Is JavaScript Case Sensitive? Understanding the Importance of Case in Coding
### Is JavaScript Case Sensitive?
In the ever-evolving landscape of web development, JavaScript stands out as one of the most widely used programming languages. Whether you’re a seasoned developer or just starting your coding journey, understanding the nuances of JavaScript is crucial for writing effective and error-free code. One fundamental aspect that often raises questions among developers is case sensitivity. This seemingly simple concept can have significant implications for how code is written and executed, making it an essential topic to explore.
At its core, case sensitivity in programming languages refers to how the language treats uppercase and lowercase letters. In JavaScript, this characteristic plays a pivotal role in variable names, function declarations, and even built-in object properties. A single misstep in capitalization can lead to unexpected errors or, worse, bugs that are difficult to trace. As we delve deeper into this topic, we will uncover how JavaScript’s case sensitivity affects coding practices, best practices for naming conventions, and tips for avoiding common pitfalls.
Understanding whether JavaScript is case sensitive is not just an academic inquiry; it has real-world implications for developers. By grasping this concept, you can enhance your coding skills, improve collaboration with other developers, and ultimately write cleaner, more efficient code. Join us as we break down this essential aspect
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive programming language, which means that it distinguishes between uppercase and lowercase letters in identifiers, keywords, and other language constructs. This characteristic is crucial for developers to understand, as it impacts how variables, functions, and other elements are defined and referenced within the code.
When defining variables, for example, the following are considered distinct identifiers:
- `myVariable`
- `MyVariable`
- `MYVARIABLE`
Each of these identifiers can hold different values and represent different entities within the code. Consequently, using consistent casing throughout your code is essential for maintaining clarity and avoiding errors.
Key Concepts of Case Sensitivity
There are several key concepts related to case sensitivity in JavaScript:
- Variable Names: Variable names are case-sensitive. `count` and `Count` would be treated as two separate variables.
- Function Names: Function declarations and expressions are also case-sensitive. Calling a function with the wrong case will result in an error.
- Object Properties: Properties of objects respect case sensitivity as well. Accessing an object property with the incorrect case leads to `undefined`.
Identifier Type | Example | Case Sensitivity |
---|---|---|
Variable | let number = 10; | number vs Number |
Function | function myFunction() {} | myFunction vs MyFunction |
Object Property | const obj = { key: ‘value’ }; | obj.key vs obj.Key |
Best Practices for Managing Case Sensitivity
To effectively manage case sensitivity in JavaScript, developers should adhere to certain best practices:
- Consistent Naming Conventions: Use a consistent naming convention throughout your codebase, such as camelCase for variables and functions, and PascalCase for classes.
- Avoiding Ambiguity: Refrain from using similar names that differ only in case, as this can lead to confusion and potential bugs.
- Code Reviews: Conduct regular code reviews to ensure that naming conventions are followed and to catch case-related issues early in the development process.
By understanding and respecting the case sensitivity of JavaScript, developers can write cleaner, more efficient, and less error-prone code.
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive programming language, meaning that it distinguishes between uppercase and lowercase letters in variable names, function names, and other identifiers. This characteristic is essential for developers to understand to avoid errors and ensure code behaves as expected.
Examples of Case Sensitivity
To illustrate case sensitivity, consider the following examples:
- Variable declarations:
javascript
let myVariable = 10;
let MyVariable = 20;
console.log(myVariable); // Outputs: 10
console.log(MyVariable); // Outputs: 20
- Function names:
javascript
function myFunction() {
return “Hello”;
}
function MyFunction() {
return “World”;
}
console.log(myFunction()); // Outputs: Hello
console.log(MyFunction()); // Outputs: World
As seen in these examples, `myVariable` and `MyVariable` are treated as distinct identifiers.
Common Mistakes Due to Case Sensitivity
Developers often encounter issues stemming from case sensitivity. Here are some common mistakes:
- Inconsistent Naming: Using different cases for the same variable.
- Typographical Errors: Misspelling a function or variable name by altering its case.
- Library Function Calls: Miscalling built-in functions or methods due to case discrepancies (e.g., using `array.push` instead of `Array.push`).
Best Practices for Handling Case Sensitivity
To mitigate issues related to case sensitivity, developers can follow these best practices:
- Consistent Naming Conventions: Adopt a naming convention (e.g., camelCase or snake_case) and stick to it throughout the codebase.
- Code Reviews: Regularly conduct code reviews to catch case-related errors.
- Use Linting Tools: Implement linting tools that can identify inconsistencies in variable and function naming.
Case Sensitivity in JavaScript Objects
Case sensitivity also applies to properties within JavaScript objects. Each property name is case-sensitive, and accessing a property using the incorrect case will yield `undefined`.
Example:
javascript
const person = {
name: “Alice”,
age: 30
};
console.log(person.name); // Outputs: Alice
console.log(person.Name); // Outputs: undefined
Comparison with Other Languages
Language | Case Sensitive |
---|---|
JavaScript | Yes |
Python | No |
C++ | Yes |
Java | Yes |
Ruby | No |
Understanding case sensitivity is crucial for effective programming in JavaScript, as it not only affects naming conventions but also the overall functionality of the code.
Understanding JavaScript’s Case Sensitivity from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “JavaScript is indeed case sensitive, which means that variable names, function names, and other identifiers must be used consistently in terms of casing. For instance, ‘Variable’ and ‘variable’ would be treated as two distinct identifiers, which can lead to significant bugs if not properly managed.”
Michael Chen (Lead Developer, Web Solutions Group). “The case sensitivity of JavaScript is a fundamental aspect that new developers often overlook. It is crucial to maintain a consistent naming convention throughout your codebase to avoid confusion and errors, especially in larger projects where multiple developers are involved.”
Sarah Patel (JavaScript Instructor, Code Academy). “Understanding that JavaScript is case sensitive helps learners grasp the importance of precision in coding. It is not just a minor detail; it can affect the functionality of the program. Therefore, teaching this concept early on can prevent many common pitfalls for beginners.”
Frequently Asked Questions (FAQs)
Is JavaScript case sensitive?
Yes, JavaScript is case sensitive. This means that variables, function names, and other identifiers must be used consistently in terms of uppercase and lowercase letters. For example, `myVariable` and `MyVariable` would be treated as two distinct identifiers.
What are the implications of JavaScript’s case sensitivity?
The case sensitivity of JavaScript can lead to errors if developers mistakenly use different cases for the same identifier. It is essential to maintain consistent naming conventions to avoid confusion and potential bugs in the code.
Are all programming languages case sensitive?
No, not all programming languages are case sensitive. For instance, languages like SQL and HTML are generally case insensitive, while languages such as Java, C++, and Python, like JavaScript, are case sensitive.
How does case sensitivity affect variable naming conventions in JavaScript?
Case sensitivity necessitates careful consideration of variable naming conventions in JavaScript. Developers often adopt camelCase or snake_case styles to ensure clarity and consistency, minimizing the risk of errors.
Can I use the same name with different cases for variables in JavaScript?
Yes, you can use the same name with different cases for variables in JavaScript. However, it is not recommended, as it can lead to confusion and make the code harder to read and maintain.
What should I do if I encounter case sensitivity issues in my JavaScript code?
If you encounter case sensitivity issues, carefully review your code to ensure that all identifiers are used consistently. Refactoring variable names to adhere to a single naming convention can help resolve these issues effectively.
JavaScript is indeed a case-sensitive programming language. This means that it distinguishes between uppercase and lowercase letters in variable names, function names, and other identifiers. For instance, the variable names `myVariable`, `MyVariable`, and `MYVARIABLE` would be treated as three distinct entities within the same scope. This characteristic is crucial for developers to understand, as it can lead to errors if not properly accounted for in coding practices.
Furthermore, the case sensitivity of JavaScript extends to its built-in functions and objects. For example, the method `getElementById` must be called with the exact casing; using variations like `getelementbyid` or `GETELEMENTBYID` would result in a reference error. This reinforces the importance of consistency in naming conventions and adherence to the language’s syntax rules to avoid potential bugs and enhance code readability.
In summary, recognizing that JavaScript is case-sensitive is fundamental for effective programming in this language. Developers should cultivate a disciplined approach to naming conventions and be vigilant about the casing of identifiers to ensure their code functions correctly. By doing so, they can minimize errors and improve the maintainability of their codebases.
Author Profile
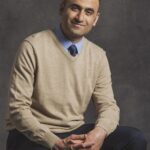
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?