Why Am I Seeing the Error ‘Invalid Plugin Specified in Babel Options: Proposal-Dynamic-Import’?
In the ever-evolving landscape of JavaScript development, tools and libraries play a crucial role in shaping the efficiency and effectiveness of our coding practices. Among these tools, Babel stands out as a powerful transpiler that enables developers to write modern JavaScript while ensuring compatibility with older environments. However, as with any sophisticated tool, users may encounter challenges along the way. One such challenge is the perplexing error message: “invalid plugin specified in babel options: proposal-dynamic-import.” For many developers, this error can be a frustrating roadblock, hindering their progress and productivity.
In this article, we will delve into the intricacies of Babel’s plugin system and explore the common pitfalls that lead to this specific error. We’ll unpack the significance of the `proposal-dynamic-import` plugin, its role in enhancing JavaScript functionality, and the reasons why it might not be recognized by Babel in certain configurations. By understanding the underlying causes of this error, developers can not only resolve the issue but also gain insights into optimizing their Babel setup for smoother development experiences.
Join us as we navigate through the nuances of Babel configuration, shedding light on best practices and troubleshooting techniques that will empower you to overcome this hurdle and harness the full potential of modern JavaScript features. Whether you’re a
Understanding Babel and Plugins
Babel is a widely-used JavaScript compiler that allows developers to use the latest JavaScript features by transpiling code to a version compatible with older browsers. This process is facilitated through the use of plugins, which extend Babel’s capabilities. Each plugin serves a specific purpose, such as transforming syntax or enabling experimental features.
When configuring Babel, developers specify these plugins in the Babel configuration file (e.g., `.babelrc`, `babel.config.js`). If an invalid plugin is referenced, such as `proposal-dynamic-import`, an error occurs, indicating that the specified plugin is not recognized.
Common Causes of Invalid Plugin Errors
Invalid plugin errors can arise from several issues:
- Plugin Not Installed: The plugin may not be included in the project’s dependencies.
- Typographical Error: A typo in the plugin name can lead to Babel being unable to locate it.
- Version Incompatibility: The plugin may not be compatible with the installed version of Babel.
- Incorrect Configuration: The plugin might be placed in the wrong configuration section.
Resolving the Invalid Plugin Error
To resolve the error regarding the invalid plugin specified in Babel options, consider the following steps:
- Install the Plugin: Ensure that the required plugin is installed. For example, to install the `proposal-dynamic-import` plugin, use the following command:
“`bash
npm install –save-dev @babel/plugin-syntax-dynamic-import
“`
- Verify Plugin Name: Double-check the spelling and ensure that the plugin name matches the official documentation.
- Check Babel Version: Review the version of Babel and its plugins. Some plugins may only work with specific versions. Update Babel if necessary:
“`bash
npm install –save-dev @babel/core@latest
“`
- Review Configuration: Ensure the plugin is correctly listed in the Babel configuration file. It should look similar to this:
“`json
{
“plugins”: [
“@babel/plugin-syntax-dynamic-import”
]
}
“`
Plugin Configuration Example
Below is an example of a complete Babel configuration using the `proposal-dynamic-import` plugin:
File | Content |
---|---|
.babelrc |
{ “presets”: [“@babel/preset-env”], “plugins”: [ “@babel/plugin-syntax-dynamic-import” ] } |
By following these steps and ensuring proper configuration, developers can effectively resolve the error associated with invalid plugins in Babel options. This will facilitate the seamless use of modern JavaScript features and improve overall code quality.
Understanding the Error
The error message `invalid plugin specified in babel options: proposal-dynamic-import` indicates that Babel is unable to recognize the specified plugin for dynamic imports. This typically arises from misconfigurations in the Babel setup or the absence of the necessary plugin.
Common Causes
- Plugin Not Installed: The required plugin for dynamic imports is not installed in your project.
- Incorrect Configuration: The Babel configuration file (e.g., `.babelrc`, `babel.config.js`) incorrectly references the plugin.
- Version Mismatch: The installed version of Babel may not support the specified plugin, or there may be a compatibility issue with other plugins.
How to Resolve the Issue
To address the error, consider the following steps:
Install the Plugin
Ensure that the required plugin is installed. You can add it using npm or yarn:
“`bash
npm install –save-dev @babel/plugin-syntax-dynamic-import
“`
or
“`bash
yarn add –dev @babel/plugin-syntax-dynamic-import
“`
Update Babel Configuration
Modify your Babel configuration file to include the newly installed plugin. Here’s an example of how to do this:
.babelrc:
“`json
{
“plugins”: [
“@babel/plugin-syntax-dynamic-import”
]
}
“`
babel.config.js:
“`javascript
module.exports = {
plugins: [
‘@babel/plugin-syntax-dynamic-import’
]
};
“`
Check Version Compatibility
Ensure that you are using compatible versions of Babel and its plugins. You can check your current Babel version using:
“`bash
npm list @babel/core
“`
Refer to the [Babel documentation](https://babeljs.io/docs/en/plugins) for information on compatibility and updates.
Verifying Your Setup
After making the necessary changes, it’s essential to verify that your setup is correct. Follow these steps:
– **Restart Your Development Server**: Sometimes, changes do not take effect until the server is restarted.
– **Run Babel in Debug Mode**: Use the debug mode to check for any additional configuration issues.
– **Test Dynamic Imports**: Create a simple dynamic import in your code and see if the error persists.
“`javascript
import(/* webpackChunkName: “my-chunk-name” */ ‘./my-module’)
.then(module => {
// Use the imported module
})
.catch(err => {
console.error(‘Error loading module:’, err);
});
“`
Additional Considerations
If the issue remains unresolved, consider the following:
- Consult the Community: Search platforms like Stack Overflow or GitHub for similar issues raised by other developers.
- Review Documentation: Check the Babel and plugin documentation for any recent changes or migration guides.
- Upgrade Dependencies: Sometimes, upgrading Babel and related plugins to their latest versions can resolve compatibility issues.
Action | Description |
---|---|
Install the Plugin | Ensure the plugin is installed correctly. |
Update Configuration | Modify your Babel config to include the plugin. |
Check Versions | Verify compatibility between Babel and plugins. |
Restart Development Server | Apply changes and test again. |
Use Debug Mode | Identify any configuration issues. |
Test Imports | Ensure dynamic imports work as expected. |
This systematic approach will aid in resolving the `invalid plugin specified in babel options: proposal-dynamic-import` error effectively.
Understanding Babel Configuration Errors: Insights from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘invalid plugin specified in babel options: proposal-dynamic-import’ typically arises when the Babel configuration file references a plugin that is either not installed or incorrectly named. It is crucial for developers to ensure that all necessary plugins are included in their project dependencies to avoid such issues.”
Michael Chen (Frontend Development Specialist, CodeCraft Academy). “When encountering the ‘invalid plugin specified in babel options’ error, I recommend checking the version compatibility of the Babel plugins being used. Sometimes, plugins like ‘proposal-dynamic-import’ may have breaking changes or require specific versions of Babel itself, leading to configuration conflicts.”
Sarah Patel (JavaScript Framework Consultant, WebDev Insights). “To resolve the ‘invalid plugin specified in babel options’ error, developers should verify their Babel configuration files for typos or outdated plugin names. Additionally, consulting the official Babel documentation can provide clarity on the correct usage and installation of plugins like ‘proposal-dynamic-import’.”
Frequently Asked Questions (FAQs)
What does the error “invalid plugin specified in babel options: proposal-dynamic-import” mean?
This error indicates that Babel is unable to recognize the specified plugin, `proposal-dynamic-import`, in your configuration. This typically happens when the plugin is not installed or is incorrectly referenced in the Babel configuration file.
How can I resolve the “invalid plugin specified in babel options: proposal-dynamic-import” error?
To resolve this error, ensure that you have installed the necessary Babel plugin by running `npm install –save-dev @babel/plugin-syntax-dynamic-import`. After installation, verify that your Babel configuration correctly includes the plugin.
Is the `proposal-dynamic-import` plugin still necessary for Babel 7 and above?
In Babel 7 and later versions, the `proposal-dynamic-import` plugin is no longer required for dynamic imports since they are part of the ECMAScript specification. However, if you are using features that require the plugin, ensure it is correctly installed and configured.
Where should I add the `proposal-dynamic-import` plugin in my Babel configuration?
You should add the `proposal-dynamic-import` plugin to the `plugins` array in your Babel configuration file (e.g., `.babelrc`, `babel.config.js`). For example:
“`json
{
“plugins”: [“@babel/plugin-syntax-dynamic-import”]
}
“`
What are the common causes of the “invalid plugin specified” error?
Common causes include not having the plugin installed, a typo in the plugin name, or using an outdated version of Babel that does not support the specified plugin. Always check your package.json and Babel configuration for accuracy.
How do I check if the `proposal-dynamic-import` plugin is installed?
You can check if the plugin is installed by looking in your `package.json` file under `devDependencies` or by running `npm list @babel/plugin-syntax-dynamic-import` in your project directory. If it is not listed, you need to install it.
The error message “invalid plugin specified in babel options: proposal-dynamic-import” typically indicates that the Babel configuration is attempting to use a plugin that is either not installed or incorrectly referenced. This specific plugin is part of the Babel ecosystem, which allows developers to use the dynamic import syntax in their JavaScript code. Dynamic imports enable asynchronous module loading, which can enhance the performance and organization of applications by loading modules only when they are needed.
To resolve this issue, developers should ensure that the necessary Babel plugin for dynamic imports is installed. This can be done by running the appropriate npm or yarn command to install the `@babel/plugin-syntax-dynamic-import` package. Additionally, it is essential to verify that the Babel configuration file (such as `.babelrc` or `babel.config.js`) correctly references the plugin. Misconfigurations or typos in the plugin name can also lead to this error.
Another important aspect to consider is the version compatibility between Babel and the plugins being used. Developers should check that their Babel core and plugin versions are compatible with each other. Keeping dependencies updated can prevent such errors and ensure that developers can leverage the latest features and improvements in the Babel ecosystem.
In summary, addressing the “invalid
Author Profile
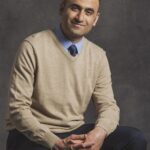
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?