Is JavaScript Case Sensitive? Unraveling the Mystery Behind Variable Names!
### Introduction
In the world of programming, attention to detail can make or break your code. Among the many languages that developers use, JavaScript stands out for its versatility and widespread application in web development. However, one fundamental aspect that every programmer must grasp is the concept of case sensitivity. Understanding whether JavaScript is case sensitive can significantly impact how you write and debug your code. In this article, we will delve into the nuances of case sensitivity in JavaScript, exploring its implications for variable names, function calls, and more, ensuring you have a solid grasp of this essential programming principle.
### Overview
JavaScript, like many programming languages, treats identifiers—such as variable names and function names—as case sensitive. This means that the language distinguishes between uppercase and lowercase letters, making `Variable`, `variable`, and `VARIABLE` three distinct identifiers. This characteristic can lead to both powerful coding practices and potential pitfalls for developers, especially those who are new to the language or transitioning from case-insensitive languages.
The implications of case sensitivity extend beyond mere naming conventions; they influence how you structure your code and interact with libraries and frameworks. A small oversight, such as miscapitalizing a variable name, can lead to frustrating errors that are often difficult to trace. As we explore the intric
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive programming language, which means that it distinguishes between uppercase and lowercase letters. This characteristic is critical to the way variables, functions, and other identifiers are defined and accessed within the code.
For example, the identifiers `myVariable`, `MyVariable`, and `MYVARIABLE` would be considered three distinct variables. This can lead to errors if a developer mistakenly uses the wrong casing when referencing a variable or function.
Key Implications of Case Sensitivity
The case sensitivity of JavaScript has several important implications for developers:
- Variable Declaration: When declaring variables, the exact casing must be used when referencing them throughout the code.
- Function Names: Similarly, function names are case-sensitive, and any deviation in casing can lead to runtime errors or unexpected behavior.
- Object Properties: Properties of objects are also case-sensitive. Accessing an object property with incorrect casing will result in `undefined`.
Examples of Case Sensitivity
To illustrate the importance of case sensitivity, consider the following code snippet:
javascript
let myVariable = 10;
console.log(myvariable); // Output: undefined
console.log(MyVariable); // Output: ReferenceError: MyVariable is not defined
In this example, attempting to access `myvariable` or `MyVariable` results in either `undefined` or a reference error, demonstrating how case differences impact variable access.
Common Mistakes Due to Case Sensitivity
Developers often encounter issues due to case sensitivity, including:
- Typographical Errors: Simple typos can lead to undefined variables or functions.
- Inconsistent Naming Conventions: Using different casing styles for similar identifiers can create confusion and bugs in the code.
To help avoid these pitfalls, consider the following best practices:
- Consistent Naming Conventions: Adopt a consistent style for naming variables and functions, such as camelCase or snake_case.
- Code Reviews: Regularly review code with peers to catch case-related errors early in the development process.
Case Sensitivity in Data Structures
When dealing with data structures, such as arrays and objects, case sensitivity remains a critical factor. The following table summarizes the case sensitivity of various JavaScript data structures:
Data Structure | Case Sensitivity | Example |
---|---|---|
Variable | Yes | let myVar = 5; (myVar vs myvar) |
Function | Yes | function myFunction() {} (myFunction vs myfunction) |
Object Property | Yes | let obj = {name: “John”}; (obj.name vs obj.Name) |
Array Element | No | let arr = [1, 2, 3]; (arr[0] vs arr[1]) |
In summary, understanding the case sensitivity of JavaScript is essential for writing effective and error-free code. By adhering to consistent naming conventions and being vigilant about casing, developers can minimize the risk of errors related to this aspect of the language.
Understanding Case Sensitivity in JavaScript
JavaScript is a case-sensitive programming language, which means that it distinguishes between uppercase and lowercase letters in identifiers. This characteristic is crucial for developers to understand as it affects variable names, function names, and object properties.
Implications of Case Sensitivity
The implications of case sensitivity in JavaScript can lead to errors if not properly managed. Here are some key points to consider:
- Variable Names: A variable named `myVariable` is different from `MyVariable` and `MYVARIABLE`. Each name refers to a distinct variable.
- Function Names: Similarly, functions such as `calculateSum()` and `calculatesum()` are treated as separate entities. Calling one will not invoke the other.
- Object Properties: Object properties are also case-sensitive, meaning that accessing `object.property` is different from `object.Property`.
Common Errors Due to Case Sensitivity
Developers often encounter errors related to case sensitivity. Common mistakes include:
- Misspelling Variable Names:
- Using `userName` instead of `username` can lead to undefined errors.
- Inconsistent Function Calls:
- Calling `getData()` instead of `getdata()` will result in a `ReferenceError`.
- Accessing Object Properties:
- Attempting to access `person.Age` when the actual property is defined as `person.age`.
Best Practices to Avoid Case Sensitivity Issues
To minimize the risk of case sensitivity errors, consider the following best practices:
- Consistent Naming Conventions:
- Use a consistent naming convention such as camelCase for variables and functions.
- Code Reviews:
- Implement regular code reviews to catch case sensitivity issues early.
- Linting Tools:
- Utilize tools like ESLint to enforce naming conventions and catch potential mistakes.
Case Sensitivity in JavaScript Libraries
JavaScript libraries and frameworks also adhere to case sensitivity. For instance:
Library/Framework | Case Sensitivity Example |
---|---|
jQuery | `$(document).ready()` vs. `$(Document).ready()` |
React | ` |
Angular | `NgModule` vs. `ngmodule` |
Understanding how case sensitivity affects these libraries can prevent integration issues and enhance code reliability.
Awareness of JavaScript’s case sensitivity is essential for effective coding practices. By adhering to consistent naming conventions, utilizing code reviews, and leveraging linting tools, developers can significantly reduce errors and improve code quality.
Understanding Case Sensitivity in JavaScript: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “JavaScript is indeed case sensitive, which means that variable names, function names, and object properties must be used consistently with their defined casing. This characteristic is crucial for developers to understand, as it can lead to unexpected errors if not properly adhered to.”
Michael Tran (Lead JavaScript Developer, CodeCraft Solutions). “In JavaScript, ‘Variable’ and ‘variable’ are treated as two distinct identifiers. This case sensitivity is a fundamental aspect of the language that reinforces the importance of precise coding practices and can significantly impact debugging processes.”
Sarah Johnson (JavaScript Educator, Digital Learning Academy). “Understanding that JavaScript is case sensitive is essential for beginners. It emphasizes the need for consistency in naming conventions, which not only helps in avoiding errors but also enhances code readability and maintainability.”
Frequently Asked Questions (FAQs)
Is JavaScript case sensitive?
Yes, JavaScript is case sensitive. This means that variable names, function names, and other identifiers must be used consistently in terms of uppercase and lowercase letters.
What are the implications of JavaScript being case sensitive?
The case sensitivity of JavaScript requires developers to be careful when naming variables and functions. For example, `myVariable` and `myvariable` would be treated as two distinct identifiers.
Are all programming languages case sensitive?
No, not all programming languages are case sensitive. For instance, languages like SQL are typically case insensitive, while others like C, C++, and Java, similar to JavaScript, are case sensitive.
How can I avoid errors related to case sensitivity in JavaScript?
To avoid errors, consistently use a naming convention, such as camelCase or snake_case, and be diligent in checking the case of identifiers throughout your code.
Does case sensitivity affect JavaScript libraries and frameworks?
Yes, case sensitivity affects libraries and frameworks in JavaScript. When importing modules or referencing functions, the exact case must match the defined names to avoid runtime errors.
Can case sensitivity lead to security vulnerabilities in JavaScript?
While case sensitivity itself does not directly lead to security vulnerabilities, it can contribute to bugs or unintended behavior if developers mistakenly use different cases for the same identifier, potentially leading to exploitable code.
JavaScript is indeed a case-sensitive programming language. This means that it differentiates between uppercase and lowercase letters in variable names, function names, and other identifiers. For instance, the variables ‘myVariable’ and ‘MyVariable’ would be treated as distinct entities. This characteristic is crucial for developers to understand, as it can lead to errors if not properly accounted for in code.
The case sensitivity of JavaScript extends to its built-in objects and methods as well. For example, the method ‘getElementById’ must be written with the exact casing; any deviation, such as ‘getelementbyid’ or ‘GetElementById’, will result in a runtime error. This reinforces the importance of adhering to the correct casing conventions when writing JavaScript code.
Furthermore, this feature of JavaScript emphasizes the need for consistency in naming conventions throughout a codebase. Adopting a clear and consistent style, such as camelCase for variable names, can help prevent confusion and reduce the likelihood of errors. Developers should be vigilant about case sensitivity, especially when collaborating with others or working on larger projects.
understanding that JavaScript is case-sensitive is fundamental for effective programming in the language. Developers must pay
Author Profile
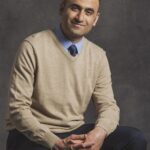
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?