How Can You Write Effective Python Scripts: A Step-by-Step Guide?
In today’s digital age, the ability to write Python scripts is an invaluable skill that opens doors to a world of possibilities. Whether you’re a budding programmer, a data analyst, or simply someone looking to automate mundane tasks, Python’s simplicity and versatility make it an ideal choice for both beginners and seasoned developers alike. With its clear syntax and extensive libraries, Python empowers you to bring your ideas to life, streamline workflows, and solve complex problems with ease. If you’ve ever wondered how to harness the power of Python scripting, you’re in the right place.
Writing Python scripts involves more than just knowing the syntax; it’s about understanding the logic and structure that underpin effective programming. At its core, a Python script is a collection of commands that the Python interpreter executes to perform a specific task. From automating repetitive processes to developing web applications, the potential applications are vast. This article will guide you through the essential concepts, tools, and best practices that will set you on the path to becoming proficient in Python scripting.
As you embark on this journey, you’ll discover the importance of planning your script, selecting the right libraries, and debugging your code. Each step in the process is crucial to creating efficient and functional scripts that can tackle real-world challenges. By the end of
Understanding Python Syntax
To effectively write Python scripts, it is crucial to have a strong grasp of Python syntax, which dictates how code is structured and written. Python emphasizes readability and simplicity, making it an ideal language for both beginners and experienced programmers. Key components of Python syntax include:
- Indentation: Python uses indentation to define blocks of code. Consistent use of spaces or tabs is essential.
- Comments: Use “ for single-line comments and triple quotes (`”’` or `”””`) for multi-line comments to enhance code clarity.
- Variables: Variables in Python are created by simply assigning a value to a name. Python uses dynamic typing, meaning you do not need to declare variable types explicitly.
Writing Your First Script
Creating a Python script is straightforward. Begin with a text editor or an Integrated Development Environment (IDE) like PyCharm or Visual Studio Code. Follow these steps to write your first Python script:
- Open your chosen text editor or IDE.
- Create a new file and save it with a `.py` extension.
- Write a simple line of code. For example:
“`python
print(“Hello, World!”)
“`
- Save the file.
To execute the script, open a terminal or command prompt, navigate to the directory containing your script, and run:
“`bash
python your_script_name.py
“`
Basic Python Constructs
Python scripts often use various constructs to manage control flow, data storage, and functionality. Below are essential constructs:
- Control Structures: Use `if`, `elif`, and `else` statements for conditional logic. Loops can be implemented with `for` and `while`.
- Functions: Define reusable blocks of code using the `def` keyword. Example:
“`python
def greet(name):
return f”Hello, {name}!”
“`
- Data Structures: Familiarize yourself with lists, dictionaries, sets, and tuples, as they are fundamental in data management.
Data Structure | Characteristics | Example |
---|---|---|
List | Ordered, mutable | [1, 2, 3] |
Tuple | Ordered, immutable | (1, 2, 3) |
Dictionary | Unordered, mutable, key-value pairs | {“name”: “Alice”, “age”: 30} |
Set | Unordered, mutable, unique elements | {1, 2, 3} |
Importing Modules and Libraries
Python supports modular programming through the use of modules and libraries. You can import existing libraries or create your own. To import a module, use the `import` statement. For example:
“`python
import math
print(math.sqrt(16)) Outputs: 4.0
“`
Additionally, third-party libraries can be installed using package managers like `pip`. For instance, to install the requests library, execute:
“`bash
pip install requests
“`
Utilizing libraries can significantly enhance your scripts by adding functionality without needing to write everything from scratch.
Debugging and Testing
Debugging is an essential part of writing Python scripts. Use built-in functions like `print()` to output variable states or the `pdb` module for step-by-step debugging. Here are some strategies for effective debugging:
- Print Statements: Use `print()` to check variable values at various stages.
- Error Messages: Pay attention to error messages; they often indicate the line number and nature of the issue.
- Unit Testing: Implement unit tests using the `unittest` framework to validate the functionality of your code.
By following these guidelines and continuously practicing, you will develop proficiency in writing effective Python scripts.
Understanding Python Syntax
Python is known for its clear and readable syntax, which is crucial for writing effective scripts. Key elements of Python syntax include:
- Indentation: Python uses indentation to define the structure and flow of the code, such as defining loops and functions. Consistency is essential; use either spaces or tabs, but not both.
- Comments: Use the “ symbol to add comments in your code, which can enhance readability and provide context for future reference.
- Variables: Declare variables without explicit type declaration. For example:
“`python
name = “Alice”
age = 30
“`
Writing Your First Script
To create a basic Python script, follow these steps:
- Install Python: Ensure you have Python installed on your system. You can download it from the official Python website.
- Choose an Editor: Use a code editor or an Integrated Development Environment (IDE) like PyCharm, VSCode, or Jupyter Notebook.
- Create a New File: Name your script with a `.py` extension, such as `my_script.py`.
- Write Code: Start typing your Python code. For example, a simple “Hello, World!” script looks like this:
“`python
print(“Hello, World!”)
“`
- Run the Script: Execute your script via the command line:
“`bash
python my_script.py
“`
Utilizing Libraries and Modules
Python’s extensive libraries can enhance your scripts significantly.
- Importing Modules: Use the `import` statement to include libraries. For example:
“`python
import math
print(math.sqrt(16))
“`
- Using Pip: Install third-party libraries using pip. For instance:
“`bash
pip install requests
“`
Debugging Your Scripts
Debugging is essential for successful Python scripting. Common techniques include:
- Print Statements: Use `print()` to output variable values and trace execution flow.
- Python Debugger (pdb): Utilize the built-in debugger by adding `import pdb; pdb.set_trace()` in your code to step through it.
- Integrated Debugging Tools: Most IDEs come with built-in debugging features that allow you to set breakpoints and inspect variables.
Structuring Your Code
Good structure improves maintainability. Consider the following practices:
- Functions: Break your code into reusable functions to encapsulate behavior.
“`python
def greet(name):
print(f”Hello, {name}!”)
“`
- Modules: Organize related functions into modules for better code organization.
- Documentation: Use docstrings to document functions and modules. For example:
“`python
def add(a, b):
“””Return the sum of a and b.”””
return a + b
“`
Best Practices for Python Scripts
Adhering to best practices ensures your scripts are efficient and maintainable:
- Follow PEP 8: Adhere to the Python Enhancement Proposal 8 style guide for clean, readable code.
- Error Handling: Use `try` and `except` blocks to handle exceptions gracefully.
“`python
try:
risky_operation()
except Exception as e:
print(f”An error occurred: {e}”)
“`
- Version Control: Utilize Git for version control to track changes and collaborate effectively.
Implement these practices and techniques to enhance your Python scripting skills, leading to more robust and maintainable code.
Expert Guidance on Writing Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When writing Python scripts, clarity and simplicity should be your primary focus. Utilize meaningful variable names and modular functions to enhance readability and maintainability. This approach not only aids in debugging but also makes collaboration with other developers more efficient.”
Mark Thompson (Lead Python Developer, CodeCrafters). “A crucial aspect of writing effective Python scripts is understanding the importance of libraries and frameworks. Leveraging existing libraries can significantly reduce development time and improve functionality. Always explore the Python Package Index (PyPI) for tools that can streamline your scripting process.”
Linda Zhang (Data Scientist, Analytics Group). “Testing and validation are often overlooked when writing Python scripts. Implementing unit tests ensures that your code behaves as expected and helps catch errors early in the development process. Utilize frameworks like pytest to create a robust testing environment for your scripts.”
Frequently Asked Questions (FAQs)
What are the basic requirements to write Python scripts?
To write Python scripts, you need a computer with Python installed, a text editor or an Integrated Development Environment (IDE) such as PyCharm, Visual Studio Code, or Jupyter Notebook, and a basic understanding of Python syntax and programming concepts.
How do I create and run a simple Python script?
To create a simple Python script, open your text editor, write your Python code, and save the file with a `.py` extension. To run the script, open a terminal or command prompt, navigate to the directory where the file is saved, and execute the command `python filename.py`.
What are the common libraries used in Python scripting?
Common libraries used in Python scripting include NumPy for numerical computations, Pandas for data manipulation and analysis, Matplotlib for data visualization, Requests for making HTTP requests, and Flask for web development.
How can I debug my Python scripts?
You can debug Python scripts by using built-in debugging tools such as `pdb`, adding print statements to track variable values, or utilizing IDE features like breakpoints and step-through execution to analyze code behavior.
What is the difference between a script and a module in Python?
A script is a standalone file that is executed directly, typically containing code meant to perform a specific task. A module, on the other hand, is a file containing Python definitions and statements that can be imported and reused in other scripts.
How can I make my Python scripts more efficient?
To make your Python scripts more efficient, optimize algorithms, use built-in functions and libraries, minimize the use of global variables, avoid unnecessary computations, and profile your code to identify bottlenecks.
Writing Python scripts involves understanding the fundamental concepts of the Python programming language, including its syntax, data types, and control structures. To effectively create scripts, one must become familiar with the Python environment, including how to set up a development environment, utilize text editors or integrated development environments (IDEs), and manage libraries and dependencies. Learning to write scripts also requires practicing problem-solving skills and applying algorithms to automate tasks or analyze data.
Another critical aspect of writing Python scripts is mastering the use of functions and modules. Functions allow for code reusability and better organization, while modules help in structuring larger projects by grouping related functionalities. Additionally, understanding how to handle exceptions and implement error-checking mechanisms is essential for creating robust scripts that can gracefully manage unexpected situations.
Finally, testing and debugging are integral parts of the script-writing process. Utilizing tools like unit tests, debugging tools, and logging can significantly enhance the reliability and maintainability of Python scripts. By following best practices in coding, such as adhering to the PEP 8 style guide, developers can produce clean and efficient code that is easier to read and understand.
Author Profile
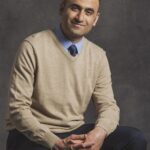
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?