How Can You Write a Square Function in Python?
In the world of programming, mastering the basics is essential for building more complex applications. One of the fundamental tasks that every budding programmer encounters is the ability to perform mathematical operations, such as calculating the square of a number. Whether you’re a novice coder looking to sharpen your skills or an experienced developer brushing up on Python’s capabilities, understanding how to write a square function in Python is a valuable exercise. This simple yet powerful operation lays the groundwork for more advanced programming concepts and can be applied in various real-world scenarios.
Calculating the square of a number in Python is not just about writing a few lines of code; it’s about understanding the underlying principles of functions and arithmetic operations in programming. Python, known for its readability and simplicity, offers several ways to achieve this task, allowing you to choose the method that best fits your coding style. From using basic arithmetic to leveraging built-in functions, the language provides flexibility that can enhance your coding experience.
As we delve deeper into this topic, we will explore different approaches to writing a square function in Python. By examining various techniques, you’ll gain insights into not only how to implement this operation but also how to think critically about problem-solving in programming. Whether for educational purposes, personal projects, or professional applications, mastering the
Using the Power Operator
To calculate the square of a number in Python, one of the simplest methods is to use the power operator `**`. This operator raises a number to the power of another number. For squaring, you would raise the number to the power of 2.
Example:
“`python
number = 5
square = number ** 2
print(square) Output: 25
“`
Utilizing the Built-in `pow()` Function
Python also provides a built-in function called `pow()`, which can be used to calculate the power of a number. This function can take two or three arguments, where the first two are the base and the exponent.
Example:
“`python
number = 6
square = pow(number, 2)
print(square) Output: 36
“`
Defining a Custom Function
For more complex applications, it might be beneficial to define your own function to compute the square. This approach enhances readability and allows for additional functionality, such as error checking.
Example:
“`python
def square(num):
return num * num
result = square(7)
print(result) Output: 49
“`
Using NumPy for Array Operations
When working with large datasets or arrays, the NumPy library can be extremely useful. NumPy allows you to perform element-wise operations on arrays efficiently.
Example:
“`python
import numpy as np
array = np.array([1, 2, 3, 4])
squared_array = np.square(array)
print(squared_array) Output: [ 1 4 9 16]
“`
Comparison of Methods
The following table summarizes the methods discussed for calculating squares in Python, along with their key features:
Method | Syntax | Pros | Cons |
---|---|---|---|
Power Operator | number ** 2 | Simple and intuitive | Limited to single numbers |
Built-in `pow()` | pow(number, 2) | Clear and built-in function | Less commonly used for squaring |
Custom Function | def square(num): return num * num | Flexible for enhancements | More verbose |
NumPy | np.square(array) | Efficient for large arrays | Requires additional library |
Each method has its advantages and can be chosen based on the specific requirements of your application, whether it be simplicity, flexibility, or efficiency.
Creating a Function to Calculate the Square
To compute the square of a number in Python, a straightforward approach is to create a function. This function will take a single argument and return its square.
“`python
def square(number):
return number * number
“`
This implementation uses the multiplication operator to calculate the square of the input `number`. You can call this function with any numeric value.
Using the Built-in Operator
In Python, you can also use the exponentiation operator (`**`) to calculate the square of a number. This method is particularly useful for readability, especially in more complex mathematical expressions.
“`python
def square_using_exponentiation(number):
return number ** 2
“`
Both methods yield the same result, but the choice of which to use can depend on personal or team coding standards.
Squaring a List of Numbers
If you need to calculate the square of multiple numbers, utilizing a list comprehension can be an efficient approach. This method allows you to apply the squaring operation to each element in a list.
“`python
numbers = [1, 2, 3, 4, 5]
squared_numbers = [square(x) for x in numbers]
“`
This will result in `squared_numbers` containing `[1, 4, 9, 16, 25]`. It’s a concise way to handle squaring operations across a dataset.
Using NumPy for Vectorized Operations
For larger datasets or when performance is a concern, leveraging the NumPy library can significantly enhance efficiency. NumPy allows for vectorized operations on arrays, making calculations faster and more concise.
“`python
import numpy as np
numbers_array = np.array([1, 2, 3, 4, 5])
squared_array = np.square(numbers_array)
“`
This approach will yield a NumPy array containing the squares, `[1, 4, 9, 16, 25]`, with the added benefit of optimized performance for larger arrays.
Handling Invalid Inputs
It is essential to manage the possibility of invalid inputs when creating functions. This can be done by implementing type checking and raising appropriate errors.
“`python
def safe_square(number):
if not isinstance(number, (int, float)):
raise ValueError(“Input must be a number.”)
return number * number
“`
This function checks if the input is a number and raises a `ValueError` if it is not, ensuring robustness in your code.
Performance Considerations
When deciding on the method for squaring numbers, consider the context and performance:
Method | Performance | Use Case |
---|---|---|
Multiplication | Fast for single values | Simple calculations |
Exponentiation | Slightly slower | Readability in complex expressions |
List Comprehension | Efficient for small lists | Batch processing of small datasets |
NumPy Vectorization | Very fast | Large datasets, performance-sensitive applications |
Choosing the right method depends on your specific needs, including the volume of data and desired performance characteristics.
Expert Insights on Writing Squares in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “To compute the square of a number in Python, one can simply use the exponentiation operator ‘**’. For example, ‘number ** 2’ effectively calculates the square of ‘number’, making it both intuitive and efficient for data manipulation.”
James Liu (Python Software Engineer, CodeCraft Solutions). “Using the built-in ‘pow()’ function is another excellent method for squaring a number in Python. The syntax ‘pow(number, 2)’ not only enhances readability but also aligns with best practices in coding for clarity and maintainability.”
Sarah Thompson (Educator and Author, Python Programming for Beginners). “For those new to Python, creating a simple function to return the square of a number can be a great learning exercise. A function like ‘def square(x): return x * x’ encapsulates the logic neatly and demonstrates the fundamentals of function definition.”
Frequently Asked Questions (FAQs)
How do I write a function to calculate the square of a number in Python?
To write a function that calculates the square of a number in Python, define a function using the `def` keyword and return the square of the input. For example:
“`python
def square(num):
return num ** 2
“`
What is the simplest way to compute the square of a number in Python?
The simplest way to compute the square of a number is to use the exponentiation operator `**`. For example:
“`python
result = 5 ** 2 This will give you 25
“`
Can I use the `math` module to calculate the square in Python?
While the `math` module does not have a specific function for squaring a number, you can use the `pow()` function from the module. For example:
“`python
import math
result = math.pow(5, 2) This returns 25.0
“`
How can I square a list of numbers in Python?
To square each number in a list, you can use a list comprehension. For example:
“`python
numbers = [1, 2, 3, 4]
squared_numbers = [num ** 2 for num in numbers] This results in [1, 4, 9, 16]
“`
Is there a built-in function in Python to square a number?
Python does not have a built-in function specifically for squaring a number, but you can easily create your own function or use the exponentiation operator `**` for this purpose.
What are some common mistakes when squaring numbers in Python?
Common mistakes include using incorrect operators (e.g., `*` instead of `**`), not handling data types properly (e.g., trying to square a string), and forgetting to return the result in a function.
In summary, writing a square in Python can be accomplished through various methods, depending on the context and requirements of the task. The most common approach involves using arithmetic operations to compute the square of a number. This can be done simply by multiplying the number by itself or using the exponentiation operator. For instance, both `x * x` and `x ** 2` will yield the square of `x` effectively.
Additionally, Python provides built-in functions and libraries that can facilitate more complex operations involving squares, such as NumPy for handling arrays and mathematical computations. When working with lists or arrays, one can utilize list comprehensions or vectorized operations to efficiently compute squares for multiple values at once. This showcases Python’s versatility and efficiency in handling mathematical tasks.
Moreover, it is essential to consider the readability and maintainability of the code. Using descriptive variable names and adhering to Python’s conventions, such as PEP 8, can enhance the clarity of the code. This is particularly important when writing functions that compute squares, as it allows others to easily understand and utilize the code in future applications.
whether you are performing simple arithmetic or working with larger datasets, Python offers robust methods for calculating
Author Profile
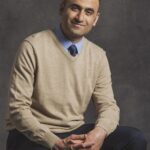
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?