Why Did the Injection of Autowired Dependencies Fail?
In the world of modern software development, dependency injection has emerged as a cornerstone of effective application design, particularly within frameworks like Spring. However, even seasoned developers can encounter a perplexing issue: the injection of autowired dependencies failed. This seemingly innocuous error can halt progress and lead to frustration, as it disrupts the seamless integration of components that is essential for robust application functionality. Understanding the nuances of this problem is crucial for developers aiming to build efficient and maintainable systems.
At its core, the failure of autowired dependency injection often stems from misconfigurations or misunderstandings of the underlying principles of dependency management. Factors such as incorrect bean definitions, scope mismatches, or even circular dependencies can contribute to this issue, leading to runtime exceptions that can be difficult to diagnose. Additionally, the rapid evolution of frameworks and libraries means that developers must stay informed about best practices and common pitfalls to avoid falling into the trap of dependency injection failures.
As we delve deeper into the intricacies of autowired dependencies, we will explore the common causes of injection failures, practical strategies for troubleshooting, and best practices for ensuring smooth dependency management in your applications. By equipping yourself with this knowledge, you can enhance your development skills and build more resilient software solutions that stand the test of time.
Common Causes of Dependency Injection Failures
The failure of dependency injection, specifically autowired dependencies, can often be attributed to several common issues. Understanding these causes is crucial for diagnosing and resolving problems effectively. The following are frequent culprits:
- Missing Bean Definitions: If a required bean is not defined in the Spring context, the framework will not be able to inject it.
- Incompatible Types: When the type of the autowired bean does not match the expected type, Spring will fail to perform the injection.
- Ambiguous Beans: If there are multiple beans of the same type, Spring will be unable to determine which bean to inject, resulting in a failure.
- Incorrect Scope: Beans with incompatible scopes (e.g., a singleton bean trying to inject a prototype bean) can cause injection issues.
- Component Scanning Issues: If the package containing the class is not scanned by Spring, the necessary beans will not be registered.
Identifying and Resolving Issues
To effectively troubleshoot dependency injection failures, follow a systematic approach to identify the root cause. The steps below can guide developers in resolving these issues:
- Check Application Context Configuration: Ensure that the application context is correctly configured and that all necessary beans are defined.
- Review Autowired Annotations: Verify that the `@Autowired` annotations are correctly placed and that the fields or constructors are appropriately set up.
- Examine Bean Scopes: Ensure that the scopes of your beans are compatible. For example, injecting a prototype bean into a singleton can lead to issues.
- Look for Errors in Logs: Check the application’s logs for any error messages related to dependency injection. These logs often provide valuable insight into the exact nature of the problem.
- Use `@Qualifier` Annotation: In cases of ambiguity, utilize the `@Qualifier` annotation to specify the exact bean to inject.
Issue | Symptoms | Resolution |
---|---|---|
Missing Bean | NullPointerException | Define the missing bean in the application context. |
Type Mismatch | ClassCastException | Ensure that the types match between the field and the bean. |
Ambiguous Beans | UnsatisfiedDependencyException | Use @Qualifier to specify which bean to inject. |
Scope Conflicts | BeanCreationException | Adjust the bean scopes to be compatible. |
Best Practices for Dependency Injection
To minimize the likelihood of injection failures, consider the following best practices:
- Use Constructor Injection: Prefer constructor injection over field injection, as it ensures that the dependencies are provided at the time of object creation.
- Define Beans Explicitly: Although component scanning is convenient, defining beans explicitly can help avoid issues related to package scanning.
- Limit Autowiring: Use autowiring sparingly and only when necessary. Explicitly defining dependencies can improve code readability and maintainability.
- Document Dependencies: Clearly document the dependencies of your classes to facilitate easier debugging and maintenance.
By following these practices and maintaining a clear understanding of the dependency injection mechanism, developers can significantly reduce the occurrence of injection failures in their applications.
Common Causes of Injection Failures
Injection of autowired dependencies can fail due to several reasons, often stemming from configuration issues, missing components, or incorrect annotations. The following are common causes:
- Missing Bean Definitions: The required beans are not defined in the application context.
- Incorrect Scope: The scope of the beans does not match the requirements (e.g., a singleton bean being injected into a prototype).
- Classpath Issues: The required classes are not available in the classpath, leading to failures in bean creation.
- Circular Dependencies: Two or more beans depend on each other, causing a circular reference that Spring cannot resolve.
- Qualifiers Not Used: When multiple beans of the same type exist, and the required bean is not specified using `@Qualifier`.
- Component Scanning Issues: The packages containing the beans are not scanned, leading to unrecognized classes.
Debugging Techniques
When faced with dependency injection failures, the following debugging techniques can be employed:
- Check Application Context: Verify that all required beans are listed in the application context by reviewing the logs on startup.
- Use `@Autowired(required = )`: This allows the injection to proceed even if the bean is not available, helping to identify the problem without failing the application.
- Enable Debug Logging: Set the logging level to DEBUG for Spring to get detailed information about bean creation and injection processes.
- Review Annotations: Ensure that the correct annotations (`@Component`, `@Service`, `@Repository`, `@Controller`) are being used appropriately.
Best Practices for Dependency Injection
To minimize issues related to dependency injection, consider the following best practices:
- Consistent Use of Annotations: Always use the same annotations for similar components to avoid confusion.
- Define Interfaces: Use interfaces for your beans to promote loose coupling and easier testing.
- Explicit Configuration: Define your beans in a configuration class or XML file to avoid ambiguity.
- Avoid Circular Dependencies: Refactor your code to eliminate circular dependencies, possibly using setter injection.
- Testing with Mocks: Utilize mocking frameworks like Mockito for testing components in isolation.
Resolving Common Issues
The following table summarizes common dependency injection issues and their resolutions:
Issue | Description | Resolution |
---|---|---|
Missing Bean Definition | Required bean is not defined in the context | Define the bean using `@Component`, etc. |
Circular Dependency | Beans depend on each other, causing a loop | Refactor code to remove circular references |
Incorrect Qualifier | Multiple beans of the same type without specification | Use `@Qualifier` to specify the desired bean |
Bean Not Found | Bean class not in classpath | Ensure the class is included in the build path |
Scope Mismatch | Scope of the injected bean does not match | Align the scopes of beans appropriately |
Advanced Troubleshooting Techniques
For more complex scenarios, consider these advanced troubleshooting techniques:
- Profile Specific Beans: Use Spring Profiles to define beans for different environments and isolate issues in specific configurations.
- Custom Annotations: Create custom annotations to encapsulate complex configurations and maintain clarity.
- Dependency Graph Analysis: Use tools like Spring’s ApplicationContext to visualize the dependency graph and identify broken links.
By applying these techniques, developers can effectively address issues related to the injection of autowired dependencies, leading to more robust and maintainable applications.
Understanding the Challenges of Autowired Dependency Injection
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The failure of autowired dependencies often stems from misconfigurations within the Spring context. Developers must ensure that all required beans are correctly defined and available in the application context to avoid runtime exceptions.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “Common pitfalls in autowired dependency injection include circular dependencies and missing component annotations. It is crucial to analyze the dependency graph to identify any issues that may prevent successful injection.”
Sarah Lee (Java Framework Consultant, DevExpertise). “When encountering injection failures, developers should leverage debugging tools to trace the lifecycle of beans. Understanding the application context and bean scopes can significantly aid in resolving these issues effectively.”
Frequently Asked Questions (FAQs)
What does “injection of autowired dependencies failed” mean?
This error indicates that the Spring framework was unable to automatically inject a required bean into a component, typically due to configuration issues or missing dependencies.
What are common causes for autowired dependency injection failures?
Common causes include missing bean definitions, incorrect component scanning configurations, circular dependencies, or mismatched bean types in the application context.
How can I troubleshoot autowired dependency injection issues?
To troubleshoot, check the application context for bean definitions, verify that the required beans are annotated correctly, and ensure that component scanning is configured properly. Additionally, review logs for specific error messages.
What is the role of the @Autowired annotation in Spring?
The @Autowired annotation is used to indicate that a dependency should be injected automatically by the Spring container, allowing for loose coupling between components.
Can I use @Autowired with constructor injection?
Yes, @Autowired can be used with constructor injection. This approach is often preferred as it allows for mandatory dependencies to be enforced at the time of object creation.
What should I do if I encounter a circular dependency?
To resolve circular dependencies, consider refactoring your code to eliminate the circular reference, use setter injection instead of constructor injection, or utilize the `@Lazy` annotation to delay the injection until necessary.
The failure of injection of autowired dependencies is a common issue encountered in Spring Framework applications. This problem typically arises when the Spring container is unable to locate or instantiate the required beans that are marked for autowiring. Various factors can contribute to this failure, including misconfiguration of the application context, incorrect component scanning, or the presence of multiple beans of the same type without proper qualification. Understanding the root causes is essential for effectively resolving these issues.
One of the key insights is the importance of proper configuration and the use of annotations such as `@Component`, `@Service`, `@Repository`, and `@Controller` to ensure that the Spring container recognizes and manages the beans correctly. Additionally, utilizing `@Autowired` with the correct qualifiers can help in scenarios where multiple beans of the same type exist. It is also advisable to check the application context configuration for any discrepancies that may lead to dependency injection failures.
Moreover, leveraging Spring’s debugging capabilities, such as enabling debug logs, can provide valuable insights into the bean creation process and highlight any issues that may arise during autowiring. Understanding the lifecycle of beans and the context in which they are created is crucial for diagnosing and resolving injection failures. By adopting best practices in configuration and being
Author Profile
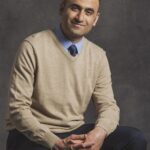
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?