How Can You Effectively Write Matrices in Python?
In the world of programming, matrices play a pivotal role in various fields, from data science and machine learning to computer graphics and engineering simulations. As a fundamental data structure, they allow for the efficient representation and manipulation of numerical data. If you’re venturing into Python, one of the most versatile and widely-used programming languages, understanding how to write and work with matrices is essential. This article will guide you through the various approaches to creating and handling matrices in Python, empowering you to harness their full potential in your projects.
Matrices in Python can be represented in several ways, each with its own advantages and use cases. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to optimize your matrix operations, Python offers a rich ecosystem of libraries and tools to facilitate your work. From simple nested lists to powerful libraries like NumPy, the options are abundant and can cater to different needs, such as performance, ease of use, and functionality.
As we delve deeper into the topic, we’ll explore the various methods for creating matrices, the benefits of using specialized libraries, and practical examples that illustrate how to manipulate matrices effectively. By the end of this article, you’ll be equipped with the knowledge and skills to confidently write and utilize matrices in your Python applications, opening doors to
Using Lists to Create Matrices
In Python, one of the simplest ways to represent a matrix is by using lists. A matrix can be represented as a list of lists, where each inner list corresponds to a row in the matrix. For example, a 2×3 matrix can be created as follows:
“`python
matrix = [
[1, 2, 3],
[4, 5, 6]
]
“`
This representation allows you to easily access individual elements using their row and column indices. For instance, `matrix[1][2]` would return `6`, the element at the second row and third column.
Using NumPy for Matrix Operations
NumPy is a powerful library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. To use NumPy for creating matrices, you first need to install the library if it is not already available:
“`bash
pip install numpy
“`
Once installed, you can create matrices using the `numpy.array()` function:
“`python
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6]])
“`
Creating Identity Matrices
An identity matrix is a square matrix with ones on the diagonal and zeros elsewhere. NumPy provides a convenient function to create identity matrices:
“`python
identity_matrix = np.eye(3)
“`
This code creates a 3×3 identity matrix. The resulting matrix looks like this:
Column 0 | Column 1 | Column 2 |
---|---|---|
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 1 |
Matrix Operations with NumPy
NumPy provides various functions to perform operations on matrices, such as addition, subtraction, and multiplication. Here are some examples:
- Matrix Addition:
“`python
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
result = matrix1 + matrix2
“`
- Matrix Multiplication:
“`python
result = np.dot(matrix1, matrix2)
“`
- Element-wise Multiplication:
“`python
result = matrix1 * matrix2
“`
These operations are efficiently handled by NumPy, making it a preferred choice for mathematical computations involving matrices.
In summary, whether you choose to use simple lists or leverage the capabilities of libraries like NumPy, Python provides flexible options for working with matrices. Understanding these methods will help you effectively manipulate and perform calculations on matrix data.
Using Lists to Represent Matrices
One of the simplest ways to create a matrix in Python is by using nested lists. A matrix can be represented as a list of lists, where each inner list represents a row of the matrix.
“`python
Creating a 2×3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6]
]
“`
This approach allows you to easily manipulate the matrix by accessing its elements through indexing.
Using NumPy for Matrix Operations
For more advanced operations and functionalities, the NumPy library is highly recommended. NumPy provides a dedicated array object that simplifies matrix creation and manipulation.
- Installation: If you haven’t installed NumPy yet, you can do so using pip:
“`bash
pip install numpy
“`
- Creating a Matrix: You can create matrices using `numpy.array()`:
“`python
import numpy as np
Creating a 2×3 matrix
matrix_np = np.array([[1, 2, 3], [4, 5, 6]])
“`
- Matrix Operations: NumPy allows for easy arithmetic and linear algebra operations:
“`python
Transpose of a matrix
transpose = matrix_np.T
Matrix addition
matrix2 = np.array([[7, 8, 9], [10, 11, 12]])
sum_matrix = matrix_np + matrix2
Matrix multiplication
product_matrix = np.dot(matrix_np, matrix2.T)
“`
Using Pandas for DataFrames as Matrices
Another powerful library is Pandas, which is primarily used for data manipulation and analysis. It allows you to work with DataFrames that can also represent matrices.
- Installation: Install Pandas via pip if it’s not already installed:
“`bash
pip install pandas
“`
- Creating a DataFrame: You can create a DataFrame using `pandas.DataFrame()`:
“`python
import pandas as pd
Creating a DataFrame
data = [[1, 2, 3], [4, 5, 6]]
df = pd.DataFrame(data, columns=[‘Column1’, ‘Column2’, ‘Column3’])
“`
- Accessing Data: You can easily access rows and columns:
“`python
Accessing a specific element
element = df.iloc[1, 2] Accessing element in 2nd row, 3rd column
Accessing an entire column
column_data = df[‘Column1’]
“`
Visualizing Matrices with Matplotlib
When working with matrices, visual representation can help in understanding the data. Matplotlib is a popular library for plotting in Python.
- Installation: Install Matplotlib if you haven’t yet:
“`bash
pip install matplotlib
“`
- Visualizing a Matrix: You can visualize a matrix using `imshow()`:
“`python
import matplotlib.pyplot as plt
Creating a matrix
matrix_to_plot = np.array([[1, 2, 3], [4, 5, 6]])
Plotting the matrix
plt.imshow(matrix_to_plot, cmap=’viridis’, interpolation=’none’)
plt.colorbar()
plt.show()
“`
This will display the matrix as an image, with color representing the values within the matrix, enhancing the understanding of its structure and values.
Expert Insights on Writing Matrices in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When writing matrices in Python, utilizing libraries such as NumPy is essential. NumPy provides a powerful array object that allows for efficient storage and manipulation of matrix data, making operations like addition, multiplication, and inversion straightforward and optimized for performance.”
Michael Chen (Software Engineer, AI Solutions Group). “For beginners, I recommend starting with nested lists to represent matrices. However, as you progress, transitioning to NumPy arrays will enhance your ability to perform complex mathematical operations with ease and speed, as well as improve code readability.”
Sarah Thompson (Mathematics Educator, Online Learning Academy). “Understanding how to write matrices in Python is crucial for anyone venturing into data analysis or machine learning. I suggest practicing matrix operations using both NumPy and pure Python to grasp the underlying concepts before relying solely on libraries.”
Frequently Asked Questions (FAQs)
How do I create a matrix in Python?
You can create a matrix in Python using nested lists or by utilizing libraries such as NumPy. For example, a 2D matrix can be created with `matrix = [[1, 2], [3, 4]]` or with NumPy using `import numpy as np; matrix = np.array([[1, 2], [3, 4]])`.
What library is best for matrix operations in Python?
NumPy is the most widely used library for matrix operations in Python. It provides efficient array handling and a comprehensive set of functions for mathematical operations on matrices.
How can I perform matrix multiplication in Python?
Matrix multiplication can be performed using the `@` operator in NumPy. For example, if `A` and `B` are two matrices, you can multiply them with `C = A @ B`. Alternatively, you can use the `np.dot(A, B)` function.
Can I create sparse matrices in Python?
Yes, you can create sparse matrices using the SciPy library. The `scipy.sparse` module provides various classes such as `csr_matrix` and `csc_matrix` to efficiently store and manipulate sparse data.
How do I access elements in a matrix in Python?
Elements in a matrix can be accessed using indexing. For example, in a nested list matrix `matrix`, you can access the element in the first row and second column with `matrix[0][1]`. In a NumPy array, you would use `matrix[0, 1]`.
What functions are available for matrix manipulation in NumPy?
NumPy offers a variety of functions for matrix manipulation, including `np.transpose()` for transposing, `np.linalg.inv()` for finding inverses, `np.linalg.det()` for determinants, and `np.linalg.eig()` for eigenvalues and eigenvectors.
In Python, writing and manipulating matrices can be accomplished using various methods, with the most common approaches involving lists, the NumPy library, or the use of libraries such as pandas. Each method offers unique advantages, depending on the complexity of the tasks and the size of the data being handled. For simple matrices, nested lists provide a straightforward way to represent and access matrix elements. However, for more advanced operations and larger datasets, utilizing NumPy is highly recommended due to its efficiency and extensive functionality.
NumPy allows for the creation of matrices through its array structure, enabling users to perform mathematical operations seamlessly. Additionally, it provides a wide range of built-in functions for matrix manipulation, such as addition, multiplication, and transposition, which significantly simplifies the coding process. For users working with data frames, pandas offers another layer of abstraction, making it easier to manage and analyze matrix-like data structures.
the choice of method for writing matrices in Python largely depends on the specific requirements of the project. For beginners or those dealing with small datasets, lists may suffice. However, for those requiring more robust capabilities, investing time in learning NumPy or pandas will yield greater benefits in terms of performance and functionality. Understanding these tools will enhance
Author Profile
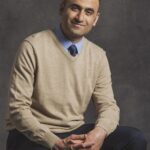
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?