Why Am I Seeing an ‘Invalid Use of Non-Static Member Function’ Error?
In the world of programming, particularly in object-oriented languages like C++, the distinction between static and non-static member functions can often lead to confusion and frustration for developers. One common pitfall that many encounter is the dreaded error message: “invalid use of non-static member function.” This seemingly cryptic notification can halt a programmer’s progress, leaving them to navigate the intricacies of class design and function invocation. Understanding the underlying principles of this error not only aids in debugging but also enhances one’s grasp of object-oriented programming concepts.
When a non-static member function is called incorrectly, it often stems from a misunderstanding of how these functions relate to instances of a class. Unlike static member functions, which can be invoked without an object context, non-static member functions require an instance of the class to operate on. This distinction is crucial, as it affects how data is accessed and manipulated within the program. In this article, we will delve into the nuances of this error, exploring its causes and implications while providing clarity on how to effectively manage member functions in your code.
As we unravel the complexities of the “invalid use of non-static member function” error, we will highlight best practices for defining and invoking member functions correctly. By equipping yourself with this knowledge, you’ll not only
Understanding Non-Static Member Functions
Non-static member functions are an essential component of object-oriented programming in C++. These functions are tied to an instance of a class and can access instance variables and other non-static member functions. However, to invoke a non-static member function, you must have an instance of the class. Attempting to call a non-static member function without an instance leads to the “invalid use of non-static member function” error.
When you declare a member function as non-static, you are indicating that it operates on instance data. This requires an implicit `this` pointer, which points to the object invoking the function. In contrast, static member functions do not require an instance and cannot access non-static members directly.
Common Causes of the Error
Several scenarios can lead to the “invalid use of non-static member function” error:
- Calling a non-static member function from a static context: Static functions or methods cannot access non-static members directly.
- Attempting to invoke a non-static member function without an object: You need to create an instance of the class before calling the member function.
- Using class scope resolution operator: Using the scope resolution operator (`::`) to call a non-static member function results in this error.
Examples of Error Situations
Here are some code snippets that illustrate when this error occurs:
“`cpp
class MyClass {
public:
void myFunction() {
// Function code here
}
static void myStaticFunction() {
myFunction(); // Error: invalid use of non-static member function
}
};
int main() {
MyClass::myFunction(); // Error: invalid use of non-static member function
}
“`
In the above examples, both instances illustrate attempts to call `myFunction` without an instance of `MyClass`.
How to Resolve the Error
To fix the “invalid use of non-static member function” error, consider the following approaches:
- Create an instance of the class: Ensure you have an object of the class before calling a non-static member function.
“`cpp
MyClass obj;
obj.myFunction(); // Correct usage
“`
- Convert the member function to static: If the function does not depend on instance-specific data, consider changing it to a static member function.
“`cpp
class MyClass {
public:
static void myFunction() {
// Function code here
}
};
“`
- Use an instance within a static function: If you must call a non-static function from a static context, create an instance of the class within that static function.
“`cpp
static void myStaticFunction() {
MyClass obj;
obj.myFunction(); // Correct usage
}
“`
Summary Table of Non-Static vs Static Member Functions
Feature | Non-Static Member Function | Static Member Function |
---|---|---|
Access to instance variables | Yes | No |
Access to other non-static members | Yes | No |
Called on class instance | Yes | No |
Called on class name | No | Yes |
Understanding Non-Static Member Functions
In C++, a non-static member function belongs to a specific instance of a class. This means that non-static member functions can access instance variables and other non-static member functions directly. The use of these functions requires an object of the class to be created.
Key characteristics include:
- Instance-Specific: Non-static member functions operate on the data of the specific instance.
- Access to `this` Pointer: Inside non-static member functions, the `this` pointer is automatically available, allowing access to the instance variables.
Common Scenarios Leading to Errors
The error message “invalid use of non-static member function” typically arises in specific contexts. Below are common scenarios that lead to this issue:
- Static Context: Attempting to call a non-static member function from a static context, such as within a static member function or a static method.
- No Object Instance: Invoking a non-static member function without creating an instance of the class.
- Incorrect Object Reference: Trying to call a non-static member function on a pointer or reference that is not properly initialized.
Examples of the Error
Consider the following example, which demonstrates the misuse of non-static member functions:
“`cpp
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
static void staticFunction() {
display(); // Error: invalid use of non-static member function
}
};
```
In this example, calling `display()` inside `staticFunction()` results in the error because `staticFunction()` is static and doesn't have access to instance members.
Correct Usage of Non-Static Member Functions
To avoid the “invalid use of non-static member function” error, ensure the following:
– **Create an Instance**: Always create an instance of the class before calling non-static member functions.
“`cpp
MyClass obj;
obj.display(); // Correct usage
“`
– **Use `this` Pointer**: When calling other non-static member functions from within a non-static member function, utilize the `this` pointer implicitly.
“`cpp
void anotherFunction() {
this->display(); // Correct usage
}
“`
Best Practices to Avoid Errors
To prevent encountering the “invalid use of non-static member function” error, consider the following best practices:
- Understand Static vs. Non-Static: Always differentiate between static and non-static contexts.
- Initialization: Ensure that all objects are properly instantiated before accessing their non-static members.
- Code Review: Regularly review code for static context misuse, particularly in team environments.
Best Practice | Description |
---|---|
Understand Context | Know when you are in a static or instance context. |
Initialize Objects | Always instantiate objects before use. |
Code Consistency | Maintain consistent coding practices to avoid confusion. |
Debugging Tips
When faced with this error, apply the following debugging strategies:
- Check Function Declaration: Confirm whether the function is declared as static or non-static.
- Review Object Instantiation: Verify that the object has been created before function calls.
- Inspect Call Sites: Look for the locations in code where the non-static member function is being invoked.
By following these guidelines, developers can effectively manage and utilize non-static member functions within their C++ applications, minimizing the occurrence of related errors.
Understanding the Invalid Use of Non-Static Member Functions
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “The invalid use of non-static member functions typically arises when developers attempt to invoke these functions without an instance of the class. This often leads to confusion, especially among those new to object-oriented programming. It is crucial to understand that non-static member functions rely on instance-specific data, which is not accessible in a static context.”
Michael Chen (Senior Developer, CodeCraft Solutions). “One common mistake is trying to call a non-static member function from a static context or another non-member function. This results in a compilation error, as the compiler cannot associate the function call with an object instance. Developers should ensure that they instantiate the class before making such calls to avoid these issues.”
Laura Patel (Lead Software Architect, FutureTech Labs). “Understanding the distinction between static and non-static members is fundamental in object-oriented programming. Non-static member functions are tied to specific instances of a class, and attempting to use them statically undermines the principles of encapsulation and object-oriented design. Properly managing object instances is essential for maintaining clean and functional code.”
Frequently Asked Questions (FAQs)
What does “invalid use of non-static member function” mean?
This error occurs when a non-static member function is called in a context where a static context is expected, such as within a static member function or without an instance of the class.
How can I fix the “invalid use of non-static member function” error?
To resolve this error, ensure that you call the non-static member function on an instance of the class rather than trying to call it directly from a static context.
What is the difference between static and non-static member functions?
Static member functions belong to the class itself and can be called without an instance, while non-static member functions require an instance of the class to be called, as they operate on instance-specific data.
Can I convert a non-static member function to static to avoid this error?
Yes, you can convert a non-static member function to static if it does not rely on instance-specific data. However, this may change the function’s behavior, so it should be done with caution.
Are there any scenarios where this error commonly occurs?
Common scenarios include attempting to call a non-static member function from within a static member function or trying to invoke it without creating an object of the class.
What are the implications of ignoring this error?
Ignoring this error can lead to compilation failures, preventing your program from running. It is essential to address it to ensure proper functionality and adherence to object-oriented principles.
The phrase “invalid use of non-static member function” typically arises in the context of object-oriented programming, particularly in languages like C++. This error occurs when a non-static member function is called in a context where the compiler expects a static context. Non-static member functions require an instance of the class to be invoked, while static member functions do not. Understanding the distinction between these two types of member functions is crucial for effective class design and implementation.
One of the primary reasons for encountering this error is the misuse of class member functions within static methods or in contexts where an object instance is not available. For instance, attempting to call a non-static member function directly from a static member function or from outside the class without an object reference will lead to this compilation error. This highlights the importance of correctly managing object instances and understanding the scope of member functions.
In summary, to avoid the “invalid use of non-static member function” error, developers should ensure that non-static member functions are always called on instances of their respective classes. This not only adheres to the principles of object-oriented programming but also promotes better coding practices by encouraging the proper encapsulation of data and behavior. Recognizing the differences between static and non-static members is essential for writing
Author Profile
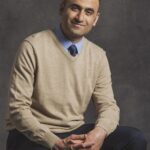
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?