How to Resolve the ‘Invalid Literal for Int with Base 10’ Error in Python?
In the world of programming, few errors can be as perplexing as the infamous “invalid literal for int() with base 10” in Python. This seemingly cryptic message often leaves developers scratching their heads, wondering what went wrong in their code. As Python continues to be a popular choice for both beginners and seasoned developers, understanding this error is essential for anyone looking to harness the full power of the language. Whether you’re parsing user input, reading data from files, or performing calculations, this error can pop up unexpectedly, derailing your program’s flow.
At its core, this error arises when Python encounters a string or a character that it cannot convert into an integer, leading to confusion and frustration. It serves as a reminder of the importance of data types and validation in programming. As we delve deeper into the intricacies of this error, we will explore common scenarios that trigger it, the underlying reasons for its occurrence, and effective strategies to prevent it from disrupting your coding journey. By the end of this article, you will not only understand the “invalid literal for int() with base 10” error but also be equipped with the tools to handle it gracefully, ensuring smoother coding experiences in the future.
Understanding the Error
The error message “invalid literal for int() with base 10” in Python occurs when you attempt to convert a string or another type of data into an integer using the `int()` function, but the string does not represent a valid integer. This typically happens when the string contains non-numeric characters, whitespace, or is empty.
Common Causes
- Non-numeric Characters: Any characters in the string that are not digits will lead to this error.
- Leading or Trailing Whitespace: Spaces before or after the number can cause the conversion to fail.
- Empty Strings: Attempting to convert an empty string will raise this error.
- Special Characters: Symbols such as commas, dollar signs, or periods (when not used for decimal points) in the string will also trigger the error.
Examples of the Error
To illustrate, consider the following examples that would generate this error:
“`python
int(“abc”) Raises ValueError
int(“123abc”) Raises ValueError
int(” 123 “) Works fine after stripping whitespace
int(“”) Raises ValueError
int(“12.34”) Raises ValueError
int(“$100”) Raises ValueError
“`
Handling the Error
To avoid encountering this error, you can implement several strategies:
- Input Validation: Check if the input is numeric before converting.
- Use Try-Except Blocks: This allows graceful handling of the error.
Input Validation Example
You can validate input using the `str.isdigit()` method, which checks if all characters in the string are digits.
“`python
user_input = “123”
if user_input.isdigit():
number = int(user_input)
else:
print(“Invalid input: Not a valid integer.”)
“`
Using Try-Except Blocks
Here is how you can use a try-except block to catch the error:
“`python
user_input = “abc”
try:
number = int(user_input)
except ValueError:
print(“Invalid input: Please enter a valid integer.”)
“`
Best Practices
To ensure robust code and minimize the occurrence of this error, consider the following best practices:
- Sanitize Input: Always clean your input to remove unwanted characters.
- User Feedback: Provide clear messages to users when their input is invalid.
- Logging Errors: Log errors for debugging purposes without crashing the application.
Summary of Best Practices
Practice | Description |
---|---|
Sanitize Input | Remove unwanted characters from user input. |
User Feedback | Inform users of invalid input with clear messages. |
Logging | Log errors to assist in debugging while maintaining application stability. |
By following these practices, you can handle integer conversion in Python more effectively and reduce the likelihood of encountering the “invalid literal for int() with base 10” error.
Understanding the Error
The error message “invalid literal for int() with base 10” indicates that Python’s `int()` function is attempting to convert a string to an integer, but the string contains characters that are not valid for the conversion. This is commonly encountered in scenarios where the input data is not sanitized or is improperly formatted.
Common Causes
- Non-numeric Characters: The string includes alphabetic characters, symbols, or whitespace.
- Empty Strings: An empty string is passed to `int()`, which cannot be converted.
- Improper Formatting: Strings formatted with commas or other non-numeric symbols are passed without appropriate cleaning.
Example Scenarios
- Direct Conversion Attempt:
“`python
value = int(“123abc”) Raises ValueError
“`
- Empty String:
“`python
value = int(“”) Raises ValueError
“`
- Comma-Separated Values:
“`python
value = int(“1,000”) Raises ValueError
“`
Handling the Error
To effectively handle this error, consider implementing the following strategies:
Input Validation
- Use regular expressions to ensure that strings contain only numeric characters before attempting conversion.
- Employ `str.isdigit()` to check if a string is composed entirely of digits.
Example Implementation
“`python
def safe_int_conversion(input_string):
if input_string.strip().isdigit():
return int(input_string)
else:
raise ValueError(“Invalid input: cannot convert to integer.”)
“`
Try-Except Block
Utilize a try-except block to gracefully handle potential errors during conversion.
“`python
input_string = “123abc”
try:
value = int(input_string)
except ValueError as e:
print(f”Error: {e}”)
“`
Cleaning Input Data
If your input data may contain non-numeric characters, consider cleaning it before conversion.
- Remove Non-Numeric Characters:
“`python
import re
def clean_and_convert(input_string):
cleaned_string = re.sub(r’\D’, ”, input_string) Removes non-digit characters
return int(cleaned_string) if cleaned_string else 0 Default to 0 if empty
“`
Debugging Tips
When encountering the “invalid literal for int() with base 10” error, follow these tips for effective debugging:
- Print the Input: Always print the value that is being converted to see its contents.
- Check Data Types: Ensure that the variable is indeed a string before conversion.
- Log Errors: Implement logging to capture and analyze recurring issues.
Example Debugging Code
“`python
input_string = “abc123″
try:
value = int(input_string)
except ValueError:
print(f”Failed to convert ‘{input_string}’ to an integer.”)
“`
By understanding the causes, handling techniques, and debugging methods associated with the “invalid literal for int() with base 10” error, developers can ensure more robust and error-free Python applications. Proper input validation and error handling are essential practices for maintaining code quality.
Understanding the ‘Invalid Literal for Int with Base 10’ Error in Python
Dr. Emily Chen (Senior Software Engineer, CodeFix Solutions). This error typically arises when a string that cannot be converted to an integer is passed to the int() function. It is crucial to validate input data before attempting conversion to ensure that it adheres to expected formats.
Michael Patel (Data Scientist, Analytics Hub). Handling this error effectively involves implementing exception handling in your code. Using try-except blocks allows developers to manage unexpected input gracefully, providing users with informative feedback rather than a program crash.
Sarah Thompson (Python Developer, Tech Innovators). To prevent the ‘invalid literal for int’ error, it is advisable to sanitize and preprocess input data. Techniques such as stripping whitespace and checking for non-numeric characters can significantly reduce the likelihood of encountering this issue during runtime.
Frequently Asked Questions (FAQs)
What does the error “invalid literal for int() with base 10” mean in Python?
This error indicates that the string being passed to the `int()` function cannot be converted to an integer because it contains non-numeric characters.
What types of inputs can cause this error?
Inputs such as strings containing letters, special characters, or whitespace will trigger this error when attempting to convert them to integers.
How can I resolve the “invalid literal for int() with base 10” error?
To resolve this error, ensure that the string being converted to an integer contains only valid numeric characters. You can also use error handling techniques, such as `try` and `except`, to manage invalid inputs gracefully.
Can I convert floating-point numbers using the int() function?
Yes, you can convert floating-point numbers to integers using the `int()` function, but it will truncate the decimal part, not round it. For example, `int(3.7)` will return `3`.
Is there a way to check if a string can be converted to an integer before attempting the conversion?
Yes, you can use the `str.isdigit()` method to check if a string consists solely of digits. However, this method does not account for negative numbers or decimal points, so additional checks may be necessary.
What should I do if I need to handle user input that may cause this error?
You should validate user input by using functions like `str.isdigit()` or implementing a `try` and `except` block to catch the `ValueError` that arises from invalid conversions. This will allow you to prompt the user for valid input without crashing the program.
The error message “invalid literal for int() with base 10” in Python indicates that the program attempted to convert a string or other non-integer type into an integer but failed because the string does not represent a valid integer. This commonly occurs when the input contains non-numeric characters, such as letters or symbols, or when the string is empty. Understanding the context in which this error arises is crucial for effective debugging and error handling in Python applications.
To resolve this issue, developers should ensure that the input being converted to an integer is indeed a valid numeric string. This can be achieved through various methods, such as using exception handling with try-except blocks to catch the ValueError that is raised when the conversion fails. Additionally, validating input before conversion, such as using regular expressions or built-in string methods, can help prevent this error from occurring in the first place.
Another important takeaway is the significance of user input sanitization. When accepting user input, it is essential to implement checks that confirm the data’s integrity before processing it. This not only helps in avoiding the “invalid literal” error but also enhances the overall robustness and security of the application. By adopting best practices for input validation and error handling, developers can create more
Author Profile
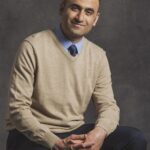
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?