How Can We Identify Implicit Downcasts and Understand Why They Are Prohibited?
In the realm of programming languages, the concept of type safety plays a pivotal role in ensuring that code runs smoothly and predictably. Among the various mechanisms that enforce type safety, implicit downcasting stands out as a particularly contentious topic. While the ability to convert one type into another without explicit instruction can streamline coding and enhance readability, it also introduces a host of potential pitfalls. In this article, we will delve into the nuances of implicit downcasts, exploring why they are often prohibited in many programming languages and the implications this has for developers.
Implicit downcasting occurs when a programming language automatically converts a variable from a parent type to a child type without the programmer’s direct intervention. This seemingly convenient feature can lead to unexpected behaviors, especially when the conversion is not safe or valid. For instance, if a variable of a more general type is treated as a more specific type, it may not possess the properties or methods expected by the code, resulting in runtime errors. Understanding the mechanics behind these conversions is crucial for developers who wish to write robust and error-free code.
The prohibition of implicit downcasts is primarily rooted in the desire to maintain type safety and prevent runtime exceptions. By requiring explicit downcasting, languages encourage developers to consciously acknowledge the risks associated with type conversions. This
Identify Implicit Downcasts
Implicit downcasts occur when a programming language automatically converts one data type into another. This usually happens in type hierarchies, specifically when converting a base class reference to a derived class reference. While this can simplify code and enhance readability, it can also lead to runtime errors if the conversion is not safe.
To identify implicit downcasts, consider the following indicators:
- Type Hierarchy: Understand the relationship between classes. If a class A is a base class of class B, a downcast might occur when using an object of type A as if it were of type B.
- Compiler Warnings: Many modern compilers provide warnings when a potential downcast occurs. Pay attention to these warnings to prevent issues.
- Code Reviews: Regular code reviews can help identify places where implicit downcasting is used, allowing for safer alternatives to be employed.
Understanding Why Implicit Downcasts Are Prohibited
Implicit downcasts are prohibited in many programming languages due to several reasons, primarily related to safety and maintainability. Here are some key points to consider:
- Type Safety: Implicit downcasts can lead to type safety issues. If an object does not actually belong to the derived class, attempting to access members specific to that class can result in runtime errors, such as `NullReferenceException` or `ClassCastException`.
- Code Clarity: When downcasting is explicit, it forces developers to acknowledge the potential risks involved. This enhances the readability and maintainability of the code by making type conversions clear.
- Performance Overhead: Implicit downcasting can introduce performance overhead due to the need for additional runtime checks. Avoiding implicit conversions can lead to more optimized code.
The following table summarizes the implications of implicit downcasts:
Aspect | Implication |
---|---|
Type Safety | Risk of runtime errors if the object is not of the expected type. |
Code Clarity | Implicit casts may obscure the intent of the code, making it harder to maintain. |
Performance | Potentially introduces runtime checks that can affect performance. |
Debugging | Implicit downcasts can complicate debugging processes, as errors may not be immediately apparent. |
while implicit downcasts can simplify certain operations, they carry significant risks that can jeopardize the integrity of software systems. Understanding the nature and consequences of these conversions is essential for writing robust and maintainable code.
Identifying Implicit Downcasts
Implicit downcasts occur when a type conversion is performed without an explicit cast. In many programming languages, this can happen automatically under certain circumstances, particularly in object-oriented programming. These conversions can lead to situations where a program behaves unexpectedly or introduces subtle bugs.
To identify implicit downcasts, consider the following scenarios:
- Inheritance Hierarchies: When a subclass is assigned to a superclass reference.
- Generic Collections: When objects of a subclass are added to a collection defined for the superclass.
- Method Overriding: When a method in a subclass overrides a method in a superclass, it may lead to implicit behavior that can obscure the actual type being used.
For example, in languages like Java, assigning an instance of a subclass to a variable of its superclass type can be done without a cast:
“`java
class Animal {}
class Dog extends Animal {}
Animal myDog = new Dog(); // Implicit downcast from Dog to Animal
“`
This can sometimes lead to potential runtime errors if the code later tries to use `myDog` as a `Dog` without proper casting.
Understanding Why Implicit Downcasts Are Prohibited
Implicit downcasts are often prohibited in strongly typed languages because they can lead to:
- Type Safety Violations: Allowing implicit downcasts can compromise type safety, making it easier to introduce bugs due to unexpected type conversions.
- Runtime Errors: If the actual object does not match the expected type at runtime, it can lead to exceptions and crashes. For example, attempting to call a method specific to a subclass on a superclass reference can result in a `ClassCastException`.
Consider the following table that outlines common issues arising from implicit downcasts:
Issue | Explanation |
---|---|
Ambiguous Code | Implicit casts can make code less readable and maintainable. |
Hidden Bugs | Bugs may remain undetected until runtime, complicating debugging. |
Performance Overhead | The runtime checks required for safety can introduce performance costs. |
Reduced Code Clarity | Explicit casts improve clarity, allowing developers to understand type relationships better. |
For these reasons, many programming languages enforce explicit casting, ensuring that developers are aware of potential type mismatches and the associated risks. This practice leads to more robust and maintainable code, improving overall software quality.
Understanding Implicit Downcasts and Their Prohibition in Programming
Dr. Emily Carter (Computer Science Professor, Tech University). “Implicit downcasts can lead to runtime errors that compromise the stability of applications. They obscure the programmer’s intent and make code harder to maintain, which is why many strongly typed languages prohibit them.”
Michael Tran (Senior Software Engineer, CodeSecure Inc.). “The prohibition of implicit downcasts is essential for type safety. When downcasts occur without explicit intent, it can result in unexpected behavior and bugs that are difficult to trace, ultimately affecting software reliability.”
Sarah Patel (Lead Developer, Innovative Solutions). “Understanding why implicit downcasts are prohibited is crucial for developers. They can introduce ambiguity in type hierarchies, making code less predictable and increasing the risk of runtime exceptions, which can severely impact user experience.”
Frequently Asked Questions (FAQs)
What are implicit downcasts in programming?
Implicit downcasts occur when a programming language automatically converts a variable from a base type to a derived type without explicit instructions from the programmer. This can lead to unintended behavior if the conversion is not safe.
Why are implicit downcasts prohibited in many programming languages?
Many programming languages prohibit implicit downcasts to prevent runtime errors and maintain type safety. Allowing such conversions can lead to situations where a variable does not contain the expected type, resulting in unpredictable behavior or crashes.
What are the risks associated with implicit downcasts?
Implicit downcasts can introduce risks such as data loss, exceptions, and behavior. If a downcast is made from a base type to a derived type that is not compatible, it may lead to runtime errors that are difficult to debug.
How can developers safely perform downcasts?
Developers can safely perform downcasts using explicit casting methods or type-checking mechanisms provided by the programming language. This ensures that the conversion is intentional and that the variable being cast is indeed of the expected type.
What is the difference between implicit and explicit downcasts?
Implicit downcasts are automatically handled by the compiler or interpreter, while explicit downcasts require the programmer to specify the conversion. Explicit downcasts provide greater control and clarity, reducing the risk of errors.
Can you provide an example of a situation where implicit downcasts might fail?
An example would be attempting to downcast an object of a base class to a derived class when the object is not actually an instance of that derived class. This would result in a runtime exception, as the conversion is invalid and the program cannot guarantee the object’s type.
Implicit downcasting refers to the automatic conversion of a reference of a base class type to a derived class type without explicit intervention from the programmer. This process can lead to potential issues, particularly when the object being referenced is not actually an instance of the derived class. Such scenarios can result in runtime errors, making implicit downcasts a risky practice in strongly typed programming languages. Consequently, many programming languages prohibit implicit downcasting to enhance type safety and prevent unforeseen errors during execution.
The prohibition of implicit downcasts is primarily rooted in the principles of type safety and maintainability. By requiring explicit downcasting, programmers are compelled to acknowledge the potential risks associated with type conversion. This practice fosters a more robust coding environment, as it encourages developers to verify the actual type of an object before performing a downcast. By doing so, the likelihood of encountering ClassCastExceptions or similar issues is significantly reduced, leading to more reliable and predictable code behavior.
Another important consideration is the impact of implicit downcasting on code readability and maintainability. When downcasts are made explicit, it becomes clearer to other developers (and the original author) what types are being manipulated. This clarity aids in understanding the code’s logic and intentions, thus facilitating easier debugging and future modifications.
Author Profile
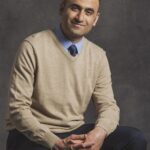
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?