Is an Empty Array Considered Falsy in JavaScript?
In the world of JavaScript, understanding how different data types behave can be both fascinating and perplexing. One area that often sparks debate among developers is the concept of “falsy” values—those that evaluate to in a boolean context. Among these, the empty array (`[]`) stands out as a particularly intriguing case. Does it follow the same rules as other falsy values, or does it possess unique characteristics that set it apart? In this article, we will dive deep into the nuances of empty arrays in JavaScript, exploring their behavior, implications, and the common misconceptions that surround them.
At first glance, it might seem intuitive to classify an empty array as a falsy value, akin to `null`, `undefined`, or `0`. However, JavaScript’s type coercion and truthiness evaluation reveal a more complex picture. While an empty array may appear devoid of content, its inherent nature as an object means it behaves differently in conditional statements. This distinction can lead to unexpected outcomes for developers who may assume that all empty structures are treated equally.
As we unravel the intricacies of empty arrays in JavaScript, we will examine how they interact with various operators and functions, the implications for control flow, and the potential pitfalls that can arise when making assumptions about
Understanding Falsy Values in JavaScript
In JavaScript, the concept of “falsy” values is crucial for understanding how the language evaluates expressions. Falsy values are those that evaluate to “ when coerced to a Boolean context. This includes:
- “
- `0`
- `””` (empty string)
- `null`
- `undefined`
- `NaN`
When evaluating conditions, if a value is falsy, it will be treated as “ in conditional statements.
Arrays and Their Truthiness
Arrays in JavaScript, even when empty, are considered truthy. This means that an empty array (`[]`) will evaluate to `true` in Boolean contexts, contrary to common misconceptions. Therefore, an empty array is not falsy.
To illustrate this behavior, consider the following examples:
javascript
let emptyArray = [];
let nonEmptyArray = [1, 2, 3];
console.log(Boolean(emptyArray)); // true
console.log(Boolean(nonEmptyArray)); // true
In both cases, the output is `true`, demonstrating that arrays, regardless of their contents, are truthy.
Comparative Evaluation of Different Types
To further clarify the distinction between falsy values and an empty array, the following table summarizes the evaluation of various data types in JavaScript:
Value | Type | Boolean Evaluation |
---|---|---|
Boolean | ||
0 | Number | |
“” | String | |
null | Object | |
undefined | Undefined | |
NaN | Number | |
[] | Array | true |
[1, 2, 3] | Array | true |
Practical Implications
Understanding that an empty array is truthy is vital for effective coding practices in JavaScript. This has practical implications in various scenarios, such as:
- Conditional Statements: When checking for the existence of elements in an array, remember to use methods like `.length` or `.some()` rather than relying on the array itself.
javascript
if (emptyArray.length === 0) {
console.log(“The array is empty.”);
}
- Logical Operations: When performing logical operations, be cautious about the behavior of arrays.
The truthiness of an empty array can lead to unexpected results in conditions or loops, emphasizing the importance of understanding these nuances in JavaScript.
Understanding Truthiness in JavaScript
In JavaScript, values are categorized as either “truthy” or “falsy” based on how they evaluate in a boolean context. This concept is essential when working with conditional statements and logical operations.
Falsy Values in JavaScript
JavaScript defines specific values that are considered falsy. These include:
- “
- `0` (zero)
- `-0` (negative zero)
- `””` (empty string)
- `null`
- `undefined`
- `NaN` (Not-a-Number)
These values will evaluate to “ in a boolean context, such as within `if` statements or logical operations.
Are Empty Arrays Falsy?
An empty array (`[]`) is not considered a falsy value in JavaScript. Instead, it is classified as a truthy value. This can lead to unexpected behaviors if one assumes that an empty array behaves similarly to other falsy values.
Examples:
- If Statement:
javascript
if ([]) {
console.log(“This will execute.”); // This will be printed
}
- Boolean Conversion:
javascript
console.log(Boolean([])); // Outputs: true
In both examples, the empty array evaluates to `true`, demonstrating its truthiness.
Comparison with Other Data Types
To better understand how empty arrays compare to other falsy values, consider the following table:
Value | Type | Falsy/Truthy |
---|---|---|
“ | Boolean | Falsy |
`0` | Number | Falsy |
`””` | String | Falsy |
`null` | Null | Falsy |
`undefined` | Undefined | Falsy |
`NaN` | Number | Falsy |
`[]` | Object (Array) | Truthy |
As illustrated, empty arrays stand out as truthy, contrasting sharply with the established falsy values.
Practical Implications
The distinction between empty arrays and falsy values can have practical implications in coding practices:
- Conditional Logic: When checking if an array is empty, use methods like `.length` to determine its content:
javascript
const arr = [];
if (arr.length === 0) {
console.log(“Array is empty.”); // Correct way to check for emptiness
}
- Type Checking: Be aware of the truthy nature of arrays, especially when integrating with functions that expect boolean inputs.
- Data Structures: In scenarios involving data structures, understanding the truthiness of arrays allows for more accurate condition evaluations.
By recognizing that empty arrays are truthy, developers can avoid logical errors and write more precise JavaScript code.
Understanding the Falsiness of Empty Arrays in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, CodeCraft Solutions). “In JavaScript, an empty array is not considered falsy. It is an object and thus evaluates to true in a boolean context. This can lead to unexpected behavior if developers assume it is falsy like null or undefined.”
Michael Chen (Lead Software Engineer, Tech Innovations Inc.). “The confusion often arises because while an empty array evaluates to true, its length property is zero. This means that checking for the presence of elements should be done using the array’s length rather than relying on its truthiness.”
Laura Kim (JavaScript Educator, Web Development Academy). “It’s crucial for developers to understand that all arrays in JavaScript, regardless of their contents, are truthy. This distinction helps prevent logical errors when performing conditional checks in code.”
Frequently Asked Questions (FAQs)
Is an empty array considered falsy in JavaScript?
No, an empty array is not considered falsy in JavaScript. It is treated as a truthy value.
What are the falsy values in JavaScript?
The falsy values in JavaScript include “, `0`, `-0`, `0n`, `””` (empty string), `null`, `undefined`, and `NaN`.
How can I check if an array is empty in JavaScript?
You can check if an array is empty by evaluating its length property. For example, `array.length === 0` returns true if the array is empty.
What is the difference between truthy and falsy values in JavaScript?
Truthy values evaluate to true in a boolean context, while falsy values evaluate to . This distinction affects how conditions are evaluated in control structures.
Can an empty array be used in conditional statements?
Yes, an empty array can be used in conditional statements. However, it will evaluate to true, leading to the execution of the code block intended for truthy values.
Why might someone think an empty array is falsy?
Some may confuse the concept due to the behavior of other empty data structures, like empty strings or null values, which are falsy. However, arrays, even when empty, are objects and thus truthy.
In JavaScript, an empty array is considered a truthy value. This means that when evaluated in a boolean context, such as within an if statement, an empty array will not be treated as . Instead, it will evaluate to true. This behavior can often lead to confusion among developers, especially those who may expect an empty array to behave similarly to other empty data structures, such as an empty string or null, which are indeed falsy.
Understanding the truthy nature of an empty array is crucial for effective programming in JavaScript. Developers should be aware that while the array contains no elements, it is still an object and thus evaluates to true. This characteristic is important when performing conditional checks or iterations, as it can affect the flow of logic in a program. Consequently, developers should take care when writing conditions that involve arrays to avoid unintended outcomes.
In summary, the distinction between truthy and falsy values in JavaScript is essential for writing robust code. An empty array, despite being devoid of elements, remains a truthy value. This insight underscores the importance of understanding JavaScript’s type coercion and boolean evaluation rules, which can significantly impact the behavior of code and the handling of data structures.
Author Profile
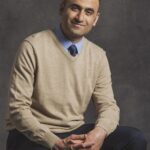
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?