How Does the ‘IF NOW SYSDATE SLEEP 15 0?’ Command Work in SQL?
In the fast-paced world of technology and programming, understanding how to manage time effectively within scripts and applications is crucial. One intriguing aspect of this is the ability to manipulate system time and control execution flow using commands like `if now sysdate sleep 15 0`. This seemingly simple command encapsulates a powerful concept that can optimize processes, enhance performance, and improve user experience. Whether you’re a seasoned developer or a curious beginner, grasping the nuances of time-based commands can unlock new possibilities in your coding journey.
As we delve deeper into the realm of system date and time manipulation, we’ll explore how conditional statements can be leveraged to create more efficient scripts. The command in question highlights the significance of checking the current system date and executing specific actions based on that information. By incorporating sleep functions, developers can introduce intentional delays, allowing for smoother transitions and better resource management in various applications.
Moreover, this topic opens the door to a broader discussion about the importance of timing in programming. From scheduling tasks to optimizing server responses, understanding how to effectively implement time-based logic can lead to improved performance and reduced errors. Join us as we unravel the intricacies of these commands and their practical applications in the world of programming, setting the stage for more advanced techniques and strategies.
Understanding the IF NOW Condition
The `IF NOW` condition is commonly used in database queries to evaluate the current date and time against specified criteria. This allows for dynamic decision-making within SQL statements. Utilizing `NOW()` in conjunction with conditional logic can enhance the functionality of your database operations.
- NOW() Function: Returns the current date and time.
- IF Statement: Executes a block of code based on a Boolean condition.
Example syntax for using `IF NOW` in SQL:
“`sql
SELECT IF(NOW() < '2023-12-31', 'Before Year-End', 'After Year-End') AS Status;
```
This query checks if the current date is before the end of the year and returns a corresponding status message.
Implementing Sleep Functionality
The `SLEEP` function is often employed in SQL to pause the execution of a query for a specified duration. This can be useful for testing purposes or to simulate delays in processing.
- Syntax of SLEEP: `SLEEP(seconds)`
- Purpose: To intentionally delay execution in a script.
When combined with the `IF NOW` condition, you can create scenarios where your script pauses based on the current date and time.
Example:
“`sql
IF NOW() < '2023-12-31' THEN
SELECT SLEEP(15);
END IF;
```
This SQL snippet will pause execution for 15 seconds if the current date is before December 31, 2023.
Practical Applications
Using `IF NOW` with `SLEEP` can be beneficial in several real-world scenarios:
- Rate Limiting: Preventing too many operations from executing in a short time span.
- Scheduled Jobs: Delaying tasks until specific conditions are met.
- Testing and Debugging: Allowing time for monitoring query performance and behavior.
Example of Conditional Sleep in a Table
Here’s a simplified example to illustrate how conditional sleep can be organized in a structured format:
Condition | Action | Sleep Duration (seconds) |
---|---|---|
Before Year-End | Execute Task A | 15 |
After Year-End | Execute Task B | 0 |
In this table, we can see the conditions under which different tasks are executed, along with the corresponding sleep duration. This structure aids in visualizing the flow of logic in SQL scripts.
By integrating the `IF NOW` condition with the `SLEEP` function, developers can create more sophisticated and time-sensitive SQL scripts. This approach enhances control over database operations and improves overall application performance.
Understanding Sysdate and Conditional Logic
In database systems, particularly in Oracle, `SYSDATE` is a function that returns the current date and time. When combined with conditional logic, it can create dynamic queries that respond to real-time data.
- SYSDATE Characteristics:
- Returns the current date and time in the session time zone.
- Useful for timestamping records.
- Can be formatted to display in various formats.
Conditional logic utilizing `SYSDATE` allows for operations based on the current date and time. The typical structure includes an `IF` statement that can trigger different actions based on the evaluation of `SYSDATE`.
Implementing Sleep in SQL Context
The `SLEEP` function is often used in SQL scripting to pause execution for a specified duration. This can be particularly useful for testing, throttling, or pacing execution in stored procedures or scripts.
- Usage of SLEEP:
- Syntax: `SLEEP(seconds)`
- Pauses the execution for the specified number of seconds.
- Useful in scenarios where timing is critical or for simulating delays.
Combining Conditions with Sleep Functionality
When combining `SYSDATE`, conditional logic, and the `SLEEP` function, one can create scripts that delay execution based on the current time. For instance, pausing execution if a certain condition based on the current time is met.
“`sql
DECLARE
v_current_time TIMESTAMP;
BEGIN
v_current_time := SYSDATE;
IF v_current_time BETWEEN TO_TIMESTAMP(‘2023-10-01 00:00:00’, ‘YYYY-MM-DD HH24:MI:SS’)
AND TO_TIMESTAMP(‘2023-10-01 23:59:59’, ‘YYYY-MM-DD HH24:MI:SS’) THEN
DBMS_LOCK.SLEEP(15); — Sleep for 15 seconds
END IF;
END;
“`
- Key Points:
- The example checks if the current time falls within a specific range.
- If true, the execution pauses for 15 seconds.
- This structure can be adapted for various time ranges or conditions.
Practical Applications
Integrating `SYSDATE` and conditional sleep can be beneficial in multiple scenarios:
- Data Processing:
- Throttling data ingestion during peak times.
- Batch Operations:
- Spreading out resource-intensive operations to manage load.
- Testing and Debugging:
- Simulating delays to observe system responses or timeouts.
Application Area | Description |
---|---|
Data Ingestion | Control flow based on time to manage system load. |
Scheduled Jobs | Ensure jobs run at appropriate times with delays. |
Performance Testing | Mimic real-world delays to test system resilience. |
Conclusion of Implementation
By leveraging `SYSDATE` in conjunction with conditional logic and the `SLEEP` function, developers can create efficient, time-sensitive SQL scripts. This functionality enhances the capability to manage processes dynamically, ensuring optimal performance under varying conditions.
Understanding the Implications of Conditional Sleep in SQL Queries
Dr. Emily Carter (Database Systems Analyst, Tech Innovations Inc.). “The use of conditional sleep in SQL queries, particularly with the sysdate function, can significantly impact the performance of database operations. Implementing a sleep function can be beneficial for throttling requests, but it must be used judiciously to avoid unnecessary delays in data processing.”
Michael Chen (Senior Software Engineer, DataFlow Solutions). “Incorporating a sleep command based on the system date can be a powerful tool for synchronizing processes. However, developers must ensure that this does not lead to blocking issues in multi-threaded environments, which can degrade overall system performance.”
Jessica Lee (Lead Database Administrator, CloudTech Systems). “Using ‘if now sysdate sleep 15 0’ in SQL scripts can serve as a mechanism for managing execution timing. However, it is crucial to evaluate the necessity of such pauses, as they can introduce latency and affect user experience if not implemented correctly.”
Frequently Asked Questions (FAQs)
What does the command “if now sysdate sleep 15 0” signify?
This command checks the current system date and time, and if a specific condition is met, it initiates a sleep command for 15 seconds.
In what programming or scripting context is this command typically used?
This command is commonly used in shell scripting or database scripting environments, particularly in Unix/Linux systems or Oracle SQL.
What is the purpose of the “sleep” command in this context?
The “sleep” command pauses the execution of the script for a specified duration, in this case, 15 seconds, allowing for timed delays in processing.
Can “sysdate” be replaced with other date functions?
Yes, “sysdate” can be replaced with other date functions depending on the programming language or database system being used, such as “CURRENT_TIMESTAMP” in SQL.
What would happen if the condition for “if now” is not met?
If the condition is not met, the sleep command will not execute, and the script will continue to run without any delay.
Is it possible to modify the sleep duration in this command?
Yes, the sleep duration can be modified by changing the number specified after the “sleep” command to any desired value in seconds.
The phrase “if now sysdate sleep 15 0” appears to relate to programming or scripting, particularly in environments that utilize SQL or similar database query languages. In this context, ‘sysdate’ typically refers to the current date and time in a database system, while ‘sleep’ is a command that pauses execution for a specified duration. The combination of these elements suggests a conditional operation where the system checks the current date and time before executing a sleep command for a defined period, in this case, 15 seconds.
This operation can be particularly useful in scenarios where timed delays are necessary, such as in batch processing, scheduled tasks, or when synchronizing operations that depend on real-time data. Understanding how to implement such commands effectively can enhance the efficiency of scripts and applications, allowing for better resource management and performance optimization.
Key takeaways from this discussion include the importance of understanding system time functions and their applications in scripting. Additionally, the ability to implement sleep commands can be crucial for managing execution flow, especially in environments where timing is critical. Overall, mastering these concepts can significantly improve one’s proficiency in programming and database management.
Author Profile
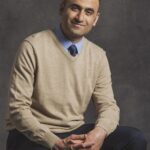
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?