Why Am I Encountering ‘ImportError: Cannot Import Name UserProfile’ in My Python Project?
In the world of Python programming, encountering errors is an inevitable part of the development journey. Among the myriad of issues that can arise, the `ImportError: cannot import name userprofile` stands out as a common yet perplexing challenge for developers. This error not only disrupts the flow of coding but also leaves many scratching their heads, wondering what went wrong in their import statements. Understanding the roots of this error is crucial for both novice and seasoned programmers, as it can serve as a gateway to mastering Python’s import system and enhancing overall coding efficiency.
At its core, this ImportError typically arises when Python fails to locate a specified module or function within a module. The `userprofile` in question may be part of a larger package, and various factors—ranging from circular imports to misnamed files—can contribute to this issue. As developers delve into the intricacies of their code, recognizing the common pitfalls associated with importing modules can pave the way for smoother debugging processes and more robust applications.
In this article, we will explore the potential causes of the `ImportError: cannot import name userprofile`, providing insights into how to diagnose and resolve the issue effectively. By unpacking the nuances of Python’s import system, we aim to equip you with the
Understanding ImportError in Python
When working with Python, encountering an `ImportError` can be a common issue that developers face. This error signifies that the Python interpreter is unable to locate the module or specific name you are attempting to import. One common variant of this error is `ImportError: cannot import name ‘UserProfile’`, which often indicates specific underlying issues in your code or environment.
Common Causes of ImportError
There are several reasons why this error might occur:
- Module Not Installed: The module you are trying to import may not be installed in your Python environment.
- Circular Imports: If two modules try to import each other, it can lead to a circular dependency, causing an ImportError.
- Incorrect Import Statements: A typo in the module name or the object being imported can lead to this error.
- File Structure Issues: If your project structure is not properly set up, Python might not be able to locate the module.
Troubleshooting Steps
To resolve an `ImportError`, follow these troubleshooting steps:
- Check Module Installation:
- Use `pip list` to verify if the required module is installed.
- If not, install it using `pip install module_name`.
- Examine Your Imports:
- Review your import statements for typographical errors.
- Ensure that you are importing the correct module and object name.
- Avoid Circular Imports:
- Refactor your code to eliminate circular dependencies.
- Consider using local imports within functions to avoid this issue.
- Check File Structure:
- Ensure that your Python files are organized correctly and that the `__init__.py` file is present in your packages.
Example of a Circular Import Issue
To illustrate how circular imports can lead to an `ImportError`, consider the following structure:
“`
project/
│
├── module_a.py
└── module_b.py
“`
module_a.py:
“`python
from module_b import UserProfile
class ProfileA:
pass
“`
module_b.py:
“`python
from module_a import ProfileA
class UserProfile:
pass
“`
In this scenario, both modules are attempting to import each other, which can lead to the `ImportError`.
Best Practices to Avoid ImportError
To minimize the occurrence of `ImportError`, consider the following best practices:
- Organize Code into Packages: Use packages to group related modules, which makes managing imports easier.
- Utilize Absolute Imports: Prefer absolute imports over relative imports to avoid confusion.
- Keep Imports at the Top: Maintain imports at the top of your module, which improves readability and reduces circular import risks.
Summary Table of ImportError Causes and Solutions
Cause | Solution |
---|---|
Module Not Installed | Install with pip |
Circular Import | Refactor code to eliminate circular dependencies |
Typographical Error | Check import statements for typos |
Improper File Structure | Ensure correct organization of Python files |
By adhering to these troubleshooting steps and best practices, developers can effectively address the `ImportError` and streamline their coding process.
Common Causes of ImportError in Python
ImportError issues, particularly the error message `cannot import name userprofile`, typically arise from several common scenarios in Python development. Understanding these causes can facilitate quicker debugging and resolution.
- Circular Imports: This occurs when two or more modules depend on each other. If module A tries to import from module B, and module B tries to import from module A, Python may not be able to resolve the imports correctly.
- Incorrect Module Path: If the module containing `userprofile` is not in the Python path or installed correctly, this error may occur. Verifying the module’s installation and ensuring it is accessible is crucial.
- Typographical Errors: Simple misspellings in the import statement can lead to this error. Double-checking the case sensitivity and spelling of the import statement is important, as Python is case-sensitive.
- Version Compatibility: The `userprofile` feature may have been deprecated or removed in certain versions of a library or framework. Consulting the library’s documentation for changes in newer versions can help identify this issue.
Debugging Steps to Resolve ImportError
To effectively troubleshoot the `ImportError: cannot import name userprofile`, follow these systematic steps:
- Check the Import Statement:
- Ensure that the syntax of the import statement is correct.
- Example:
“`python
from module_name import userprofile
“`
- Verify Module Installation:
- Use pip to check if the module is installed:
“`bash
pip show module_name
“`
- If not installed, add it using:
“`bash
pip install module_name
“`
- Inspect Module Structure:
- Open the module file and confirm that `userprofile` is defined within it.
- Ensure that the `__init__.py` file (if applicable) is correctly exporting `userprofile`.
- Check for Circular Dependencies:
- Refactor the code to avoid circular imports by reorganizing the import statements or restructuring the modules.
- Update Libraries:
- If the issue is due to version compatibility, consider updating the library:
“`bash
pip install –upgrade module_name
“`
Best Practices to Avoid ImportError
Implementing best practices can help prevent the `ImportError` in future developments:
- Modular Design: Structure code into separate modules with clear responsibilities to minimize interdependencies.
- Explicit Imports: Use explicit imports rather than wildcard imports to avoid ambiguity.
- Environment Management: Utilize virtual environments to isolate dependencies for different projects, reducing the risk of version conflicts.
- Documentation Review: Regularly review library documentation for any updates or deprecated features before upgrading.
Example Scenario
Consider a scenario where a Python project has the following structure:
“`
project/
│
├── module_a.py
├── module_b.py
└── __init__.py
“`
If `module_a.py` contains:
“`python
from module_b import userprofile
“`
And `module_b.py` looks like this:
“`python
from module_a import some_function Circular import
def userprofile():
pass
“`
This will raise the `ImportError` due to the circular dependency. Refactoring to remove the dependency can resolve the issue.
By understanding the common causes and following the debugging steps, developers can effectively address and prevent the `ImportError: cannot import name userprofile`. Adopting best practices in module design and import management can significantly reduce the incidence of similar errors.
Resolving Import Errors in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘ImportError: cannot import name userprofile’ typically arises when the specified module or class does not exist in the current namespace. It is crucial to verify that the module is correctly defined and accessible within your project structure.”
James Liu (Software Engineer, Open Source Contributor). “This import error often indicates a circular import issue or a typo in the module name. To troubleshoot, check for any circular dependencies in your code and ensure that the spelling of the module and class names is accurate.”
Maria Gonzalez (Technical Support Specialist, Python Solutions Co.). “When facing the ‘ImportError: cannot import name userprofile’, it is advisable to review the __init__.py files in your package directories. These files are essential for Python to recognize the directory as a package and can often lead to import issues if misconfigured.”
Frequently Asked Questions (FAQs)
What does the error “ImportError: cannot import name userprofile” indicate?
This error indicates that the Python interpreter is unable to locate the specified name `userprofile` in the module you are trying to import from. This could be due to a typo, a missing definition, or an incorrect module path.
How can I resolve the “ImportError: cannot import name userprofile” error?
To resolve this error, ensure that the module you are importing from actually contains the `userprofile` definition. Check for typos in the import statement and verify that you are using the correct module path.
Could the “ImportError” be caused by circular imports?
Yes, circular imports can lead to this error. If two modules are trying to import each other, it can result in one of the modules not being fully initialized when the import statement is executed.
Is it possible that the `userprofile` function or class was removed in a recent update?
Yes, if you are using a third-party library, it is possible that the `userprofile` function or class was removed or renamed in a recent update. Check the library’s documentation or changelog for any such changes.
What steps can I take to debug this issue further?
To debug, first check the module’s source code to confirm the presence of `userprofile`. Use print statements or logging to trace the import process. Additionally, consider using a virtual environment to isolate dependencies.
Are there any common pitfalls to avoid when importing modules in Python?
Common pitfalls include using incorrect module names, failing to install required packages, and not considering the order of imports. Always ensure that your Python environment is properly set up and that you are following best practices for module organization.
The ImportError related to the inability to import the name ‘UserProfile’ typically arises in Python when the specified module does not contain the defined class or function. This can occur due to various reasons, such as typos in the import statement, changes in the module structure, or the absence of the required class in the version of the library being used. It is essential to verify that the module being imported is correctly installed and that the class or function is present within it.
Another common cause of this error is circular imports, where two or more modules depend on each other, leading to a situation where Python cannot resolve the dependencies. To address this issue, developers should consider restructuring their code to avoid circular dependencies, possibly by consolidating related functions or classes into a single module.
Furthermore, ensuring that the correct version of the library is being utilized can prevent such import errors. Developers should check the documentation of the library for any updates or changes that might affect the availability of certain classes or functions. Using virtual environments can also help manage dependencies effectively and isolate project-specific requirements.
In summary, resolving the ImportError related to ‘UserProfile’ requires careful attention to the module structure, verification of installed libraries, and an understanding of
Author Profile
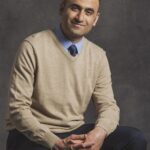
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?