How Can You Write a New Line in a Text File Using Python?
When working with text files in Python, one of the most fundamental tasks you’ll encounter is writing data in a structured and readable format. Among the various operations you can perform, inserting new lines is crucial for organizing your content effectively. Whether you’re logging information, generating reports, or simply saving user inputs, knowing how to create new lines in a text file can significantly enhance the clarity and usability of your output.
In Python, the process of writing to a text file is straightforward, thanks to its built-in file handling capabilities. However, many beginners often overlook the importance of formatting their text properly. New lines play a vital role in ensuring that each piece of information is presented clearly, making it easier for readers to digest the content. This article will delve into the various methods you can use to insert new lines when writing to a text file, ensuring that your data is not only saved but also well-organized.
As we explore the different techniques for adding new lines in text files, you’ll discover how simple commands can make a significant difference in the readability of your data. From using escape characters to leveraging built-in functions, there are multiple approaches to achieve your desired formatting. By mastering these methods, you’ll be well-equipped to handle any text-based project with confidence and precision.
Writing a New Line in a Text File
To write a new line in a text file using Python, you can utilize the built-in `open()` function combined with the file method `write()` or `writelines()`. Each method allows for the inclusion of newline characters to separate lines effectively.
When you want to insert a new line, you can do so by using the newline character `\n`. Here are the methods commonly used for writing to a text file:
- Using the `write()` Method: This method writes a string to the file. To add a new line, append `\n` at the end of the string you are writing.
- Using the `writelines()` Method: This method takes a list of strings and writes them to the file. Each string in the list should include the newline character if you want to separate them.
Here is an example demonstrating both methods:
“`python
Using write()
with open(‘example.txt’, ‘w’) as file:
file.write(‘First line\n’)
file.write(‘Second line\n’)
Using writelines()
lines = [‘First line\n’, ‘Second line\n’, ‘Third line\n’]
with open(‘example.txt’, ‘w’) as file:
file.writelines(lines)
“`
File Modes
Choosing the correct file mode is crucial when writing to files. The most common modes are:
Mode | Description |
---|---|
`w` | Write mode. Creates a new file or truncates an existing file. |
`a` | Append mode. Adds new content to the end of the file without truncating it. |
`r+` | Read and write mode. Allows both reading and writing. |
When writing new lines, especially in append mode, you should ensure you do not accidentally overwrite existing content.
Handling New Lines Across Operating Systems
It’s important to note that newline characters can differ across operating systems:
- Unix/Linux: Uses `\n` for new lines.
- Windows: Uses `\r\n` for new lines.
- Mac (old versions): Used `\r`, but modern systems use `\n`.
To ensure compatibility across different systems, you can use Python’s built-in `os` module to determine the correct newline character:
“`python
import os
newline_char = os.linesep
with open(‘example.txt’, ‘w’) as file:
file.write(f’First line{newline_char}’)
file.write(f’Second line{newline_char}’)
“`
Utilizing `os.linesep` will automatically adapt to the operating system’s newline convention, ensuring your file is formatted correctly regardless of where it is opened.
Writing a New Line in a Text File
In Python, writing a new line in a text file can be achieved using various methods. The newline character is represented by `\n`. When you want to write to a file, you typically open the file in write (`’w’`) or append (`’a’`) mode.
Using the `write()` Method
The `write()` method allows you to write strings to a file. To include a new line, you can manually add `\n` at the end of your string.
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(“First line\n”)
file.write(“Second line\n”)
“`
In the example above:
- The first line is followed by `\n`, which creates a new line before the second line.
Using the `writelines()` Method
The `writelines()` method can be used to write multiple lines at once. Each string in the list should already contain a newline character if you want them to appear on separate lines.
“`python
lines = [“First line\n”, “Second line\n”, “Third line\n”]
with open(‘example.txt’, ‘w’) as file:
file.writelines(lines)
“`
This method is efficient when dealing with multiple lines, as it reduces the number of write calls.
Appending to an Existing File
To add new content to an existing file without overwriting it, use append mode (`’a’`). This is particularly useful when you need to log information or add entries.
“`python
with open(‘example.txt’, ‘a’) as file:
file.write(“Fourth line\n”)
“`
Using append mode ensures that the new line is added at the end of the file.
Writing with Automatic Newlines
Python also provides a way to handle new lines automatically. When opening a file in text mode, you can specify the newline behavior using the `newline` parameter.
“`python
with open(‘example.txt’, ‘w’, newline=”) as file:
file.write(“First line\nSecond line\n”)
“`
The `newline=”` setting prevents Python from translating `\n` into the platform-specific line ending.
Considerations for Different Operating Systems
Different operating systems may handle line endings differently:
- Windows uses `\r\n`.
- Unix/Linux uses `\n`.
- Mac (pre-OS X) uses `\r`.
When writing files that will be used across different systems, it’s advisable to standardize line endings.
Example: Writing Multiple Lines with a Loop
You can also write multiple lines using a loop, which is particularly useful for dynamically generated content.
“`python
lines = [“Line 1”, “Line 2”, “Line 3″]
with open(‘example.txt’, ‘w’) as file:
for line in lines:
file.write(f”{line}\n”)
“`
This approach ensures that each entry is properly formatted with a new line.
Summary of Methods
Method | Description |
---|---|
`write()` | Writes a single string to the file. |
`writelines()` | Writes a list of strings to the file. |
Append mode | Adds content without overwriting. |
Automatic newlines | Handles line endings based on system. |
By utilizing these methods effectively, you can manage file writing operations in Python with precision and ease.
Expert Insights on Writing New Lines in Text Files with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When writing new lines in text files using Python, it is crucial to utilize the newline character, typically represented as `\n`. This ensures that each entry in your file is properly separated, enhancing readability and organization.”
Michael Chen (Python Developer, Data Solutions Co.). “Using the `with open()` statement is a best practice when writing to files in Python. This not only simplifies the code but also automatically handles file closure, which is essential for preventing data loss, especially when inserting new lines.”
Sarah Thompson (Lead Data Scientist, Analytics Group). “For multi-line strings, employing triple quotes can be very effective. This allows you to write extensive text blocks directly into your file, including new lines, without needing to manually insert `\n` after each line.”
Frequently Asked Questions (FAQs)
How do I write a new line in a text file using Python?
To write a new line in a text file, use the newline character `\n` within the string you are writing. For example, `file.write(“Hello World\n”)` will write “Hello World” followed by a new line.
What mode should I use to write to a text file in Python?
Use the `’w’` mode to write to a text file. This mode will create a new file or overwrite an existing file. If you want to append to an existing file, use the `’a’` mode.
Can I write multiple lines at once in a text file?
Yes, you can write multiple lines at once by using the `writelines()` method. Pass a list of strings, each ending with a newline character, to `file.writelines()`, for example, `file.writelines([“Line 1\n”, “Line 2\n”])`.
How do I ensure that the file is properly closed after writing?
Use a `with` statement when opening the file. This automatically closes the file when the block is exited, ensuring that resources are released properly. For example:
“`python
with open(‘file.txt’, ‘w’) as file:
file.write(“Hello World\n”)
“`
What if I want to write a new line without adding extra spaces?
To write a new line without adding extra spaces, simply include `\n` at the end of your string. Avoid adding additional spaces before or after the newline character.
Is there a way to write a new line in a text file without using `\n`?
Yes, you can use the `os.linesep` constant from the `os` module, which provides the appropriate line separator for the operating system. For example:
“`python
import os
file.write(“Hello World” + os.linesep)
“`
In Python, writing a new line in a text file can be accomplished using various methods, primarily through the built-in `open()` function combined with the `write()` method. When opening a file in write mode (‘w’) or append mode (‘a’), developers can insert newline characters (`\n`) to create line breaks. This allows for structured data entry and formatting within text files, which is crucial for readability and organization.
Additionally, Python’s `print()` function can also be utilized to write to a file by specifying the file parameter. By default, `print()` adds a newline after each call, making it a convenient option for writing multiple lines. Understanding these methods is essential for effective file manipulation in Python, particularly when dealing with large datasets or generating reports.
Key takeaways include the importance of choosing the appropriate file mode based on the desired outcome—whether to overwrite existing content or append to it. Moreover, recognizing the significance of newline characters in maintaining the structure of the text file can enhance the clarity of the output. Overall, mastering these techniques enables developers to efficiently manage text file operations in their Python applications.
Author Profile
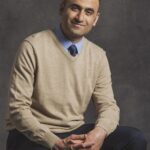
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?