Is a List Mutable in Python? Exploring the Flexibility of Python Lists
In the world of programming, understanding the nature of data structures is crucial for writing efficient and effective code. Among the many data types available in Python, lists stand out for their versatility and ease of use. But what does it mean for a data structure to be mutable, and how does this characteristic influence the way we interact with lists in Python? If you’ve ever wondered about the inner workings of lists and how they can be manipulated, you’re in the right place. This article will delve into the concept of mutability, specifically focusing on lists in Python, and explore the implications of this feature in your coding practices.
Lists in Python are a fundamental data structure that allows for the storage of an ordered collection of items. One of their most defining traits is mutability, which means that you can change, add, or remove elements from a list after it has been created. This flexibility is a double-edged sword; while it enables dynamic data handling, it also requires careful management to avoid unintended side effects. Understanding how mutability works in lists can significantly enhance your programming skills and help you write more robust code.
As we explore the mutability of lists, we will examine how this characteristic affects various operations, such as indexing, slicing, and modifying elements. Additionally, we
Understanding Mutability in Python Lists
In Python, a list is a mutable data structure, meaning that it can be modified after its creation. This mutability allows for various operations that can change the contents of the list without creating a new list.
The characteristics of mutable objects include:
- In-place Modification: You can change the elements of the list directly.
- Dynamic Sizing: Lists can grow or shrink in size as elements are added or removed.
- Support for Various Data Types: Lists can contain elements of different types, including other lists.
To illustrate the mutability of lists, consider the following example:
“`python
my_list = [1, 2, 3]
my_list[0] = 4 Modifying the first element
print(my_list) Output: [4, 2, 3]
“`
In this example, the first element of `my_list` is changed from `1` to `4`, demonstrating that lists can be altered without creating a new instance.
Common List Operations
Several operations highlight the mutability of lists:
- Appending Elements: Add an element to the end of the list.
- Inserting Elements: Place an element at a specific position in the list.
- Removing Elements: Delete an element by value or index.
Here’s a brief overview of these operations:
Operation | Method | Description |
---|---|---|
Append | list.append(value) | Adds `value` to the end of the list. |
Insert | list.insert(index, value) | Adds `value` at the specified `index`. |
Remove | list.remove(value) | Removes the first occurrence of `value` from the list. |
Pop | list.pop(index) | Removes and returns the element at the specified `index`. |
Each of these methods modifies the list in place, reinforcing the concept of mutability.
Comparing Mutable and Immutable Types
In Python, data types are categorized as mutable or immutable. Understanding the difference can clarify the behavior of lists versus other data types like tuples or strings, which are immutable.
Mutable Types:
- Lists
- Dictionaries
- Sets
Immutable Types:
- Tuples
- Strings
- Frozensets
The main distinctions include:
- Modification: Mutable types can be changed; immutable types cannot.
- Memory Usage: Mutable types can be more memory efficient as they allow in-place modifications.
This difference affects how you use these types in your programs. For instance, when passing a mutable object to a function, changes to the object will persist outside the function, while changes to an immutable object will not.
the mutability of lists in Python provides a flexible approach to handling collections of items, allowing for efficient updates and management of data. Understanding how to leverage this mutability is crucial for effective programming in Python.
Understanding Mutability in Python Lists
In Python, a list is a mutable data structure, meaning that its contents can be changed after it has been created. This mutability allows for a variety of operations to be performed on lists, making them highly versatile for programming tasks. The following points outline the key characteristics and behaviors of mutable lists in Python:
- Modification of Elements: Individual elements within a list can be changed by accessing their index. For example:
“`python
my_list = [1, 2, 3]
my_list[1] = 5 Changes the second element to 5
“`
- Adding Elements: New elements can be appended to the end of a list using methods such as `append()` and `extend()`. For instance:
“`python
my_list.append(4) Appends 4 to the end of the list
my_list.extend([5, 6]) Extends the list with multiple elements
“`
- Removing Elements: Elements can be removed using methods like `remove()` and `pop()`. For example:
“`python
my_list.remove(2) Removes the first occurrence of 2
my_list.pop() Removes the last element and returns it
“`
- Slicing: Lists can be sliced to create sublists. This does not modify the original list but provides a new list based on specified indices:
“`python
sub_list = my_list[1:3] Creates a sublist from index 1 to index 2
“`
Comparison with Immutable Structures
Understanding the difference between mutable and immutable data types in Python is crucial. Immutable structures, such as tuples and strings, cannot be altered once created. Here’s a comparison to highlight these differences:
Feature | Mutable (List) | Immutable (Tuple/String) |
---|---|---|
Modification | Allowed | Not allowed |
Methods | append(), remove(), etc. | N/A |
Performance | Slower due to flexibility | Faster due to immutability |
Use Cases | Dynamic collections | Fixed collections |
Practical Use Cases of Mutable Lists
The mutability of lists lends itself to various practical applications:
- Dynamic Data Storage: Lists are ideal for scenarios where the number of elements can change, such as user input or dynamically generated data.
- Data Manipulation: They enable complex data manipulations, such as sorting and filtering, using built-in methods.
- Collecting Results: Lists can be used to collect results in iterative processes, such as loops or comprehensions, simplifying the accumulation of output.
“`python
results = []
for i in range(5):
results.append(i * 2) Collects the doubled values
“`
- Data Structures: Lists can serve as building blocks for more complex data structures like stacks and queues due to their flexible nature.
Conclusion on List Mutability
The mutability of lists in Python significantly enhances their functionality, making them essential for a wide range of programming tasks. Understanding and leveraging this characteristic allows developers to create dynamic and efficient applications.
Understanding the Mutability of Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, lists are indeed mutable, meaning that their contents can be changed after the list has been created. This characteristic allows developers to modify, add, or remove elements without needing to create a new list, which is a fundamental aspect of Python’s flexibility and efficiency in handling data.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “The mutability of lists in Python is a crucial feature that distinguishes them from tuples, which are immutable. This property enables programmers to efficiently manage collections of data, making lists an ideal choice for scenarios where dynamic data manipulation is required.”
Sarah Thompson (Data Scientist, Analytics Hub). “Understanding that lists are mutable is essential for Python developers. This allows for in-place modifications, which can significantly enhance performance when dealing with large datasets. However, it also necessitates careful consideration of side effects when passing lists to functions.”
Frequently Asked Questions (FAQs)
Is a list mutable in Python?
Yes, lists in Python are mutable, meaning their elements can be changed, added, or removed after the list has been created.
How can I modify a list in Python?
You can modify a list using methods such as `append()`, `extend()`, `insert()`, `remove()`, and `pop()`, which allow you to add or remove elements.
What happens if I try to change an element in a tuple?
Tuples are immutable in Python, so attempting to change an element will result in a `TypeError`.
Can I create a list of tuples in Python?
Yes, you can create a list that contains tuples as its elements, allowing for a combination of mutable and immutable data structures.
Are there any performance implications of using mutable lists?
Yes, mutable lists can lead to performance issues in certain scenarios, especially when copying or passing them to functions, as changes in one reference affect all references to the list.
How do I check if a list is mutable in Python?
You can check if an object is mutable by attempting to change its content or by using the `id()` function to see if the object’s identity remains the same after modification.
In Python, lists are indeed mutable, meaning that their contents can be modified after the list has been created. This mutability allows for a range of operations, such as adding, removing, or changing elements within the list without the need to create a new list. This characteristic is fundamental to how lists are utilized in Python programming, enabling dynamic data manipulation and efficient memory usage.
Moreover, the mutability of lists distinguishes them from other data types in Python, such as tuples, which are immutable. This difference is significant when considering the appropriate data structure for a specific task. Lists provide greater flexibility for scenarios that require frequent updates, while tuples offer stability and protection against accidental changes.
Key takeaways from the discussion on list mutability include the importance of understanding how this feature impacts performance and functionality in Python. Programmers can leverage the mutable nature of lists to create more efficient algorithms and data management strategies. Additionally, recognizing when to use mutable versus immutable types can lead to better code practices and improved program reliability.
Author Profile
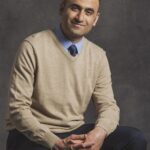
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?