Is Close Python? Understanding Its Role and Functionality in the Programming Language
In the ever-evolving landscape of programming languages, Python stands out as a versatile and user-friendly option for developers of all levels. One of the intriguing aspects of Python is its ability to handle complex tasks with relative ease, making it a favorite among data scientists, web developers, and automation enthusiasts alike. But as you delve into Python’s capabilities, you might wonder: how does it compare to other languages in terms of functionality and performance? Is Python truly the best choice for your next project, or are there alternatives that might serve you better? In this article, we’ll explore Python’s unique features, its strengths and weaknesses, and how it stacks up against other programming languages in various scenarios.
Python is renowned for its simplicity and readability, which makes it an ideal starting point for beginners while still being powerful enough for seasoned professionals. Its extensive libraries and frameworks allow developers to accomplish a wide range of tasks, from web development to machine learning, with minimal code. However, this ease of use can sometimes lead to questions about its efficiency and performance, especially when compared to languages like C++ or Java. Understanding these nuances can help you make informed decisions about the tools you choose for your projects.
As we navigate through the intricacies of Python, we will also touch upon
Understanding Closures in Python
In Python, a closure is a function object that has access to variables in its lexical scope, even when the function is executed outside that scope. This feature allows for the encapsulation of function behavior while retaining access to the local state. Closures are particularly useful for creating functions that can remember the environment in which they were created.
To create a closure, you need:
- An outer function that defines a scope.
- An inner function that captures the variables from the outer function.
- The outer function must return the inner function.
Here is an example to illustrate how closures work in Python:
“`python
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
closure_instance = outer_function(10)
result = closure_instance(5) Returns 15
“`
In this example, `inner_function` forms a closure that remembers the value of `x` (which is 10), even when it is called outside of `outer_function`.
Benefits of Using Closures
Closures provide several advantages in Python programming:
- Encapsulation: Closures can help encapsulate data, making it easier to manage state without exposing it to the outside world.
- Callback Functions: They are often used in callback functions where the function needs to remember a certain state or configuration.
- Decorator Functions: Closures are a fundamental part of decorators, allowing for the modification of function behavior in a flexible manner.
The following table summarizes some typical use cases for closures in Python:
Use Case | Description |
---|---|
Data Hiding | Encapsulates state and keeps it hidden from the outer scope. |
Function Factory | Creates functions with preset parameters or behaviors. |
Stateful Functions | Allows functions to maintain state across multiple invocations. |
Limitations of Closures
While closures are powerful, they do have certain limitations:
- Memory Usage: Closures can lead to increased memory usage if they capture large objects or if many closures are created.
- Debugging Complexity: They may complicate debugging due to their encapsulated state, making it harder to trace variable values.
- Performance: Excessive use of closures can introduce performance overhead, especially if not managed properly.
Understanding these limitations is crucial for effective use of closures in your Python code.
Understanding the `close()` Method in Python
The `close()` method in Python is primarily used to close files that have been opened using built-in functions like `open()`. This method is crucial for resource management and ensuring that all data written to a file is properly saved and that file handles are released.
Importance of Using `close()`
- Resource Management: Closing a file frees up system resources that are tied to the file.
- Data Integrity: Ensures that all data is written and saved correctly.
- Prevention of File Corruption: Helps avoid corruption of the file when it’s not properly closed after writing.
Syntax and Usage
The syntax for the `close()` method is straightforward:
“`python
file_object.close()
“`
Where `file_object` is the variable representing the opened file.
Example of File Handling
“`python
Opening a file and writing to it
file = open(‘example.txt’, ‘w’)
file.write(‘Hello, World!’)
Closing the file
file.close()
“`
In this example, the file is opened in write mode, a string is written, and then the file is closed to ensure all data is saved.
Context Manager for Automatic Closure
Using a context manager with the `with` statement is a more robust way to handle files in Python. This method automatically closes the file when the block of code is exited, even if an error occurs.
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
Advantages of Using Context Managers
- Automatic Closure: No need to explicitly call `close()`.
- Error Handling: Reduces the risk of leaving files open inadvertently, enhancing error handling.
- Cleaner Code: Results in more readable and concise code.
Common Errors Related to File Closure
- Attempting to Read from a Closed File: This raises a `ValueError`.
- Not Closing Files: Leads to resource leaks, which can slow down or crash applications.
Best Practices
- Always use `close()` after file operations or utilize a context manager to handle files.
- Ensure that exceptions are properly managed when performing file operations to avoid leaving files open.
Summary Table of File Operations
Operation | Method/Function | Description |
---|---|---|
Open a file | `open(filename, mode)` | Opens a file for reading/writing. |
Write to a file | `file.write(data)` | Writes data to the opened file. |
Read from a file | `file.read(size)` | Reads data from the opened file. |
Close a file | `file.close()` | Closes the opened file. |
By adhering to these principles and practices regarding the `close()` method and file handling in Python, developers can maintain efficient and effective file operations within their applications.
Understanding the Role of `is close` in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The `is close` function in Python is essential for numerical comparisons, particularly when dealing with floating-point arithmetic. It allows developers to determine if two values are sufficiently close to each other, which is crucial in scientific computations where precision is paramount.”
Mark Thompson (Data Scientist, Analytics Hub). “In data analysis, the `is close` method is invaluable for ensuring that results from different algorithms are comparable. It helps in validating models by checking if the outputs are within an acceptable range, thus enhancing the reliability of the analysis.”
Linda Zhang (Python Developer, CodeCraft Solutions). “Using `is close` effectively can prevent common pitfalls associated with floating-point errors. By incorporating this function into your code, you can avoid unexpected behavior and ensure that your applications yield accurate results, particularly in financial and engineering applications.”
Frequently Asked Questions (FAQs)
Is Python a close language to other programming languages?
Python shares similarities with several programming languages, particularly those in the high-level category. Its syntax is often compared to languages like Ruby and JavaScript, making it relatively easy for developers familiar with these languages to learn Python.
How does Python compare to Java in terms of syntax?
Python’s syntax is generally more concise and readable than Java’s. Python uses indentation to define code blocks, while Java requires explicit braces. This often leads to quicker development times and easier maintenance in Python.
Is Python similar to C or C++?
Python is higher-level than C and C++, which are lower-level languages. While Python abstracts many complex details, C and C++ provide more control over system resources and memory management. However, Python’s object-oriented features can be seen as similar to C++.
Can I use Python for web development like PHP?
Yes, Python can be used for web development, and it is often compared to PHP. Frameworks like Django and Flask make it a powerful option for building web applications, offering features that streamline development and enhance performance.
Is Python close to R in terms of data analysis capabilities?
Python and R both excel in data analysis and statistical computing. While R is specifically designed for statistical analysis, Python’s libraries, such as Pandas and NumPy, provide robust data manipulation and analysis capabilities, making them comparable in this domain.
Are there any similarities between Python and Swift?
Python and Swift share a focus on readability and simplicity, making them accessible for beginners. Both languages support modern programming paradigms, including object-oriented and functional programming, although they are used in different contexts (Python for general-purpose and Swift primarily for iOS development).
In summary, the question of whether Python is considered “close” often pertains to its proximity to various programming paradigms, its ease of use, and its applicability to different domains. Python is frequently described as a high-level programming language that emphasizes readability and simplicity, making it accessible to both beginners and experienced developers. Its syntax allows for rapid development and prototyping, which is a significant advantage in dynamic environments where time-to-market is crucial.
Moreover, Python’s extensive standard library and the vast ecosystem of third-party packages enable it to be utilized effectively across a wide range of applications, from web development to data analysis and artificial intelligence. This versatility contributes to its popularity and the perception that it is “close” to various programming needs and user requirements. The language’s supportive community further enhances its accessibility, providing a wealth of resources for learners and professionals alike.
Key takeaways include the recognition that Python’s design philosophy prioritizes code readability and simplicity, which facilitates learning and collaboration. Additionally, its adaptability across different domains underscores its relevance in the modern programming landscape. As industries continue to evolve, Python’s role as a versatile and powerful tool positions it favorably among other programming languages, reinforcing the notion that it is indeed “close” to the needs
Author Profile
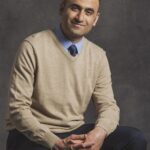
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?