How Can You Write Pi in Python? A Step-by-Step Guide
Introduction
In the world of programming, few constants are as celebrated and universally recognized as the mathematical symbol π (pi). This enigmatic number, approximately equal to 3.14159, represents the ratio of a circle’s circumference to its diameter and has fascinated mathematicians, scientists, and engineers for centuries. As a Python enthusiast or a budding programmer, understanding how to work with pi can open up a realm of possibilities, from simple calculations to complex simulations. Whether you’re plotting graphs, performing geometric calculations, or delving into numerical analysis, knowing how to effectively incorporate pi into your Python code is essential.
In Python, there are several straightforward methods to represent and utilize pi, making it accessible for both novices and seasoned developers. The language’s built-in libraries provide an easy way to access this mathematical constant, allowing you to focus on your creative coding endeavors rather than getting bogged down in the intricacies of numerical representation. Furthermore, Python’s versatility means that you can seamlessly integrate pi into various applications, from data visualization to algorithm development.
As we explore the different ways to write and use pi in Python, you’ll discover not only the technical steps but also the broader implications of incorporating this fundamental constant into your projects. Whether you’re calculating areas, working with trigonometric functions
Using the math Module
To represent the mathematical constant pi in Python, the most straightforward approach is to use the built-in `math` module. This module provides a constant `math.pi`, which is a floating-point representation of pi.
Here’s how you can use it:
python
import math
# Accessing the value of pi
pi_value = math.pi
print(pi_value) # Output: 3.141592653589793
This method is highly recommended for its simplicity and accuracy. The value of `math.pi` is defined to a high degree of precision, making it suitable for most mathematical computations involving pi.
Using the Decimal Module for Higher Precision
In scenarios where higher precision is required, such as in financial calculations or when dealing with very small numbers, the `decimal` module can be utilized. This module allows you to define the precision needed for your calculations.
Here’s an example of how to represent pi using the `decimal` module:
python
from decimal import Decimal, getcontext
# Set the precision
getcontext().prec = 50 # Set precision to 50 decimal places
# Define pi with high precision
pi_value = Decimal(‘3.141592653589793238462643383279502884197169399375105820974944592307816406286208998628034825342117067982148086513282306647093844609550582231725359408128481117450284102701938521105559644622948954930381964428810975665933446128475648233786783165271201909145648566923460348610454326648213393607260249141273724587006606315588174881520920962829254091715364367892590360011330530548820466521384146951941511609433057270365759591953092186117381932611793105118548074462379962749567351885752724891227938183011949128831426076796116130906907596116173163691176680164317698425055026427918872765659451737558492813558503352217108208919407111788756428184162426745469095330509834746574136493657192577961034275084658180438644471424383993438164002224715683649708417546609064497304864020054574908644138824198305345248026793800745721135988615134659336402012646326946295669918328198509888807835568280347409030908016782137803205642560318537210080030547905127506545765639804210436697740928108757882694074386046618580195664211268287679908725476397559713239800020825977460379792327694646888774059202263858528199547688034206055933015620399343166973886918983086602514440658946091665050982754807031221141698217569869280234250016828253632820014325010142066164367320317800156636276639482473317225766918157545282586578016995569379725680998020382551860564517588272258283200949424472506292569470536865104340068811386688817282303500202502151198354292891274077477559869331858135128020671285667022055639764913347707552259747924516914063924790151645539052082973155530950206454228147679313401136118754846943565180484460814597434489129511109503315563292535868634568267304566407679327803377420688064901105771728613689490682743113406530822066038045053501814611934365268663670278671693725254174093731032161014144988588726530537212879569038643204450157630218196626329812064167548353783905925905128735651150619634903920292399241363377396020654368464805295263362674203865496317181045557439581473733027837801406015731322713981270581828017329440255810341348149507264882690914588081357819199512086477244584832811124928681470758117027949633748913046285070634641307151032211676873515739753485279116445879448097924336334469457664682535919082049956772958017981026043703601093244328787990268843798160864130613703220119743892237492024510032132697993586611329244596525310372175949626424848451876136288130490840991618965104728981100669150930491407017130830908624117967665335829046679576183100679789136007212235154170784250082553368637148547079294467006503826052534458725853437734708324242176716300040753796869370150888905926434566586748887702918812102364448061046543780302948067595215203469950597879189036018455602377084315327259981671115246824172582266314771094594387719271889399982365018555766160592899620787453258447018949261914981125034335504272615736969186695282760565271631513657032000038088453690075300224803344931716740100070864672546585289151279508655369816516224882838535931930196996745283469996783963037972057016575080962126999581216057913803032472800054813704626608611038327874426597907780314452568239436157555659813988888
Using the `math` Module
Python offers a built-in module called `math`, which provides a constant for π (pi). To access this constant, you can simply import the module and use `math.pi`.
python
import math
print(math.pi) # Output: 3.141592653589793
This method is straightforward and efficient for most applications requiring the value of pi.
Calculating Pi Using Series
If you need a specific precision or wish to calculate π programmatically, you can use series expansions. One common series is the Leibniz formula for π:
\[ \pi = 4 \cdot \sum_{n=0}^{\infty} \frac{(-1)^n}{2n + 1} \]
Here’s how you can implement this in Python:
python
def calculate_pi_leibniz(terms):
pi = 0
for n in range(terms):
pi += ((-1) ** n) / (2 * n + 1)
return 4 * pi
print(calculate_pi_leibniz(100000)) # More terms yield a more accurate result
Using NumPy for Higher Precision
For scientific computations requiring higher precision, consider using the NumPy library, which also provides π. The constant can be accessed as follows:
python
import numpy as np
print(np.pi) # Output: 3.141592653589793
NumPy can also be used for calculations involving arrays and matrix operations, making it suitable for more complex applications.
Custom Pi Calculation with Gauss-Legendre Algorithm
Another method to compute π is the Gauss-Legendre algorithm, known for its rapid convergence. The algorithm follows these steps:
- Initialize variables:
- \( a_0 = 1 \)
- \( b_0 = \frac{1}{\sqrt{2}} \)
- \( t_0 = \frac{1}{4} \)
- \( p_0 = 1 \)
- Repeat the following until convergence:
- Compute \( a_{n+1} = \frac{a_n + b_n}{2} \)
- Compute \( b_{n+1} = \sqrt{a_n \cdot b_n} \)
- Compute \( t_{n+1} = t_n – p_n \cdot (a_n – a_{n+1})^2 \)
- Update \( p_{n+1} = 2 \cdot p_n \)
- Calculate π using:
\[ \pi \approx \frac{(a_n + b_n)^2}{4 \cdot t_n} \]
Here’s how to implement the Gauss-Legendre algorithm in Python:
python
import math
def calculate_pi_gauss_legendre(iterations):
a = 1
b = 1 / math.sqrt(2)
t = 1 / 4
p = 1
for _ in range(iterations):
a_next = (a + b) / 2
b = math.sqrt(a * b)
t -= p * (a – a_next) ** 2
a = a_next
p *= 2
return (a + b) ** 2 / (4 * t)
print(calculate_pi_gauss_legendre(10)) # Higher iterations increase accuracy
Using Decimal for Arbitrary Precision
For applications requiring arbitrary precision, the `decimal` module can be employed. This module allows for precise decimal arithmetic.
python
from decimal import Decimal, getcontext
getcontext().prec = 50 # Set precision to 50 decimal places
def calculate_pi_decimal():
C = 426880 * Decimal(10005).sqrt()
M = Decimal(1)
L = Decimal(13591409)
X = Decimal(1)
K = Decimal(6)
S = L
for n in range(1, 100): # Adjust iterations for desired precision
M = (K3 – 16*K) * M / n3
L += 545140134
X *= -262537412640768000
S += M * L / X
K += 12
return C / S
print(calculate_pi_decimal()) # Output will be precise to the specified decimal places
Expert Insights on Writing Pi in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “To write the value of pi in Python, one can utilize the built-in `math` library, which provides a constant `math.pi`. This method is not only efficient but also ensures precision in calculations involving pi.”
Michael Tran (Python Software Engineer, CodeCraft Solutions). “For those looking to represent pi with a custom precision, using the `decimal` module allows for greater control over the number of decimal places. This is particularly useful in scientific computations where accuracy is paramount.”
Sarah Jenkins (Computer Science Educator, Future Coders Academy). “When teaching how to write pi in Python, I emphasize the importance of understanding both the mathematical significance of pi and the various ways to represent it in code. Using `math.pi` is straightforward, but creating a function to approximate pi, such as through the Monte Carlo method, can greatly enhance a student’s comprehension of programming and mathematics.”
Frequently Asked Questions (FAQs)
How can I define the value of pi in Python?
You can define the value of pi in Python using the `math` module. Import the module and use `math.pi` to access the value of pi, which is approximately 3.14159.
What is the best way to use pi in mathematical calculations in Python?
To use pi in mathematical calculations, import the `math` module and utilize `math.pi` in your formulas. This ensures precision in calculations involving circles, trigonometric functions, and other mathematical operations.
Can I calculate pi to more decimal places in Python?
Yes, you can calculate pi to more decimal places using libraries like `mpmath` or `decimal`. The `mpmath` library allows for arbitrary-precision arithmetic, enabling you to compute pi to thousands of decimal places.
Is there a way to approximate pi using a formula in Python?
Yes, you can approximate pi using various mathematical formulas, such as the Leibniz formula or the Monte Carlo method. Implementing these algorithms in Python can yield an approximation of pi based on iterations or random sampling.
How do I display pi in a formatted string in Python?
You can display pi in a formatted string using Python’s f-string syntax or the `format()` method. For example, `f”{math.pi:.5f}”` will format pi to five decimal places.
Are there any libraries that provide additional functionalities related to pi in Python?
Yes, libraries such as `numpy` and `scipy` provide additional functionalities related to pi, including advanced mathematical functions and numerical computations that utilize pi in various scientific and engineering applications.
In Python, the mathematical constant pi can be represented using the built-in `math` module, which provides a precise value for pi as `math.pi`. This approach is straightforward and ensures accuracy in calculations involving pi. Additionally, for scenarios requiring a custom representation of pi, one can define it manually using a floating-point number, although this is less common due to the availability of the `math` module.
Furthermore, for more advanced applications, such as simulations or graphical representations, libraries like NumPy also provide access to pi through `numpy.pi`. This can be particularly beneficial in scientific computing or when working with large datasets, as it integrates seamlessly with other numerical operations.
Overall, the most efficient and accurate way to utilize pi in Python is to leverage the `math` or `numpy` libraries. This not only simplifies the code but also enhances its readability and maintainability. Understanding these methods allows developers to effectively incorporate pi into their mathematical computations and algorithms.
Author Profile
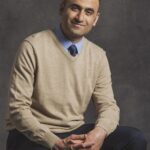
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?