How Can I Check if a File Exists in a Batch File?
Batch files are a powerful tool in the Windows operating system, allowing users to automate tasks and streamline workflows with simple scripts. Among the many commands available to batch file creators, the ability to check for the existence of files, directories, or even specific conditions is crucial for ensuring that scripts run smoothly and efficiently. The `if exists` command is a cornerstone of this functionality, enabling users to implement conditional logic that can enhance the robustness of their scripts. Whether you’re a seasoned programmer or a casual user looking to automate repetitive tasks, understanding how to leverage this command can significantly elevate your batch file capabilities.
In the realm of batch scripting, the `if exists` command serves as a gatekeeper, allowing scripts to make decisions based on the presence or absence of files and directories. This functionality is not only essential for error handling but also for optimizing the flow of operations within a script. By incorporating checks for existence, users can prevent unnecessary errors and ensure that their scripts only attempt to access resources that are available, leading to more reliable outcomes.
Moreover, the versatility of the `if exists` command extends beyond simple file checks. It can be used in various scenarios, such as verifying prerequisites before executing critical operations or managing dependencies between different scripts. As you delve deeper into the world of
Using IF EXISTS in Batch Files
In batch files, the `IF EXISTS` condition is not directly supported as it is in some other programming languages. However, you can achieve similar functionality by utilizing the `IF EXIST` command. This command checks for the existence of files or directories and allows you to execute specific commands based on the result.
To use `IF EXIST`, the general syntax is:
“`
IF EXIST “path\to\file_or_directory” (
) ELSE (
)
“`
This construct enables you to create conditional logic based on the presence of files or directories, effectively allowing you to manage operations dynamically.
Common Use Cases
The `IF EXIST` command is particularly useful in various scenarios:
- File Backup: Check if a backup file exists before creating a new one to avoid overwriting.
- Log File Management: Verify if a log file is present before attempting to read or process it.
- Script Control: Ensure necessary files are in place before executing critical scripts.
Examples of IF EXIST
Here are some practical examples demonstrating how to implement `IF EXIST` in a batch file.
Example 1: Checking for a File
“`batch
IF EXIST “C:\example\myfile.txt” (
echo The file exists.
) ELSE (
echo The file does not exist.
)
“`
Example 2: Conditional Copying
“`batch
IF EXIST “C:\example\source.txt” (
COPY “C:\example\source.txt” “C:\example\destination.txt”
) ELSE (
echo Source file not found, skipping copy.
)
“`
Using IF EXIST with Directories
You can also check for the existence of directories using the same command. This is particularly useful for verifying that a directory structure is in place before performing operations such as file copying or moving.
Example 3: Checking a Directory
“`batch
IF EXIST “C:\example\myfolder” (
echo The directory exists.
) ELSE (
echo The directory does not exist, creating it now.
mkdir “C:\example\myfolder”
)
“`
Considerations
When using `IF EXIST`, keep in mind:
- Quoting Paths: Always enclose paths in quotes if they contain spaces or special characters to avoid errors.
- File vs. Directory Checks: The command can check both files and directories but does not differentiate between them.
Command | Description |
---|---|
IF EXIST “file.txt” | Checks if “file.txt” exists. |
IF EXIST “C:\folder” | Checks if the folder exists. |
IF NOT EXIST “file.txt” | Executes commands if “file.txt” does not exist. |
Utilizing the `IF EXIST` command effectively can streamline your batch file operations and enhance error handling capabilities, making your scripts more robust and reliable.
Using `IF EXISTS` in Batch Files
In batch scripting, the `IF EXISTS` construct is not directly available as it is in some other scripting languages. However, you can achieve similar functionality using the `IF EXIST` command, which allows you to check for the existence of files or directories.
Syntax and Usage
The basic syntax for checking if a file or directory exists is as follows:
“`
IF EXIST “path\to\your\file_or_directory” (
REM Commands to execute if the file or directory exists
) ELSE (
REM Commands to execute if the file or directory does not exist
)
“`
Example
“`batch
@ECHO OFF
SET filePath=”C:\example\myfile.txt”
IF EXIST %filePath% (
ECHO File exists.
) ELSE (
ECHO File does not exist.
)
“`
In this example, if `myfile.txt` exists in the specified path, the script will output “File exists.” If it does not, it will output “File does not exist.”
Multiple File Checks
You can also check for multiple files using a combination of `IF EXIST` statements. This is useful when you want to verify the existence of several files before proceeding with further operations.
Example
“`batch
@ECHO OFF
SET file1=”C:\example\file1.txt”
SET file2=”C:\example\file2.txt”
IF EXIST %file1% (
ECHO File 1 exists.
) ELSE (
ECHO File 1 does not exist.
)
IF EXIST %file2% (
ECHO File 2 exists.
) ELSE (
ECHO File 2 does not exist.
)
“`
Directory Checks
The same `IF EXIST` command can be used to check for directories. This is particularly useful for validating paths before attempting to read from or write to them.
Example
“`batch
@ECHO OFF
SET dirPath=”C:\example\myfolder”
IF EXIST %dirPath% (
ECHO Directory exists.
) ELSE (
ECHO Directory does not exist.
)
“`
Combining Conditions
For more complex conditions, you can combine multiple checks using `&&` and `||`. This allows you to perform actions based on various scenarios.
Example
“`batch
@ECHO OFF
SET filePath=”C:\example\myfile.txt”
IF EXIST %filePath% (
ECHO File exists. && ECHO Proceeding with operation…
) ELSE (
ECHO File does not exist. || ECHO Exiting script.
)
“`
Using `IF EXIST` in batch files is a powerful method for controlling the flow of execution based on the presence of files or directories. By incorporating conditional checks, you can ensure that your scripts behave predictably and handle files safely.
Understanding the Use of “IF EXISTS” in Batch Files
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In batch file scripting, the ‘IF EXISTS’ command is crucial for checking the presence of files or directories before executing further commands. This prevents errors and enhances the robustness of scripts, especially in automated processes.”
Michael Chen (Systems Administrator, Cloud Solutions Corp.). “Utilizing ‘IF EXISTS’ in batch files allows administrators to create conditional workflows that can adapt based on the existence of certain files. This capability is essential for managing system resources effectively and ensuring smooth operations.”
Sarah Thompson (IT Consultant, Digital Transformation Group). “The ‘IF EXISTS’ statement is a fundamental component in batch scripting that enables developers to write more intelligent scripts. By incorporating this command, one can avoid unnecessary processing and improve script efficiency, particularly in large-scale deployments.”
Frequently Asked Questions (FAQs)
What is the purpose of using “if exists” in a batch file?
The “if exists” statement in a batch file is used to check for the presence of a specified file or directory. If the file or directory exists, the subsequent commands will be executed.
How do I use “if exists” to check for a file in a batch file?
You can use the syntax `if exists “filename” (commands)` where “filename” is the path to the file you want to check. If the file is found, the commands within the parentheses will execute.
Can “if exists” be used to check for directories as well?
Yes, “if exists” can be used to check for both files and directories. Simply specify the directory path in the same manner as you would for a file.
What happens if the file or directory does not exist in an “if exists” check?
If the specified file or directory does not exist, the commands following the “if exists” statement will be skipped, and the batch file will continue executing any subsequent commands.
Is it possible to use “if exists” with wildcards in batch files?
Yes, you can use wildcards with “if exists” to check for multiple files. For example, `if exists “C:\path\*.txt” (commands)` checks for the existence of any text files in the specified directory.
Can I combine “if exists” with other conditional statements in a batch file?
Yes, you can combine “if exists” with other conditional statements using logical operators. For instance, you can use `if exists “file1” if exists “file2” (commands)` to check for the existence of multiple files before executing commands.
In the context of batch files, the concept of “if exists” is pivotal for controlling the flow of execution based on the existence of files or directories. This conditional statement allows users to implement logic that can check for the presence of specific files before proceeding with further commands. By utilizing this functionality, batch scripts can avoid errors related to missing files and ensure that subsequent operations are only executed when the necessary prerequisites are met.
Moreover, the use of “if exists” enhances the robustness and reliability of batch scripts. By incorporating checks for file existence, developers can create scripts that are more resilient to changes in the file system, such as missing files or directories. This capability is particularly valuable in automated tasks, where the script’s performance can be significantly impacted by the presence or absence of required resources.
In summary, the “if exists” statement in batch files serves as a fundamental tool for conditional execution. It empowers users to write more intelligent scripts that can adapt to their environment, thereby improving the overall efficiency of automated processes. Understanding and effectively implementing this command is essential for anyone looking to optimize their batch scripting capabilities.
Author Profile
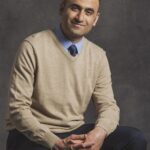
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?