Why Am I Seeing the Error: ‘Index Signature for Type ‘String’ is Missing in Type’?
In the world of TypeScript, where type safety and clarity reign supreme, developers often encounter a variety of errors that can be both perplexing and frustrating. One such error that can leave even seasoned programmers scratching their heads is the infamous “index signature for type ‘string’ is missing in type”. This seemingly cryptic message can halt progress and lead to confusion, especially for those new to the intricacies of TypeScript’s type system. Understanding this error is not just about fixing a bug; it’s about grasping the underlying principles of how TypeScript handles object types and their properties.
At its core, this error arises when TypeScript’s strict type-checking encounters an object that lacks the necessary structure to accommodate dynamic property access. In a language that prides itself on ensuring that the types of variables and objects are well-defined, the absence of an index signature can signal a mismatch between what the code expects and what it actually receives. This article delves into the nuances of index signatures, exploring why they are essential for certain types of objects and how they can enhance the robustness of your TypeScript code.
As we journey through the intricacies of this error, we’ll uncover practical strategies for defining index signatures and how to apply them effectively in your projects. Whether you’re debugging a complex
Understanding Index Signatures in TypeScript
Index signatures in TypeScript allow you to define the shape of an object when you don’t know all the properties in advance. This is particularly useful for objects that can hold a dynamic set of properties. An index signature is defined using a key type (usually `string` or `number`) followed by a value type.
For example:
“`typescript
interface StringDictionary {
[key: string]: string;
}
“`
In this example, any property name that is a string will have a value that is also a string. If you try to assign a value of a different type to a property, TypeScript will throw an error.
Common Errors Related to Index Signatures
One frequent error developers encounter is the message: “Index signature for type ‘string’ is missing in type.” This typically occurs when you attempt to assign an object that does not conform to the expected shape defined by an index signature.
Here are some common scenarios that might lead to this error:
- Missing Index Signature: If your object lacks the required index signature, TypeScript cannot guarantee that it will match the expected type.
- Type Mismatch: Even if an index signature is present, if the value types do not align, this error may arise.
- Incorrect Object Structure: If the object structure does not follow the defined interface, TypeScript will raise this error.
Example Scenarios
Consider the following example:
“`typescript
interface User {
name: string;
age: number;
[key: string]: string | number; // index signature
}
const user: User = {
name: “Alice”,
age: 30,
location: “Wonderland” // This is valid due to the index signature
};
“`
If you were to define the interface without the index signature:
“`typescript
interface UserWithoutIndex {
name: string;
age: number;
}
const userWithoutIndex: UserWithoutIndex = {
name: “Alice”,
age: 30,
location: “Wonderland” // Error: Index signature for type ‘string’ is missing in type ‘UserWithoutIndex’
};
“`
In this case, the error arises because `location` is not defined in the `UserWithoutIndex` interface.
Resolving the Error
To resolve the “Index signature for type ‘string’ is missing in type” error, consider the following approaches:
- Add an Index Signature: Modify your interface to include an index signature if you expect additional properties.
“`typescript
interface User {
name: string;
age: number;
[key: string]: string | number; // Add index signature
}
“`
- Ensure Proper Assignment: Verify that the object conforms to the defined type, ensuring all properties match the expected structure.
- Use a Type Assertion: If you are sure about the structure, you can use a type assertion, though this should be done cautiously.
“`typescript
const userWithAssertion = {
name: “Alice”,
age: 30,
location: “Wonderland”
} as User; // Type assertion
“`
Summary of Solutions
The following table summarizes how to address the index signature error:
Solution | Description |
---|---|
Add Index Signature | Include an index signature in the interface to allow dynamic properties. |
Check Object Structure | Ensure the object conforms to the defined interface without extra properties. |
Type Assertion | Use type assertions cautiously to bypass type checking when necessary. |
By understanding the role of index signatures and how to properly implement them, you can effectively manage dynamic object properties in TypeScript.
Understanding Index Signatures in TypeScript
Index signatures in TypeScript allow you to define the types of properties that an object can have when the property names are not known in advance. This feature is particularly useful for creating objects that can have dynamic keys while ensuring type safety.
- Syntax:
“`typescript
interface Example {
[key: string]: number; // ‘key’ is a string, and its value is a number
}
“`
- Key Points:
- The key type can be either `string` or `number`.
- The value type can be any valid TypeScript type (e.g., `string`, `number`, `boolean`, etc.).
- Index signatures can be combined with other properties in an interface.
Common Error: Missing Index Signature
The error message `index signature for type ‘string’ is missing in type` typically arises when an object is assigned a type that does not satisfy the index signature requirements. This often occurs in scenarios where an object is expected to have dynamic properties but is defined with a more rigid type.
- Example of the Error:
“`typescript
interface StrictObject {
a: number;
b: number;
}
const obj: StrictObject = {
a: 1,
b: 2,
c: 3 // Error: index signature for type ‘string’ is missing in type ‘StrictObject’
};
“`
Resolving the Error
To resolve the error, you need to ensure that the object conforms to the index signature defined in the expected type. You can do this by modifying the interface or type definition to include an index signature.
- Approach 1: Adding an Index Signature:
“`typescript
interface FlexibleObject {
[key: string]: number; // Adding index signature
a: number;
b: number;
}
const obj: FlexibleObject = {
a: 1,
b: 2,
c: 3 // Now valid
};
“`
- Approach 2: Using Record Utility Type:
If the structure is uniform, you can utilize the `Record` utility type:
“`typescript
const obj: Record
a: 1,
b: 2,
c: 3 // Valid
};
“`
Best Practices for Using Index Signatures
When using index signatures, adhere to the following best practices:
- Limit the Use: Use index signatures only when necessary to avoid overly permissive types.
- Document the Structure: Clearly document the expected structure of the objects to aid maintainability.
- Type Narrowing: Consider using type guards or discriminated unions for better type safety when dealing with potential variations in object shapes.
Practice | Description |
---|---|
Limit the Use | Restrict the use of index signatures to essential cases. |
Document the Structure | Provide clarity on expected types and properties. |
Type Narrowing | Utilize type guards to enhance type safety. |
By following these guidelines, you can effectively manage the flexibility that index signatures provide while maintaining robust type safety in TypeScript.
Understanding the ‘Index Signature’ Error in TypeScript
Dr. Emily Chen (Senior Software Engineer, TypeScript Innovations). The error message ‘index signature for type ‘string’ is missing in type’ typically arises when an object is expected to have a flexible structure but does not conform to the defined types. It is essential to ensure that your interfaces or types include an index signature if you intend to access properties dynamically.
Michael Thompson (Lead TypeScript Developer, CodeCraft Solutions). This error serves as a crucial reminder of TypeScript’s strict type-checking capabilities. When defining types, always consider whether an index signature is necessary. If you anticipate using dynamic keys, incorporating an index signature can prevent runtime errors and improve code maintainability.
Sarah Patel (Technical Architect, FutureTech Systems). Addressing the ‘index signature for type ‘string’ is missing in type’ error requires a clear understanding of how TypeScript handles object types. By explicitly defining an index signature, you can enhance the flexibility of your data structures while ensuring type safety, which is a fundamental principle of TypeScript.
Frequently Asked Questions (FAQs)
What does “index signature for type ‘string’ is missing in type” mean?
This error indicates that a TypeScript object type is expected to have an index signature for string keys, but it is not defined. Essentially, the object is being accessed with a string key that is not recognized by its type definition.
How can I resolve the “index signature for type ‘string’ is missing” error?
To resolve this error, you can either add an index signature to the type definition, allowing for dynamic keys, or ensure that you are only using keys that are explicitly defined in the type.
What is an index signature in TypeScript?
An index signature in TypeScript allows you to define the types of keys and values for an object, enabling the use of dynamic property names. It is defined using the syntax `[key: string]: valueType`.
Can I use a number as an index signature in TypeScript?
Yes, you can use a number as an index signature in TypeScript. However, it is important to note that when using a numeric index signature, the keys will be converted to strings.
What are the implications of adding an index signature to a type?
Adding an index signature to a type allows for greater flexibility in object properties but can also lead to less strict type checking, as any string key will be accepted. This can potentially introduce runtime errors if not managed carefully.
Are there performance concerns with using index signatures?
While using index signatures can simplify code and enhance flexibility, there may be performance implications in terms of type checking and object property access. It is advisable to use them judiciously, especially in performance-critical applications.
The error message “index signature for type ‘string’ is missing in type” typically arises in TypeScript when an object is expected to have a specific structure that includes an index signature, but the provided type does not meet this requirement. An index signature allows an object to have properties that are not explicitly defined in its type, enabling flexibility in the keys used. When this error occurs, it indicates that the TypeScript compiler has detected a mismatch between the expected type and the actual type being used.
This issue often surfaces in scenarios where developers attempt to access properties of an object using dynamic keys or when working with objects that should conform to certain interfaces. To resolve this error, developers can either define an index signature in the type definition or ensure that the object being used aligns with the expected structure. By doing so, they can maintain type safety while allowing for the dynamic nature of JavaScript objects.
In summary, understanding the role of index signatures in TypeScript is crucial for managing object types effectively. Developers should be aware of how to define these signatures to avoid common pitfalls associated with dynamic property access. By adhering to TypeScript’s type system, developers can create more robust and maintainable code, minimizing runtime errors and enhancing overall application stability.
Author Profile
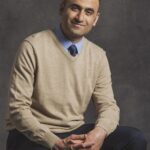
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?