidance from Python Programming Experts
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To write an effective Python script, one must first clearly define the problem the script is intended to solve. A well-structured plan will guide the coding process and help in maintaining clarity throughout the development.”
Mark Thompson (Lead Python Developer, CodeMaster Solutions). “Utilizing Python’s extensive libraries can significantly enhance the functionality of your script. Familiarity with libraries such as NumPy for numerical computations or Pandas for data manipulation is essential for writing efficient and powerful scripts.”
Sarah Patel (Technical Writer, Python Programming Journal). “Commenting your code and following PEP 8 style guidelines are crucial for readability and maintainability. A well-documented script not only aids others in understanding your code but also serves as a valuable reference for future modifications.”
Frequently Asked Questions (FAQs)
How do I start writing a Python script?
To start writing a Python script, open a text editor or an Integrated Development Environment (IDE) such as PyCharm or Visual Studio Code. Create a new file with a `.py` extension and begin coding using Python syntax.
What are the basic components of a Python script?
A Python script typically includes variables, data types, control structures (like loops and conditionals), functions, and modules. These components work together to perform tasks and execute logic.
How can I run a Python script?
You can run a Python script by opening a terminal or command prompt, navigating to the directory where the script is saved, and executing the command `python script_name.py`, replacing `script_name.py` with your actual file name.
What libraries should I import in my Python script?
The libraries you should import depend on the functionality you need. Common libraries include `os` for operating system interactions, `sys` for system-specific parameters, `math` for mathematical functions, and `requests` for making HTTP requests.
How do I handle errors in a Python script?
You can handle errors in a Python script using try-except blocks. This allows you to catch exceptions and handle them gracefully without crashing the program.
What are some best practices for writing Python scripts?
Best practices include writing clear and concise code, using meaningful variable names, adding comments for clarity, following the PEP 8 style guide, and testing the script thoroughly to ensure functionality.
Writing a Python script involves several key steps that are essential for both beginners and experienced programmers. First, it is crucial to define the purpose of the script, which helps in outlining the necessary functionalities and determining the appropriate libraries or modules to use. Understanding the problem you are trying to solve is foundational to creating an effective script.
Next, setting up the development environment is an important step. This includes installing Python and any required packages, as well as choosing a suitable code editor or integrated development environment (IDE). Familiarity with the tools at your disposal can significantly enhance productivity and streamline the coding process.
Once the environment is ready, writing the script involves structuring the code logically, using clear and concise syntax. It is advisable to incorporate comments to explain complex sections and to follow best practices for code organization, such as using functions and classes where appropriate. Testing the script regularly during development ensures that any issues are identified and resolved promptly.
Finally, after completing the script, it is important to document it thoroughly. This includes providing a README file that explains how to run the script, its dependencies, and any other relevant information. Proper documentation not only aids in future maintenance but also enhances the script’s usability for others who may wish
Author Profile
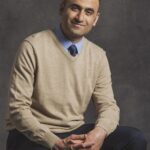
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?