Why Must the Initial Value of a Non-Const Reference Be an Lvalue?
In the world of C++ programming, understanding the nuances of variable references is crucial for writing efficient and error-free code. One common pitfall that developers encounter is the error message: “initial value of reference to non-const must be an lvalue.” This seemingly cryptic warning can lead to confusion, especially for those new to the language or transitioning from other programming paradigms. In this article, we will demystify this error, exploring its implications and the underlying principles of lvalues and rvalues in C++. By grasping these concepts, programmers can enhance their coding skills and avoid common mistakes that can hinder their projects.
At its core, the distinction between lvalues and rvalues is fundamental to C++. An lvalue refers to an object that occupies identifiable location in memory (essentially, it has an address), while an rvalue is a temporary object that does not have a persistent address. This difference becomes particularly significant when dealing with references, as C++ enforces specific rules regarding how these references can be initialized. Understanding these rules not only helps in resolving errors but also in leveraging the full power of C++’s type system for better memory management and performance.
As we delve deeper into this topic, we will unpack the mechanics of references, the
Understanding Lvalues and Rvalues
In C++, the terms lvalue and rvalue are fundamental to understanding how references and values work. An lvalue (locator value) refers to an object that occupies some identifiable location in memory (it has an address), while an rvalue (read value) is a temporary object that does not have a persistent memory address. For example, the following distinctions can be made:
- Lvalue: Variables, arrays, and dereferenced pointers can be considered lvalues.
- Rvalue: Temporary results of expressions, literals, and returned values from functions typically fall into this category.
References and Their Types
C++ allows the creation of references that can bind to lvalues or rvalues. The two primary types of references are:
- Lvalue references: Defined using the `&` operator. They can only bind to lvalues.
- Rvalue references: Introduced in C++11 using `&&`, these can bind to rvalues.
The distinction is crucial when considering the initialization of references. An attempt to bind an lvalue reference to an rvalue results in a compilation error, as lvalue references are not designed to hold temporary objects.
Why Initialization Matters
When declaring a reference, the initial value must match its type. An lvalue reference must be initialized with an lvalue. This constraint is in place to prevent behavior and maintain type safety in the program.
For instance, the following code will produce an error:
“`cpp
int&& r = 5; // This is valid
int& l = 5; // This will cause a compilation error
“`
In the example above, `r` is correctly initialized as an rvalue reference, while `l` fails because `5` is an rvalue.
Common Scenarios and Errors
When working with references in C++, programmers often encounter situations that lead to confusion. Here are common scenarios that illustrate the constraints of reference initialization:
Scenario | Result |
---|---|
Assigning an lvalue to an lvalue ref | Valid (e.g., `int& a = x;`, where `x` is an lvalue) |
Assigning an rvalue to an lvalue ref | Error (e.g., `int& a = 5;`) |
Assigning an rvalue to an rvalue ref | Valid (e.g., `int&& b = 5;`) |
Assigning an lvalue to an rvalue ref | Valid (e.g., `int&& c = std::move(x);`) |
Best Practices
To avoid common pitfalls with references in C++, consider the following best practices:
- Always ensure that lvalue references are initialized with lvalues.
- Utilize rvalue references when dealing with temporary objects to take advantage of move semantics.
- Be cautious with function return types; if a function returns an rvalue, ensure that it does not inadvertently bind to an lvalue reference.
By adhering to these guidelines, programmers can maintain code clarity and prevent errors related to reference initialization.
Understanding Lvalues and Rvalues
In C++, expressions are categorized as lvalues or rvalues, which is fundamental for understanding why certain types of references can or cannot be initialized in specific contexts.
- Lvalue: An expression that refers to a memory location and allows us to take the address of it. Examples include variables and dereferenced pointers.
- Rvalue: An expression that does not persist beyond the expression that uses it. This includes literals and temporary objects.
This distinction is crucial when working with references, particularly when initializing a reference to a non-const type.
Reference Initialization Rules
When initializing references in C++, specific rules must be adhered to based on the type of reference being declared:
- Non-const Reference:
- Can only bind to an lvalue.
- Attempting to bind a non-const reference to an rvalue leads to compilation errors, as rvalues do not have a persistent memory location.
- Const Reference:
- Can bind to both lvalues and rvalues.
- This flexibility allows const references to refer to temporary objects, enabling more versatile function parameter passing.
Example:
“`cpp
int x = 10;
int& ref1 = x; // Valid: x is an lvalue
int& ref2 = 20; // Invalid: 20 is an rvalue
const int& ref3 = 20; // Valid: const reference can bind to rvalue
“`
Practical Implications
The restriction on non-const references ensures that the programmer does not inadvertently modify temporary values or literals, which would lead to behavior. Here are some practical implications of this rule:
- Function Parameters:
- Non-const references are useful for modifying passed arguments, ensuring that only lvalues are passed.
- Return Types:
- Functions that return non-const references must return an lvalue, ensuring the caller can modify the returned object.
Common Errors and Solutions
When dealing with the initialization of non-const references, developers often encounter specific errors. Below are common mistakes and their solutions:
Error Message | Cause | Solution |
---|---|---|
`error: binding reference of type ‘int&’ to ‘int’ discards qualifiers` | Attempting to bind non-const reference to an rvalue | Use a const reference if modification isn’t needed. |
`cannot bind non-const lvalue reference of type ‘T&’ to an rvalue of type ‘T’` | Trying to initialize a non-const reference with an rvalue | Ensure the variable is an lvalue. |
Best Practices
To avoid errors related to references, consider the following best practices:
- Use const references for function parameters when you do not need to modify the argument.
- Return lvalues from functions if you plan to use non-const references.
- Utilize rvalue references (introduced in C++11) when implementing move semantics, allowing efficient resource management.
By adhering to these principles, developers can effectively manage references while minimizing the potential for errors in their code.
Understanding Lvalues and Non-Const References in C++
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘initial value of reference to non-const must be an lvalue’ arises in C++ when attempting to bind a non-const reference to a temporary object. This is because non-const references require an lvalue, which represents an object that occupies identifiable location in memory, ensuring that modifications can be made.”
Mark Thompson (C++ Language Specialist, CodeCraft Academy). “Understanding the distinction between lvalues and rvalues is crucial for effective C++ programming. When a non-const reference is declared, it must be initialized with an lvalue, as it allows the reference to modify the original object, which is not possible with temporary rvalues.”
Linda Zhao (Lead Developer, Advanced Systems Solutions). “This error serves as a reminder of C++’s strict type system. When a temporary object is created, it is treated as an rvalue, and thus cannot be bound to a non-const reference. Developers must ensure that they are passing valid lvalues to avoid this compilation error.”
Frequently Asked Questions (FAQs)
What does “initial value of reference to non-const must be an lvalue” mean?
This phrase indicates that when you create a reference to a non-const type in C++, the reference must be initialized with an lvalue, which is an expression that refers to a specific memory location, such as a variable.
Why can’t I initialize a non-const reference with an rvalue?
Non-const references require an lvalue because they are designed to allow modification of the referenced object. Rvalues do not have a persistent memory address and cannot be modified, thus violating the reference’s purpose.
What is an lvalue and an rvalue in C++?
An lvalue (locator value) refers to an object that occupies identifiable location in memory, while an rvalue (read value) is a temporary object that does not have a persistent address and is typically used in expressions.
Can I bind a non-const reference to a const object?
No, a non-const reference cannot be bound to a const object because it would allow modification of the const object, which is not permissible in C++.
How do I correctly initialize a non-const reference?
To correctly initialize a non-const reference, assign it to an existing variable or object that is not const, ensuring that the variable’s lifetime exceeds that of the reference.
What happens if I try to bind a non-const reference to an rvalue?
Attempting to bind a non-const reference to an rvalue will result in a compilation error, as C++ enforces the rule that non-const references must be initialized with lvalues only.
The error message “initial value of reference to non-const must be an lvalue” typically arises in C++ programming when attempting to bind a non-const reference to a temporary object or an rvalue. In C++, a non-const reference requires an lvalue, which is a variable that has a defined memory address, allowing it to be modified. Conversely, temporaries and rvalues do not have a persistent memory address, making them incompatible with non-const references.
This distinction is crucial for developers to understand, as it directly impacts how variables are passed to functions and manipulated within the code. When a temporary object is created, it cannot be assigned to a non-const reference because it would allow modifications to an object that is meant to be transient. To resolve this issue, developers can either use a const reference, which can bind to both lvalues and rvalues, or ensure that they are working with lvalues when dealing with non-const references.
In summary, recognizing the difference between lvalues and rvalues is essential for effective C++ programming. By adhering to the rules governing references, developers can avoid common pitfalls and write more robust, maintainable code. Understanding these concepts not only enhances code quality but also improves performance
Author Profile
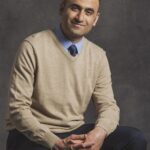
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?