How Do You Write an If Statement in JavaScript?
In the world of programming, decision-making is a fundamental concept that allows developers to create dynamic and responsive applications. One of the most essential tools in a programmer’s toolkit is the “if statement.” In JavaScript, this powerful construct enables you to execute specific blocks of code based on whether certain conditions are met, making your applications more interactive and intelligent. Whether you’re a budding coder or a seasoned developer looking to brush up on your skills, understanding how to effectively use if statements in JavaScript is crucial for crafting robust and efficient code.
At its core, an if statement evaluates a condition and determines the flow of execution based on the result. This simple yet versatile tool can help you manage complex logic, allowing your programs to react differently under various circumstances. From validating user input to controlling the behavior of web applications, mastering if statements opens the door to a world of possibilities in JavaScript programming.
As we delve deeper into this topic, we will explore the syntax and structure of if statements, along with practical examples that illustrate their usage in real-world scenarios. By the end of this article, you will not only grasp the fundamentals of if statements but also be equipped with the knowledge to implement them effectively in your own JavaScript projects. Get ready to enhance your coding skills and bring your applications
Basic Structure of an If Statement
An if statement in JavaScript allows developers to execute a block of code based on a specified condition. The basic syntax is as follows:
javascript
if (condition) {
// code to be executed if condition is true
}
In this structure:
- condition: This is a boolean expression that evaluates to either true or .
- The code block within the curly braces `{}` will only execute if the condition evaluates to true.
Using Else and Else If
To manage multiple conditions, JavaScript provides the `else` and `else if` statements. This allows for more complex decision-making processes. The syntax expands as follows:
javascript
if (condition1) {
// code to be executed if condition1 is true
} else if (condition2) {
// code to be executed if condition2 is true
} else {
// code to be executed if both conditions are
}
Here is a breakdown of the components:
- else if: This allows you to check another condition if the first one is .
- else: This block will execute if none of the previous conditions are met.
Example of an If Statement
Consider a simple example where we check the age of a person to determine if they are eligible to vote:
javascript
let age = 20;
if (age >= 18) {
console.log(“You are eligible to vote.”);
} else {
console.log(“You are not eligible to vote.”);
}
In this example, the `console.log` statement will only execute if the condition `age >= 18` evaluates to true.
Logical Operators in If Statements
Logical operators can be used to combine multiple conditions within an if statement. The most common logical operators are:
– **&& (AND)**: True if both conditions are true.
– **|| (OR)**: True if at least one condition is true.
– **! (NOT)**: Reverses the boolean value of the condition.
Here’s how you might use these operators:
javascript
let age = 25;
let hasID = true;
if (age >= 18 && hasID) {
console.log(“You can vote.”);
} else {
console.log(“You cannot vote.”);
}
Comparing Values
When evaluating conditions, JavaScript provides various comparison operators. Here’s a table summarizing these operators:
Operator | Description | Example |
---|---|---|
== | Equal to | 5 == ‘5’ // true |
=== | Strict equal to | 5 === ‘5’ // |
!= | Not equal to | 5 != ‘5’ // |
!== | Strict not equal to | 5 !== ‘5’ // true |
> | Greater than | 5 > 3 // true |
< | Less than | 5 < 3 // |
>= | Greater than or equal to | 5 >= 5 // true |
<= | Less than or equal to | 5 <= 3 // |
These operators can be utilized within if statements to perform various comparisons and control the flow of the program accordingly.
Basic Syntax of an If Statement
In JavaScript, an if statement is used to execute a block of code based on a specified condition. The basic syntax is as follows:
javascript
if (condition) {
// code to be executed if the condition is true
}
- condition: This is an expression that evaluates to true or .
- code block: This is the set of instructions that runs if the condition is met.
Example of an If Statement
Here is a straightforward example demonstrating the use of an if statement:
javascript
let temperature = 30;
if (temperature > 25) {
console.log(“It’s a hot day.”);
}
In this case, if the `temperature` variable is greater than 25, the message “It’s a hot day.” will be printed to the console.
Using Else and Else If
To handle multiple conditions, you can extend the if statement with else and else if clauses:
javascript
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
} else {
// code if both conditions are
}
**Example**:
javascript
let score = 75;
if (score >= 90) {
console.log(“Grade: A”);
} else if (score >= 80) {
console.log(“Grade: B”);
} else if (score >= 70) {
console.log(“Grade: C”);
} else {
console.log(“Grade: D”);
}
This example categorizes scores into grades based on the specified conditions.
Logical Operators in If Statements
You can combine multiple conditions using logical operators such as AND (`&&`), OR (`||`), and NOT (`!`):
– **AND (`&&`)**: Both conditions must be true.
– **OR (`||`)**: At least one condition must be true.
– **NOT (`!`)**: Reverses the truth value of a condition.
**Example Using Logical Operators**:
javascript
let age = 20;
let hasPermission = true;
if (age >= 18 && hasPermission) {
console.log(“You can enter the club.”);
} else {
console.log(“Entry denied.”);
}
In this instance, the code checks if the user is both of legal age and has permission to enter.
Nested If Statements
If statements can also be nested within one another to check additional conditions:
javascript
if (condition1) {
if (condition2) {
// code if both conditions are true
}
}
**Example of Nested If**:
javascript
let age = 16;
if (age >= 18) {
console.log(“You can vote.”);
} else {
if (age >= 16) {
console.log(“You can drive.”);
} else {
console.log(“You are too young for voting or driving.”);
}
}
This structure allows for multiple layers of decision-making, providing more granularity in control flow.
Switch Statement as an Alternative
For scenarios with multiple discrete conditions, a switch statement can be a more organized alternative:
javascript
switch (expression) {
case value1:
// code if expression matches value1
break;
case value2:
// code if expression matches value2
break;
default:
// code if no cases match
}
Example:
javascript
let day = 3;
switch (day) {
case 1:
console.log(“Monday”);
break;
case 2:
console.log(“Tuesday”);
break;
case 3:
console.log(“Wednesday”);
break;
default:
console.log(“Not a valid day.”);
}
This example uses a switch statement to determine the day of the week based on the `day` variable.
Expert Insights on Writing If Statements in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Writing an if statement in JavaScript is fundamental for controlling the flow of your program. It allows developers to execute specific blocks of code based on certain conditions, enhancing the logic and functionality of applications.”
Michael Chen (JavaScript Educator, Code Academy). “To write an effective if statement in JavaScript, one must understand the syntax clearly: ‘if (condition) { /* code to execute */ }’. This structure is crucial for creating dynamic and responsive web applications.”
Sarah Thompson (Lead Developer, Web Solutions Group). “Utilizing if statements effectively can significantly improve your code’s readability and maintainability. Always consider edge cases and ensure your conditions are well-defined to avoid unexpected behaviors.”
Frequently Asked Questions (FAQs)
How do I write a basic if statement in JavaScript?
To write a basic if statement in JavaScript, use the following syntax:
javascript
if (condition) {
// code to execute if condition is true
}
Replace `condition` with a boolean expression.
Can I include an else clause with my if statement?
Yes, you can include an else clause to execute code when the condition is . The syntax is as follows:
javascript
if (condition) {
// code if condition is true
} else {
// code if condition is
}
Is it possible to chain multiple if statements?
Yes, you can chain multiple if statements using else if. The syntax is:
javascript
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
} else {
// code if both conditions are
}
What types of conditions can I use in an if statement?
You can use various types of conditions, including comparisons (e.g., `==`, `===`, `!=`, `!==`, `<`, `>`, `<=`, `>=`), logical operators (e.g., `&&`, `||`), and function returns that yield boolean values.
Are there shorthand ways to write if statements in JavaScript?
Yes, you can use the ternary operator as a shorthand for simple if statements:
javascript
condition ? expressionIfTrue : expressionIf;
This allows for concise conditional assignments or expressions.
What happens if the condition in an if statement is not met?
If the condition is not met (evaluates to ), the code block within the if statement will not execute. If there is an else clause, the code within that block will execute instead.
In JavaScript, an if statement is a fundamental control structure that allows developers to execute a block of code based on a specified condition. The basic syntax involves the keyword “if,” followed by a condition enclosed in parentheses, and a block of code enclosed in curly braces. If the condition evaluates to true, the code within the braces is executed; otherwise, it is skipped. This structure enables developers to implement decision-making capabilities in their scripts, enhancing the interactivity and functionality of web applications.
Moreover, JavaScript supports various extensions of the if statement, such as the else and else if constructs. The else statement provides an alternative block of code to execute when the initial condition is , while else if allows for additional conditions to be evaluated sequentially. This flexibility enables developers to create complex conditional logic, accommodating a wide range of scenarios and user inputs within their applications.
In summary, mastering the if statement and its variations is crucial for any JavaScript developer. It forms the backbone of control flow in programming, allowing for responsive and dynamic coding practices. By effectively utilizing if statements, developers can enhance their applications’ logic and user experience, making it an essential skill in the toolkit of modern web development.
Author Profile
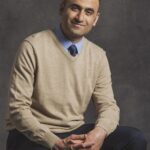
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?