Why Am I Getting an ‘Illegal Start of Expression’ Error in Java?
In the world of Java programming, encountering errors is an inevitable part of the development journey. Among the myriad of error messages that can pop up during coding, the “illegal start of expression” error stands out as a particularly perplexing one. This cryptic message can leave even seasoned developers scratching their heads, wondering what went wrong in their carefully crafted code. Understanding this error is crucial for anyone looking to enhance their Java skills, as it often points to fundamental issues in syntax or structure that can derail an otherwise flawless program.
The “illegal start of expression” error typically arises when the Java compiler encounters a line of code that does not conform to the expected syntax rules. This can happen for various reasons, such as missing punctuation, misplaced keywords, or incorrect use of operators. As Java is a strongly typed language with strict syntax requirements, even a minor oversight can lead to this frustrating error message. For beginners, it can be particularly daunting, as deciphering the root cause requires a solid grasp of Java’s syntax and conventions.
In this article, we will delve into the common scenarios that lead to the “illegal start of expression” error, exploring the underlying principles of Java syntax that every developer should know. By breaking down the causes and providing practical examples, we aim to
Understanding the Illegal Start of Expression Error
The “illegal start of expression” error in Java is a compilation error that typically occurs when the Java compiler encounters a syntax issue in the code. This error can be frustrating for developers, especially when the offending line seems to be correct at first glance. Identifying the root cause requires a careful examination of the code structure.
Common causes of the “illegal start of expression” error include:
- Incorrect Use of Braces or Parentheses: Mismatched or misplaced braces, brackets, or parentheses can confuse the compiler.
- Improper Variable Declarations: Declaring variables outside of methods or in inappropriate contexts leads to this error.
- Missing Semicolons: Forgetting to terminate a statement with a semicolon can cause the compiler to misinterpret subsequent lines.
- Using Reserved Keywords: Attempting to use Java reserved keywords as variable names or identifiers will trigger this error.
Examples of Causes
To illustrate how this error manifests, consider the following examples:
- Mismatched Braces
“`java
public class Example {
public void method() {
System.out.println(“Hello, World!”
} // Missing closing parenthesis
}
“`
- Incorrect Variable Declaration
“`java
public class Example {
int x;
public void method() {
int y = 10;
}
int z = 5; // Illegal start of expression
}
“`
- Missing Semicolon
“`java
public class Example {
public void method() {
int a = 5
System.out.println(a);
}
}
“`
Identifying the Error
When debugging the “illegal start of expression” error, follow these steps to systematically identify the issue:
- Check for Syntax Errors: Review the code for missing or mismatched symbols.
- Validate Variable Scope: Ensure that variables are declared within the correct context (e.g., inside methods).
- Use an IDE: Integrated Development Environments (IDEs) often highlight syntax errors, making it easier to spot issues.
Resolving the Error
To fix the “illegal start of expression” error, consider the following strategies:
- Correct Mismatched Symbols: Ensure all braces, brackets, and parentheses are properly matched.
- Reorganize Code Structure: Move variable declarations to the appropriate locations.
- Add Missing Semicolons: Ensure every statement is properly terminated.
Error Cause | Example Code | Resolution |
---|---|---|
Mismatched Parentheses | System.out.println("Hello, World!" |
Add closing parenthesis |
Improper Variable Declaration | int z = 5; |
Move declaration inside a method |
Missing Semicolon | int a = 5 |
Add semicolon after statement |
By carefully examining these common pitfalls, developers can efficiently troubleshoot and resolve the “illegal start of expression” error in their Java applications.
Understanding the “Illegal Start of Expression” Error
The “illegal start of expression” error in Java typically occurs during the compilation phase, indicating that the Java compiler has encountered a piece of code that does not conform to the syntactical rules of the language. This error can arise from various issues, primarily related to misplaced symbols, incorrect structure, or improper declarations.
Common Causes
Identifying the root cause of this error can significantly simplify the debugging process. Here are some frequent scenarios that lead to this error:
- Misplaced Keywords: Using keywords inappropriately, such as placing a method definition inside another method.
- Incorrect Syntax: Missing semicolons, parentheses, or curly braces can create confusion in code structure.
- Variable Declarations: Declaring variables outside of a method or block incorrectly can trigger this error.
- Comments: Incorrectly formatted comments, such as an unclosed multi-line comment, can lead to unexpected behavior.
- Class and Method Structure: Class definitions or method signatures not following proper syntax.
Example Scenarios
To illustrate the error, consider the following code snippets:
“`java
public class Example {
public void method() {
int a = 5
System.out.println(a); // Missing semicolon
}
}
“`
This code will produce an “illegal start of expression” error due to the missing semicolon after the variable declaration.
Another example is:
“`java
public class Example {
public void method() {
int a = 5;
}
public void method2() {
int b = 10;
}
// A misplaced return statement
return; // Illegal start of expression
}
“`
Here, the return statement is incorrectly placed outside any method.
How to Fix the Error
Fixing the “illegal start of expression” error involves carefully reviewing the code for syntax issues. Follow these steps:
- Check Syntax:
- Ensure all statements end with a semicolon.
- Verify that all opening parentheses, braces, or brackets have corresponding closing counterparts.
- Review Declarations:
- Confirm that all variable declarations occur within a method or a block of code.
- Inspect Comments:
- Ensure comments are correctly formatted. Use `//` for single-line comments and `/* … */` for multi-line comments.
- Validate Structure:
- Ensure that methods are declared correctly within the class and not nested improperly.
Code Structure Best Practices
To prevent such errors, adhere to the following coding best practices:
Practice | Description |
---|---|
Use Consistent Indentation | Helps in visualizing code blocks and reduces errors. |
Comment Your Code | Explain the purpose of complex code sections. |
Modularize Code | Break down code into smaller methods for clarity. |
Regularly Compile | Compile code frequently to catch errors early. |
By applying these principles, developers can significantly reduce the incidence of syntax errors, including the “illegal start of expression” error in Java.
Understanding the “Illegal Start of Expression” Error in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘illegal start of expression’ error in Java typically occurs due to syntax issues, such as misplaced brackets or incorrect variable declarations. It is crucial for developers to carefully review their code structure to identify and rectify these errors.”
James Patel (Java Programming Instructor, Code Academy). “This error often confuses novice programmers. It is essential to understand that Java is sensitive to syntax, and even a small mistake, like a missing semicolon or an extra comma, can trigger this message. Encouraging students to use an IDE with syntax highlighting can help them catch these errors early.”
Linda Choi (Lead Developer, Software Solutions Group). “In my experience, the ‘illegal start of expression’ error can also arise from incorrect use of keywords or reserved words in Java. Developers should familiarize themselves with Java’s syntax rules and best practices to minimize such occurrences.”
Frequently Asked Questions (FAQs)
What does “illegal start of expression” mean in Java?
The “illegal start of expression” error in Java indicates that the compiler has encountered code that does not conform to the expected syntax, often due to misplaced symbols or incorrect structure.
What are common causes of the “illegal start of expression” error?
Common causes include missing semicolons, incorrect use of parentheses or braces, misplaced keywords, and defining variables or methods incorrectly.
How can I resolve the “illegal start of expression” error?
To resolve this error, carefully review the code for syntax issues, ensure all statements are correctly terminated, and verify that all expressions are properly structured.
Can the “illegal start of expression” error occur in Java comments?
Yes, if comments are improperly formatted or if comment syntax is mixed with code incorrectly, it can lead to this error.
Does the “illegal start of expression” error affect the entire program?
Yes, this error typically prevents the program from compiling, meaning that the entire codebase must be error-free before it can be executed.
Is the “illegal start of expression” error specific to certain Java versions?
No, this error is not specific to any Java version; it is a general syntax error that can occur in any version of Java when the code does not follow the language’s syntax rules.
The phrase “illegal start of expression” in Java typically refers to a compilation error that occurs when the Java compiler encounters a syntax issue in the code. This error can arise from various causes, such as misplaced punctuation, incorrect use of keywords, or improper structuring of statements. Understanding the context in which this error occurs is crucial for effective troubleshooting and debugging in Java programming.
Common scenarios leading to this error include missing semicolons, incorrect placement of braces, or attempting to declare variables in an invalid manner. Additionally, the error may surface if there are issues with comments or if the code is not properly formatted. Developers should pay close attention to the lines indicated in the error message, as they often provide clues about the nature of the syntax problem.
To prevent the “illegal start of expression” error, it is essential for programmers to adhere to Java’s strict syntax rules and conventions. Utilizing an Integrated Development Environment (IDE) can significantly aid in identifying syntax errors early in the coding process. Furthermore, regular code reviews and adherence to best practices in programming can enhance overall code quality and reduce the likelihood of encountering such errors.
Author Profile
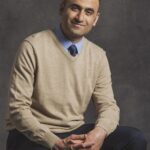
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?