Why Am I Encountering ImportError: Cannot Import Name ‘Builder’ from ‘Google.Protobuf.Internal’?
In the ever-evolving landscape of software development, encountering errors is an inevitable part of the journey. Among these, the `ImportError: cannot import name ‘builder’ from ‘google.protobuf.internal’` has emerged as a common stumbling block for developers utilizing the Google Protocol Buffers (protobuf) library. This error not only disrupts workflow but also raises questions about compatibility, library versions, and the intricacies of Python’s import system. As developers strive to build robust applications, understanding the nuances behind such errors becomes crucial for effective troubleshooting and resolution.
This article delves into the heart of the `ImportError` associated with the protobuf library, exploring its causes and implications. We will dissect the underlying reasons why this error occurs, particularly in the context of version mismatches and changes in library structure. Additionally, we will highlight best practices for managing dependencies and ensuring a smooth development experience, equipping you with the knowledge to tackle similar issues in the future.
As we navigate through the complexities of Python imports and the protobuf library, you’ll gain insights into not only resolving this specific error but also enhancing your overall approach to debugging in Python. Whether you’re a seasoned developer or just starting your coding journey, understanding these concepts will empower you to build more resilient applications and streamline your development
Understanding the ImportError
The error message `ImportError: cannot import name ‘builder’ from ‘google.protobuf.internal’` typically indicates a problem with the Python environment or the installed version of the `protobuf` library. This issue arises when the specified module or component cannot be located within the given package.
Several factors can contribute to this error:
- Version Mismatch: Different versions of `protobuf` may have varying internal structures. If your code is referencing a component that has been moved or renamed in a newer or older version, this error may occur.
- Corrupted Installation: An incomplete or corrupted installation of the `protobuf` package can lead to missing components, causing import failures.
- Environment Conflicts: When multiple Python environments or installations are present, the desired module may not be correctly referenced.
Steps to Resolve the ImportError
To troubleshoot and resolve the `ImportError`, follow these steps:
- Check Your Protobuf Version: Ensure that you are using a compatible version of the `protobuf` library that contains the required module.
“`bash
pip show protobuf
“`
- Upgrade or Downgrade Protobuf: Depending on the compatibility with your code, you may need to upgrade or downgrade the `protobuf` package.
“`bash
pip install –upgrade protobuf
“`
Or for downgrading:
“`bash
pip install protobuf==
“`
- Reinstall the Protobuf Package: If the installation seems corrupted, reinstall the package.
“`bash
pip uninstall protobuf
pip install protobuf
“`
- Verify Import Statements: Ensure that your import statements are correctly referencing the intended module and that there are no typos.
- Check for Environment Issues: If using virtual environments, confirm that you are operating within the correct environment where `protobuf` is installed.
Checking Python and Protobuf Compatibility
It is essential to ensure that the version of Python you are using is compatible with the version of the `protobuf` library. The following table outlines compatibility between Python versions and `protobuf`:
Python Version | Compatible Protobuf Version |
---|---|
3.6 | 3.12.0 or later |
3.7 | 3.12.0 or later |
3.8 | 3.12.0 or later |
3.9 | 3.12.0 or later |
3.10+ | 3.15.0 or later |
Common Alternatives and Solutions
If the above steps do not resolve the issue, consider the following alternatives:
- Use an Alternative Library: Depending on your requirements, you may find other libraries that can fulfill your needs without the complications associated with `protobuf`.
- Check GitHub Issues: Review the `protobuf` GitHub repository for any known issues related to your error. Often, the community or maintainers may have documented solutions.
- Seek Community Help: Engaging with developer communities on forums like Stack Overflow can provide insights and solutions from others who have faced similar issues.
By systematically following these steps, you can effectively troubleshoot and resolve the `ImportError` related to `google.protobuf.internal`.
Understanding the ImportError
The error message `ImportError: cannot import name ‘builder’ from ‘google.protobuf.internal’` typically indicates that the Python interpreter is unable to locate the specified module or name within the module. This can arise due to several reasons:
- Version Mismatch: The version of the `protobuf` library installed may not contain the `builder` module. Newer versions may have deprecated or restructured certain components.
- Installation Issues: The `protobuf` library might not be installed correctly, or there may be conflicting installations in the Python environment.
- Environment Problems: If multiple environments (like virtual environments) are being used, the module may not be accessible in the current environment.
Troubleshooting Steps
To resolve the ImportError, follow these troubleshooting steps:
- Check Installed Version:
- Verify the version of `protobuf` installed:
“`bash
pip show protobuf
“`
- Upgrade or Install:
- If the version is outdated or missing, you can upgrade or install it using:
“`bash
pip install –upgrade protobuf
“`
- Inspect the Module:
- Check the content of the `google.protobuf.internal` package to ensure `builder` exists:
“`python
import google.protobuf.internal
print(dir(google.protobuf.internal))
“`
- Check for Conflicts:
- Ensure there are no conflicting installations:
“`bash
pip list
“`
- Virtual Environment:
- If using a virtual environment, ensure it is activated and contains the `protobuf` package:
“`bash
source
“`
Common Solutions
Here are several solutions that have proven effective for similar issues:
- Reinstall the `protobuf` Library:
“`bash
pip uninstall protobuf
pip install protobuf
“`
- Check for Compatibility:
- Ensure that other dependencies are compatible with the version of `protobuf` you are using. Use:
“`bash
pip check
“`
- Use an Alternative Import:
- If the `builder` functionality is not critical, consider if there are alternative imports or methods to achieve the same goal.
Example of Correct Import Usage
To avoid ImportErrors, ensure you are using imports correctly. Here’s an example of how to import from `protobuf`:
“`python
from google.protobuf import message
from google.protobuf import descriptor
“`
If `builder` is indeed required, check the latest documentation for any changes in its import path or usage.
When to Seek Further Help
If the problem persists after following the above steps:
- Consult the official [protobuf GitHub repository](https://github.com/protocolbuffers/protobuf) for issues related to the specific version.
- Review the [Stack Overflow](https://stackoverflow.com/) community for similar questions or ask a new question with specific details.
- Refer to the Python community forums to get insights from other developers facing similar issues.
Understanding the ImportError in Protobuf Libraries
Dr. Emily Chen (Software Engineer, Google Cloud). “The ‘ImportError: cannot import name ‘builder’ from ‘google.protobuf.internal” typically arises due to version mismatches between the protobuf library and the codebase. Ensuring that the installed version of protobuf aligns with the expected version in your project can resolve this issue.”
Michael Thompson (Senior Python Developer, Tech Innovations Inc.). “This error often indicates that the specific module or class you are trying to import has either been deprecated or relocated in recent updates. A thorough review of the library’s documentation can provide clarity on the current structure and available components.”
Lisa Martinez (DevOps Specialist, Cloud Solutions Group). “When encountering this import error, it is essential to check your environment for conflicting installations of protobuf. Utilizing virtual environments can help isolate dependencies and prevent such conflicts from arising.”
Frequently Asked Questions (FAQs)
What causes the error “importerror: cannot import name ‘builder’ from ‘google.protobuf.internal'”?
This error typically occurs when there is a version mismatch between the installed `protobuf` library and the code attempting to import the `builder` module. It may also arise if the `protobuf` package is not correctly installed or if there are conflicting installations.
How can I resolve the import error related to ‘builder’ in protobuf?
To resolve this error, ensure that you have the correct version of the `protobuf` library installed. You can update or reinstall it using pip with the command `pip install –upgrade protobuf`. Additionally, verify that there are no conflicting versions in your environment.
What version of protobuf should I use to avoid this import error?
The recommended version of `protobuf` can vary depending on your specific project requirements. However, it is generally advisable to use the latest stable release unless your project explicitly requires an older version. Check the project’s documentation for compatibility.
Can this error occur in virtual environments?
Yes, this error can occur in virtual environments if the `protobuf` library is not installed or if an incompatible version is installed. Always ensure that your virtual environment is properly set up with the required dependencies.
Is there a way to check the installed version of protobuf?
Yes, you can check the installed version of `protobuf` by running the command `pip show protobuf` in your terminal. This will display the currently installed version along with other package details.
What should I do if the problem persists after reinstalling protobuf?
If the problem persists, consider checking for other dependencies in your project that may rely on `protobuf`. Additionally, review your code for any typos or incorrect import statements. If necessary, consult the official `protobuf` documentation or community forums for further assistance.
The error message “ImportError: cannot import name ‘builder’ from ‘google.protobuf.internal'” typically indicates a problem with the installation or compatibility of the Google Protocol Buffers library (protobuf) in a Python environment. This issue can arise due to various reasons, such as using an outdated version of the library, conflicts with other installed packages, or changes in the internal structure of the protobuf library itself. Understanding the context of this error is crucial for troubleshooting and resolving the issue effectively.
One of the primary steps to resolve this error is to ensure that the protobuf library is correctly installed and updated to a compatible version. Users should check their current installation using package management tools like pip and consider upgrading to the latest version. Additionally, it is essential to verify that there are no conflicting installations of protobuf that could lead to import errors. This may involve checking the Python environment and ensuring that the correct version is being referenced in the code.
Another key takeaway is the importance of reviewing the official documentation and release notes of the protobuf library. Changes in the library’s structure or functionality can lead to deprecated components, such as the ‘builder’ module, which may no longer be available in newer versions. Staying informed about these changes can help developers avoid potential pitfalls and
Author Profile
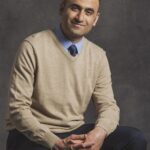
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?